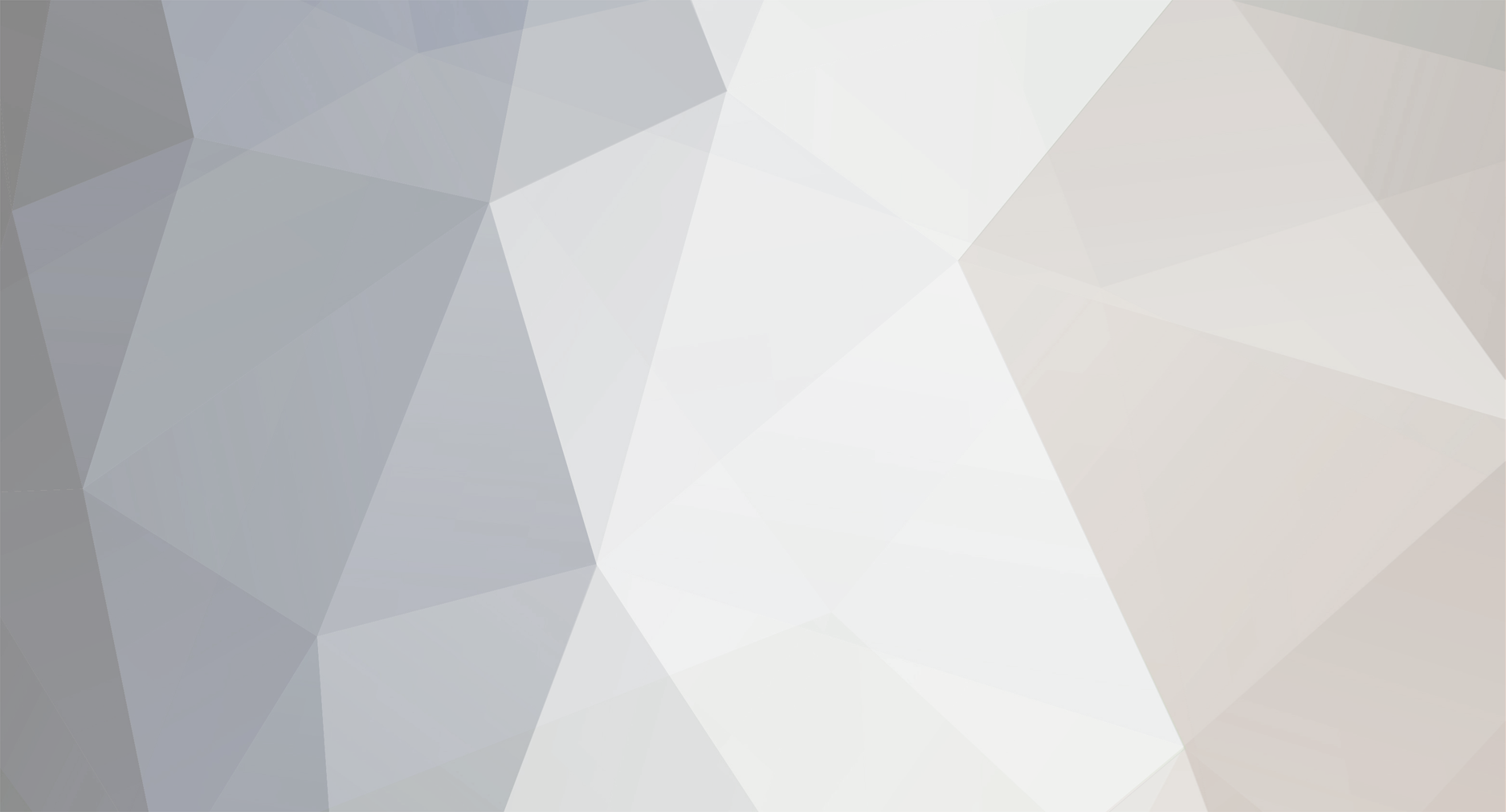
AyKay47
-
Posts
3,281 -
Joined
-
Last visited
-
Days Won
1
Posts posted by AyKay47
-
-
this has to do with the integer limit of PHP. I have ran the expression that you have provided and get an int of 2250700398. Since it is dependant on uniqid, this number is always incresing since it is based off of the current time in milliseconds which then gets converted with hexdec. I'm assuming that your platform is 32 bit otherwise you would not be having this issue. Basically what is happening is the number has reached its maximum integer limit and is being converted into type float, which apparently is a bad thing. I don't know the specifics here so it is hard to tell you exactly what you need to do. Making the subtracted number (52230000000) is on way of handling this, but it is inefficient as it will require you to change the number frequently. My thinking right now is that this is the only solution, as you could subtract an ever increasing number like (time() * 20), this would increase the time span that the number would remain valid, but you will still need to change it.
Further reading: http://us2.php.net/manual/en/language.types.integer.php
There is most likely a better way to do whatever it is that this does.
-
aha! Yep, that did it. Had to do it through .htaccess with: php_flag magic_quotes_gpc Off
as simple as that!
you don't have access to the servers php.ini file?
