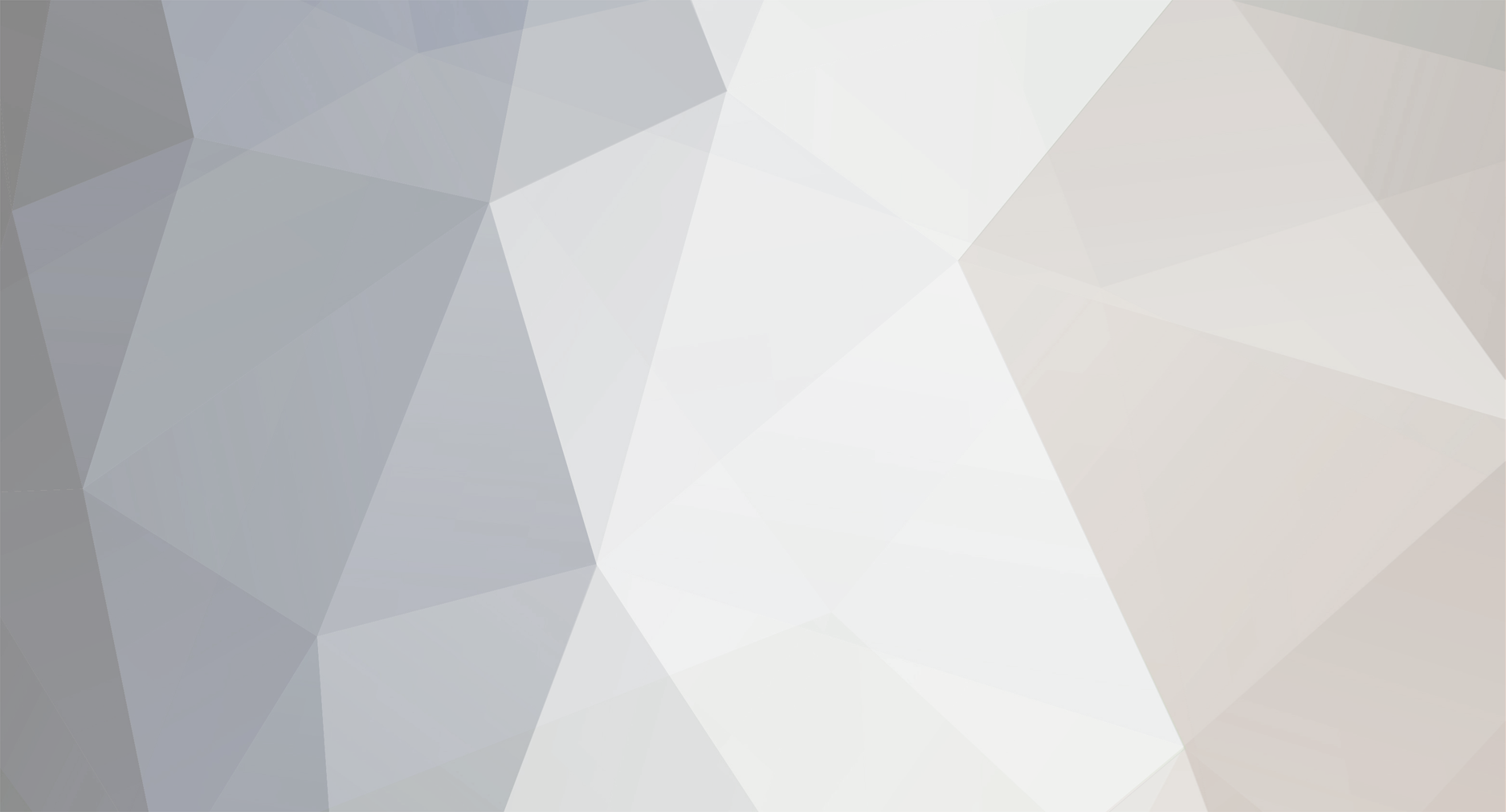
joe92
Members-
Posts
304 -
Joined
-
Last visited
Everything posted by joe92
-
Can you be a bit more specific please. Are you trying to validate the data to make sure no numbers are passed through? An example input and desired output would be very helpful.
