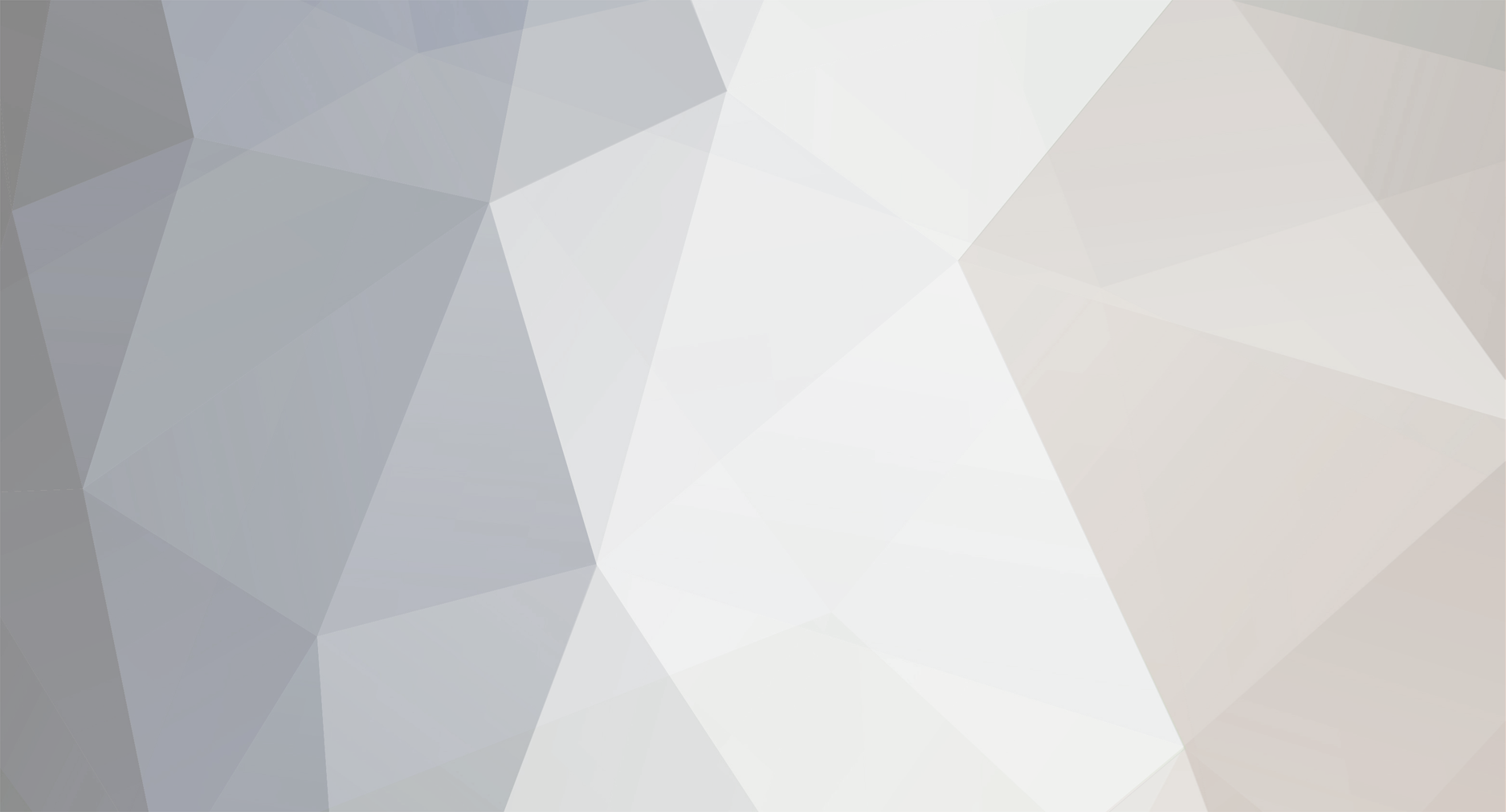
Philip
Staff Alumni-
Posts
4,665 -
Joined
-
Last visited
-
Days Won
20
Everything posted by Philip
-
If / Else Multiple conditions? Is this possible?
