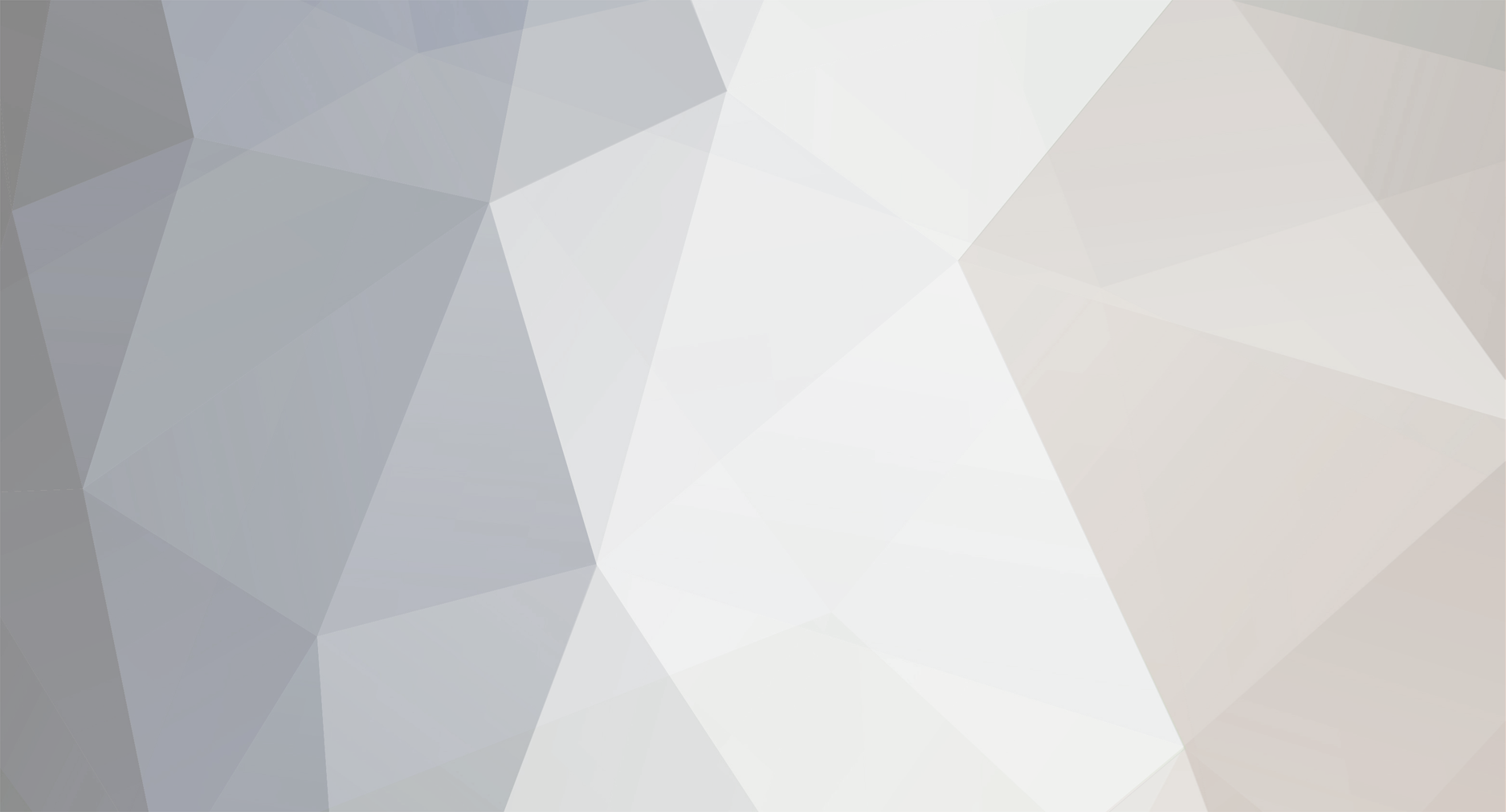
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
So someone is typing out 120+ user's info and then inputting it? Are they importing it from a CSV or XML or something? What are you doing with it after they input it?
