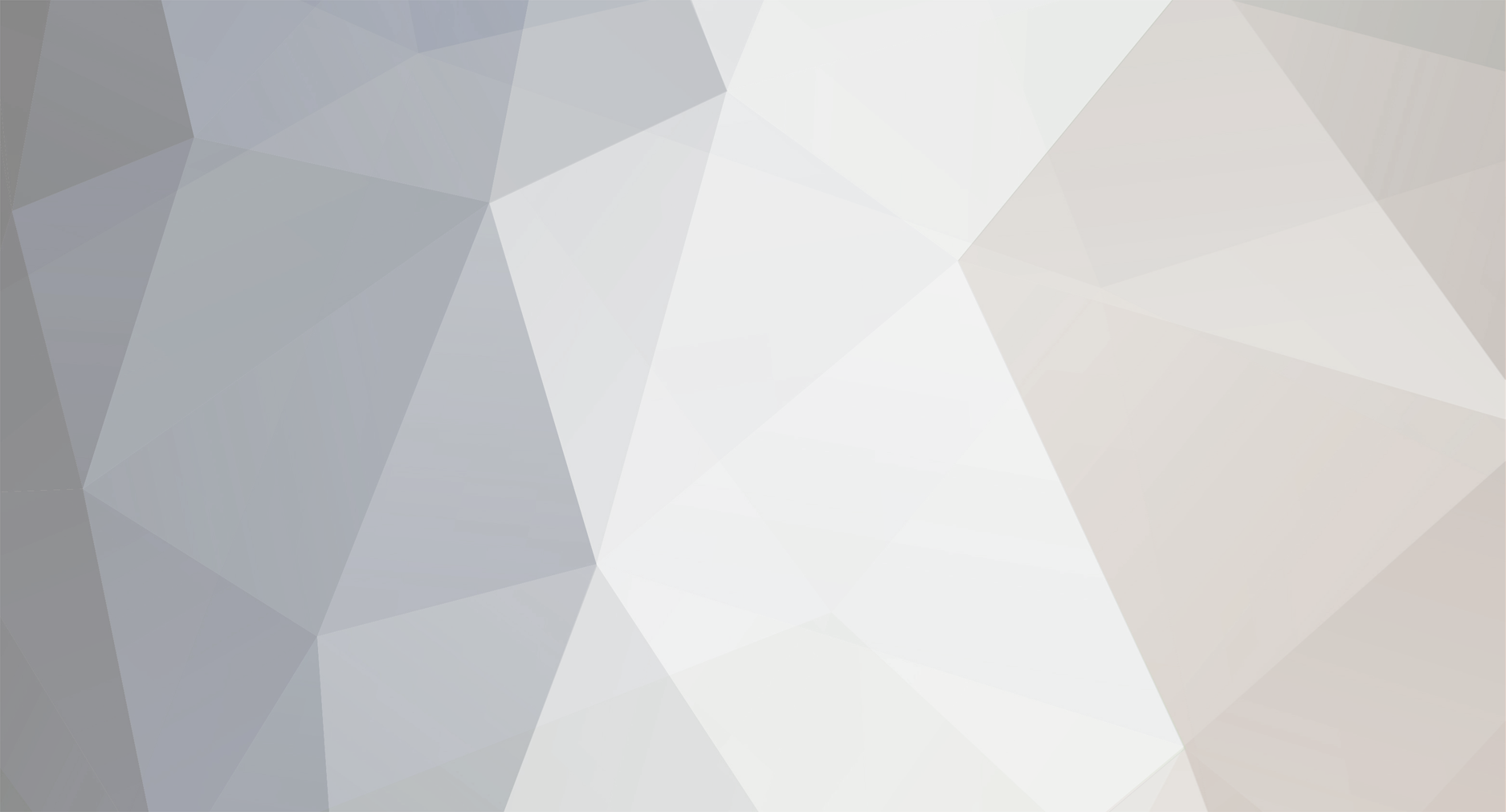
QuickOldCar
Staff Alumni-
Posts
2,972 -
Joined
-
Last visited
-
Days Won
28
Everything posted by QuickOldCar
-
Convert the first letter of every paragraph to upper case
QuickOldCar replied to soma56's topic in PHP Coding Help
<?php function upperFirstWord($str) { return preg_replace('/([.!?])\s*(\w)/e', "strtoupper('\\1 \\2')", ucfirst(strtolower($str))); } echo upperFirstWord("This is a paragraph. is this the car blue? let's go for a pint of beer!"); ?> -
Get and Post request in PHP with RollingCurl library
QuickOldCar replied to konkhra's topic in Third Party Scripts
Here it is with using a text area for multiple urls, I didn't spend any time styling this or anything, but to show you how can do it. <form name="input" action="" method="post"> <textarea cols="100" rows="10" name="url" placeholder="Place urls each line" value="<?php echo $_POST['url'];?>"/><?php echo $_POST['url'];?></textarea> <input type="submit" value="fetch urls"/> </form> <?php if(isset($_POST['url']) && $_POST['url'] != ''){ function request_callback($response, $info) { print_r($info); echo "<hr>"; } $url_values = array(); $show_urls = str_replace(array(",","\r",",\n "," "), "|",$_POST['url']); $urls_array = explode("|",$show_urls); foreach($urls_array as $urls){ if($urls != ''){ $url_values[] = trim($urls); } } require("RollingCurl.php"); echo "<hr>"; $rc = new RollingCurl("request_callback"); $rc->window_size = 20; foreach ($url_values as $url) { $request = new RollingCurlRequest($url); $rc->add($request); } $rc->execute(); } ?> -
Get and Post request in PHP with RollingCurl library
QuickOldCar replied to konkhra's topic in Third Party Scripts
Here is the basics to do multiple urls <?php function request_callback($response, $info) { print_r($info); echo "<hr>"; } require("RollingCurl.php"); echo "<hr>"; $urls = array("http://phpfreaks.com", "http://www.facebook.com", "http://www.yahoo.com", "http://www.youtube.com", "http://www.live.com", "http://www.wikipedia.com", "http://www.msn.com", "http://www.yahoo.co.jp", "http://www.myspace.com", "http://www.twitter.com", "http://www.microsoft.com"); $rc = new RollingCurl("request_callback"); $rc->window_size = 20; foreach ($urls as $url) { $request = new RollingCurlRequest($url); $rc->add($request); } $rc->execute(); ?> For parsing or other information needed for each url, you get it the same way as any other normal curl request. Add whatever you need in the request_callback function -
That could get pretty complicated. I suppose if you exploded the search terms into each keyword, and also query each user, then have them all in an array and display the highest number of results first......it's possible. But that would be pretty intensive usage to do that for all your users. I would suggest looking into mysql fulltext search in boolean mode, and read about the minimum word length, creating indexes http://dev.mysql.com/doc/refman/5.6/en/fulltext-search.html The following query will display a result if it contains at least one or more of the keywords in the search term. $q = "SELECT * FROM info WHERE MATCH (interests) AGAINST ('$search_term' IN BOOLEAN MODE) ORDER BY RAND() ";
-
Php Search Files Script Help (Code Canyon)
QuickOldCar replied to Zola's topic in Third Party Scripts
