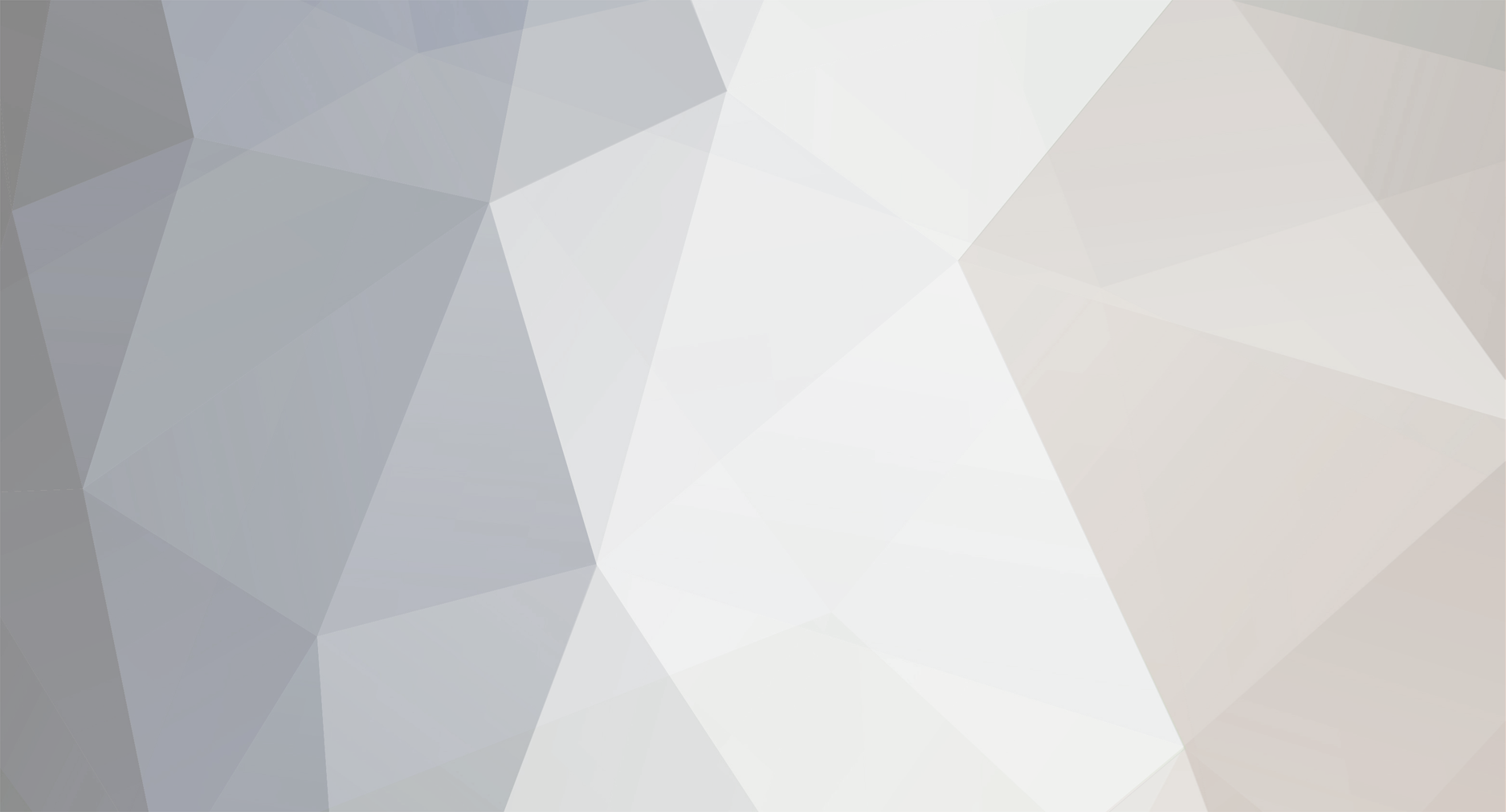
zq29
-
Posts
2,752 -
Joined
-
Last visited
-
Days Won
1
Posts posted by zq29
-
-
A guy over at smiledsoft.com has written a class for creating compressed archives within PHP. I've used it within several projects, does the job nicely.
-
[quote author=kenwvs link=topic=100421.msg396289#msg396289 date=1152792928]
Positive versus Negative
Depending on whether you want it to come back true or false?
[/quote]Correct. -
I'm not sure if this will work, but could you do it with a mix of JavaScript and PHP?
[code]if(valid) {
echo "<script type='text/javascript'>document.myformname.submit();</script>";
} else {
//print error messages
}[/code]
Alternatively, you could store the information you want to pass within a session variable, or even pass it through the URL... -
You can work it out from the cities grid references with a bit of Pythagoras. There are a number of databases about online with postcodes/references for free. Quick search on Google should help you out.
[code]<?php
function distance($na,$ea,$nb,$eb) {
$dist_n = $na - $nb;
$dist_e = $ea - $eb;
$hypot = sqrt(($dist_n * $dist_n) + ($dist_e * $dist_e));
return "Distance between $na(N) $ea(E) and $nb(N) $eb(E) is: ".round($hypot/1000,2)."kms";
}
?>[/code] -
Ok, reading back through your original post, I'm not following you 100%. So lets clear this up...
Originally, you used your code [i]without[/i] the header and your browser returned a bunch of text.
