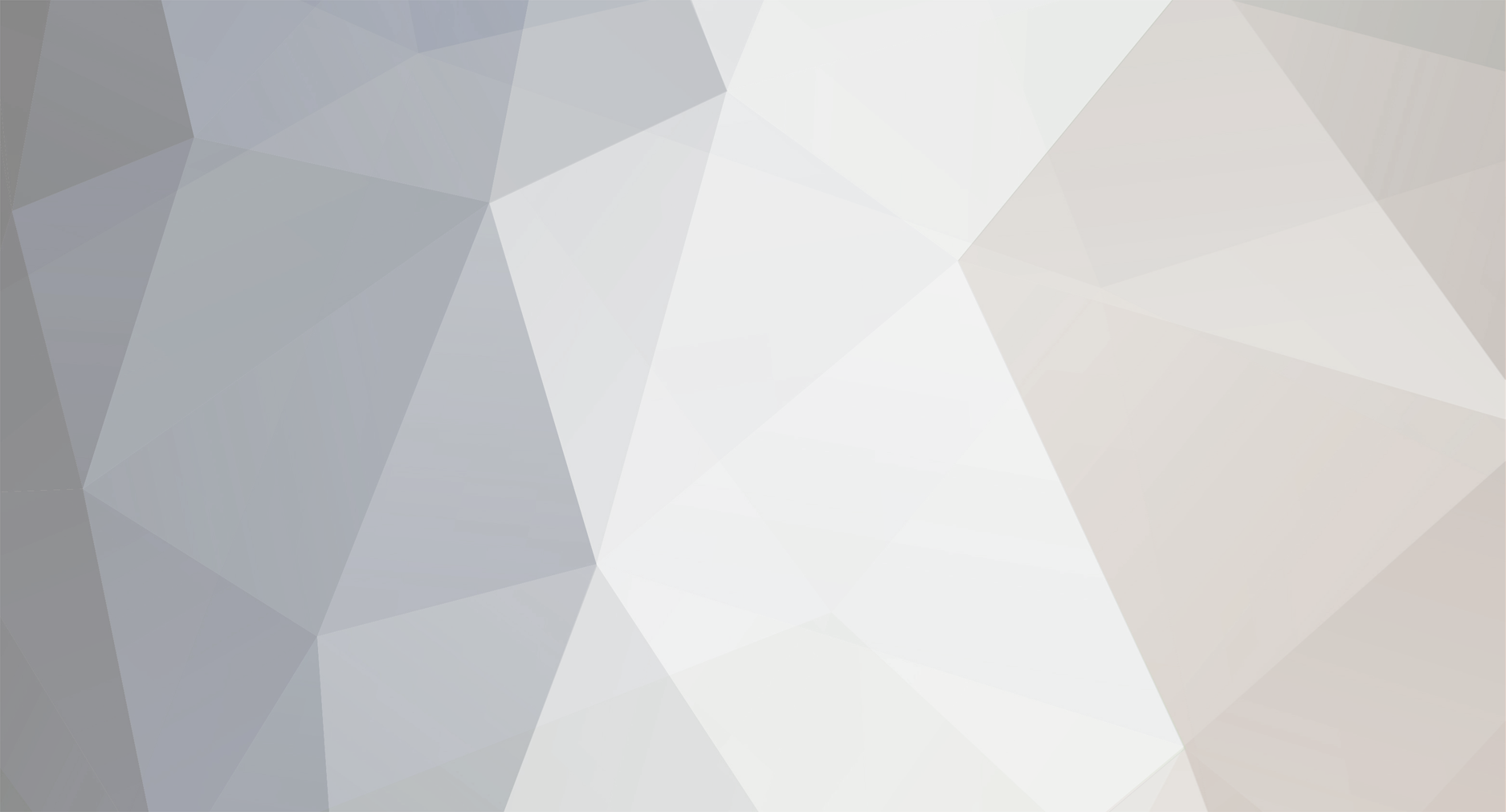
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
Convert form inputs for date into a mysql date format
scootstah replied to MTWKfal's topic in PHP Coding Help
Sure. If you go the second route you'll just have to add the right formatting, which can be found here. -
Basically, you'll need to use a little logic to end rows and create new ones. One way to do that is to increment a counter and then just test the counter's value. My code is a little different than yours, just for simplicity but it's the same concept. // create an array to loop through $array = range(1,20); // set number of columns per row $cols = 5; // start our counter $i = 0; echo '<table>'; foreach($array as $arr) { // check the modulus of our counter // if it is 0 we have to start a new row if ($i % $cols == 0) { if ($i > 0) { // end the current row if it isnt the first row echo '</tr>'; } echo '<tr>'; } echo '<td>' . $arr . '</td>'; // increment the counter // this is important, dont forget this $i++; } echo '</tr>'; echo '</table>';
-
Convert form inputs for date into a mysql date format
scootstah replied to MTWKfal's topic in PHP Coding Help
You can either convert it to a UNIX timestamp with PHP and then convert it into MySQL's format with MySQL, or just convert it into MySQL format all in one go. $year = $_POST['year']; $month = $_POST['month']; $day = $_POST['day']; $timestamp = strtotime($year.'-'.$month.'-'.$day); $sql = "INSERT INTO table (date) VALUES (FROM_UNIXTIME($timestamp))"; $year = $_POST['year']; $month = $_POST['month']; $day = $_POST['day']; $sql = "INSERT INTO table (date) VALUES (STR_TO_DATE('$year-$month-$day', '%Y-%m-%d'))"; -
To or not to display Messages on updates, confirmation, etc...
