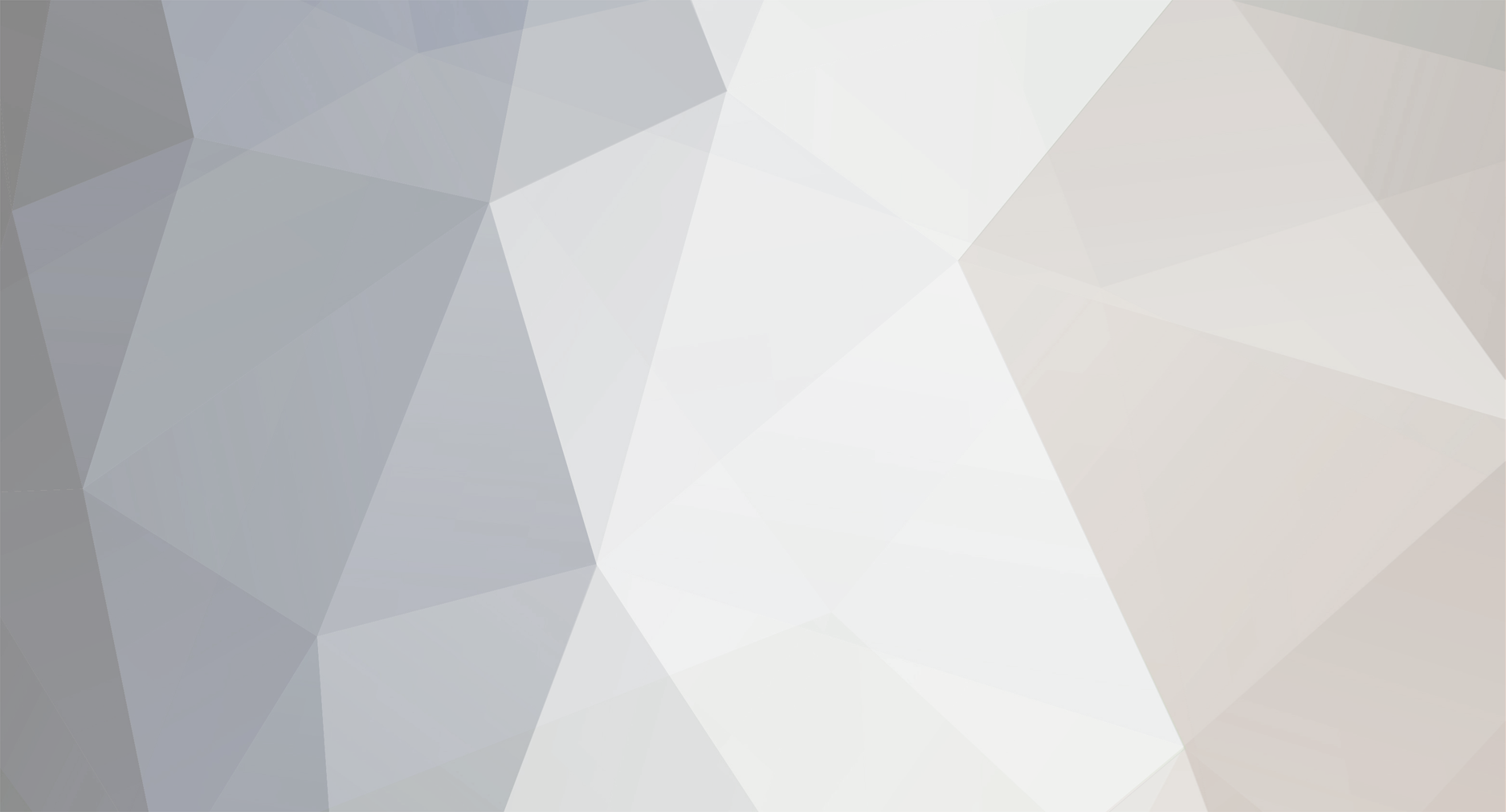
zq29
-
Posts
2,752 -
Joined
-
Last visited
-
Days Won
1
Posts posted by zq29
-
-
[code]<?php
//You forgot to edit this line when you changed the arrays name:
foreach($tmp as $k => $v) {
$new[] = $array[$k];
}
//It needs to be:
foreach($tmp as $k => $v) {
$new[] = $pricearray[$k];
}
?>[/code] -
POST is a method of passing data between scripts. Here's an example:
[code]<form name="test" action="next.php" method="POST">
<input type="name" name="name"/>
<input type="submit" name="submit" value="GO!"/>
</form>
//PHP page "next.php"
<?php
echo "Hello $_POST[name]";
?>[/code] -
array_diff()
array_intersect()
Check the manual for details. -
[quote author=lional link=topic=101254.msg400611#msg400611 date=1153416583]
If somebody turns off javascript o their browser will this ten stop functioning
[/quote]Yes. -
The x and y coordinates define the basepoint of the first character (roughly the lower-left corner of the character). Not the top left.