Basically what I wrote earlier, but they are using $_REQUEST instead, it's better to specify it, but we'll go with what they already use. if(isset($_REQUEST['search']) && $_REQUEST['search'] != ''){ include("search.php"); } else { //or include a normal content file echo "show content"; } -
Php Search Files Script Help (Code Canyon)
QuickOldCar replied to Zola's topic in Third Party Scripts
It's a little difficult to do anything without seeing any code for this. In the search form, make the action="" so it stays on the same page. For your search form there is a POST or GET value, whichever it may use...do a code similar to this in your content area to display it. Change the values to your own values. if(isset($_POST['search']) && $_POST['search'] != ''){ include("search-results.php"); } else { //or include a normal content file echo "show content"; } -
trying to make text and images into clickable links
QuickOldCar replied to scmeeker's topic in PHP Coding Help
You should probably switch these around to make more sense. from: $detail = $newArray['id']; $photo = $newArray['photo']; $id = $newArray['title']; $price = $newArray['price']; to: $id = $newArray['id']; $photo = $newArray['photo']; $detail = $newArray['title']; $price = $newArray['price']; You should really do all the positioning with some sort of css styling to get it the exact way you want. echo "<p><a href=\"$photo\"><img title=\"$detail\" alt=\"$detail\" width=\"$maxWidth\" src=\"$photo\" /></a><br />$detail"; Other ways you can do the same links. If wrapped with double quotes, all single quotes within echo "<p><a href='$photo'><img title='$detail' alt='$detail' width='$maxWidth' src='$photo' /></a><br />$detail"; or use concatenation echo "<p><a href='".$photo."'><img title='".$detail."' alt='".$detail."' width='".$maxWidth."' src='".$photo."' /></a><br />".$detail; -
There was a tutorial that had some errors in it that I got working, it would still need some more checking, but it works. create table sql CREATE TABLE IF NOT EXISTS `dbusers` ( `id` int(11) NOT NULL auto_increment, `username` varchar(32) collate utf8_unicode_ci default NULL, `password` char(32) collate utf8_unicode_ci default NULL, `email` varchar(32) collate utf8_unicode_ci default NULL, PRIMARY KEY (`id`), UNIQUE KEY `username` (`username`) ) ENGINE=MyISAM DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci; dbConfig.php <?php //use your database connection information $host = "localhost";//usually localhost,change if need different $db_username = "user";//change database user name $db_password = "password";//change database password $db_name = "database";//change database name $db = mysql_pconnect($host, $db_username, $db_password); if ( !$db ) { echo "Error connecting to database.\n"; } mysql_select_db($db_name,$db); ?> index.php <?php session_start(); include('nav.php'); echo "This is the index page"; ?> login.php <?php session_start(); include('dbConfig.php'); if(isset($_POST)){ $username = trim($_POST['username']); $password = trim($_POST['password']); $md5pass = md5($password); if (!empty($_POST["username"]) || !empty($_POST["password"])) { $sql_query = mysql_query("SELECT * FROM dbUsers WHERE username='$username'"); $row = mysql_fetch_array($sql_query) or die(mysql_error()); $user_id = $row['id']; $user_name = $row['username']; $user_password = $row['password']; if($username == $user_name && $md5pass == $user_password) { // Login good, create session variables $_SESSION["valid_id"] = $user_id; $_SESSION["valid_user"] = $user_name; $_SESSION["valid_time"] = time(); //change where to redirect after login //header("Location: index.php"); header("Location: members.php"); } else { $message = "Invalid Login."; } } else { $message = "Insert user name or password."; } include('nav.php'); echo "<form action='' method='POST'>"; echo "Username: (32 Characters Max) <input name='username' size='32'><br />"; echo "Password: (32 Characters Max) <input type='password' name='password' size='32'><br />"; echo "<input type='submit' value='Login'>"; echo "</form>"; echo $message; } ?> logout.php <?php session_start(); session_unset(); session_destroy(); // Logged out, return home. header("Location: index.php"); ?> members.php <?php session_start(); if (!$_SESSION["valid_user"]) { // User not logged in, redirect to login page header("Location: login.php"); } include('nav.php'); // Display Member information echo "<p>User ID: " . $_SESSION["valid_id"]; echo "<p>Username: " . $_SESSION["valid_user"]; echo "<p>Logged in: " . date("m/d/Y", $_SESSION["valid_time"]); // Display logout link echo "<p><a href=\"logout.php\">Click here to logout!</a></p>"; ?> nav.php <a href="index.php"> HOME </a> <a href="members.php"> Members </a> <a href="login.php"> Login </a> <a href="logout.php"> Logout </a> <a href="register.php"> Register </a> <br /> register.php <?php // dbConfig.php is a file that contains your // database connection information. This // tutorial assumes a connection is made from // this existing file. include ("dbConfig.php"); //Input vaildation and the dbase code if ( $_GET["op"] == "reg" ) { $bInputFlag = false; foreach ( $_POST as $field ) { if ($field == "") { $bInputFlag = false; } else { $bInputFlag = true; } } // If we had problems with the input, exit with error if ($bInputFlag == false) { die( "Problem with your registration info. " ."Please go back and try again."); } $user = mysql_real_escape_string(trim($_POST['username'])); $pass = md5(mysql_real_escape_string(trim($_POST['password']))); $mail = mysql_real_escape_string(trim($_POST['email'])); // Fields are clear, add user to database // Setup query $r = mysql_query("INSERT INTO dbUsers (username, password, email) VALUES('$user', '$pass', '$mail' ) ") or die(mysql_error()); // Make sure query inserted user successfully if ( !$r ) { die("Error: User not added to database."); } else { // Redirect to thank you page. header("Location: register.php?op=thanks"); } } // end if //The thank you page elseif ( $_GET["op"] == "thanks" ) { echo "<h2>Thanks for registering!</h2>"; echo "Redirecting you to log in<br />"; echo "<meta http-equiv='refresh' content='5;url=login.php'>"; } //The web form for input ability else { include('nav.php'); echo "<form action='?op=reg' method='POST'>\n"; echo "Username: <input name='username' MAXLENGTH='32'><br />\n"; echo "Password: <input name='password' MAXLENGTH='32'<br />\n"; echo "Email Address: <input name='email' MAXLENGTH='32'><br />\n"; echo "<input type='submit'>\n"; echo "</form>\n"; } // EOF ?>
-
Is no meta description or keywords. Is lots of white area, so looks like everything is spaced really far apart. Not entirely sure why your home link leads to http://uaefreelancers.net/index.php/site Since isn't much there, isn't anything else to comment about.
