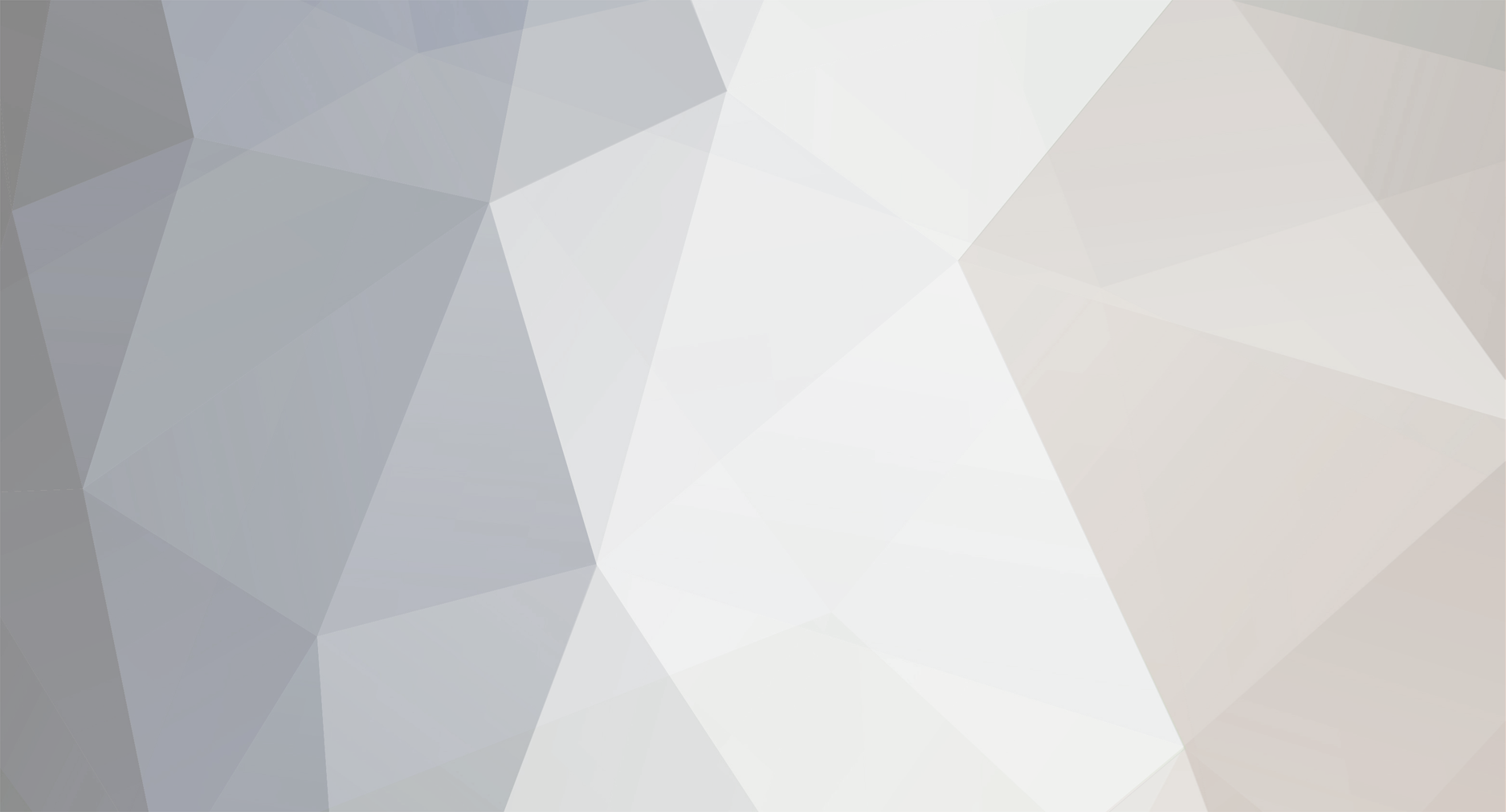
fugix
-
Posts
1,483 -
Joined
-
Last visited
Posts posted by fugix
-
-
well if you arent passing an id through your form then what is the purpose of
$id = (int)$_GET['id'];
then?
-
so what happens when you go ahead an use the copy() funciton?
-
alright try this
$con = mysql_connect($hostname, $username, $password) OR DIE ('Unable to connect to database! Please try again later.'); $db = mysql_select_db($dbname, $con); $query = "SELECT teamname, division_records.division, div_win, div_loss FROM division_records, owners WHERE owners.owner_id = division_records.owner_id AND division_records.year = 2011 ORDER BY division_records.division"; $result = mysql_query($query); $num_rows = mysql_num_rows($result); if ($num_rows == 0) { echo 'Never achieved'; exit; } echo '<ol>'; while ($row = mysql_fetch_array($result)) { echo '<li><b>' . $row['division'] . '</b>: ' . $row['teamname'] . '<br/>'; echo 'Divisional Record: ' . $row['div_win'] . '-' . $row['div_loss'] . '</li>'; } echo '</ol>'; mysql_close($con);
-
yeah, the mysql_seek_data() function will return with an E_WARNING if the result is null
-
so you would like to know how to set up all of the things that you listed for use in your process_form.php page?
