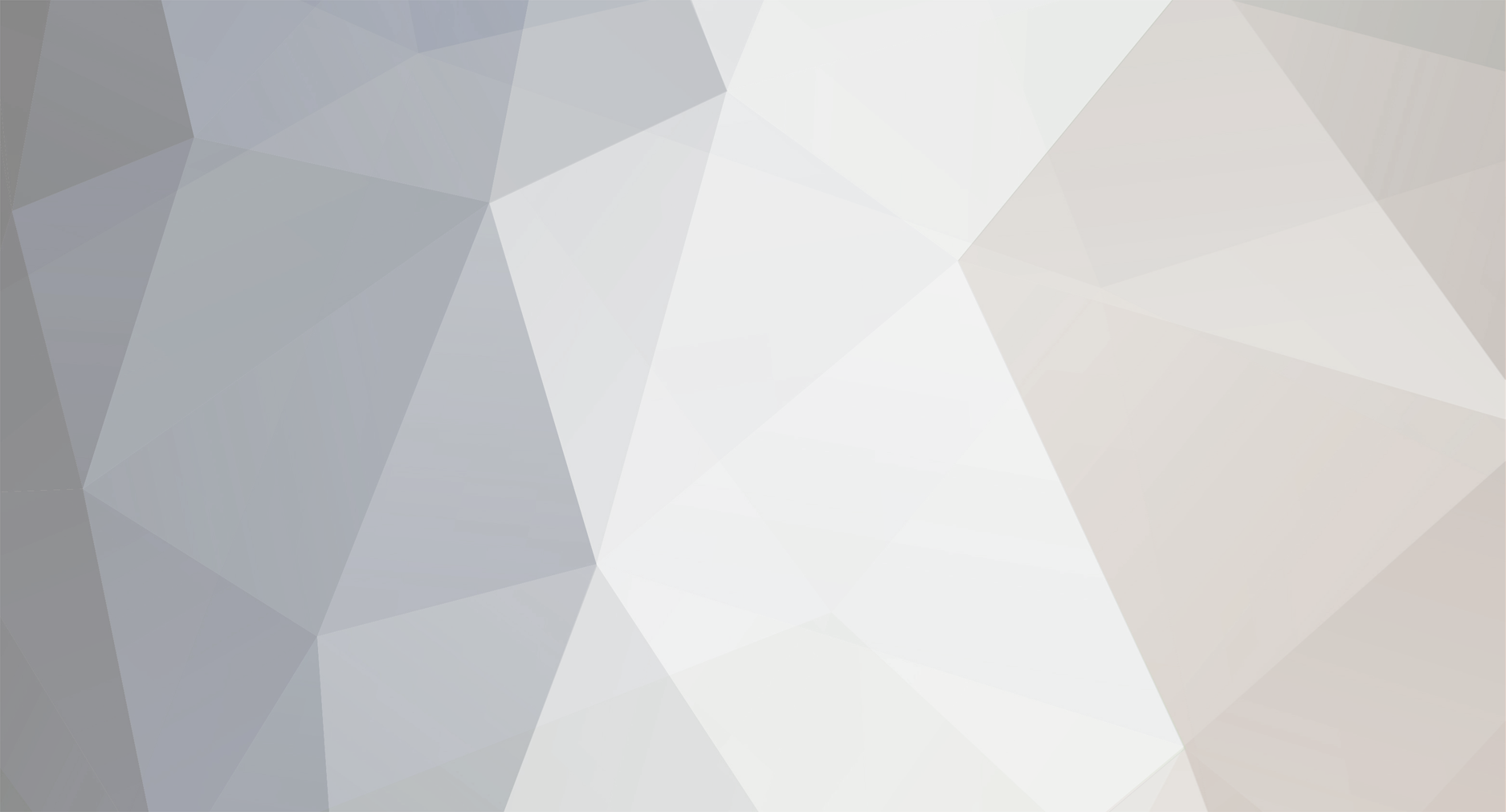
Philip
Staff Alumni-
Posts
4,665 -
Joined
-
Last visited
-
Days Won
20
Everything posted by Philip
-
It really depends on what your function is for and how you plan to use it now & in the future.