scootstah replied to rudyten's topic in Application Design
I usually use an unobtrusive fixed-positioned bar at the top of the page that's maybe 50 pixels tall or something for my success messages. I usually run a bit of Javascript to make it disappear after a few seconds, as well as a close button. After say an admin uses the site enough times, adds new content, etc, they're not going to want to see some big annoying success message in the way. They're not going to want to close a bunch of popups every time they make a small adjustment either. The way I do it makes it up out of the way and it disappears by itself. As far as errors, it really depends what kind of error we are talking about. Form validation? Those go back to the form by their respective inputs. If it is some kind of error that should never happen I'll probably log it and throw a HTTP 500 response. An example would be trying to use data that should never be missing, but is - this would probably be a result of someone messing with hidden form data or something, something that just wouldn't happen naturally. -
That's the problem, they haven't updated it since then.
-
Sometimes I pluralize, sometimes I don't. It depends on the context. For example, if we are talking about an associative array for a single user I will make the name singular; probably $user. If it is a multidimensional array containing many users, I will likely pluralize it, so it would be $users. Honestly, it really makes no difference. It's just personal preference.
-
Suggest projects for beginner php programmer?
scootstah replied to christomax's topic in Miscellaneous
What have you learned so far? What specifically are you in the process of learning or improving on? I would suggest a blog CMS. You will learn most of what you will use as a PHP developer. There's a lot of room to expand to more advanced topics, like: caching, user authentication, file handling, dynamic URL's, AJAX, etc. You can apply a lot of what PHP offers to a CMS. -
Netbeans is okay. Personally I like Eclipse + PDT.
-
I assume they are logging by IP, since two different browsers don't increase the view. Yep, log by IP or with a cookie.
-
Making a text adventure in PHP-very basic/beginner
scootstah replied to DeadlyAzn's topic in PHP Coding Help
Yes. Read the manual. http://us3.php.net/manual/en/language.types.array.php -
w3schools is an amazing site to learn bad and outdated code. It's terrible, to be honest. It's mildly useful as a quick reference and nothing more.
-
Yeah, I agree with thorpe. I'd rather have a torrent program that excelled at working with torrents, instead of one that was meh at working with torrents and meh at streaming media. It's not a very big deal to have two programs working in unison for this. As long as the download directory is visible to your network, I don't see a reason that any media streamer couldn't automatically pick up new files.
-
PHP/Html Table class, is this a good design?
scootstah replied to Hall of Famer's topic in Application Design
You shouldn't be overwriting the classes vars when you call buildtable() or getheader(). As an end user, this would be aggravating. Every time I call one of those methods I'd have to reset any attributes I have explicitly set. -
If you have all your classes in one file, why do you even need an autoloader? Just include the one file and all the classes will be loaded. You're going against pretty much every OOP standard, though. Split them up.
-
Maybe something like... $num = 1234; if (!is_float($num)) { $num = (float) ($num . '.00'); } There's probably a better way, but it works.
-
There's no sure-fire way to tell if a request is unique or not. You can either check by IP or set a cookie or something. Both are not very reliable, but it's the best you can do aside from a login system.
-
Making a text adventure in PHP-very basic/beginner
scootstah replied to DeadlyAzn's topic in PHP Coding Help
Sorry to be blunt but if you do not know how to create a database, you do not have the ability to do this. -
I believe they said it's a security risk to let your users know if the username is wrong. Personally, I usually just go with an ambiguous "user/password combination is invalid" approach. It just makes it that much harder to brute force if you don't even know if the username is valid.
-
Making a text adventure in PHP-very basic/beginner
scootstah replied to DeadlyAzn's topic in PHP Coding Help
It sounds like you are trying to run a marathon before you can crawl. I think you need to take a step back, start learning the fundamentals of PHP, and then come back when you are ready. -
Damn, you guys must be boring IRL.
-
PHP/Html Table class, is this a good design?
scootstah replied to Hall of Famer's topic in Application Design
Instead of explicitly stating which attributes can be placed, just allow everything. It's easier and you won't run the chance of missing something. -
Making a text adventure in PHP-very basic/beginner
scootstah replied to DeadlyAzn's topic in PHP Coding Help
-
That doesn't make sense. You have to specifically look for the break. If you only indent the code in the case but not the break, you know that as soon as the indentation stops there is a break. It is a lot faster to just look at a column of indented text than to find a specific keyword.
-
My guess is they are selecting something, then looping through it and selecting something else for each result.