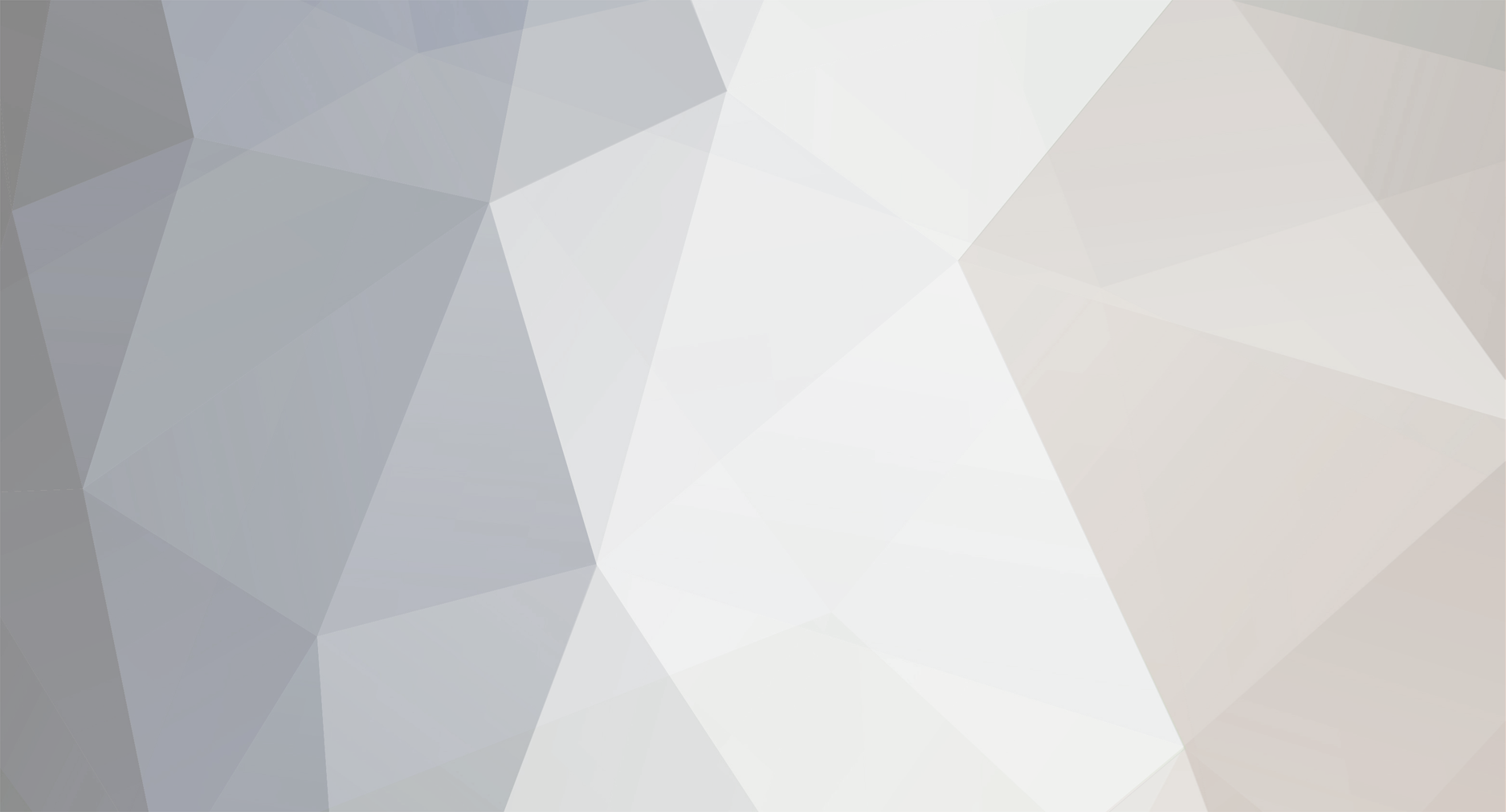
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
Cross-Domain Ajax, Multiple Site Syntax Issues
scootstah replied to LLLLLLL's topic in Javascript Help
The idea is to use PHP (or whatever language) to look at the HTTP origin, and compare it against a list of allowed origins. If you have a match, you send the Access-Control-Allow-Origin header for that request. -
I just showed you how to construct the query, and you did none of it. $data is going to be an array. You can't insert an array into MySQL, you need to make it a string first. You also need to make sure the right values are going to the right columns. What does your table look like?