-
Double posting, is the same question here. http://www.phpfreaks.com/forums/index.php?topic=358927.0
-
Posting your code would help. But it's best to use css to place items where you want them.
-
Display images from different sub-folders located in one folder
QuickOldCar replied to manhunt234's topic in PHP Coding Help
Are welcome, mark as solved -
Display images from different sub-folders located in one folder
QuickOldCar replied to manhunt234's topic in PHP Coding Help
oops, sorry, the line should be this $dynamicList .= '<td width="135"><a href="product_details.php?productID=' . $id . '"><img src="' . $image_source . '" alt="' . $product_name . '" width="129" height="169" border="0"></a></td> -
Display images from different sub-folders located in one folder
QuickOldCar replied to manhunt234's topic in PHP Coding Help
I guess can use file_exists() Providing that each id is unique to both men and women. I used default.jpg as a filler image if none of the 2 images were found, just so can display something. Just make an image for it. if (file_exists("images/products/Men/".$id.".jpg")) { $image_source = "images/products/Men/".$id.".jpg"; } elseif (file_exists("images/products/Women/".$id.".jpg")) { $image_source = "images/products/Women/".$id.".jpg"; } else { $image_source = "images/default.jpg"; } And for this line you can use this instead $dynamicList .= '<td width="135"><a href="product_details.php?productID=' . $id . '"><img src="$image_source" alt="' . $product_name . '" width="129" height="169" border="0"></a></td> If that doesn't work for you, then add a field to designate if is Men or Women in your database so you can use those values to determine which folder. And an even better suggestion would be to save the exact image location in the database. -
it should be >= not =>
-
I figure I would add to this and just show you the exact search query SELECT * FROM (`posts`) WHERE MATCH(title,content) AGAINST('\"$complete_search_term\"' IN BOOLEAN MODE); But if you just do a simple query, and have them just wrap in quotes for exact phrases will work also. SELECT * FROM (`posts`) WHERE MATCH(title,content) AGAINST('$complete_search_term' IN BOOLEAN MODE); What I do on my simple search is explode all the words, add a + before it if there isn't a + or a - already there, but not if the phrase is wrapped in quotes. (exact) with quotes: http://dynaindex.com/search.php?s=%22watch+free+tv%22&filter=on&page=1 (must contain) without quotes: http://dynaindex.com/search.php?s=watch+free+tv&filter=on&page=1 $trimmed_words = ''; foreach($explode_trimmed as $trim_words){ if(substr($trim_words,0,1) != "-" || substr($trim_words,0,1) != '"'){ $trim_words = trim($trim_words); $trimmed_words .= " +$trim_words"; } else { $trim_words = trim($trim_words); $trimmed_words .= " $trim_words"; } } $trimmed_words = trim($trimmed_words); $trimmed_words = preg_replace('/\s+/', ' ', $trimmed_words);
-
