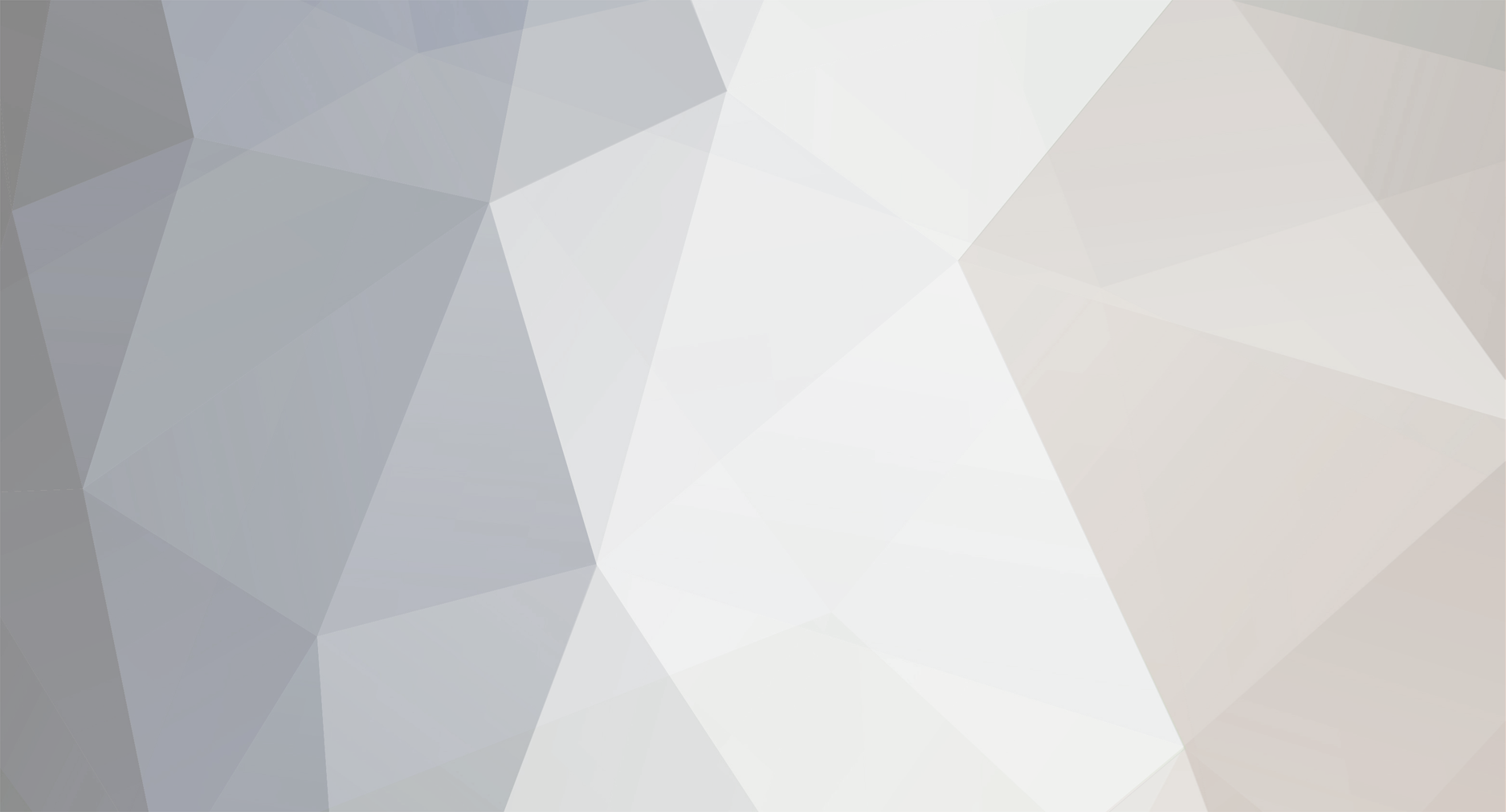
AyKay47
-
Posts
3,281 -
Joined
-
Last visited
-
Days Won
1
Posts posted by AyKay47
-
-
Okay. I'll break this down a bit. Let's start with your code:
<?php $array = mysql_query(); $data = mysql_fetch_row($array); while ($data) { dosomething }
now, by declaring $data = mysql_fetch_row($array) outside of the while loop, you are setting the value of $data to a numerical array of strings that correspond to the fetched row, and this value will be static since it is outside of the while loop. the while loop that you have checks for that value to be true, which it always will be since it is a valid array.
