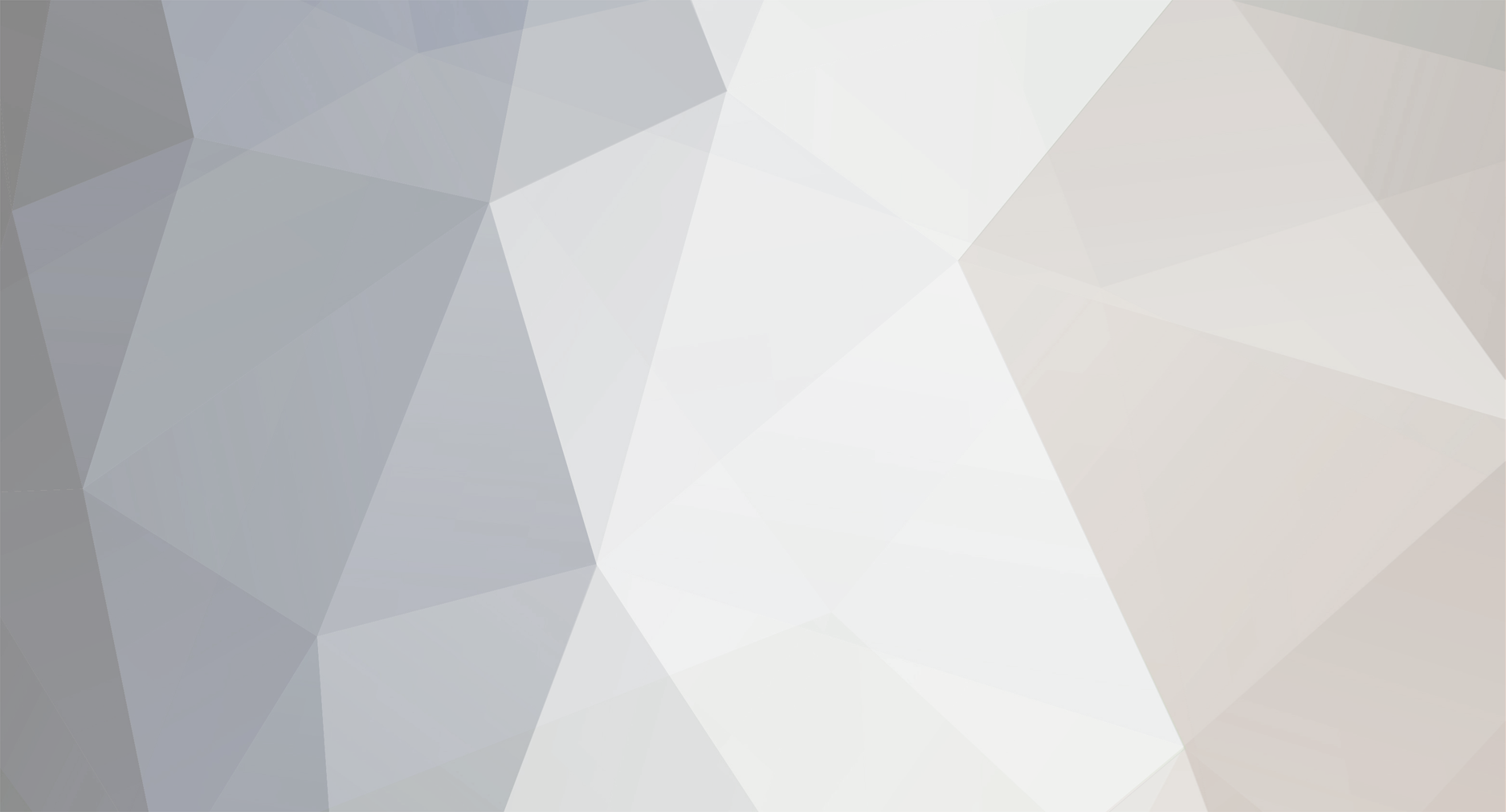
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
loading image during ajax process not working properly
scootstah replied to EHTISHAM's topic in Javascript Help
Works here: http://jsfiddle.net/pqart7tk/ -
http://davidwalsh.name/css-flip Looks to be what you want then.
-
header('Content-Type: image/png');Because you're doing that. If you're not outputting the image then you can remove that line.
-
As per the manual, the second argument for imagepng() is a filename to save the image.
-
Flip in what way?
