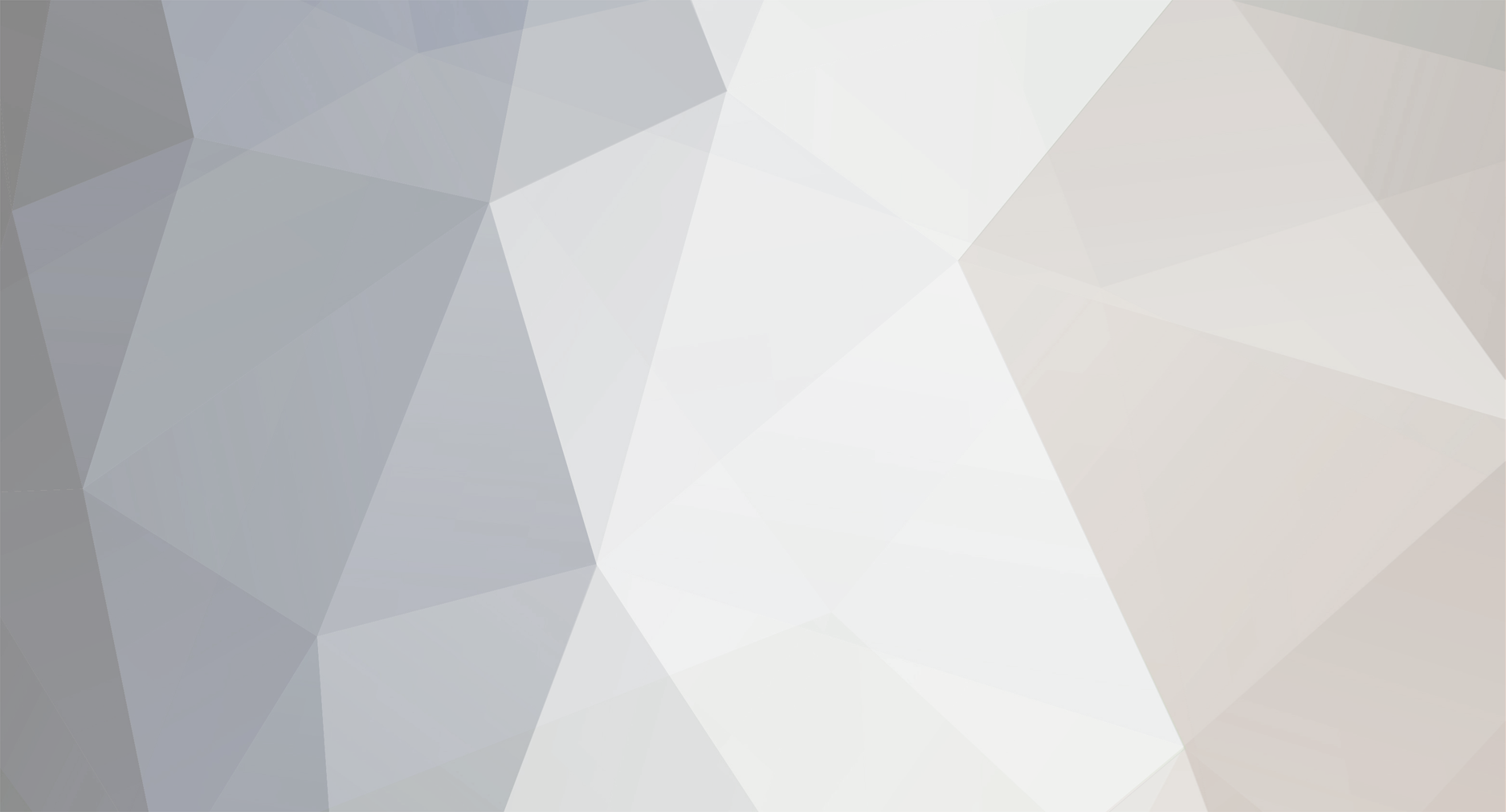
fugix
-
Posts
1,483 -
Joined
-
Last visited
Posts posted by fugix
-
-
you might be able to call the plain array
if (preg_match_all($regexp, $html, $matches)) { echo $matches; }
but if not, then you might have to draw them out manually
if (preg_match_all($regexp, $html, $matches)) { echo $matches[0][0]; echo $matches[][1]; echo $matches[1][0]; }
etc...
