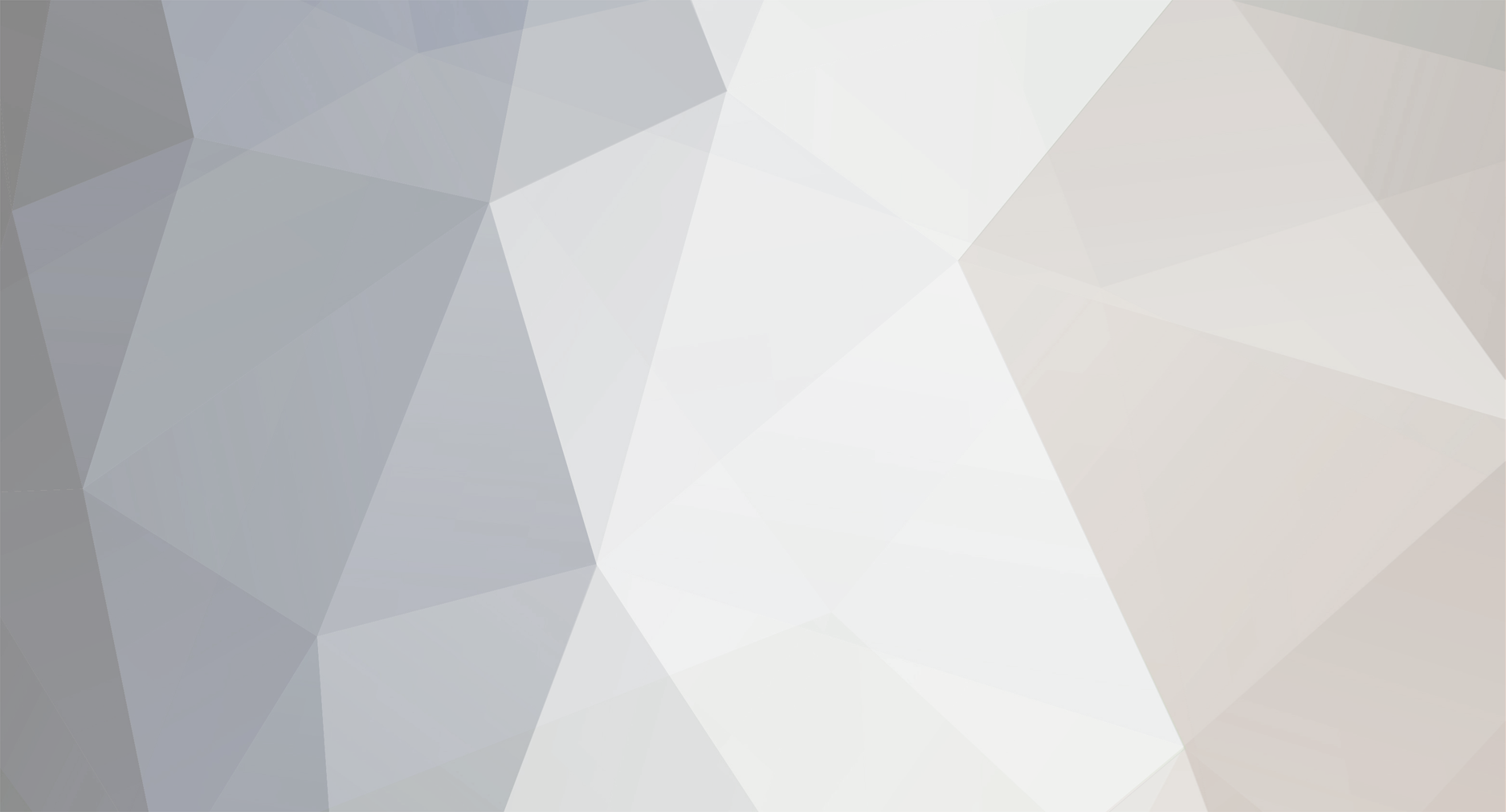
zq29
-
Posts
2,752 -
Joined
-
Last visited
-
Days Won
1
Posts posted by zq29
-
-
You mean like this?
