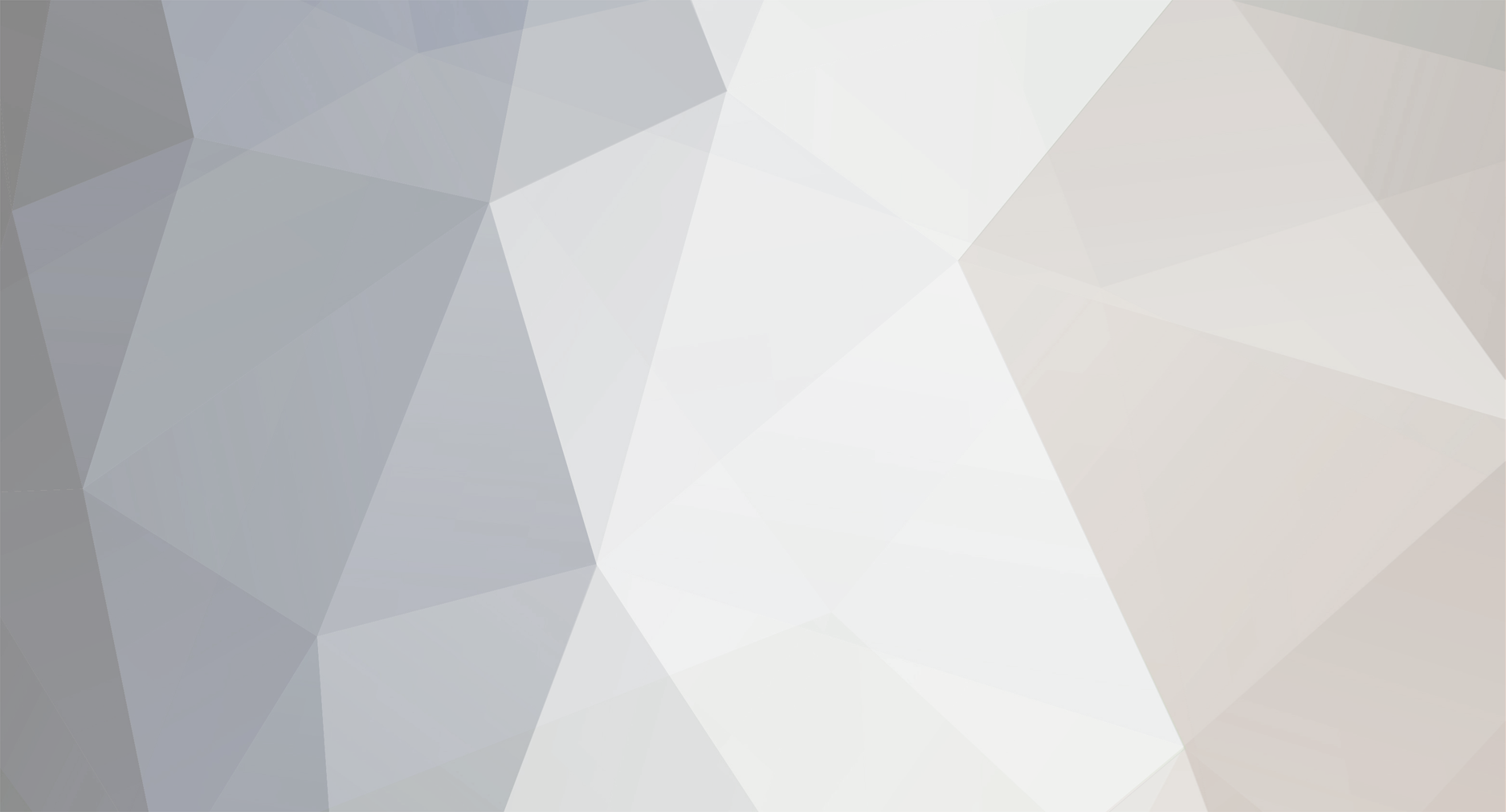
joe92
Members-
Posts
304 -
Joined
-
Last visited
Everything posted by joe92
-
Cheers! That's sorted it
-
Add between the two queries: <?php require_once('Connections/myConnect.php'); $detnum = $_GET['Details']; $detinfo = mysql_query("SELECT* FROM `Agency Stores - Table 1` WHERE F11=".$detnum); $det = mysql_fetch_array($detinfo); if(mysql_num_rows($detinfo)) { $salesnum = $det['F3']; $salesinfo = mysql_query("SELECT* FROM `By Agency Store _` WHERE store_store=".$salesnum); $sales = mysql_fetch_array($salesinfo); if(mysql_query("SELECT* FROM `By Agency Store _` WHERE store_store=".$salesnum)) { //do whatevers going in here } } else{ echo 'Row does not exist'; } ?> That is saying, if rows are returned, carry on with the script. Else echo 'Row does not exist'. Info on mysql_num_rows
-
I am setting up PHPMailer to send pdf's from my server and I have just come into the following error: The code in question is this (I've pointed out the lines mentioned above): <?php /** * Encodes attachment in requested format. * Returns an empty string on failure. * @param string $path The full path to the file * @param string $encoding The encoding to use; one of 'base64', '7bit', '8bit', 'binary', 'quoted-printable' * @see EncodeFile() * @access private * @return string */ private function EncodeFile($path, $encoding = 'base64') { try { if (!is_readable($path)) { throw new phpmailerException($this->Lang('file_open') . $path, self::STOP_CONTINUE); } if (function_exists('get_magic_quotes')) { function get_magic_quotes() { return false; } } if (PHP_VERSION < 6) { $magic_quotes = get_magic_quotes_runtime(); set_magic_quotes_runtime(0); //<-- Here } $file_buffer = file_get_contents($path); $file_buffer = $this->EncodeString($file_buffer, $encoding); if (PHP_VERSION < 6) { set_magic_quotes_runtime($magic_quotes); } //<-- Here return $file_buffer; } catch (Exception $e) { $this->SetError($e->getMessage()); return ''; } } So my question is, how can I get rid of this error? How should I best edit this function to no longer use the deprecated set_magic_quotes_runtime? Any help is appreciated, Joe
-
Route 3 of josh's explanation is by far the easiest route to logically follow. And when you return to your own script in a weeks time or more, being able to logically understand and follow it will save you so much time and stress. Structure your scripts in accordance with route 3
-
The mistake lies in that are referencing the wrong name. <input type="text" name="Username" id="Username" /> That is your username field, it has a name 'Username'. The name of an input field is what you place in the quotation marks when you wish to receive it. Hence, you need to change your $_POST['name'] to $_POST['Username'] If you change the print to just print_r($_POST); it shall show you all of the post in an array. This is very helpful in bug finding. Hope this helps, Joe
-
Can you give an example of the input please? Can you also make sure that $_POST['name'] is what you want it to be. Put print_r($_POST['name']); before the if statement to print it at the top of the page, and make sure it is the required/designated field. Ha, never knew about that ctype. Thats a handy little set of functions!
-