-
well insert the proper id into the form action like i said and you should be good
-
what is your actual question?
-
what do you receving when you change your query line to this.
$result = mysql_query($query) or die(mysql_error());
-
i think that you are receiving that error because your form action is not dynamic...meaning that it is not sending an id to itself...so when this
$id = (int)$_GET['id'];tries to retrieve an id...it doesn't receive one, and you get your error, you need to make your form send an id like this
<form action="image_show.php?id=1" method="POST">
and you would replace the one with whatever the id is supposed to be
-
okay i think that it might be because i forgot to put quotes around the variable...try this
$Bad_Words = "crap"; $strQuery = "select * from Comments where Message != '$Bad_Words' order by dtAdded desc"; $queryGetComments = mysql_query($strQuery) or die(mysql_error());
-
if you want to see some cool websites in the making, you should check out the "beta testing" section of the forums.
-
well your bad photoid error comes from this
$id = (int)$_GET['id']; if( $id > 0 ) { $result = mysql_query("SELECT `name`, `photopath`, `state` FROM `photo` WHERE `id` = $id") or die(mysql_error()); $row = mysql_fetch_array( $result ); echo " <img src=\"/{$row['photopath']}\" height=\"400px\" width=\"400px\" />". "<br /> "; echo $row['name']. "\n". "<br/>"; echo $row['state']. "\n"; } else { echo 'BAD PHOTO ID'; }
so what i would do is echo the id before running the if statement to see if i am returning anything
$id = (int)$_GET['id']; echo $id; if( $id > 0 ) { $result = mysql_query("SELECT `name`, `photopath`, `state` FROM `photo` WHERE `id` = $id") or die(mysql_error()); $row = mysql_fetch_array( $result ); echo " <img src=\"/{$row['photopath']}\" height=\"400px\" width=\"400px\" />". "<br /> "; echo $row['name']. "\n". "<br/>"; echo $row['state']. "\n"; } else { echo 'BAD PHOTO ID'; }
-
You really should test more thoroughly. These code pasted directly into a .php file shows the following errors:
Notice: Undefined index: item in /var/www/private_html/projects.smsmarketeers.com/html/scripts/vending_machine.php on line 36 Notice: Undefined index: credit_input in /var/www/private_html/projects.smsmarketeers.com/html/scripts/vending_machine.php on line 39 Notice: Undefined index: submit in /var/www/private_html/projects.smsmarketeers.com/html/scripts/vending_machine.php on line 42 You do not have sufficient funds to purchase a . Notice: Undefined index: submit in /var/www/private_html/projects.smsmarketeers.com/html/scripts/vending_machine.php on line 46 You do not have sufficient funds to purchase a . Notice: Undefined index: submit in /var/www/private_html/projects.smsmarketeers.com/html/scripts/vending_machine.php on line 50 You do not have sufficient funds to purchase a . Notice: Undefined index: submit in /var/www/private_html/projects.smsmarketeers.com/html/scripts/vending_machine.php on line 55 Coke resources depleted. Notice: Undefined index: submit in /var/www/private_html/projects.smsmarketeers.com/html/scripts/vending_machine.php on line 61 Sprite resources depleted. Notice: Undefined index: submit in /var/www/private_html/projects.smsmarketeers.com/html/scripts/vending_machine.php on line 67 Fanta resources depleted.Your current funds accumlate to
The problem is that "submit" is not defined because "submit" is the submit button which is not defined until the form is submitted. I suggested checking to see if the form was submitted a different way, perhaps using $_SERVER variables. For example:
<html> <head> <title>Vending Machine</title> <style type="text/css"> * { font-size:12px; font-family:Arial; margin:0px; outline:0px; padding:0px; } body { background:#ffffff; color:#000000; margin:10px 0px 0px 0px; } img { border:0px; } p { margin:5px 0px 10px 0px; } form { border:none; margin:0px; padding:0px; } a { cursor:pointer; } a:link { color:#9AB324; text-decoration:none; } a:visited { color:#9AB324; text-decoration:none; } a:hover { color:#9AB324; text-decoration:underline; } a:active { color:#9AB324; text-decoration:none; } .container { margin:0px auto; width:400px; } .success { background:#EEF5CD; border:1px dashed #9AB324; color:#608339; margin-bottom:5px; padding:5px; text-align:left; } .warning { background:#eed4d2; border:1px dashed #a94637; color:#ac241a; margin-bottom:5px; padding:5px; text-align:left; } .attention { background:#fefbcc; border:1px dashed #e6db55; color:#ada019; margin-bottom:5px; padding:5px; text-align:left; } h1 { color:#9AB324; font-size:16px; } form, fieldset { border:none; margin:0px; padding:0px; } input, textarea, select { font:100% arial, sans-serif; vertical-align:middle; } input[type='text'] { background:#ffffff; border:1px