Mysql fulltext is the proper way to do this as you are. You may want to explode all the words and modify the operators for each type of search you need. http://dev.mysql.com/doc/refman/5.6/en/fulltext-boolean.html EXACT PHRASE wrapped with double quotes "word" OR just words with spaces word word AND words with a + means must contain +word MUST CONTAIN multiple + words would narrow the search down more to contain just those words +word +word EXCLUDE words with a - would exclude that word word -word or can do combinations "word word" +word -word There are more but these above are the most useful You could even do a multiple if/elseif statement and different types of searches. Here is an example of how I do it. I do the advanced search for all or each one of my fields, that's why is so many lines of code there. http://www.phpfreaks.com/forums/index.php?topic=318221.msg1500385#msg1500385
-
I personally have never seen the issue. Is a thread about someone else that did http://www.phpfreaks.com/forums/index.php?topic=339051.0
-
you need to use the double == for checking the values if ($ampm == "am"){ elseif ($ampm == "pm"){
-
Totally agree with what gizmola said. If you really want full control over what your server can do it would be best to rent a dedicated server. http://www.limestonenetworks.com/ was about my best host. Not gonna be able to do much besides normal websites on the $8 a month hosts or similar priced. You get what you pay for, and sometimes not even that.
-
something like this if(is_valid_uk_postcode($strSearch) == TRUE)) { //use code or message for when is good echo 'Valid postcode'; } else { //do something else with it echo 'Invalid postcode'; } going by how you are using this in your code, I guess something like this could work if((!isset($_POST['strSearch'])) || (strlen(trim($_POST['strSearch'])) <5) || (trim($_POST['strSearch']) != preg_replace("/[^a-zA-Z0-9\_]/", "", trim($_POST['strSearch'])))) { $error_message = "You must enter a valid postcode<br>"; $error_message = $error_message . "Valid postcodes must not contain and spaces and consist of letters and numbers only.<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['strSearch'] . "</font><hr>"; }else{ $postcode = mysql_real_escape_string(trim($_POST['strSearch'])); if(is_valid_uk_postcode($postcode) == FALSE)) { exit("Invalid postcode"); } } or just do the error message as you did others, and add this check to this line as so if((!isset($_POST['strSearch'])) || is_valid_uk_postcode(trim($_POST['strSearch']))) == FALSE || (strlen(trim($_POST['strSearch'])) <5) || (trim($_POST['strSearch']) != preg_replace("/[^a-zA-Z0-9\_]/", "", trim($_POST['strSearch'])))) {
-
What you should be doing is trimming the www. off in case people try to use it.
-
use "when" or "whenever" versus "when ever" in your take a drink rules Some sort of border or content area for the details, it's just text on a big page. I guess some sort of style all around would be nice. error in your pagination when trying to go back to first pages using << http://drinktothecredits.com/<?php movies.php?currentpage=1
-
the term pagelets is a very old term, try looking for "user controls" Look into any of the template engines such as smarty you can easily do something like this with an include() <html> <head> <title>My title</title> </head> <body> <?php include('header.php'); ?> <?php include('body.php'); ?> <?php include('footer.php'); ?> </body> </html>
-
http://www.tizag.com/phpT/fileupload.php // Where the file is going to be placed $target_path = "Upload/"; /* Add the original filename to our target path. Result is "Upload/filename.extension" */ $target_path = $target_path . basename( $_FILES['file']['name']);