The ! in php means 'not'. Your if statement no longer includes the ! so it is matching the user name in the preg_match and executing the echo rather than the mysql executions. Incorrect names such as $%^& are not matching the preg_match so are being executed in the else. They then don't match any records in the database so echo 'Account doesn't exist'. Its the wrong way round. What would logically make sense in my head would be to keep the preg_match like it is but rearrange the other code. I.e. <?php if(isset($_POST['Login'])) { if(preg_match('/^[A-Za-z0-9]{5,20}/',$_POST['name'])){ // before we fetch anything from the database we want to see if the user name is in the correct format. $query = "SELECT password,id,login_ip FROM users WHERE name='".mysql_real_escape_string($_POST['Username'])."'"; //do all the other mysql stuff } else{ echo 'Invalid Username.'; } To keep it in your format though will require you placing the ! back at the start of the preg_match since that regex is looking for correct characters. Furthermore, you will need to place the $ at the end of the pattern otherwise scenario two of my previous post with the username obiwan"£$%^&* will still be matched. Use this as the pattern /^[A-Za-z0-9]{5,20}$/
-
Please use code tags when posting code. Its the button with a picture of the hash symbol '#'. It makes it so much easier to read. Ereg functions use POSIX language in the pattern. Preg functions use PCRE language in the pattern. That is the difference between the two. The reason it is still not working is because you haven't placed the pattern in delimiters. Secondly the pattern will match once and return true as there is no repetition, so usernames such as o"£$%^&* would match because the o at the beginning would be matched and the engine would stop reading. To match the entire username you will have to tell the pattern to repeat. If your standards are a minimum length of 5 and a maximum of 20 for your usernames, this would become if(!preg_match('/[A-Za-z0-9]{5,20}/',$_POST['name'])) However, you will still have a problem in that names such as obiwan"£$%^&* will still match because the first 5 characters are proper characters so the function will return true. To get around this, you want to tell your regex that the start till the end of $_POST['name'] are all to be matched in the regex. You would do that by placing a ^ at the start and a $ at the end, making it if(!preg_match('/^[A-Za-z0-9]{5,20}$/',$_POST['name'])) Actually, in a character class it denotes 'not the following characters'. I.e. [^a] would mean not a.
-
You need delimiters to make it work. Forward slashes are the most common delimiters if(!preg_match('/[^A-Za-z0-9]/',$_POST['name'])) Also you have a double negative there. You are saying !preg_match and the character class, [], is saying not these characters A-Za-z0-9. Therefore my username joe92 would not match that regex therefore would be output as an invalid username. You need to remove the ^ in the character class.
-
An else statement is a counterpart of an if statement. You are basically saying: if(statement is true) { //do this code } else { //do this code } In your code, you have placed the else after the while loop. This cannot be executed because you can't be saying: whiles(statement is true) { //do this code } else { //do this code } That would mean the else statement would be constantly running if the while loop wasn't running. It would create an infinite loop and the server would just say "No, I'm not doing that, get stuffed", which it is doing. What you need to do is move the else statement to be right after the if statement whiles both being contained in the while loop. Does that make a bit more sense?