-
Could you give an example of input and desired output? What silkfire has given will match the literal string 'A-Za-z ,'. I'm guessing for your first question you were looking to match something more like 'said, '? In which case you will need to alter the pattern to look like: /[A-Za-z]+, / Placing the A-Z in the square brackets makes it a character class and will search for letters A to Z. Not inside the square brackets is just looking for the literal string 'A-Z', not the required result right? Also, you will not want the ^ sign at the beginning and the $ sign at the end. That will mean that the regex has to match from the beginning (^) till the end ($) of the input string. Again I'm presuming as you didn't provide an example with your question, but it sounds like you are looking for a match in a large section of text? Hope this helps, Joe
-
This would be tricky, but I think regex could possibly help you here. Try searching for something the begins with a variation \r\n and is at the end of the textarea, then replace it with a string consisting of nothing. Give this a try; function delete(){ var textarea = document.getElementById('area'); var string = teatarea.value; var new_string = string.replace(/\r?\n.?*$/, ''); textarea.value = new_string; } This is untested but should be a good starting point, Joe
-
No problem. Don't forget to mark it as solved
-
Wrong forum board By the way, that regex will allow for patterns such as RF99999999999, and also 99999999999. Characters don't need to go in character classes (the square brackets) and in your case shouldn't. The beginning of your regex says ([FR]{2})* That is searching for an F or an R, twice. So will match, FF, FR, RF and RR. You also say match this between zero and infinity times (the *). So it could also match '' or 'FFFRRRRRRRFRFRFFFRFRFR' - as that is F or R twice, eleven times (which is between zero and infinity). You see where I'm going with this right? You need to be matching a literal string at the beginning if it always begins with 'FR'. The regex you need is: ^FR[A-Za-z0-9]{2}\d{9}$ Hope this helps, Joe
-
change background image of div from textfield
joe92 replied to joshgarrod's topic in Javascript Help
function changebg() { var docEl = this.parentNode.getElementsByTagName('greenhighlight'); docEl.style.backgroundImage = "url('images/form-highlight-bg.jpg')"; } You are searching for elements by TagName... .greenhighlight {float:left; width:980px; height:60px;} greenhighlight is a css ClassName... Searching by tag name is used to search for tags, i.e. divs or span's etc. You need to search for your class name, so change the function to say 'getElementsByClassName' and that should sort it. Hope this helps, Joe -
Hi there, what your asking for will require more than just regex I reckon. The following regex will find the variables: /([$][a-zA-Z_][a-zA-Z0-9_]*)/sm The only problem I foresee is that I was using this regex indiscriminatingly to find all variables and replace. You have three unique variables all with all unique meanings. I don't know what your current attempt at this is as you have provided no code, but I think you will probably have to run a preg_match_all using this pattern and filter out the results using array_unique on the matches to grab the unique values. Then you know where each variable is, you can go about finding what they each mean and execute the mathematical function within? Good luck! Joe
-
Use the javascript match to search for brackets, and only execute the replace if they are found. Something along the lines of var inputText = 'this is my text. I want to (change this).'; if(inputText.match(/\([^)]*?\)/g)) { var outputText = inputText.replace(/\([^)]*?\)/g, "(new variable)"); } else { alert('No brackets found'); }
-
Yes, I think you would need to use regex and javascripts replace function. Without testing I think it would go something along these lines: var inputText = 'this is my text. I want to (change this).'; var outputText = inputText.replace(/\([^)]*?\)/g, "(new variable)"); Hope this helps, Joe
-
Close the speech marks after seconds.
-
Use the JavaScript match method. Try if(txtfield1.match(/ /g)) //g stands for global, means it will keep looking through entire string rather than stop at the first character if it returns false { alert('You entered a space.'); } else{ //continue with function } P.s. Please use code tags to display code. It's the button with a '#' on it.
-
You can't enclose a variable in single quotes, it will read it as a literal. The options would be to either end the singles, place the variable in, and then start the singles again $numincorrect = '<div id="incorrectnum">Sorry, that number was not "'.$code.'".</div>'; Or to have the string all in speech marks, as variables get read as variables in speech marks, and cancel out the other speech marks that you need within the string $numincorrect = "<div id=\"incorrectnum\">Sorry, that number was not \"$code\".</div>";
-
Calling a javascript function with a select option?
joe92 replied to son.of.the.morning's topic in Javascript Help
The usual event gang, onclick etc. The best to use is onchange though as it will not do anything if you select the same option. <select onchange=""> Unless your asking about executing the js from within the option tag itself (your question is a bit ambiguous). Then I believe no events are supported by the option tag. However, if you are trying to find the value of the option that the user has selected, you just have to ask what the value of the select tag is. It will equal the selected option tag. -
Add a new value to the current value of a textbox
joe92 replied to son.of.the.morning's topic in Javascript Help
Did you google for this answer? There are many threads around the internet regarding this subject and are not hard to find. Yes you can. The IE route is different to the other's (as usual), requiring the creation and manipulation of ranges. All the other browsers use just selectionStart and selectionEnd along with setSelectionRange. -
Calling a javascript function with a select option?