-
okay well thats good. Basically what you need is however you got the photoid for the pictures...i think you got it from you database...you need to use that same id as your action in your comment form....thats what im trying to do here....if you know what im talking about you will have an easier time doing it since you coded it
-
try changing your if statement to this
if (preg_match_all($regexp, $html, $matches)) { echo $matches[0][0]; }
-
this article talks about using salt...hope its helpful...http://pbeblog.wordpress.com/2008/02/12/secure-hashes-in-php-using-salt/
-
yeah you could use a foreach() loop to pull out each key and trim each one...not sure if thats what you're looking for
-
try
$strQuery = "select * from Comments where Message LIKE '%$Bad_Words%' order by dtAdded desc";
-
im guessing that your message has multiple words..in this case it will still show everything....lets say your message is something like. "Test Message, crap".....that entire message does not equal "crap" even though it has the word in it...make sense?
-
Notice: Undefined index: submit in /home/ebermy5/public_html/form.php on line 9\
that error means that either $_POST['submit'] hasnt been submitted yet or doesnt exist...do what PFMaBiSmAd said and use isset()
-
i dont see why it wouldn't work, would add extra protection for sure
-
yeah, the query you are using now is efficient for you needs
-
sweet, welcome to phpfreaks!
-
firstly you need to wrap your names in quotes this
<select name=provincias>
should be
<select name="provincias">
-
hey im back...fell asleep last night. i wanted you to change the query code that i posted, for you image_show.php so you would have this
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>What do you know about.......</title> <style type="text/css"> #What { text-align: center; } </style> </head> <body> <?php $hostname='yoddce.com'; $username='yd29'; $password='Ad1!'; $dbname='d9'; $usertable='do'; mysql_connect('yourdddd!') or die(mysql_error()); mysql_select_db("d") or die(mysql_error()); $id = (int)$_GET['id']; if( $id > 0 ) { $result = mysql_query("SELECT `name`, `photopath`, `state` FROM `photo` WHERE `id` = $id") or die(mysql_error()); $row = mysql_fetch_array( $result ); echo " <img src=\"/{$row['photopath']}\" height=\"400px\" width=\"350px\" />". "<br /> "; echo $row['name']. "\n". "<br/>"; echo $row['state']. "\n"; } else { echo 'BAD PHOTO ID'; } ?> <?php $hostname='dm'; $username='yd'; $password='d1!'; $dbname='d9'; $usertable='dto'; $myconn=mysql_connect('yddd!') or die(mysql_error()); mysql_select_db("dd") or die(mysql_error()); $name=$_POST['name']; $comment=$_POST['comment']; $submit=$_POST['submit']; if($submit) { if($name&&$comment) { $query=mysql_query("INSERT INTO comment (id,name,comment) VALUES ('','$name','$comment')"); } else { echo "Please fill out all the fields."; } } $query_Recordset1 = "SELECT * FROM photo"; $Recordset1 = mysql_query($query_Recordset1) or die(mysql_error()); $row_Recordset1 = mysql_fetch_assoc($Recordset1); ?> <body> <div id="comments"> <form action="image_show.php?id=<?php echo $row_Recordset1['id'];?>" method="POST"> <label>Name: </label><br /><input type="text" name="name" input id="name"value="<?php echo "$name" ?>" /><br /><br /> <label>Comment: </label><br /><input type="text" input id="comment"/><textarea name="comment" "cols="25" rows="7"></textarea><br /><br /><br /> <input type="submit" name="submit" value="Comment" /><br /> </form></div> <hr width="1100px" size="5px" /> </body> <?php $query=mysql_query("SELECT * FROM comment ORDER BY id DESC"); while($rows=mysql_fetch_assoc($query)) { $id=$rows['id']; $name=$rows['name']; $comment=$rows['comment']; $linkdel="<a href=\"delete.php?id=" . $rows['id'] . "\">Delete User</a>"; echo '<font color="red">Name:</font> ' . $name . '<br />' . '<br />' . '<font color="red">Comments:</font> ' . '<br />' . $comment . ' ' . ' ' . ' ' . ' ' . $linkdel . '<br />' . '<br />' . '<hr size="5px" width="500px" color="blue" />' ; } ?> </html> </body> </html>
-
yes that will need to be changed to, your form and header need to go to the dynamically created page, not the base page okay so change this
$query_Recordset1 = "SELECT `id`, `name`, `photopath`, `state` FROM photo ORDER BY `name` ASC"; $query_limit_Recordset1 = sprintf("%s LIMIT %d, %d", $query_Recordset1, $startRow_Recordset1, $maxRows_Recordset1); $Recordset1 = mysql_query($query_limit_Recordset1, $myconn) or die(mysql_error()); $row_Recordset1 = mysql_fetch_assoc($Recordset1); ?>
to
$query_Recordset1 = "SELECT * FROM photo"; $Recordset1 = mysql_query($query_Recordset1) or die(mysql_error()); $row_Recordset1 = mysql_fetch_assoc($Recordset1); ?>
-
can i see the form that sends to this page too please
-
can i see your delete script please
-
it is now since i told you to change it, im perplexed as to why it is still not showing an id...what does you "view source" on your browser show the photo id being now?