Then, you added the header and your browser returned the expected image.
You want to know why it does not work as expected without the header?
It does this as there is nothing telling the browser that it is an image, it's opening it as a plain text file I guess and unable to decode it correctly as it's not actually plain text. Therefore you add the header to tell the browser what to expect the file output to be. An alternative is to remove the header, and reference the file as an image in another script with the image tag: <img src="imagescript.php" alt="" border="0px"/> -
I think this is something that you need to set when creating the pdf file within Acrobat Pro.
-
Heh, I have had a pretty much exact same experience with one of my clients, except mine started in January 2005 and is finally being sorted this week. I personally haven't found it that frustrating, yeah they still have ~£500 (~$920) left to pay and all I have to do is dump their provided content into a few pages but I have had plenty more work in the mean time to keep me busy. But for clients like this, I just don't drop everything I'm currently working on to please them when they're finally ready.
Yeah they're a pain in the arse, but every industry has them. Oh, and I also have had a few clients that pay their invoices 30-60 days after they have been issued, this appears to be standard practices in some industries, that time delay. Don't know why. -
Use a temporary image with an auto-generated filename. That way, in the unlikely event that two people submit at exactly the same time, two different images will be created.
-
You would pretty much just compare the timestamps...
[code=php:0]<?php
if($timestamp1 > $timestamp2) echo "Timestamp 1 is more recent than timestamp 2";
?>[/code] -
Posting up your code might be a good idea ;)
-
Very possible, although I personally would have taken a completely different path and gone with a database driven solution... But onto your method, you could do [i]something[/i] like this:
[code]<?php
$k = (isset($_GET['k'])) ? $_GET['k'] : 0; //Get they array key from the URL
$files = glob("path/to/your/files/*.txt"); //Get the list of files from your directory, they should be auto-sorted by default.
$content = file_get_contents($files[$k]); //Get the content of the correct file in the $files array
echo $content;
?>[/code]
If you want to go back a file, increment $k - myscript.php?k=1 - Would go back a file. -
Can we see the query?
-
You could use the copy() function as the basis of your script, then run it periodically with a cron job.
-
Correct me if I have the wrong end of the stick here, but you're only querying for three 'items' instead of five. skill1, skill2 and skill3 from the members table...
-
If you want someone to write this for you, let me or another mod know and your post will be moved to our freelancing forum. If you want to give it a go yourself, you could look up the following functions in the manual:
glob() - To get the conents of a directory, loop through the output with a foreach() loop.
filemtime() - To get the time/date that the file was last modified on.
You can chuck all of the returned data into an array, sort it by the date/time and only use the first 10 keys. -
[code]<?php
$array = array();
$result = mysql_query("SELECT `col1`,`col2`,`col3` FROM `table`") or die(mysql_error());
while($row = mysql_fetch_assoc($result)) {
$array[] = $row['col1'];
$array[] = $row['col2'];
$array[] = $row['col3'];
}
$array = array_unique($array);
sort($array);
?>[/code] -
isset() is used to check if a variable has been set/initialised. It is used in the example you have given where both your form and its validation are in the same script. if you have a button with the name "submit" and run if(isset($_POST['submit'])), this checks if the button has been pressed, if so, it then goes on to validate the submitted fields.
-
The backslashes in your example are used to escape the double quotes. Alternatively you'd have:
[code=php:0]$query = "select * from the_table where 1st_field like '%$trimmed%' order by 1st_field";[/code] -
You could do it this way:
[code]function checkEmail($email) { //Check Email Format
if(empty($email)) {
return true;
} else {
$pattern = "/^[A-z0-9\._-]+"
. "@"
. "[A-z0-9][A-z0-9-]*"
. "(\.[A-z0-9_-]+)*"
. "\.([A-z]{2,6})$/";
return preg_match ($pattern, $email);
}
}[/code] -
You actually don't even need to close it most of the time as the link to the server will be closed as soon as the execution of the script ends. You only need to close it with mysql_close() if you have a specific reason to do so.
-
Maybe you should try removing the @ symbol from the front of your call to the mail() function.
-
They both have their uses, as well as their pros and cons. But I personally prefer to use sessions when I have the choice.
-
Oh, I understand now. There is no 'global' way of embeding media files into a webpage as each different type of media file is embeded slightly different.
For example, you can embed a video file, within a Windows Media Player window with the following code:
[code]<object id='MediaPlayer' width='292px' height='290px' classid='CLSID:22D6F312-B0F6-11D0-94AB-0080C74C7E95' standby='Loading Windows Media Player components...' type='application/x-oleobject'>
<param name='FileName' value='videos/$row[filename]'>
<param name='ShowControls' value='true'>
<param name='ShowStatusBar' value='false'>
<param name='ShowDisplay' value='false'>
<param name='autostart' VALUE='false'>
<embed type='application/x-mplayer2' src='videos/$row[filename]' name='MediaPlayer' width='292px' height='290px' ShowControls='1' ShowStatusBar='0' ShowDisplay='0' autostart='0'>
</embed>
</object>[/code]
If you do a bit of a search on Google it's pretty straight forward to find out how to include other types of media, aswell as the list of other parameters you can use in the above example. -
Unfortunatly you won't be able to do this with any ease - The value attribute for file inputs is ignored by all of the popular browsers for security reasons.
Date Calculation
in PHP Coding Help
Posted
[b]EDIT:[/b] Beaten to it!