-
It's an alternative to using brackets, common in templates and such. In my opinion it looks a lot cleaner and easier to follow when you are opening/closing PHP a lot. // this looks clean <?php if($something === true): ?> <some html> <?php endif; ?> // this looks ugly <?php if($something === true) { ?> <some html> <?php } ?> When you have a few nested brackets (like a conditional inside a loop inside a conditional), all the random closed brackets look awkward and hard to follow. When you have explicit "endif", "endforeach", "endwhile" it's easier to see what goes to what.
-
Are you getting this information from a database? If so you can sort them right in the query with the ORDER BY statement.
-
You need quotes around the query.
-
storing and displaying images with php/mysql
scootstah replied to davids_media's topic in PHP Coding Help
You can store it as base64 encoded and then display it that way. You'd probably want a TEXT type though. It's a better idea to just store the path like aykay said. -
please help: Fatal error: Class 'Engine_IP' :(
scootstah replied to ray_nl's topic in Third Party Scripts
You're going to need to post code. The error is because you're trying to use a class that is not defined. Does your application use an autoloader? -
http://blog.orite.com.au/web_development/2009-01-22/setting-up-wildcard-virtual-hosts-for-web-development-environment/ See if this helps.
-
It's been a while since I've used Smarty but I think this might work... {$cate.$i}
-
I assume you are using CodeIgniter? By default form_error() places the error inside [tt<p></p>[/tt] tags. You can change these tags though, and define a CSS class. <?php echo form_error('passconf', '<p class="error"'); You can use the third parameter to close a tag other than <p>. <?php echo form_error('passconf', '<div class="error">', '</div>'); Or, you can set the error delimiters globally. $this->form_validation->set_error_delimiters('<div class="error"', '</div>'); Check the docs for more information.
-
Do you mean it allows you to enter a username even if one exists? I'm not sure what you are using for a database layer, but I don't think $query would directly contain the number of rows. I would expect $query to be an object. So with the little code I have to work with I would say you need to do two things. 1) You need to check the actual number of rows returned and 2) you need to check if the rows returned are > (greater than) 0, not 1. As it stands if you have a single username already in the database it will allow another entry. Why? Because 1 is not greater than 1.
-
You can try http://www.phpcompiler.org/
-
please could you comment on my comments OOP beginner!
scootstah replied to wright67uk's topic in PHP Coding Help
No, it won't. He created a new instance of the class, so he has two different objects. -
I'm not sure about the first but the second one appears to attempt to inject a potentially malicious image into the page. The "image" could potentially execute code to do various bad things.
-
PHP and .NET are two different technologies. You can't use one to build the other.
-
Restricting an ip adress to not vote twice
scootstah replied to razorsese's topic in Application Design
Indeed, and making PHP accept session ID's from only cookies is one of them. Sure, but the circumstances are different. Allowing session ID's in the URL can be manipulated in a number of ways. For example if the site doesn't use SSL then every out-going link will potentially contain the session ID which can be found in the receiving sites HTTP referrer. It is much more difficult for a third party to read a user's cookie from another website, modern browsers prevent that. You'd need to explicitly send the cookie data from the original site to a third party site - like through an XSS attack, which is a lot easier to manage. Passing session ID's through the URL creates more problems than it solves. In fact, it doesn't even solve this problem - the client can still reset the session data. -
The is_unique() method expects that the standard database library is loaded. Do you have it loaded? If you don't want to do that you'll need to extend the form_validation class and write your own is_unique method using doctrine.