-
This is the basic idea: $insert = ''; foreach(array(1,2,3,4,5) as $arr) { $insert[] = "($arr)"; } $query = "INSERT INTO table VALUES " . implode(',', $insert); This example produces: INSERT INTO table VALUES (1),(2),(3),(4),(5) So, that would create 5 rows in one query.
-
CSV's are typically read line-by-line. Check the manual for fgetcsv to see how you can loop it. Also, you should be constructing an INSERT query with multiple rows and then executing it once, instead of executing it on each iteration. We're talking an absurdly large number of potential queries. Check the MySQL manual for multi-insert syntax.
-
So what's wrong with the fgetcsv() result?
-
Or better yet, LOAD DATA INFILE.
-
I'd say insert a colon in the middle (07:25), and then use strtotime to get a UNIX timestamp from that. Something like: $time = '0725'; $time = strtotime(implode(':', str_split($time, 2))); EDIT: You only need the strtotime() if you want to use something like date.
-
echo $query and post the result in your next reply. EDIT: You need to use $mysqli->real_escape_string(), not $mysqli->escape_string(). There is a difference.
-
You need to escape after the implode().
-
MySQL would only think it is another column if you ended the single quotes. So, in fact quotes are your problem, not commas. Escape all input to the database with mysql_real_escape_string.
-
Passing Value To Ruby File Using Php System
scootstah replied to bugcoder's topic in PHP Coding Help
You'd do it the same way you would with a regular command line. -
From the manual: So, you can either run two queries or you can count() the result set.
-
You should be dealing with the date in the query, not after the fact.
-
Also, don't echo "so and so is Invalid" in your function. Outputting stuff is going beyond the responsibility of the function. Just return true or false, and then you can use that return value to output something. if (validate($email, 'email')) { echo 'Email is good!'; } else { echo 'Email is invalid!'; }
-
Logical Statement Always Returning False
scootstah replied to Colton.Wagner's topic in PHP Coding Help
Try var_dump'ing each variable ($row['Password'] and $encrypt->password($_POST['Current_Password'])) and see what you get. -
Logical Statement Always Returning False
scootstah replied to Colton.Wagner's topic in PHP Coding Help
Perhaps the first part of the expression is failing. -
Oops, I meant to say strtotime. Sorry about that.
-
Use strtotime to get the UNIX timestamp of both dates, and then do the subtraction, and then use date again.
-
Disabling Php Input Without Disabling Html
scootstah replied to deathadder559's topic in PHP Coding Help
All SQL injection relies on abusing special characters, like quotes. mysql_real_escape_string() escapes those special characters, so SQL injection is not possible. It may still be possible to manipulate the database with user input though, without SQL injection taking place. For example, let's say you have a Users table with a column called "status". Let's say there are three possible values for the "status" column: "enabled", "disabled", and "banned". Let's say you only want normal members to view users by "enabled" or "disabled", and administrators can also view "banned". For argument's sake, let's say the input is in the form of a dropdown box. If you don't validate the input, any user can just change "enabled" or "disabled" to "banned", and view all the banned users. With that said, <?php ?> isn't going to harm your database in the slightest way. -
Disabling Php Input Without Disabling Html
scootstah replied to deathadder559's topic in PHP Coding Help
Unless they are writing the input to a .php file and then including it. -
Disabling Php Input Without Disabling Html
scootstah replied to deathadder559's topic in PHP Coding Help
I would recommend using something like HTML Purifier, which will let you define which HTML tags should be allowed, and it will protect against XSS attacks. -
So, as you can see, the "cid" index does not exist in $_REQUEST.
-
What does the following give you? <?php session_start(); include ("functions.php"); include ("config.php"); $cid = $_REQUEST['cid']; $_SESSION['cid'] = $cid; echo "<pre>Request:\n\n" . print_r($_REQUEST, true) . "</pre>"; echo "<pre>Session:\n\n" . print_r($_SESSION, true) . "</pre>";