joe92 replied to son.of.the.morning's topic in Javascript Help
Yes. -
Yeah sure, when you download PHP Mailer it comes with quite a few example scripts. It uses OOP. Here's a sample script I edited to use only the options I need: <?php $HTML_message = '<div style="color:#FF000;margin-left:5em;">HTML Testing message with red text and a margin</div>'; require_once '../class.phpmailer.php'; $mail = new PHPMailer(true); //defaults to using php "mail()"; the true param means it will throw exceptions on errors, which we need to catch $mail->AddReplyTo('[email protected]', 'First Last'); //who to reply too $mail->SetFrom('[email protected]', 'First Last'); // who sent it $mail->Subject = 'PHPMailer Test Email'; // title of email $mail->AltBody = 'To view the message, please use an HTML compatible email viewer!'; // optional non html message- MsgHTML will create an alternate automatically $mail->MsgHTML($HTML_message); // your html message $mail->AddAttachment('images/phpmailer.gif'); // attachment $mail->AddAttachment('images/phpmailer_mini.gif'); // another attachment $mail->Send(); // send the email! ?> Have you made sure the file is definitely being uploaded?
-
I have no idea how to do this with jQuery. However, I have just been learning how to do it with PHP Mailer and can tell you it is as simple as anything with this script (takes about half an hour to get used to everything about it). You might want to consider looking into it $mail->AddAttachment("Document to attach.extension", "[optional]Alternative name for attachment"); // attachment
-
Hmm, not entirely sure on what you need now However, something like this might work. Again, untested: <?php $variable = 'answer_comment'; preg_match("/(?<=$variable)(\d+#[a-zA-Z]\d+(:\d+)?)+?(?![a-zA-Z]{2})/sm", $subject, $match); That will look for: - Something that begins with the variable. - Is constantly looking ahead to make sure that: Two letters don't match (indicates a word) - The following will happen between one and infinity times - Starts with a digit. - There is at least one and infinity digits. - Followed by a hash symbol - Followed by one letter, upper or lower case - Followed by between one and infinity digits - Between one and zero times the following will happen - Followed by a colon - Followed by between one and infinity digits If you have another variation of a regex question, before asking it, please go to http://www.regular-expressions.info/tutorial.html and read up on regex. If, if, you still cannot understand how to get what you need, then ask the question. Hope this helps, Joe
-
Not sure on solving this specific problem, however there is absolutely no point in over complicating your regex by using (?<!\w) and (?!\w). If you need to make sure your pattern is not contained within a word, use the word boundaries metacharacter. This makes sure it is matching a whole word only. Yours would become: '#\b<3\b#i' Also, the i modifier means case-insensitive. There is absolutely no point in using it for this pattern. A 3 does not have a lower or upper case, neither does a <. '#\b<3\b#'
-
Will the only non digits always be '#c'? Or could it be '#g' or even '$Ylpqw'?
-
Thank you very much! This has been annoying me for weeks.
-
Is it possible to designate an email address to a folder in Mozilla Thunderbird? So, for example, I create a folder called 'PHP Freaks' in my Thunderbird account. Is it possible to label the email address '[email protected]' and have every email from this account bypass the main inbox folder and go directly to the folder 'PHP Freaks'? Hotmail implemented this feature a while ago and it is brilliant. Made me like using Hotmail again. Does anyone of a way to do it with Thunderbird? I've searched Google but nothings coming up. Cheers, Joe
-
You will need to use a look-behind. Be aware a look-behind has to be a fixed length (i.e. not a pattern). It would go something like: [This is untested] <?php $variable = 'answer_comment'; preg_match("/(?<=$variable)[^A-Za-z]+/sm", $subject, $match); That's looking for something that begins with the variable and is not letters and can happen between one and infinity times. Hope that helps, Joe
-
I think you are missing the point here. JavaScript is a programming language. jQuery is not a programming language, JQuery is a framework of the language JavaScript. Essentially, jQuery is a massive library of functions which are written in JavaScript. It exists to save you time. MooTools is also a framework/library of JavaScript. With regards to making a tooltip field. I would use CSS for that - http://sixrevisions.com/css/css-only-tooltips/ Live examples of CSS tooltips As for creating a tabbed menu. It depends entirely on what you want the tabbed menu to do. If its something fancy, you will most likely end up using a combination of CSS and JavaScript (or jQuery). This guy has compiled a massive list of cool menu's to look at - http://www.1stwebdesigner.com/css/38-jquery-and-css-drop-down-multi-level-menu-solutions/ And, finally, in regards to learning JavaScript, I would say at least learn some before you use a framework. Learn how JavaScript works and how to make simple functions. Else you'll never understand why your framework works like it does (and even then, you'll most likely still have difficulties understanding some of it). Also, if you understand JavaScript yourself, you'll be able to whip up simple functions in just as much time as it would take to find to find it in the library. Hope that clears things up, Joe
-
JavaScript has not become overhauled. It has simply been left aside as people would rather use the framework of JavaScript, jQuery, than learn actual JavaScript. This is mainly because there are a lot of cross browser compatibility issues with pure JavaScript which are all ironed out in the functions of jQuery. Its just a matter of choice. Learn the core language, or learn the framework which utilises it?