-
Honestly, there isn't anything wrong with outputting straight from a function (at least in my eyes.) Typically, you'd want to return the variable so you can separate code from design, thus having like: echo some_function('title'); For your specific function, you could do something like this: <?php function getApprovedComments( ) { // Call your query for comments $query = mysql_query("SELECT * FROM `comments` WHERE `is_approved`=1"); // Setup a blank array $comments = array(); while($row = mysql_fetch_assoc($query)) { // Attach the current to the array of comments. $comments[] = $row; } // Return all the comments return $comments; } // grab all comments.... $comments = getApprovedComments(); // And see, now you can break out of PHP - this will allow for more seperated code using the function return ?> <div id="allcomments" style="border:4px solid black;padding:0px 2px 2px 5px;">All comments <?php // Loop through each comment in the comments array. foreach($comments as $comment) { echo "<div style=\"border:0px solid black;padding:0px 2px 2px 5px;\">"; echo "<a href=\"http://bllllarrrrgggg/index.php?page={$comment['page']}&article={$comment['art_id']}\">{$comment['page']} - Article {$comment['art_id']}</a>"; echo "<form name=\"appordel\" method=\"POST\" action=\"\">"; echo "<b><font size=\"+2\" face=\"arial\">{$comment['name']}</font></b> said on {$comment['date']}...</a><br>"; echo "<span style=\"font-size:50pt;color:blue;z-index:-1;float:left;\">“</span><span style=\"z-index:2;\">{$comment['comments']}</span><span style=\"font-size:25pt;color:blue;\">”</span><br /><hr width='25%' align='left'>"; echo "<input type=\"hidden\" name=\"page\" value=\"{$comment['page']}\">"; echo "<input type=\"hidden\" name=\"art_id\" value=\"{$comment['art_id']}\">"; echo "<input type=\"hidden\" name=\"com_id\" value=\"{$comment['com_id']}\">"; echo "IP address: {$comment['ip']}<br>"; echo "<input type=\"radio\" name=\"comment_action\" value=\"approve\" id=\"approve_action_{$comment['com_id']}\"> <label for=\"approve_action_{$comment['com_id']}\">Approve</label> - "; echo "<input type=\"radio\" name=\"comment_action\" value=\"delete\" id=\"delete_action_{$comment['com_id']}\"> <label for=\"delete_action_{$comment['com_id']}\">Delete</label>"; echo " <input type=\"submit\" value=\"<-- Do it!\">"; echo "</form>"; echo "</div>"; } ?> </div> <?php //.. continue script... ?> I'd suggest if you did it the above way to do something like the following, where you function will define the name of the indexes. That way in the future, if you were to change the column names in the database, you wouldn't have to change every script that you calls the column names - just the function. <?php function getApprovedComments( ) { $query = mysql_query("SELECT * FROM `comments` WHERE `is_approved`=1"); $comments = array(); while($row = mysql_fetch_assoc($query)) { // When creating the array, you would define easy to use keys... // like cID for comment ID, etc. $comments[] = array( 'cID' => $row['commentID'], 'uID' => $row['userID'], 'name' => $row['firstname'], 'date' => $row['postdate'], ); } return $comments; } $comments = getApprovedComments(); foreach($comments as $comment) { echo $comment['cID'],'--',$comment['name'],'<br>'; } ?> Hopefully that makes sense.
-
Do you see the difference between the two: var_dump($drop) => string(95) "Third Grading" var_dump("Third Grading") => string(13) "Third Grading"
-
Well, technically $drop is Since PHP is server side, that would not parse until the user views it - at which point PHP has already done its job
-
How to return random result from table with this script....
Philip replied to friendspimp's topic in PHP Coding Help
http://www.phpfreaks.com/forums/index.php/topic,125759.0.html -
[SOLVED] 2nd pair of eyes :: insert fails here
Philip replied to RyanSF07's topic in PHP Coding Help
Change: $content .="<p>We're sorry, but your info can't be uploaded at this time.<br><br><br><br></p>"; to: $content .="<p>We're sorry, but your info can't be uploaded at this time. ".mysql_error()."<br><br><br><br></p>"; -
im a nervouse person nothings ever perfect for me
-
[SOLVED] cant get variable to work. seems so simple.