[code=php:0]<?php
function count_to_one_hundred() {
for($i=1; $i<=100; $i++) echo "$i<br/>";
}
count_to_one_hundred();
?>[/code]
You just enclose your code in [php[b][/b]][/php[b][/b]] tags. Although, I too would prefer to see code within the [code[b][/b]] tags, mainly because I prefer to read code with fixed width characters. It looks neater that way. -
If you want it to just jump to the bottom of your iframe, you could just use an anchor like the following, but if you want it to scroll as it loads, yes, you need to use the ajax technique.
[code]//test.html
<iframe src="test2.php#btm" width="400" height="500" scrolling="auto" frameborder="1">
[Your user agent does not support frames or is currently configured
not to display frames. So <a href="http://www.getfirefox.com">get firefox</a>.]
</iframe>
//test2.php
<?php
for($i=0; $i<500; $i++) echo $i."<br/>";
echo "<a name='btm'></a>";
?>[/code] -
Have you tried:
[code]$stateresult = mysql_query($statesql) or die(mysql_error());[/code] -
[quote author=brown2005 link=topic=102059.msg404528#msg404528 date=1154006335]
ok.. ill try.. so in a rating system wat would you use, just a normal average?
[/quote]Personally, yes. Maybe you could filter out all ratings that are full of 1's... -
Off the top of my head, I don't know a function that lists [i]all[/i] set variables, but you could list the posted variables like so:
[code]<?php
echo "<pre>", print_r($_POST), "</pre>";
?>[/code]
[b]EDIT:[/b] Beaten to it! -
I think you're going to struggle even looking for a class that actually creates images without the use of either the GDLib or 3rd party applications such as ImageMagick. Can't you ask your host to enable / recompile with GD support?
-
Could do it with:
[code]<?php
foreach(glob("path/to/files/*.gif") as $file) unlink($file);
?>[/code] -
If your Excel output is in csv format, you could use the function fgetcsv() - There is a good example of how to use it in the manual.
-
I don't understand how this will help, RMS "is a statistical measure of the magnitude of a varying quantity". I'm not brilliant in maths, but I think this is how you work out the RMS of an array of values:
[code]<?php
function rms() {
$vals = func_get_args();
$new = "";
foreach($vals as $n) $new += pow($n,2);
$new = sqrt($new / count($vals));
echo $new;
}
?>[/code]
Someone that [i]is[/i] good at maths, please do go ahead and correct me if I'm wrong. -
[quote author=brown2005 link=topic=102059.msg404505#msg404505 date=1154002874]
hi,
does anyone know how to write php code for a root mean square, i was brosing on the net and saw it and wanted to know how it was done.
[/quote]I don't fully understand, do you want to know how to calculate the RMS of an array of values? -
As far as I'm aware, include doesn't allow you to append a query string. You'll have to define your variables before you include the files...
[code]<?php
$stringy = "test";
include "test.php";
?>[/code] -
[code]<?php
$str = str_replace(" ","-",$str).".txt";
?>[/code] -
The third parameter in the imagejpeg() function is for compression. On a scale of 0 - 100, 0 being the highest compression.
-
You could try:
[code]<?php
$name = $_POST['name'];
$check = mysql_result(mysql_query("SELECT COUNT(*) FROM `table` WHERE `name`='$name'"),0);
if($check > 0) {
//UPDATE
} else {
//INSERT
}
?>[/code] -
[quote author=redarrow link=topic=101912.msg403779#msg403779 date=1153914620]
gd is built into php enable it in php.ini
and lookup hash ok
[/quote]
Yep, GD has been packaged with PHP since version 4.3. Although I believe all hashing algorythms are one way.