-
i tried now again and something it's working. if i put the email johnsmith@example.com it's working, but when i put john.smith@example.com it's not working anymore. i don't understand.
i just discovered that even if i use return preg_match("/^[a-zA-Z0-9]+[a-zA-Z0-9_-]+@[a-zA-Z0-9]+[a-zA-Z0-9.-]+[a-zA-Z0-9]+.[a-z]{2,4}$/", $email); the problem it's the same.
:wtf:
thank you!
curious, have you tried the pattern that I gave you?
-
an email regex:
$pattern = "~\b[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]{2,4}\b~i"; $string = "example@example.com"; if(preg_match($pattern,$string,$ms)) { //email is valid print_r($ms); }
Edit: Good catch by Zane as well that you should look into.
-
yes, my mistake on that part.
-
If I am understanding that expression correctly you could simplify that as
preg_match( "~^[\w-/]+$~", $value )
yes, another way of writing it, except that you want to keep the dash immediately following the opening character class bracket so it is not treated as a meta-character. Also, the OP was using eregi(), which performs a case-insensitive search, thus the reason for the appended "i" modifier.
-
Yes, the function has been deprecated as of PHP version 5.3.0
you can use preg_match instead.
if(!preg_match( "~^[-a-zA-Z_0-9/]+$~i", $type )) unset($type); if(!preg_match( "~^[a-zA-Z\-_0-9/]+$~i", $act )) unset($act); if(!preg_match( "~^[a-zA-Z\-_0-9/]+$~i", $id )) unset($id);
-
phppup: you can continue this conversation on the other thread that you started about this subject.
-
Also, in your latest code, every iteration of the loop that contains an even value of $index will echo both sets of <li> tags because $index % 1 == 0 will evaluate to true for all positive integers.
fair point with that, overlooked it.
alot of code for only needing
echo "<li class='{$liclass[(((++$index)%2)+1)]}'>{$item_name}</li>";
but position 0 in the array has to be blank like in my example or this wont work...
this just worked perfect.
thanks so much.
I hope one day I can understand these loop stuff and match stuff very well in php. I was trying to figure out this from the morning.
also thanks for understanding my terrible English.
Thanks so much.
so the code is like this
<?php function GetMenuContent($id){ $sql = mysql_query("SELECT * FROM items WHERE Category = '".$id."' AND Active = '2'"); if(mysql_num_rows($sql)){ while($ROW = mysql_fetch_array($sql)){ $liclass = array('','ac_menu-item', 'menu-item-last'); $data .= t_MenuContent($ROW,$liclass[(((++$r)%2)+1)]); } }else{ $data = "This section is empty!"; } return $data; } ?>
Again, this code will work, but it is not optimized. Hard coding an array inside of a loop is pointless and will decrease performance slightly. I understand that is how t_menuContent is setup. But at least place the array outside of the loop. For the love of God. Also, please use the debugging steps that me and pika have provided, proper debugging is crucial and can save your hours of searching for errors in your code.
-
alot of code for only needing
echo "<li class='{$liclass[(((++$index)%2)+1)]}'>{$item_name}</li>";
but position 0 in the array has to be blank like in my example or this wont work...
true, it is a little more code, my style. The readability of your code is terrible. The classes most certainly do not need to be stored in an array, and more yet should not be included in the loop, being redefined each iteration. For 2 classes, hard coding them is fine. This is why I wrote the code the way I did.
-
Ok my point of confusion is even with the hashing, the task for the hacker is only trying to find a matching or similar original password that generates the same 32 character md5 hash is it not?
Typically, yes, a brute force approach is the best way to match a hashed string. A salt will obscure the data and make it more difficult to match.
I am also aware that I have used a 10 character password which increases the brute force attack time.typically,the longer the string, the longer the brute force attack time.
So no matter how I process the password with hashing, I have to do the same process in my login form processing to compare it to the stored hash or they wont match?with a hash and salt, yes.
Methods of securing passwords will vary from programmer to programmer. However I think it is a pretty widespread ideology that simply md5 hashing a password is a no-no. Even prepending a string onto the password string and then md5ing the string can still be matched by a brute force attack and the algorithm can be traced.
My preferred method is to use crypt. I will not ramble about this function, as there is plenty of documentation on the link I provided. But I use a tweaked version of these functions found on php.net.
function makeSalt() { static $seed = "./ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789"; $algo = '$2a'; $strength = '$08'; $salt = '$'; for ($i = 0; $i < 22; $i++) { $salt .= substr($seed, mt_rand(0, 63), 1); } return $algo . $strength . $salt; } function makeHash($password) { return crypt($password, makeSalt()); } function verifyHash($password, $hash) { return $hash == crypt($password, $hash); } ?> Which then means that this relation will always hold: <?php true === verifyHash($password, makeHash($password));
-
Alright, so a typical loop would look something like this:
$sql = "SELECT * FROM items WHERE Category = '".$id."' AND Active = '2'"; $query = mysql_query($sql) or die($sql . "<br />" . mysql_error()); if(mysql_num_rows($query) != 0) { $index = 1; while($row = mysql_fetch_array($sql)) { $item_name = $row['ItemName']; if($index % 1 == 0) { echo "<li class='classtop'>{$item_name}</li>"; } if($index % 2 == 0) { echo "<li class='classbottom'>{$item_name}</li>"; } $index++; } }
-
2. this line:
if($numrows = mysql_num_rows($sql)){
does nothing, as it will always equate to TRUE. What you should do is check to make sure that at least one row has been grabbed from the query. e.g
Since mysql_num_rows() is being passed a string instead of a query result resource, it will throw a warning and the conditional will evaluate to FALSE. If it's changed to operate on the result resource, the conditional will work as intended, in spite of the fact that the assignment of mysql_num_rows() to a variable isn't necessary.
