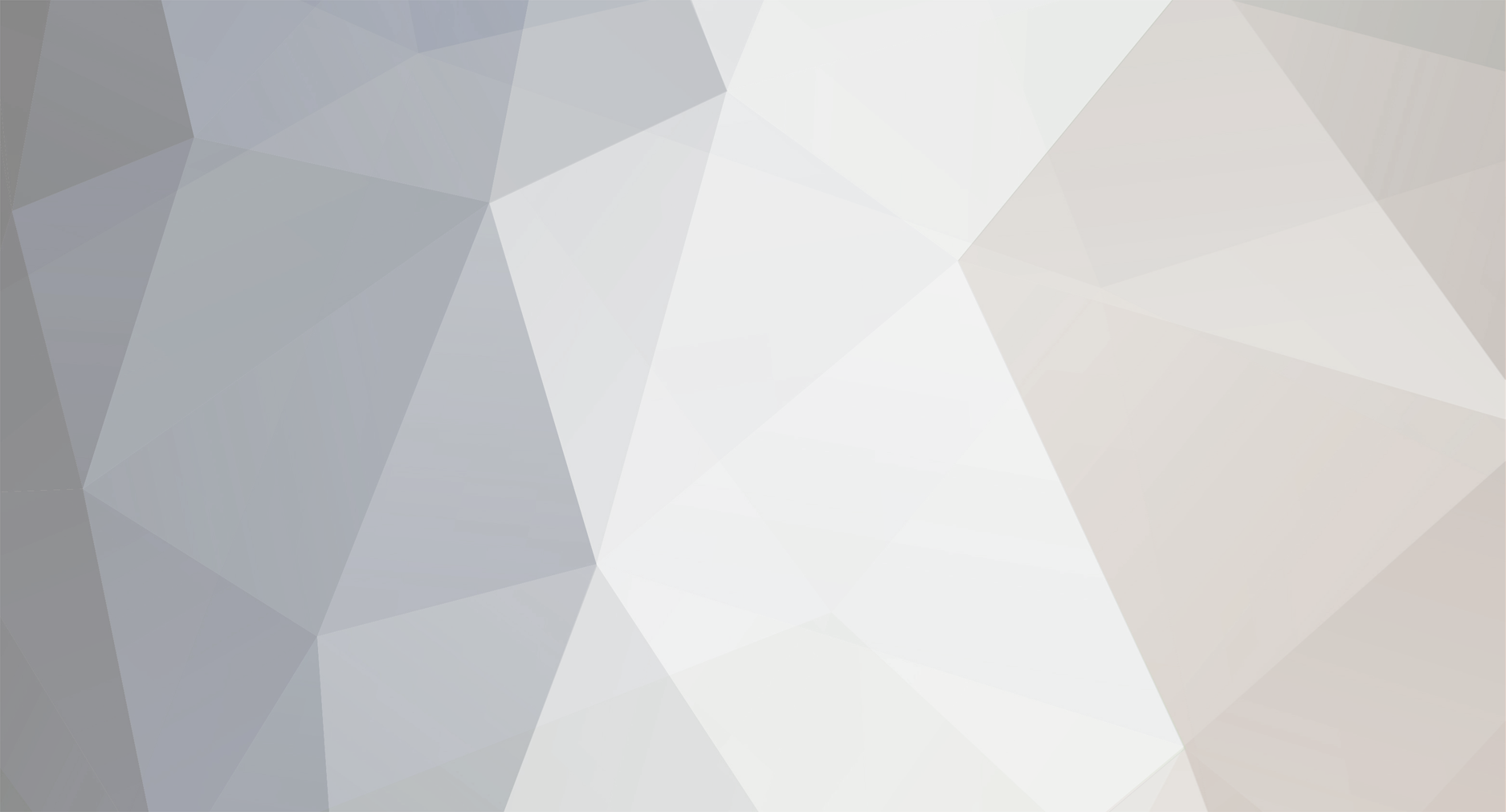
zq29
-
Posts
2,752 -
Joined
-
Last visited
-
Days Won
1
Posts posted by zq29
-
-
I've never done this before, but here's where I'd start if I wanted to:
[0] Read the documentation for your mail server software
[0] Figure out how to create an account with the command line
[0] Use PHPs system functions to run these commands
As far as building the interface for your users to log in and check their mail, I'd read though the section of the PHP manual entitled [url=http://uk2.php.net/manual/en/ref.imap.php]LXIII. IMAP, POP3 and NNTP Functions[/url]. -
Ahhh, I see! Thanks a bunch Fenway :)
-
Please do not double post. You have posted the same question in our MySQL forum, where it should be - So this one is locked and will be deleted shortly.