The reason why you specify:
while($data = mysql_fetch_row())
inside of the while loop is because the nature of mysql_fetch_row(). I will quote from the manual here:
Returns a numerical array that corresponds to the fetched row and moves the internal data pointer ahead.
this means that when mysql_fetch_row() is called, the internal pointer is moved forward to the next results set row. So:
while($data = mysql_fetch_row())
will check for mysql_fetch_row() to be true every iteration of the loop. Once it returns false (there are no more rows to return) the loop is stopped.
-
Here is a little comparison, I will refer to the values as HTTP_HOST and SERVER_NAME
1. HTTP_HOST is obtained from the HTTP header request and is completely controlled form the client browser and therefore may not be reliable.
2. SERVER_NAME is defined in the server configuration file (normally httpd.conf), which means the value is more reliable however you must make sure that it is configured correctly and available.
-
So I should be using HTTP_HOST instead of DOCUMENT_ROOT?
I would use the relative paths to the files.
/path/from/server/to/css/template.css
EDIT: since the paths are different for both servers, you will need to use another $_SERVER value as Pika has suggested.
-
So I would have:
$record_id = (isset($_POST['record_id'])) ? $_POST['record_id'] : '';
//check for $record_id emptiness
if(!empty($record_id))
{
"SELECT * FROM mytable WHERE id = $record_id "
}
Is that correct?? Am I missing any brackets or braces??
if this code is on the page where you form action points to, it will hold the select value correctly, but the code your posted itself will not actually do anything. You also forgot to store the SQL string inside of a variable.
-
Read the link in my signature for why w3schools sucks.
And here's a better client side resource: https://developer.mozilla.org/en-US/learn/
I was waiting for your response, heh. I took a look at that link almost a year ago now. I never use the site anymore, I simply happen to stumble upon it as I was googling for error reporting. Unfortunately, as scootstah mentioned, it is almost always ranked 1 for any PHP search. I will have to filter it out of my search results. I use the mozilla dev website more often than not to find anything related to client side that I need.
-
my results using the exact code that you have provided:
$file = '"1" "+447980123456","+447980123456","","2009-06-03","13:15:17","hello world","Orange","843822651" "+447980123456","+447980123456","","2009-06-03","15:17:13","helloworld","Orange","843822652" "+447980123456","+447980123456","","2009-06-03","17:13:15","hello,world","Orange","843822653" '; $toinks = 0; $array = explode(',',$file ); foreach($array as $key => $value) { $value = preg_replace('/#1#/','',$value); $value = preg_replace('/[^+0-9]/','',$value); if ($value != '' || $value != NULL) { if (preg_match('/^\+/',$value)) { $newarray[$toinks] = $value; $toinks++; } } } $wenkz = $newarray; print_r($wenkz);
results:
Array ( [0] => +447980123456 [1] => +447980123456 [2] => +447980123456 )
-
First, I would specify a query that would produce all of the ID numbers to display to the user and have it populate a drop down list.
<form action='' method='post'> <select name='record_id'> <?php $sql = "select ID from table"; $query = mysql_query($sql) or die($sql . "<br />" . mysql_error()); if(mysql_num_rows($query) > 0) { while($row = mysql_fetch_assoc($query)) { $record_id = $row['ID']; echo '<option value="' . $record_id . '">' . $record_id . '</option>'; } } ?> </select> </form>
then, wherever you have the form action pointing to, you would add this code to grab the value of the select tag.