-
I'm not sure I totally understand your question, but I think you want to select rows from a database, loop through them, and return modified values. Is that right? Currently you are returning the result inside the foreach loop on every iteration. Therefore you are ignoring any modifications and just returning the original result set. Instead you'll need to build a new array of results, add the modified values on each iteration, and then return that afterwards. On an unrelated note, you don't need to run input through the xss_clean method from the security class. You can simply use the second parameter on input's post method to run xss_clean. // this... $age = $this->security->xss_clean($this->input->post('select')); $place = $this->security->xss_clean($this->input->post('select2')); // ...is the same as this... $age = $this->input->post('select', true); $place = $this->input->post('select2', true);
-
Restricting an ip adress to not vote twice
scootstah replied to razorsese's topic in Application Design
The session id is, by default, stored in a cookie. Not the data. With a cookie, the time remaining is stored on the user's browser. The user can modify the time remaining to, ex., 0 seconds left. With a session, the time remaining is stored on the web server. The time can not be modified by the user, and thus is more secure. Once again if you delete the session cookie the session will be destroyed and all data in the session will become unset. Therefore if you set a session flag like $_SESSION['already_voted'] = true; and then you delete the session cookie, this data is unset and the session array will be empty. You cut out the part of my post that breaks this logic. Because that's a bad idea that can lead to session hijacking, so it's not a valid alternative. The only way to semi-reliably do what the OP wants is to have user accounts. You could use logic to make it so user's could only vote once, which means you would need multiple accounts to vote multiple times. Still possible, but more work. Other than that, it cannot be done reliably without punishing innocent users. You either block too many users, or don't block enough users. -
Restricting an ip adress to not vote twice
scootstah replied to razorsese's topic in Application Design
The session id is, by default, stored in a cookie. Not the data. With a cookie, the time remaining is stored on the user's browser. The user can modify the time remaining to, ex., 0 seconds left. With a session, the time remaining is stored on the web server. The time can not be modified by the user, and thus is more secure. Once again if you delete the session cookie the session will be destroyed and all data in the session will become unset. Therefore if you set a session flag like $_SESSION['already_voted'] = true; and then you delete the session cookie, this data is unset and the session array will be empty. -
Restricting an ip adress to not vote twice
scootstah replied to razorsese's topic in Application Design
The PHP session is stored as a cookie. If you delete the cookie, you destroy the session. -
Restricting an ip adress to not vote twice
scootstah replied to razorsese's topic in Application Design
The problem is that this sort of thing can't be fully prevented without penalizing innocent users. Making decisions based on a users IP is always not a very good solution, since there is no guarantee that a users IP is unique to that user, or that it will stay the same. Some user's IP changes frequently, other user's share the same IP (like people in a college or in office buildings). So if you simply store the user's IP and not let anyone else with that IP vote again, you're potentially disallowing anyone on the same network from voting. Yes, I will agree with you. But, storing data only through cookies is quite situational. Sometimes cookies just aren't secure enough. But restricting purely based on IP is sloppy and overly restrictive. I haven't looked a lot into the ability to save PHP sessions to a file/database, but perhaps that may work best in this scenario. A cookie isn't secure because it is data stored on the client's browser. A PHP session is a server-sided per-user key/value storage, though. Normally, they can't be trusted over a prolonged period because they don't last all that long - just long enough for the current session. But, PHP's session_set_save_handler may be able to remedy this, allowing the backend to store sessions upon deletion, instead of just permanently deleting them. But, as I said before, I am not all that experienced with the practice of saving/storing sessions, although it seems possible without too much hassle. So, if anyone has a better grasp of saving sessions, please don't hesitate to comment. Sessions use cookies, so if you think cookies are not enough then neither are sessions. -
I'm confused. You said the game was for jQuery, but ActionScript is Flash. Is the game written in Javascript or Actionscript? Either way, they wouldn't be able to encrypt their own values because they wouldn't have the encryption key.
-
Restricting an ip adress to not vote twice
scootstah replied to razorsese's topic in Application Design
The problem is that this sort of thing can't be fully prevented without penalizing innocent users. Making decisions based on a users IP is always not a very good solution, since there is no guarantee that a users IP is unique to that user, or that it will stay the same. Some user's IP changes frequently, other user's share the same IP (like people in a college or in office buildings). So if you simply store the user's IP and not let anyone else with that IP vote again, you're potentially disallowing anyone on the same network from voting. -
You could probably encrypt the POST data, and obfuscate the Javascript. It's still beatable but it's better than plaintext.
-
That is JSON. You'll need to decode it, which will turn it into an object. $json = json_decode($output); $api_url = "http://localhost/?access_token=" . $json->access_token;