-
[quote author=fenway link=topic=107126.msg429417#msg429417 date=1157566652]
No problem... you were simply returning N**3 rows. I've done that before, on a 4-table join, before I switched to join syntax, and accidentally return >9 trillion records. That'll take down a server real fast, trust me.
[/quote]All I did was remove references to tables I wasn't actually using from the 'FROM' part of my query. Would it be possible for you to elaborate on what you mean by 'join syntax'? I've done a bit of searching here and there and I can only find references to left and right joins, and I don't think that is what I need here... -
I really don't understand the appeal of wrestling. Unless it's naked hot lesbian wrestling - then I'm all for it.
-
Thanks Fenway, it was indeed the lack of a join condition that was the main cause of my problem. I wouldn't have thought that would have been a problem, but it obviously was.
-
Hi guys, I'm having a problem with a query that is generated by some PHP code. Here's the first which pretty much just crashes my machine, I have to kill the mysql process to get my machine back in a useable state:
[code]SELECT DISTINCT(l.`company`), l.`id`, l.`bullets`, l.`level`, l.`address_1`, l.`address_2`, l.`town`, l.`postcode`, l.`telephone`, l.`email`, l.`map`, l.`website`
FROM `listings` as l, `listing_category` as lc, `categories` as c
WHERE ((l.`company` LIKE '% car %' OR l.`company` LIKE 'car %' OR l.`company` LIKE '% car' OR l.`company` LIKE 'car'))
ORDER BY l.`level` DESC, l.`top` ASC, l.`company` ASC[/code]
And here's the second query, that is pretty much the same as the one above, except that it queries a different table, this one returns results instantly:
[code]SELECT DISTINCT(l.`company`), l.`id`, l.`bullets`, l.`level`, l.`address_1`, l.`address_2`, l.`town`, l.`postcode`, l.`telephone`, l.`email`, l.`map`, l.`website`
FROM `listings` as l, `listing_category` as lc, `categories` as c
WHERE (((c.`name` LIKE '% car %' OR c.`name` LIKE 'car %' OR c.`name` LIKE '% car' OR c.`name` LIKE 'car') AND c.`id`=lc.`category_id` AND lc.`listing_id`=l.`id`))
ORDER BY l.`level` DESC, l.`top` ASC, l.`company` ASC[/code]
Can anyone offer any insight as to why the first one is giving me problems?
Oh, the first table holds around 3000 records, the second about 500. Could this be the reason? If so, any ideas on how to optimise the queries? -
[quote author=redarrow link=topic=106956.msg429089#msg429089 date=1157540375]
I went to the link as you said and looked at the website and had a play, the way the website desiner has acheved this function is using a search function.
[/quote]Thanks for that contribution redarrow... -
Note that you can also use 'AND' instead of '&&', but they have different priorities.
-
Posting in the incorrect forum won't get you the response you are looking for. Topic moved.
-
It is a shame, he always made me laugh whenever I cuaght him on TV. But you have to admit, this was sure to happen sooner or later, the guy was a nutcase...
-
I couldn't care less, but since I'm English - We'll go with them.
-
Could you explain what you mean by "shortest road" please?
-
I don't think this is an Apache configuration issue - I know what you mean though as a site I visit regularly doesn't display full sized images in FireFox, I just get a bunch of garbage text. I have a feeling it's an OS issue, but I don't know for sure. But as far as I know, there are no configuration options for image support in Apache.