Philip replied to Reaper0167's topic in PHP Coding Help
As well as calling mysql_fetch_assoc -
I think I'm just blind. Maybe it's the brake instead of break throwing me off, but each time I read it I read the "keep doing the same processing" as process something for each line. BLAHH.
-
[Tutorials] Copy code to clipboard
Philip replied to soycharliente's topic in PHPFreaks.com Website Feedback
Ah, I'm blind. I see it now, same thing with me. -
Make sure you're sending all the correct headers (the full FROM and such) Are you by chance on GoDaddy? I've heard of a lot of people that use GoDaddy hosting having to contact them to take their site off a spam list to send out emails.
-
[Tutorials] Copy code to clipboard
Philip replied to soycharliente's topic in PHPFreaks.com Website Feedback
It copies? I thought it just selected all in the code box. :-\ -
Oh, sorry, I didn't see you didn't want words to be broken. In that case, here's how I'd do it: <?php // the following's value should be something unique and never show in your string: define('DELIMITER', '|||'); // Your string: $string = 'Advanced Training Institute for Mental Health Professional Evidence-based Mental Health Treatment of Traumatized A Seinfeldian Model of Ethical Reasoning: Jerry, George, Elaine, and Kramer Teach Aspirational Ethics Psychiatric Emergencies: Best Practices in the Assessment and Management of the Patient in Crisis'; // wordwrap will place the delimiter at or before every 90 chars, and will not break words // we will then explode the string by our unique delimiter into an array $array = explode(DELIMITER,wordwrap($string, 90, DELIMITER, false)); // then loop through the results foreach($array as $value) { // do whatever to string... // and output it. echo $value,'<br>'; } ?> Outputs:
-
Have you looked at [http://php.net/str_split]str_split()[/url] ?
-
Seems you still haven't found the edit button either.
-
Haha, yeah yeah, thats just a minor detail. I can get a decent FPS off most games @ highest settings, and have run Crysis on High (not ultra because didn't have dx10 at the time) with I think it was 8xAA and still was hitting low-mid 40FPS.
-
@haku: Variables won't execute in single quotes. echo '<TITLE>',$item_title,'</TITLE> <meta name="description" content="',$item_desc,'"> <meta name="keywords" content="',$item_keywords,'">'; or: echo "<TITLE>$item_title</TITLE> <meta name=\"description\" content=\"$item_desc\"> <meta name=\"keywords\" content=\"$item_keywords\">";
-
If it shows that, it means your query is failing. Have you tried running it in PHPMyAdmin, or the like?
-
@Ken - yes. It is even stated that is a non-object in the error: The only call to a method of build_url is using the $settings variable.
-
If I'm understanding you correctly, you want to store your results for a later use? $sql = mysql_query("SELECT `id`, `foo`, `bar` FROM `table` WHERE `status`=1"); $results = array(); while($row = mysql_fetch_assoc($sql)) { $results[] = $row; // You could even limit what you save for later: // $results[] = array('id'=>$row['id'], 'bar'=>$row['bar']); echo $row['foo'].'<br>'; } // ... later in the script: foreach($results as $key=>$value) { echo $value['bar'].' '.$value['id'].'<br>'; }
-
It is all about the scope though. Example script you can test with: <?php error_reporting(E_ALL); $var = 'test'; class scope { public $test = 'scoped'; function __construct( ) { echo $var; echo '<br>'; echo $this->test; } } $myClass = new scope; ?>
-
I'll be honest here, that is a lot of data to read through to see what is different. It'd be better to make a smaller batch of example data to show us. One thing I'd change right off the bat: mysqli_fetch_array to mysqli_fetch_assoc. There is no need to create an array with both the numeric keys and the associate keys. That'll get rid of half of your entries right there.
-
Actually, for the non-object problem: $settings->build_url(); $settings isn't defined anywhere - thus you can't call a method on a non-object.