solid #c3c3c3; border-left-color:#7c7c7c; border-top-color:#7c7c7c; padding:2px; } input[type='password'] { background:#ffffff; border:1px solid #c3c3c3; border-left-color:#7c7c7c; border-top-color:#7c7c7c; padding:2px; } input[type='radio'] { margin:0px 5px 0px 5px; } input[type='hidden'] { display:none !important; } select { border:1px solid #c3c3c3; border-left-color:#7c7c7c; border-top-color:#7c7c7c; min-width:100px; padding:1px; } select option { padding:0px 5px 0px 5px; } textarea { background:#ffffff; border:1px solid #c3c3c3; border-left-color:#7c7c7c; border-top-color:#7c7c7c; padding:2px; } table.data th { background:#9AB324; border-bottom:1px solid #596E0E; color:#ffffff; font-weight:bold; padding:5px; text-align:center; } table.data td { padding:5px; } table.data td.rowOne { background:#f0f0f0; border-bottom:1px solid #dddddd; } table.data td.rowTwo { background:#f5f5f5; border-bottom:1px solid #dddddd; } table.data td.button { background:#ffffff; border:none; text-align:right; } </style> </head> <body> <div class="container"> <?php // Check if form was submitted if ($_SERVER['REQUEST_METHOD'] == 'POST') { // Cost of items $price = array('coke' => '1.25', 'diet' => '1.50', 'sprite' => '1.75'); // Quantity Available $quantity = array('coke' => '5', 'diet' => '7', 'sprite' => '2'); // Selected Item Information $quantAvail = $quantity[strtolower($_POST['item'])]; $itemPrice = $price[strtolower($_POST['item'])]; // First check Quantity if ($quantAvail < $_POST['quantity']) { echo '<div class="warning">Insufficient quantity available.</div>'; } elseif ($quantAvail >= $_POST['quantity']) { echo '<div class="success">Sufficient quantity available. Checking funds...</div>'; } // If we passed quantity, check funds if (($_POST['quantity'] * $itemPrice) > $_POST['funds']) { echo '<div class="warning">Insufficient funds available.</div>'; } elseif (($_POST['quantity'] * $itemPrice) <= $_POST['funds']) { echo '<div class="success">Sufficient funds available.</div>'; } // Remaining Funds $remainingFunds = $_POST['funds'] - ($_POST['quantity'] * $itemPrice); echo '<div class="attention">You have <strong>$' . $remainingFunds . '</strong> funds remaining</div>'; } ?> <h1>Vending Machine</h1> <p>What would you like to purchase?</p> <form action="" method="post" name="vend"> <table align="center" border="0px" cellpadding="0px" cellspacing="1px" class="data" width="400px"> <tr> <th width="30px"> </th> <th style="text-align:left;">Item</th> <th width="70px">Price</th> </tr> <tr> <td align="center" class="rowOne"><input name="item" type="radio" value="Coke" /></td> <td class="rowOne">Coke</td> <td align="center" class="rowOne">$1.25</td> </tr> <tr> <td align="center" class="rowTwo"><input name="item" type="radio" value="Diet" /></td> <td class="rowTwo">Diet Coke</td> <td align="center" class="rowTwo">$1.50</td> </tr> <tr> <td align="center" class="rowTwo"><input name="item" type="radio" value="Sprite" /></td> <td class="rowTwo">Sprite</td> <td align="center" class="rowTwo">$1.75</td> </tr> <tr> <td align="center" class="rowOne" colspan="3"> Qty: <input type="text" size="2" name="quantity" value="1" /> Available Funds: $<input type="text" value="5.00" name="funds" size="5" /> </td> </tr> <tr><td class="button" colspan="3"><input name="submit" type="submit" value="Vend" /></td></tr> </table> </form> </div> </body> </html>
Wow that is pretty amazing code right there compared to mine. I'm obviously starting out but oneday I want to code as well as you can.
A few of those errors were merely stupid mistakes. I'm using a school laptop that can't run php or test it, so I practically use notepad without testing.
stupid mistakes happen to all of us so dont worry, you will learn alot more as you progress...just stick with it!
-
salathe, are you blowing a kiss at me? lol
-
Thanks you guys, for being awesome!
just doing what I can to help out
-
looks like you are receiving this error because
$id = (int)$_GET['id'];
is not returning anything. Where is the $_GET['id']; coming from?
-
$string = "Hello Friends [Test] My name is John"; $strpos = strpos($string, ']'); $substr = substr($string,0,$strpos);
should be what you are looking for
-
no problem
-
$answer = explode(',', $_POST['answersList']);
$_POST[''] is an array
-
nice...best of luck with it
-
yep that will work
-
if (db_num_rows($queryGetComments) > 0) {
where is db_num_rows() coming from...
-
thats sounds like a good idea...welcome to the forums...im sure we will help you along the way
-
show me your full sql code please
PHP copy
in PHP Coding Help
Posted
okay so what you'll want to do before using copy is use chmod() on the file to allow you permissions