-
well i know why your photo id is dissapearing, when you click on the photo link
<a href="image_show.php?id=<?php echo $row_Recordset1['id'];?>"><img src='<?php echo $row_Recordset1['photopath'] ;?>' height="100" width="100"/></a>
it is setting the url to image_show.php?id=<?php echo $row_Recordset1['id'];?/>
however, when you post a comment, you have the action set to just image_show.php
therefore when the form is submitted, you are going from a dynamic url to plain image_show.php, thus you lose your id
-
okay so you're going to try this
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>What do you know about.......</title> <style type="text/css"> #What { text-align: center; } </style> </head> <body> <div id="What"><img src="images/what.png" width="477" height="36" alt="do you know something i don't" /></div> <?php $hostname='fd.db.fdffd.d.com'; $username='d'; $password='d!'; $dbname='d'; $usertable='d'; mysql_connect('yd.com', 'yd9', 'Ad!') or die(mysql_error()); mysql_select_db("yd9") or die(mysql_error()); $id = (int)$_GET['id']; if( $id > 0 ) { $result = mysql_query("SELECT `name`, `photopath`, `state` FROM `photo` WHERE `id` = $id") or die(mysql_error()); $row = mysql_fetch_array( $result ); echo " <img src=\"/{$row['photopath']}\" height=\"400px\" width=\"400px\" />". "<br /> "; echo $row['name']. "\n". "<br/>"; echo $row['state']. "\n"; } else { echo 'BAD PHOTO ID'; } ?> <?php $hostname='durce.com'; $username='yod9'; $password='Ad1!'; $dbname='yd'; $usertable='d'; mysql_connect('yd, 'yd9', 'Ad!') or die(mysql_error()); mysql_select_db("yd") or die(mysql_error()); $name=$_POST['name']; $comment=$_POST['comment']; $submit=$_POST['submit']; if($submit) { if($name&&$comment) { $query=mysql_query("INSERT INTO comment (id,name,comment) VALUES ('','$name','$comment')"); } else { echo "Please fill out all the fields."; } } $query_Recordset1 = "SELECT `id`, `name`, `photopath`, `state` FROM photo ORDER BY `name` ASC"; $query_limit_Recordset1 = sprintf("%s LIMIT %d, %d", $query_Recordset1, $startRow_Recordset1, $maxRows_Recordset1); $Recordset1 = mysql_query($query_limit_Recordset1, $myconn) or die(mysql_error()); $row_Recordset1 = mysql_fetch_assoc($Recordset1); ?> <body> <div id="comments"> <form action="image_show.php?id=<?php echo $row_Recordset1['id'];?>" method="POST"> <label>Name: </label><br /><input type="text" name="name" input id="name"value="<?php echo "$name" ?>" /><br /><br /> <label>Comment: </label><br /><input type="text" input id="comment"/><textarea name="comment" "cols="25" rows="7"></textarea><br /><br /><br /> <input type="submit" name="submit" value="Comment" /><br /> </form></div> <hr width="1100px" size="5px" /> </body> <?php $query=mysql_query("SELECT * FROM comment ORDER BY id DESC"); while($rows=mysql_fetch_assoc($query)) { $id=$rows['id']; $name=$rows['name']; $comment=$rows['comment']; $linkdel="<a href=\"delete.php?id=" . $rows['id'] . "\">Delete User</a>"; echo '<font color="red">Name:</font> ' . $name . '<br />' . '<br />' . '<font color="red">Comments:</font> ' . '<br />' . $comment . ' ' . ' ' . ' ' . ' ' . $linkdel . '<br />' . '<br />' . '<hr size="5px" width="500px" color="blue" />' ; } ?> </html> </body> </html>
and let me know what you get
-
okay so let me get organized here when you click this link
<a href="image_show.php?id=<?php echo $row_Recordset1['id'];?>"><img src='<?php echo $row_Recordset1['photopath'] ;?>' height="100" width="100"/></a>
everything works as it should....the picture shows and everything, however when someone writes and comment and hits the submit button...the image does not show anymore?
-
and from where in your code is the
$id = (int)$_GET['id'];
coming from?
this line
$id = (int)$_GET['id'];
is causing all of your problems, if we can just get this sorted out you'll be good
-
maybe, found this tough.
-
il check my cPanel for it
-
sweet, what you were doing by have (!result) was telling it that if the query failed, to echo your 'Never achieved'. But even if the query returned 0 rows it still wasnt failing, so you would never see your empty query message..glad i could help
Query to Exclude Certain Results
in MySQL Help
Posted
i found this code from a website...looks to be what you need...will need to change the query to NOT LIKE and the var values