-
Problem with registration form written in PHP and AJAX
scootstah replied to Smashden's topic in PHP Coding Help
You created an Array for params but it should be an Object. Change var params = new Array();Tovar params = {}; -
Code to check and prevent duplicate record entry in MySQL
scootstah replied to mnwuzor's topic in Other Libraries
You have many issues with this code. 1. You should not be using the mysql_* library. It is deprecated and will soon be removed entirely from PHP. You should be using PDO. 2. You need to be escaping input that is used in queries, otherwise you are open to SQL injection attacks. You should wrap all input that gets used in a query in mysql_real_escape_string(). Or, if you're using PDO you would use a prepared statement. 3. You have some weird thing going on with using extra braces inside conditionals. This is unnecessary and adds clutter to your code. 4. The header() call at the bottom is supposed to be a redirect I assume? You do not have the correct syntax there - a redirect header needs to start with Location: . Also, you should put an exit() after a header redirect to prevent the rest of the script from executing. I've revised your code to make it more readable and fix some of the things I pointed out above. if (isset($_POST['submit'])) { $con = mysql_connect('localhost', 'root', '', 'rmwworg_dbms') or die('Error connecting to MySQL server'); $Camp = mysql_real_escape_string($_POST['Camp']); $Hostel = mysql_real_escape_string($_POST['Hostel']); $Validate = mysql_real_escape_string($_POST['Validate']); $Event_Title = mysql_real_escape_string($_POST['Event_Title']); $Regno = mysql_real_escape_string($_POST['regno']); $Title = mysql_real_escape_string($_POST['Title']); $Surname = mysql_real_escape_string($_POST['surname']); $OtherNames = mysql_real_escape_string($_POST['OtherNames']); $Address = mysql_real_escape_string($_POST['Address']); $City = mysql_real_escape_string($_POST['City']); $Age = mysql_real_escape_string($_POST['Age']); $State = mysql_real_escape_string($_POST['State']); $Country = mysql_real_escape_string($_POST['Country']); $Assembly= mysql_real_escape_string($_POST['Assembly']); $Sex = mysql_real_escape_string($_POST['Sex']); $MaritalStatus = mysql_real_escape_string($_POST['MaritalStatus']); $Phone = mysql_real_escape_string($_POST['Phone']); $Email = mysql_real_escape_string($_POST['Email']); $photo2 = mysql_real_escape_string($_POST['photo2']); $datetime = mysql_real_escape_string($_POST['datetime']); $Year = mysql_real_escape_string($_POST['Year']); $validatedby = mysql_real_escape_string($_POST['validatdby']); $category = mysql_real_escape_string($_POST['category']); $result = mysql_query("SELECT * FROM validatedparticipants WHERE RegNo = '$regno' AND Year = year(now())"); //check for duplicates $num_rows = mysql_num_rows($result); //number of rows where duplicates exist if ($num_rows==0) { //if there are no duplicates...insert $sql="INSERT INTO validatedparticipants (Camp, Hostel, Validate, Event_Title, RegNo, Title, Surname, OtherNames, Address, City, Age, State, Country, Assembly, Sex, MaritalStatus, Phone, Email, photo2, datetime, Year, validatdby, category) VALUES ('$Camp', '$Hostel', '$Validate', '$Event_Title', '$regno', '$Title', '$surname', '$OtherNames', '$Address', '$City', '$Age', '$State', '$Country', '$Assembly', '$Sex', '$MaritalStatus', '$Phone', '$Email', '$photo2', '$datetime','$Year','$validatedby','$category')"; $result1 = mysql_query($con, $sql) or die('Error querying database.'); } else { echo"The Participant added"; } header("Location: valpartcard.php?RegNo=" . $row_rsValidateParticipant['RegNo']); exit; }Note that there is no variable called $row_rsValidateParticipant defined in the code you gave. Either you defined that elsewhere or you're trying to use an undefined variable. So what is not working exactly? You're not getting any results from this query when you think you should be? SELECT * FROM validatedparticipants WHERE RegNo = '$regno' AND Year = year(now())What type is the "Year" column in your database? -
Remove the semicolon in front of error_reporting and set its value to -1. error_reporting = -1 ; Default Value: E_ALL & ~E_NOTICE & ~E_STRICT & ~E_DEPRECATED ; Development Value: E_ALL ; Production Value: E_ALL & ~E_DEPRECATED & ~E_STRICTDo the same with display_errors (except its value will be set to On). So then the call to mail() is failing and returning false. This is possibly due to a server configuration problem. You can attempt to display the last error message and see if it gives any helpful clues. if(!$mailSent) { echo '<pre>' . print_r(error_get_last(), true) . '</pre>'; exit; $errors['mailfail'] = true; }This is just a temporary quick bodge for debugging purposes. After you figure out the problem you can remove those two new lines. If you get any output from that please include it with your next reply.