-
Does the company you bought the domain from have a forwarding service? I get all my domains from 123-reg.co.uk and I have the option to forward my domains somewhere else but keep the forwarding domain in the location bar. It's probably done with frames or something...
-
[quote author=skatermike21988 link=topic=106915.msg428261#msg428261 date=1157445330]
no errors are coming up
[/quote]
You might have to switch on your errors with something like error_reporting(15); or something... -
Care to post up your code? The only way I think this can happen is if you have a type in the destination parameter like:
copy("path/to/source/script.php","path/to/destination/script.html"); -
This is more of a hack than a proper solution, but I'm not sure of the proper way to do this.
[code]SELECT DISTINCT(posts.title), posts.post_body, posts.poster_name, posts.post_time, posts.postid, posts.comment_number, users.avatar FROM posts, users WHERE posts.post_type = '0' ORDER BY posts.postid DESC[/code] -
I think I understand, but why are several records getting the same ID? If the user closes his browser 6 times and then pays on the 7th, I'd assume you'd only want the seventh basket marked as paid with the other 6 being left marked as 'abandoned basket'.
Why are you storing the baskets in a temporary table anyway? For saving abandoned baskets, or so that if a user does close their browser their basket is saved for their next visit? Even still, I don't understand how you're getting duplicate rows in the table... -
I take it that your code is deleting all of the rows with the supplied id, and not just the duplicates, right? I think it might help if you use a LIMIT in your DELETE query...
[code]<?php
$result = mysql_query("SELECT * FROM `payment_info` WHERE `id`='$id'") or die(mysql_error());
$count = mysql_num_rows($result);
if($count > 1) {
$limit = $count - 1;
mysql_query("DELETE FROM `payment_info` WHERE `id`='$id' LIMIT $limit") or die(mysql_error());
}
?>[/code]
ID fields are commonly regarded as unique fields, if you are finding you have ID fields with the same numbers in you have a problem somewhere. Deleting those fields is not the solution, preventing them from appearing in the first place would be more ideal. -
Try this:
[code]<?php
echo "<select name='font'>";
$result = mysql_query("SELECT `font_name` FROM `fonts`");
while($row = mysql_fetch_assoc($result)) {
echo "<option value='$row[font_name]'>$row[font_name]</option>";
}
echo "</select>";
?>[/code] -
To do this without refreshing you will need to use an Ajax technique, if you're wanting to do this purely with PHP, you're pretty much out of luck I'm affraid...
-
You can create a directory with the mkdir() function - If I remember correctly, it's second parameter can be used to chmod the directory at the same time. You can copy files with the copy() function. For more info onhow to use them, check out the manual over at php.net
-
Looks ok to me, what's the problem you are having?
Image resize and rename
in PHP Coding Help
Posted
[code]<?php
function thumbnail($image_path,$thumb_path,$image_name) {
$max_width = 75;
$max_height = 75;
$size = getimagesize($image_path.$image_name);
$width = $size[0];
$height = $size[1];
if($width != FALSE && $height != FALSE) {
$x_ratio = $max_width / $width;
$y_ratio = $max_height / $height;
if(($width <= $max_width) && ($height <= $max_height)) {
$disp_width = $width;
$disp_height = $height;
} elseif(($x_ratio * $height) < $max_height) {
$disp_height = ceil($x_ratio * $height);
$disp_width = $max_width;
} else {
$disp_width = ceil($y_ratio * $width);
$disp_height = $max_height;
}
} else {
return false;
}
$src_img = imagecreatefromjpeg($image_path.$image_name);
$dst_img = imagecreatetruecolor($disp_width,$disp_height);
imagecopyresized($dst_img,$src_img,0,0,0,0,$disp_width,$disp_height,imagesx($src_img),imagesy($src_img));
imagejpeg($dst_img, $thumb_path.$image_name);
return true;
}
?>[/code]