Philip replied to Skipjackrick's topic in PHP Coding Help
As should the next if statement I just don't understand why you're pulling stuff from a database, and then setting the result array to another value: $results[3]['angler'] = 0; -
If / Else Multiple conditions? Is this possible?
Philip replied to Skipjackrick's topic in PHP Coding Help
Umm, what exactly are you trying to do with your script? -
Well, technically, isn't mysql_numrows() deprecated? Anyways, change: $result = mysql_query($q); to: $result = mysql_query($q) or die(mysql_error());
-
mysql_num_rows, perhaps?
-
You should define the variable. Where do you currently create $_SESSION['login']?
-
So, you only have one session variable set, and that's $_SESSION['SESS_LOGGEDIN']
-
$slogin = $_SESSION['login']; It means there is no index in the array with the key of login. In otherwords, $_SESSION['login'] doesn't exist. Under session_start(); put: echo '<pre>'; print_r($_SESSION); echo '</pre>';
-
Have you tried placing memory_get_usage() in places you think might consume a lot of memory?
-
$path="C:\Program Files\Apache Software Foundation\Apache2.2\htdocs\upload\". " " .$_FILES["file"]["name"]. " " .$tp =$_FILES["file"]["type"]; Maybe you can see what it's doing that with some highlighting $path="C:\\Program Files\\Apache Software Foundation\\Apache2.2\\htdocs\\upload\\".$_FILES["file"]["name"].$tp.$_FILES["file"]["type"]; You needed to escape the quotes
-
I'm sorry, mysql_free_result(); should be: mysql_free_result($result); I also figured that the script would take longer than 60 seconds, again:
-
<?php $gold = '<td class="td" align="Right">968.00</td>'; preg_match_all('~<td class="td" align="Right">(.+?)</td>~is', $gold, $goldMatch); echo str_replace('<td class="td" align="Right">(.+?)</td>', '(.+?)', $goldMatch[0][0]); ?> Outputs 968.00
-
What is the value of $row['sort'] show?
-
edit, you got the image working. Anyways, the questions appearing after one is answered can be done with plain old javascript, unless you want them saved after the answer the question (then that would become ajax.) I'd recommend using radio buttons instead of checkboxes for yes/no questions, so they can only select one option.
-
It happens to the best of us
-
I don't understand why you're having trouble with that, when the rest of your code does it just fine. echo '<td width="10%" valign="top" bgcolor="'.$bg_color.'"><a href="contact_edit.php?id='.$rows['id'].'">Update</a>';
-
// Technically, you won't need trim() if(trim($_POST['age']) < 18) { echo '{lease Download the parental consent form <a target="_blank" href="www.wokinghambikeathon.co.uk/documents/parentalconsentform.pdf">here</a><br>then click <a href="www.wokinghambikeathon.co.uk/register2.php">here</a> to continue'; // I find it easiest to use single quotes on echo and double quotes in my html. // it requires less escaping }else{ header("Location: http://www.wokinghambikeathon.co.uk/register2.php"); ?> Should be: // Technically, you won't need trim() if(trim($_POST['age']) < 18) { echo 'Please Download the parental consent form <a target="_blank" href="www.wokinghambikeathon.co.uk/documents/parentalconsentform.pdf">here</a><br>then click <a href="www.wokinghambikeathon.co.uk/register2.php">here</a> to continue'; // I find it easiest to use single quotes on echo and double quotes in my html. // it requires less escaping }else{ header("Location: http://www.wokinghambikeathon.co.uk/register2.php"); } // you were missing this! ?> Edit: fixed typo with { instead of P
-
Are you on shared hosting? - If so, you might have used too many resources, and your host shut you off.