-
This is where taking the extra few seconds to lay out your work nicely helps. I would have spotted the problem in a split second had you indented nicely The problem is that the else statement, after your if(empty($img)) statement, is written after the while loop closes.
-
If no rows are returned $num_rows should be blank, not equal to zero. Therefore change the first if to: if ($num_rows == '') { echo "<p>No pet tributes for the letter.</p>"; } else { //the rest of your code } Edit:: Changed to == '';
-
You'll need to edit the onclick to something along these lines; onclick="document.getElementById('id').disabled = false;" .. Give an id to the textfield and replace the above id with the id of the text field
-
jquery / ajax call to reload a specific "div" ****
joe92 replied to son.of.the.morning's topic in Javascript Help
Yeah, that was my thinking as well. Once the message is in the database the php will write it to the page on next load. The javascript is to please the user who just sent the message by removing the need for a complete page reload. -
jquery / ajax call to reload a specific "div" ****
joe92 replied to son.of.the.morning's topic in Javascript Help
I'm doing an extremely similar thing on a side project of mine, but I haven't implemented it yet so I can only say how I'm planning to go about it. Storing all comment divs within a container div, id=container: 1. Use javascript to do very simple checks like to make sure it isn't blank etc. 2. If checks are ok, store the required details in javascript variables 3. Start an Ajax POST request to send the message and use the GET to send small length details like the name. 4. Update the mysql database and if it was successfully updated send back a success message to the javascript, if failed, a failed one. 5. If successful, create a div in javascript using var newdiv = document.createElement('div'); 6. Set the class/style and any js events to the newdiv and using the details stored earlier set the innerHTML of the newly generated div to be that of the message (and any other details that are needed) 7. Using the following, append the container div with the newly created message div var divToAppend = document.getElementById('container'); divToAppend.appendChild(newdiv); (I create a var because I intend to append multiple items, if only one is needed you could execute that on one line) 8. If it failed on the update just alert them saying so. Hope this helps, Joe P.s. I like your website design. -
You could use pathinfo to get the basename and run the preg_match on that: $url = "http://www.test.com/forums/attachment/f39/745d1267084199-official-ar-m16-m4-picture-thread-dsc00005.jpg"; $getpath = pathinfo($url); $basename = $getpath[basename]; $getpath = preg_match("/^[\d]+/", $basename, $match);
-
preg_match("/(?<=f39\/)([\d]+)/", $thatURL, $match); That will match all the digits after 'f39/' until it stops reading digits, and will then place that match in an array called $match. Hope this helps, Joe
-
Change: $regex = '#<figure><img src="([^\']*)" alt="([^\']*)" /><figcaption>([^\']*)</figcaption></figure>#'; into $regex = '#<figure><img src="([^\']*?)" alt="([^\']*?)" /><figcaption>([^\']*?)</figcaption></figure>#'; Your * quantifier was being greedy and matching everything it could. It needed the ? after it to tell it to lookout for the next literal character that needs to be matched, that being the closing speech mark.
-
No, I mean that regex you provided will change: {{http://variouspictures.tk/wp-content/uploads/2011/07/Cat.bmp||text||text}} into <figure><img src="http://variouspictures.tk/wp-content/uploads/2011/07/Cat.bmp" alt="text" /><figcaption>text</figcaption></figure> Where is the regex to change: <figure><img src="http://variouspictures.tk/wp-content/uploads/2011/07/Cat.bmp" alt="text" /><figcaption>text</figcaption></figure> into {{http://variouspictures.tk/wp-content/uploads/2011/07/Cat.bmp||text||text}} That is the desired question right?
-
That regex you provided in your first post looks like the one to change it back to the <figure>etc.?
-
I don't think it is possible to figure out which operating system the user has using css, however the JavaScript route is very easy. Just place the following in your head; <script type="text/JavaScript"> if (navigator.appVersion.indexOf("Win")!=-1) { OSName="Windows"; //get windows css file } if (navigator.appVersion.indexOf("Mac")!=-1) { OSName="MacOS"; //get mac css file } if (navigator.appVersion.indexOf("Linux")!=-1) { OSName="Linux"; //get Linux css file } </script>
-
Are there any free keyloggers out there that apply to Mac?
joe92 replied to Tinney's topic in Miscellaneous
When I was younger I was inflicted with having k9 web protection on my computer. It didn't bother me when I was 13-15 because I spent more time outdoors than in, but as I got older and started using the computer more I hated it so much I learnt how to hack it and remove it and then installed my own version with my own password on it (could do that because it was free). It might be preferable option for young children using the internet who can't make responsible decisions yet, but don't burden a 16 year old with it because he will not like it. -
Try php.net, it is a wonderful resource, date. If you look down the list you will find which letter you need to input for month-day-year, being just a rearranged version of the Y-m-d you provided, m-d-Y If you wanted it written out longhand like 22nd November 2011 for example you would use, date('js F Y', $variable_holding_time_in_seconds);
-
You can press f5 whiles viewing the source to refresh just the source. Don't know if that's what you mean?