-
Image upload/convert/resize and add to mysql problem
scootstah replied to cobusbo's topic in PHP Coding Help
And the proper way to do this is to look at the MIME type. The extension of a file (.jpg, .png) is simply part of the file name. It is not required and it does not define what type of file it is. So the proper steps to take would be: - Obtain MIME type of the uploaded file - Make sure the MIME type is allowed - Generate a file name/modify file name and use the extension associated with the MIME type Since you seem to only want to store .jpg, then you would check whether the MIME type is "image/jpeg" or not. If it is, just store as normal. If not, convert it from whatever type it is into .jpg. -
Problem with registration form written in PHP and AJAX
scootstah replied to Smashden's topic in PHP Coding Help
In Chrome Developer Tools you simply have to open the Network tab and then click your button. The Network tab has to be opened before you click the button, otherwise nothing will show up. If you get a request to show up you can then click it and inspect the request body to see what kind of data was sent. -
Hint: Variables must start with a $ Well, in a lot of places you're only handling the "true" part of the conditional. If it is "false", your script will just silently do nothing. Edit your php.ini and: - set "error_reporting" to "-1" - set "display_errors" to "On" Make sure to restart Apache afterwards.
-
That site looks super sketchy, to be honest. I suggest you use a proper service such as Twilio.
-
Problem with registration form written in PHP and AJAX
scootstah replied to Smashden's topic in PHP Coding Help
What response? You said there was no request being made. Sorry, I'm not familiar with Prototype. But it sounds like your PHP script isn't receiving the expected values in $_POST. -
Because that is not its job. A class shouldn't do anything until a method is called. Putting business logic in a constructor breaks the Single Responsibility Principle, makes testing harder, and could cause problems with subclasses. Indeed, but you also said this, which is what a router does:
-
Sounds like you're trying to make a routing system. Your "task" should be a controller class, and your upload/update/delete should be methods within that controller. A basic example might be: <?php class DoSomethingController { public function upload() { // upload image } public function update() { // upload image and replace an existing one } public function delete() { // delete an image } }You'd then have some routing logic to determine which controller to execute based on the "task" parameter.
-
That is a very bad approach. You should be using actual, structured classes and not arbitrary arrays with anonymous functions. Also, for the record, constructors should only be concerned with setting up the class... and not actually doing anything.
-
Problem with registration form written in PHP and AJAX
scootstah replied to Smashden's topic in PHP Coding Help
Okay so something is failing before the request gets sent out. The easiest way to figure out where is to use breakpoints and step through the lines in the function. Here's a video that shows how to use chrome debugger to do that. -
Image upload/convert/resize and add to mysql problem
scootstah replied to cobusbo's topic in PHP Coding Help
You really should be working with MIME type, instead of doing it how you are. You can use finfo to do that. Something like this. Untested, but should work $finfo = new finfo(FILEINFO_MIME_TYPE); $mimetype = $finfo->file($fileTmpLoc); $allowedMimeTypes = array( 'image/jpeg', 'image/png', 'image/gif', ); if (in_array($mimetype, $allowedMimeTypes)) { // file is good } else { // invalid mime type } -
Problem with registration form written in PHP and AJAX
scootstah replied to Smashden's topic in PHP Coding Help
Is there a network request now? -
Problem with registration form written in PHP and AJAX
scootstah replied to Smashden's topic in PHP Coding Help
Okay. Have you verified if your onSubmit() handler is being called? You can either use a breakpoint or just put a simple alert at the very top of it to confirm. If your handler is working, then the easiest way is to use the debugging tools to step through it and find where it's breaking. Are you getting any JS errors? Have you looked in the network pane of the debugger to see if a new network request is being made after you click submit? -
You have a bunch of conditionals that could fail, but you're not handling that failure. My guess is that $mailSent is false. You also have a syntax error here: if(!mailSent) { $errors['mailfail'] = true; }Make sure you are developing with all errors turned on to prevent things like that.
-
Image upload/convert/resize and add to mysql problem
scootstah replied to cobusbo's topic in PHP Coding Help
Because your $imageTypeArray contains JPEG but you're later looking for jpg with: } else if (!preg_match("/.(gif|jpg|png)$/i", $fileName) ) { -
Problem with registration form written in PHP and AJAX
scootstah replied to Smashden's topic in PHP Coding Help
You have a disabled button - so it cannot register click events. Typically you would use an onsubmit event on the form, and then use an event.preventDefault() to prevent the default the default submit action.