-
No problem :)
-
[code]<?php
$im = imagecreatefrompng('$template');
//Change to...
$im = imagecreatefrompng($template);
//Or...
$im = imagecreatefrompng("$template");
//Because...
$foo = "bar";
echo $foo; //Displays "bar"
echo "$foo"; //Displays "bar"
echo '$foo'; //Displays "$foo"
?>[/code] -
[quote author=thorpe link=topic=101238.msg400411#msg400411 date=1153402698]
Um... phpversioin(), funny enough.
[/quote]Spelt phpversion() though, mind ;) -
Heres a commented version:
[code]<?php
//An array of arrays containing our data
$array = array(array("ashop.com","A Shop","1.20","In Stock","5"),
array("bshop.com","B Shop","3.20","In Stock","3"),
array("cshop.com","C Shop","2.20","In Stock","10"),
array("dshop.com","D Shop","0.20","Out of Stock","8")
);
$tmp = array(); //A temporary array we will be using
//Loop through our main array filling our temp array with just the prices,
//setting the temp array keys the same as the keys in our main array.
foreach($array as $k => $v) {
$tmp[$k] = $v[2];
}
//Sort our temp array (of prices) from lowest to highest,
asort($tmp);
$new = array(); //An array that will contain all of the data in order
//Loop through our temp array of prices, filling our new array with the full contents of our original array,
//this time ordered by price
foreach($tmp as $k => $v) {
$new[] = $array[$k];
}
//Show the contents of our final array
echo "<pre>"; print_r($new); echo "</pre>";
?>[/code]
Here, $new[0] will allways hold the details of the entery with the cheapest price, so $new[0][1] holds the shop name, the shop URL is in $new[0][0] and $new[0][2] holds the price. -
Not that I'm aware of. Can you not ask them to compile / enable GD support?
-
Sorry, I really don't know what to suggest at this point, there doesn't appear to be anything obviously wrong with your code... Hopefully someone else will read your thread and offer some suggestions.
-
Hows this?
[code]<?php
$array = array(array("ashop.com","A Shop","1.20","In Stock","5"),
array("bshop.com","B Shop","3.20","In Stock","3"),
array("cshop.com","C Shop","2.20","In Stock","10"),
array("dshop.com","D Shop","0.20","Out of Stock","8")
);
$tmp = array();
foreach($array as $k => $v) {
$tmp[$k] = $v[2];
}
asort($tmp);
$new = array();
foreach($tmp as $k => $v) {
$new[] = $array[$k];
}
echo "<pre>"; print_r($new); echo "</pre>";
?>[/code] -
What kind of table? Another database table, or a HTML table?
-
Could you give us some sample data?
-
Have you tried using file() instead of file_get_contents()? Also, what operating system is the code running on, as some OS's recognise a newline as \r and others use \n. You can check your arrays contents with print_r($array); <- allyways helpful when debugging!
-
Where is your data comming from? If you're pulling from a database, you can order it at that level:
[sql]SELECT * FROM `table` ORDER BY `age` DESC, `surname` ASC, `forename` ASC[/sql] -
First off, a tip:
[code]<?php
$theFile = file_get_contents('file.txt');
$lines = array();
$lines = explode("\n", $theFile);
//The above can be replaced with just:
$lines = file("file.txt");
?>[/code]
Maybe you could give us an extract of file.txt? You are aware that for there to be a match, "string" has to be sitting on a line of its own, right? Not in the middle of a larger string... -
Ok, slightly modified version, this one colours slected letters different colours. Plus I took out some stuff that wasn't really neccesary. Commented this time...
[code]<?php
$str = "the quick brown fox jumped over the lazy dog"; //The string!
//Option to auto generate an array of letters/colours
//$letters = array();
//foreach(range("a","z") as $l) $letters[$l] = "#".str_pad(dechex(rand(0,255)),2,"0",STR_PAD_LEFT).str_pad(dechex(rand(0,255)),2,"0",STR_PAD_LEFT).str_pad(dechex(rand(0,255)),2,"0",STR_PAD_LEFT);
$letters = array("a" => "#ff0000","b" => "#00ff00","c" => "#0000ff"); //Our array of letters and colours
$new = ""; //This will hold our final string
for($x=0; $x<strlen($str); $x++) { //Loop through our string letter by letter
$l = $str{$x};
if(array_key_exists($l,$letters)) { //If the current letter in the string exists in the letter/colour array, colour it.