For what reason do you want to be able to encrypt/decrypt strings? I don't think there are any encrypt/decrypt functions built into PHP as standard... -
[quote author=Emma ...x link=topic=101910.msg403768#msg403768 date=1153913251]
Hey, i wasnt sure wether i had to post somewhere before making a topic.. so correct me if i'm wrong.[/quote] Nope, post away!
But on to your issue. I think the problem is most likely that you are not referencing your other files correctly within tagme.php. to include, for example, your header.php (which I guess is stored in the directory above 'site') you would have to reference it as '../header.php' instead of just 'header.php'. Without seeing your code, that would be my best guess.
By the way, your site doesn't work correctly in FireFox. -
^^ EDITED MY POST ABOVE ^^
-
You could do something like this:
[code]<?php
function mask_email($email) {
$patterns = array("/\./","/@/");
$replacements = array(" [dot] "," [at] ");
return preg_replace($patterns, $replacements, $email);
}
echo mask_email("mail@somewhere.com");
?>[/code]
[b]EDIT:[/b] I just re-read your post, and you want to know how to [i]find[/i] the email address in a block of text, right? How about this:
[code]<?php
$str = "Hello, my email address is mail@somewhere.com what is your email address? Is it somewhere@mail.com ?";
preg_match_all("/[a-z0-9_]+@[a-z0-9\-]+\.[a-z0-9\-\.]+/",$str,$out);
foreach($out[0] as $email) {
$str = str_replace($email,mask_email($email),$str);
}
echo $str;
?>[/code]
An alternative option would be to use preg_replace() I guess, but I'm not that good at regex to offer a way of using it to the extent that you need to! -
[quote author=01706 link=topic=101901.msg403737#msg403737 date=1153909965]
i think my first post was a repley to a script on www.phpfreaks.com but i cant rember what script it as it was ages ago
[/quote]
Ok, I'm sure an admin will be along soon to offer some insight. -
Personally, I'm not sure how this has happened, even as a moderator I can not see what your email address is. Although I guess the admins can, but see no reason why they would give your specific email address to anyone... I see this is your second post, did your first post happen to be in our freelancing forum?
-
[code]<?php
$str = "alpha beta gama";
$new = str_replace(" ","-",$str).".txt";
?>[/code] -
Hi, my name is SA, I am 6 and a half years old and I am fluent in both binary and assembly. I'd also say I was somewhat proficient in Fortran, Lisp, COBOL and Pascal (inc. Turbo). So far this year, having already learnt the garden variety web languages such as XHTML, CSS and JavaScript, I have picked up PHP at an intermediate level and am progressing well. In my spare time, while not watching the Teletubbies on TV, I work at the R&D lab at Nvidia designing ICs for the next generation graphics solutions.
-
[quote author=Barand link=topic=101778.msg403339#msg403339 date=1153852857]
Alternatively, if you convert the sentence to an array of words
[code]<?php
$words = array ('cat','dog','fish','bucket','cheese','wheel');
$words2 = explode(' ', strtolower("the monkey jumped from the bucket"));
$found = array_intersect($words, $words2);
// view results
echo '<pre>', print_r($found, true), '</pre>';
?>
[/code]
[/quote]Yeah, that was one of the other ways I was thinking too. If you go about it this way you'll have to strip out any punctuation if it's present in your actual data though... -
Also, a good method of learning, as previously mentioned elsewhere by one of our members (Crayon_Violent), is to read peoples problems on our forums, and even if you don't know the answer - Do some research and build a working solution.
On the odd occasion I learn something new, or improve on something I already knew by offering solutions to peoples problems. For example, a few days back I learnt how to list all of the physical drives attached to the web server because someone asked how it could be done, I went off and done some research to see if a few of my possible ideas would work, turns out it did work, and I learnt something I probably wouldn't use myself - But hey, you never know!
Which PHP-Editor do you think is the best?
in Miscellaneous
Posted
I've switched to using Komodo on my PC now from Maguma. Maguma has had a tendancy to just crash on me quite regularly and it's started to annoy me now. I think the syntax highlighting is better on Maguma than it is on Komoda though, but hey, you can't have it all.