In the OP's code, $sql contains the result resource. This will also not account for a valid result resource with 0 rows grabbed. Unless I am missing something.
-
I'm having trouble trying to figure out what exactly you are trying to do, your code is pretty broken, I will break it down:
1. I like to separate the SQL and mysql_query call into separate variables, makes for easier debugging.
$sql ="SELECT * FROM items WHERE Category = '".$id."' AND Active = '2'"; $query = mysql_query($sql) or die($sql . "<br />" . mysql_error());
2. this line:
if($numrows = mysql_num_rows($sql)){
does nothing, as it will always equate to TRUE. What you should do is check to make sure that at least one row has been grabbed from the query. e.g
if(mysql_num_rows($query) != 0) { //execute code }
3. this line:
while($ROW = mysql_fetch_array($sql)){
will store both numerical and associative arrays in the variable $ROW, so to grab each individual field value you can either use the name of the field or the numeric order that the field is in the row. e.g
$id = $ROW['id']; //or $id = $ROW[0];
4. this line:
$data .= t_MenuContent($ROW,$liclass);
makes no sense, unless t_MenuContent is a function with a return value. What is the logic for this line?
-
There would be a slight performance decrease simply because the regex is capturing two segments as opposed to one. However I really don't see anything that would cause 1 - 2 seconds of increased page load time. There are a couple of suggestions to the regex you have though:
RewriteEngine on RewriteRule ^([^/]+)/([^/.]+)$ index.php?id=$2&cat=$1
Added a string ending anchor
Removed the preceding backslash to the dot (the dot is not a meta character inside of a character class).
Have you changed anything else before noticing the performance decrease?
Is your .htaccess file located in the root directory?
-
The first regex will match unicode characters, the second will match normal ASCII characters (a,b,c etc..).
-
I have a string that can be
"Billy Bob <Billy.Bob@gmail.com>"
or
"Billy.Bob@gmail.com"
how can I make it so that if it is like the first example, it will just pull the email address?
Does any sanitation need to be done to the string? Will the string always contain a valid email address or is this unreliable data that needs to be checked for a valid email address as well?
-
The logic for removing the negation operator makes no sense, if a user has logged in then why would they need to be directed to the login.php page again. Really, either you require a login or you don't. If you want users who are logged in to have certain privileges over the guests, then that is a different story. With this said, login would be optional, and since you have not posted your login script I do not know what $_SESSION['login'] actually holds. But to give you a raw answer, the logic should be that a user should only be redirected if they attempt to login and fail. Again, using the logic you have stated, logging in is optional. Here is a skeleton of what I am talking about:
session_start(); if(!isset($_SESSION['login'])) //depends on the logic of setting this value { //add whatever error handling you want header("Location: login.php"); } //proceed with normal code
this will not affect guests, who will not have to login to view the page.
-
well first you are telling us that the "campaigns" table consists of the fields: campaign_id, user_id, budget. However, in your insert statement (which uses incorrect syntax btw):
mysql_query ("INSERT INTO campaigns ('','id','campaign','budget','category','advert') VALUES '','$query','$campaign', '$budget', '$category','$advert')");
you have specified different fields then what you initially stated, i don't see a campaign_id field, i don't see a user_id field. Please get your facts straight, help us help you.
Does $_SESSION['id']; contain the user's id? more information is needed to solve this correctly.
-
and i think i need to add something that he knows who the current user id ?
Couple of questions:
Are you using sessions at all to store the users id?
Do you have a auto_increment primary key set up in your database that would be the users id?
-
This first issue would be related to your logout script and the way that you are using cookies.
-
Have you not taken a look at the PHP math functions?
-
For absolute control, hard code the constant to the base URL of the server.
This is the best way, I think. Just make a config file and hard code the URL into it. You could always do a check that if the setting isn't set to try to guess the right URL.
why would you need to make a config file.
Why not?
There should be several other things you would need such as database credentials. Why not put them in a common place?
an include file? It could be convenient but is not needed for a simple hard coded base url.
-
Warning: Cannot modify header information - headers already sent by (output started at /home/local/public_html/members-new/login1.php:1) in /home/local/public_html/members-new/login1.php on line 34
since the output is started on line 1, this makes me think that your text editor is using a BOM (Byte Order Mark). You can google BOM to learn more about them. If this is the case, you will need to change your text editors encoding to something that does not use a BOM. Also for clarification, this is not a session error, it is a header error.
session array issue
in PHP Coding Help
Posted
radio names do not need to be an array since the nature of radio buttons is for only one to be selected.