$new .= "<span style='color:$letters[$l]'>$l</span>";
} else {
$new .= $l; //If it doesn't exist, just print it normally
}
}
echo $new; //Echo our new coloured string!
?>[/code] -
Think I might have gone about this the long way around, but here's what I dreamed up:
[code]<?php
$str = "hi my name is redarrow i like my motorbike its class";
$tmp = array();
for($x=0; $x<strlen($str); $x++) {
$tmp[] = $str{$x};
}
$letters = array("a","b","c");
$new = "";
foreach($tmp as $l) {
if(in_array($l,$letters)) {
$new .= "<span style='color:#ff0000'>$l</span>";
} else {
$new .= $l;
}
}
echo $new;
?>[/code] -
Technically, gifs and 8bit pngs do not support different opacities, other then totally transparent or not transparent at all. So you'd be looking at replacing a range of colours in the palette. Looking at the manual, I am unsure of how you'd easily get a list of all of the colours in the palette, so it looks like it might be a case of checking each pixel. This option would also then work with truecolour images too.
I'm personally working on a colour replacement script at the moment for replacing colours in photographs, although my script only replaces the hue (still working on the saturation and value - Having difficulty trying to get it to work there).
So here's what I have found out so far - You'll need a range of colours you want to replace - a 'threshold'. The best awy I could work out how to do this is to convert the colour from hexadecimal, to RGB, and then from RGB into HSV. I chose HSV because of how it works makes it a lot easier to select 'similar' colours, or in my case hues. If you are not clued up on the HSV model there's a good explination on Wikipedia, but basically, HSV is made up of Hue, Saturation and Value. Hue ranges from 0 - 360 degrees (normally displayed as a 'ring' of merging colours), Saturation and Value are pretty much other names for Brightness and Contrast I believe and each range from 0 - 100%.
When seeing the colour wheel, you'll see why this is going to be the easier option for working out the 'range' or colours you want to change.
You'll need to loop through each pixel in the image with two for loops, one nested inside the other to loop through both the x and y coordinates, and then check each colours hue to see if it is in your selected 'range' by doing the conversion and checking if its hue (degrees) lies within the threshold of your predefined (converted) hue. If so, change the pixels hue (this time, doing the conversion in reverse - HSV -> RGB -> HEX).
Thats pretty much it, in a nutshell. I think it's a good starting point for you :)
The formulae for the conversions, HEX - > RGB can be seen in my above example, made a bit clearer in Barands adaptation. And RGB -> HSV, there is a formula on the Wikipedia entery for HSV I think... -
[code=php:0]echo session_id();[/code]
Does that work? -
Have you tried using file_get_contents() ?
-
For a gif or an 8bit png, you can change the specific color in the palette:
[code]<?php
$image = imagecreatefromgif("image.gif");
$from = "ff0000";
$to = "0000ff";
$c1 = sscanf($from,"%2x%2x%2x");
$c2 = sscanf($to,"%2x%2x%2x");
$index = imagecolorexact($image,$c1[0],$c1[1],$c1[2]);
imagecolorset($image,$index,$c2[0],$c2[1],$c2[2]);
header("Content-Type: image/png");
imagepng($image);
?>[/code]
If you are wanting to do this with a true colour image, such as a jpeg, it's a little more involving - The only way I have thought about doing this is checking/changing the colour of each pixel (pixel by pixel). -
[code=php:0]exec("nohup /wwwroot/htdocs/test/ticker/tick_test.sh",$output);
echo "<pre>"; print_r($output); echo "</pre>";[/code]
Check out [url=http://www.php.net/exec]the manual page for exec()[/url] for any further details regarding the workings of the function.
Calculating Age
in PHP Coding Help
Posted
Ummm, That would have been right in 1920. Unix time functions don't work on dates before '1970-01-01'
[/quote][quote author=brown2005 link=topic=101345.msg401128#msg401128 date=1153484750]
well that wasnt my own code, it was the code someone else gave to me, so i will change it to your code..
SO NOT MY FAULT
[/quote]
That is a variation of some code I posted the other week, looking back on it, it's not the most ideal solution (there's a bug in there too I have noticed). But it does work on dates prior to the epoc on some installs. Negative timestamps are supported on a *NIX/PHP5 combo I believe...