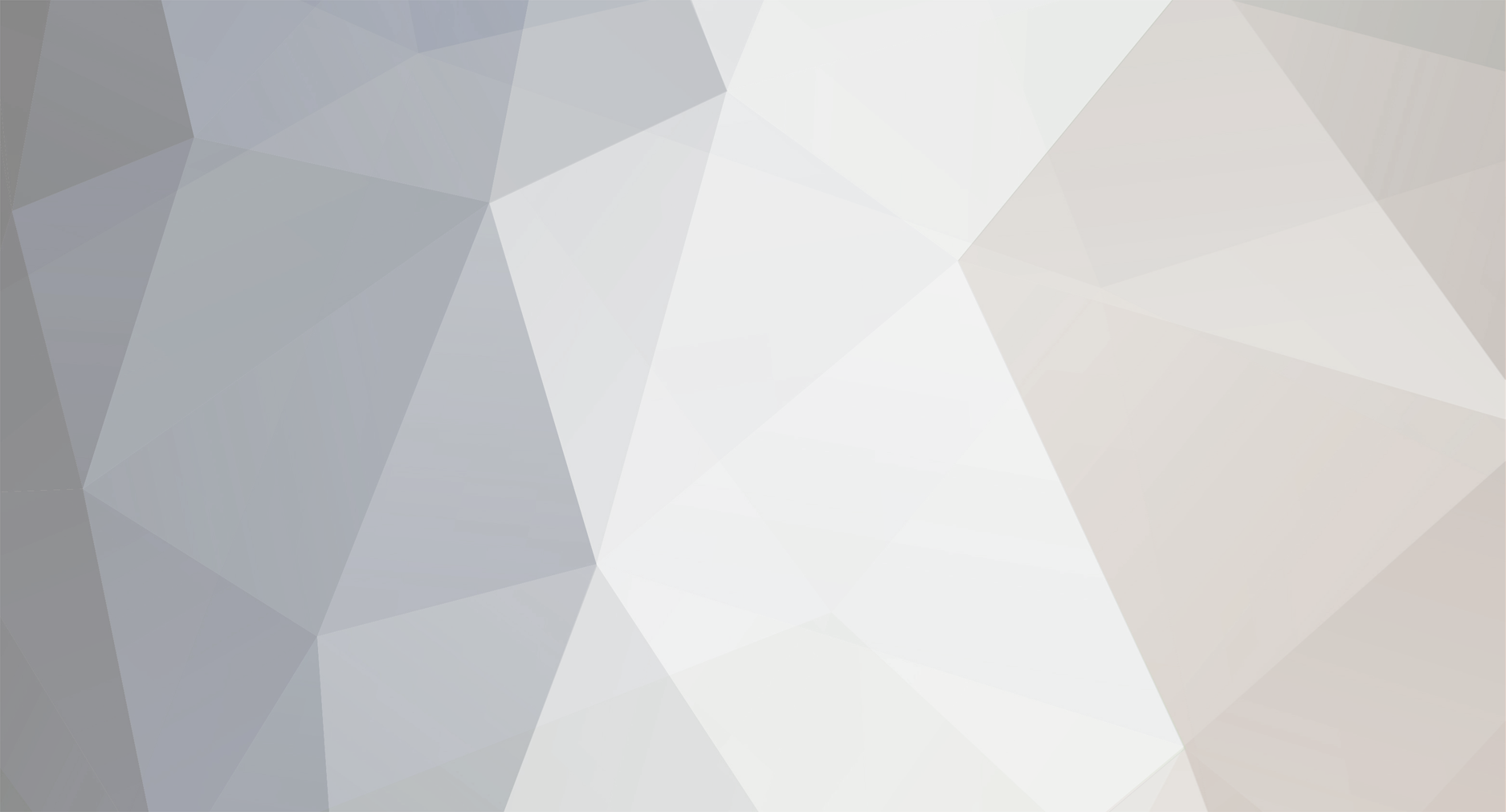
ober
-
Posts
5,327 -
Joined
-
Last visited
Posts posted by ober
-
-
Correct, but relative to your webserver... not the c drive.
-
You're going to want to use preg_replace(). If you don't know how to use regular expressions, check out this site: [a href=\"http://www.regular-expressions.info/\" target=\"_blank\"]http://www.regular-expressions.info/[/a]
-
I think that's mostly correct, but you have to specify a path to the file.