-
Of course, this is untested, and I'm sure someone will come up with a better way, but yeah it should you get you started. <?php // put at top: set_time_limit(60); // that will allow for 60 seconds of execution, you can up it if needed // get count of how many rows we'll be dealing with $total_rows = mysql_num_rows(mysql_query("SELECT `isbn` FROM `BOOKS`")); // how many rows per loop execution - you can up this too, probably like a 1000 is a good number $rows_per_loop = 250; // Get the most amount of loops possible. $total_loops = ceil($total_rows/$rows_per_loop); // just make it look nice: echo 'Running rows: <br />'; // run the loop, while loop counter is less than the total number of loops: for($i=0; $i<$total_loops; $i++) { // get the numbers for the limit, // start is just the current loop number multiplied by the rows per loop // and end is loop counter + 1, multiplied by the rows per loop $limit_start = $rows_per_loop*$i; $limit_end = $rows_per_loop*($i+1); // Remember, only call the columns you need, not * -> that'll help a lot $result = mysql_query("SELECT `price`, `isbn` FROM `BOOKS` LIMIT $limit_start, $limit_end") or die(mysql_error()); // now run the while loop while ($myrow = mysql_fetch_assoc($result)) { // ***a side note, I change mysql_fetch_array to assoc since you're calling just the assoc names, not their index #'s. $item = $myrow['isbn']; // get DOM from URL or file $html = file_get_html('http://mysite.com/price.aspx?ItemNo=$item'); // ***how big is this? // find all div tags with id=gbar foreach($html->find('div#price') as $e) $num= $e->innertext; $new_num = preg_replace ('~[^0-9.]~','', $num); $margin_num=$new_num*1.15; // ***IS THIS SUPPOSED TO BE IN THE LOOP TOO? YOU FORGOT THE { } ON FOREACH if($margin_num!=0) mysql_query("UPDATE BOOKS set `price` = '$margin_num' WHERE `isbn` ='$item'", $db); } // end while loop. // now that we've run those, let's clear the results so that we don't run out of memory. mysql_free_result(); // show where we are at echo $limit_start,' - ',$limit_end,'<br />'; sleep(1); // give PHP a little nap to keep from overloading server ob_flush(); // as recommended by MadTechie, in case we go beyond the max execution time flush(); // add flush, to make sure it is outputted } //end for loop echo 'Rows updated!'; ?> A few things I would add in: make sure nobody else can run this script possibly add input where you can start/stop the updating (kinda like what you were trying to do with pagination) - update rows 500-2500
-
I can give you some basic pseudo code: main mysql query get total number of rows free result number of loops = row count/execute in each loop loop [ number of loops-- ] { mysql query with limit(start row, end row) do something with results, while loop free results sleep for a second }
-
Okay, well, another way to do it is.... ini_set("memory_limit","12M"); Increase memory limit or, Break up your query, run it X rows at a time (use mysql LIMIT), free the result, run the next, repeat. edit: of course I'm just throwing 12M as an example
-
Err, I'm sorry - typo: if($i/%250==0) { Change to: if(($i%250)==0) {
-
Good point.
-
<?php class test { public $var1; public $var2; function testlol($var) { $this->var1 = $var; $this->var2 = 'haha'; } } $myClass = new test; $myClass->testlol("hi"); print_r($myClass); ?> Will show:
-
$i=0; while ($myrow = mysql_fetch_array($result)) { ++$i; if($i/%250==0) { echo $i; sleep(1); // give PHP a break, if you have 43,000 rows } $item = $myrow["isbn"]; // get DOM from URL or file $html = file_get_html("http://mysite.com/price.aspx?ItemNo=$item"); // find all div tags with id=gbar foreach($html->find('div#price') as $e) $num= $e->innertext; $new_num = preg_replace ('~[^0-9.]~','', $num); $margin_num=$new_num*1.15; if($margin_num!=0) mysql_query("UPDATE BOOKS set `price` = $margin_num WHERE `isbn` =$item", $db); } That will show when your on rows that are multiples of 250. It'll also give PHP a little break each time, so you don't overload the server. Of course, that number could be higher, like every 1000.