$record_id = (isset($_POST['record_id'])) ? $_POST['record_id'] : ''; //check for $record_id emptiness if(!empty($record_id)) { //execute code block }
-
On the contrary, even though w3schools has misinformation like this, I still use it from time to time as a quick reference. It's pretty much always the top Google result, and usually has what I'm looking for right away. For example, the charts for HTML entities are quite handy.
well, it's a large scale site, so everything on there can't be incorrect, (close though). However it is inconsistencies in a site like this that would make me question it's integrity. While I will say that some of the charts etc. that are on there can be helpful at times. The inconsistencies cause me to never recommend anything on it.
It's pretty much always the top Google resultWhich is why it is blocked from my search results
that's not a bad idea.
-
Found this quote from w3Schools that made me chuckle, since PHP 6.0 isn't in discussion anymore, and E_STRICT became a part of E_ALL in version 5.4.0. Yet another reason why w3schools is terrible.
E_ALL All errors and warnings, except level E_STRICT (E_STRICT will be part of E_ALL as of PHP 6.0)
just thought I'd share that with the bunch.
-
Are you planning on doing this yourself? Or would you be better off hiring someone?
-
the error handler is using the bitwise or ( | ) operator. So $amfphpErrorLevel and $level must be integer representations of error levels. If an exception is not being thrown, then the conditions are not being met. Just from this code snippet I can't really help, there are too many things unanswered here.
-
this is because the code that outputs the errors is above the form, so this is where it will be displayed. If your want to position the error below the corresponding field. Store the error in a variable instead of outputting it right away. Then check for the variable being set where you want to display the error.
if(some error occurs) { $first_name_error = "An error has occurred in your first name."; //return false does nothing in this context } ?> <p>Your first name: <span class="required">*</span></p> <p><input type="text" name="first" size="40" placeholder="Ex: Paul"/></p> <?php if(isset($first_name_error)) echo '<p>' . $first_name_error . '</p>';
There are multiple ways to go about this, this is just an example. For input error handling, I prefer to also have real time error responses using jquery.
-
A function from php.net towards the bottom of the set_error_handler documentation that detects PHP fatal errors:
function shutdown(){ $isError = false; if ($error = error_get_last()){ switch($error['type']){ case E_ERROR: case E_CORE_ERROR: case E_COMPILE_ERROR: case E_USER_ERROR: $isError = true; break; } } if ($isError){ echo "Script execution halted ({$error['message']})"; } else { echo "Script completed"; } }
this checks for several different fatal errors. You can adjust to your needs.
-
To retain user input, you need to echo the value that was POSTed in each field. Example:
<p><input type="text" name="first" size="40" placeholder="Ex: Paul" value="<?php if (isset($_POST['first'])) echo $_POST['first']; ?>/></p>
-
Do not use
<?php echo $_SERVER['PHP_SELF'];?>
in the form action.
for an explanation as to why: here
-
instead of using an extra line of code for str_replace(), simply add 1 to the strpos() output.
$one = "none:0"; function cut($string) { $string = substr($string, strrpos($string, ':') + 1); return $string; } echo $one = cut($one);
-
this will eliminate invalid characters. Again, this will depend on where the urls are coming from.[...] and there are always either (and only) numbers, underscores or letters after the = sign.
There's no sense in slowing down the regex if there will only ever be numbers, letters and underscores.
it won't slow anything down and will provide a tighter pattern. Again I will state, it depends on where the url is coming from. If it is coming from an unknown source of some sort., using the dot to match is not okay. Now I know that the OP said that those are the only values that can be in the results, but I am skeptical. xyph has also stated the sanitation usage of a tighter pattern.
-
are you ordering DESC? by the correct field? its hard to help you without seeing the SQL
-
ten is greater than 3
-
You are using invalid syntax for the INSERT statement. Check the documentation for the correct syntax.
-
Depending on where the data is coming from, I would use this instead.
#/ref=\w*#
this will eliminate invalid characters. Again, this will depend on where the urls are coming from.
-
You should have error reporting set to E_ALL and display_errors on. Looking at your code, $newname doesn't include the full path, just the name. Also, where is mysqlconnect() coming from? Take a look at move_uploaded_photo
-
I hope that isn't your actual db credentials. What exactly do you need help with?
-
U Freakin ROCK!!! Thank you!
Make sure that you donate like you said you would, please.
Define base URLs
in PHP Coding Help
Posted
why would you need to make a config file.