-
[b]DO NOT PM MEMBERS ABOUT YOUR THREADS WHEN YOU JUST POSTED THEM.[/b]
-
If you're running PHP 5, you can test it with the following code:
[code]<?php
$time_start = microtime(true);
// your function and calls go here
$time_end = microtime(true);
$time = $time_end - $time_start;
echo "Ran Analysis in $time seconds<br/><br/>";
?>
[/code]
There is a slight different way to do it if you're running PHP4. Use the following function instead of microtime():
[code]<?php
function microtime_float()
{
list($usec, $sec) = explode(" ", microtime());
return ((float)$usec + (float)$sec);
}
?>[/code]
Either way, I think the first one is going to be WAY faster.
-
The first error is always because the session_start() is after something is sent to the browser. Anything, white space or the wrong placement of the PHP tag can throw it off. I don't know that an include of the session_start() would do it, but it's possible.
Try placing the code without the include. -
Put your other variables into hidden inputs. As far as the javascript call:
[code]<a href="index.php" onclick="document.name_of_form.submit()">Edit</a>[/code] -
One way to do it would be to copy the image to a temporary folder when someone requests it. Once the user loads the image into cache (presumably at the end of the page load), you fire off an AJAX call that removes the image from the temp folder. They don't see you do it, and your images are safe. The only problem is that they've cached the image at this point, and anyone with half a brain can rob the file out of the browser cache.
So really, the only way to protect images is to watermark them. -
Did you run it? Did it provide any errors? Give us a little more information!!!!!!! We're not mind readers!
-
Why not??
-
How is that any different than a Meta refresh? They're the same to the user!
More importantly... what if the user has JS disabled? -
If you go to a full edit, you should be able to edit the title of the thread.
-
Ok... a few things:
1) when using a foreach, it doesn't put the elements into an array.
2) You can push an array onto another array with array_push, but it will be stored as an array within an array. -
No, you cannot stop a user from refreshing the page through the browser. And AFAIK, closing the actual browser window does not fire the onUnload() function. Leaving the page will, however, but that doesn't fix your problem.
-
You could run something like this:
[code]<?php
$query = "SELECT field1, COUNT(field1) AS Cnt
FROM tablename
GROUP BY field1
HAVING (COUNT(field1) > 1) ";
?>[/code]
That assumes you can use the HAVING clause in your DBMS. -
Well, first of all, your code can be reduced:
[code]<? php
$year = $_POST['year'];
echo "$year";
$month = $_POST['month'];
echo "$month";
$day = $_POST['day'];
echo "$day";
?>[/code]
Can be:
[code]<? php
extract($_POST);
echo "$year<br/>";
echo "$month<br/>";
echo "$day<br/>";
?>[/code]
Secondly, are you absolutely sure that's what the output is? The way you echoed your variables, they would have ended up right beside each other. With the breaks in there, it should print out each on it's own line. Also, if you're just echoing the variable with no other HTML, you don't need the double quotes around it.
-
kplang,
Until you can describe your problem better or produce a picture of a schematic that describes an algorithm or something you're trying to write, I have to assume that you're talking about the look and layout, not the coding of a project, especially since you said you already have the PHP code done.
Moved to HTML.
If you post it in PHP Help again, I will delete the thread without warning. -
<?php
echo "test";
?>
-
$_GET is a global array that grabs information out of the URL. If someone goes to your site or clicks on a link from your site with the following address: "mysite.com/index.php?page=123" then you're going to find "123" in the variable: $_GET['page'].
So in your index page, you need to handle that:
[code]<?php
if(isset($_REQUEST['page']))
{
switch($_GET['page'])
{
case "123":
include("page123.php");
break;
case "foo":
include("foobar.php");
break;
}
else
include("main.php");
?>[/code]
Does that help? Also, please do not bump your thread 12 minutes after posting a question. Someone will get to it. -
AJAX is pretty easy to use once you get used to it. The coding is pretty simple, it's the concept that you have to wrap your head around and what your limitations are.
-
AJAX uses Javascript to make backend calls to a server side script and return the results to update the page without refreshing the page. It allows you to update content on the page without reloading all the surrounding information.
If you want some help and more tutorials, check out phpfreaks.com's sister site: [a href=\"http://www.ajaxfreaks.com\" target=\"_blank\"]http://www.ajaxfreaks.com[/a] -
Ash is right, but you should be able to query your database on both the username and the ID. If you want more info about the AJAX solution, I suggest you head over to ajaxfreaks.com.
-
That means that you should either put something in your script to detect when it goes past date x and calculate the date accordingly. Either that or you'll have to update the script everytime the time shifts.
OR... you could take the date off of your site completely and let your users get the date and time from their own computers!
OR you could post the time and specify that it's based off of GMT. -
wildteen, I'm going to assume that "british summer time" is different from DST. The clocks don't change here for another week or so. This all may be dependent on where the server is physically located.
image upload
in PHP Coding Help
Posted