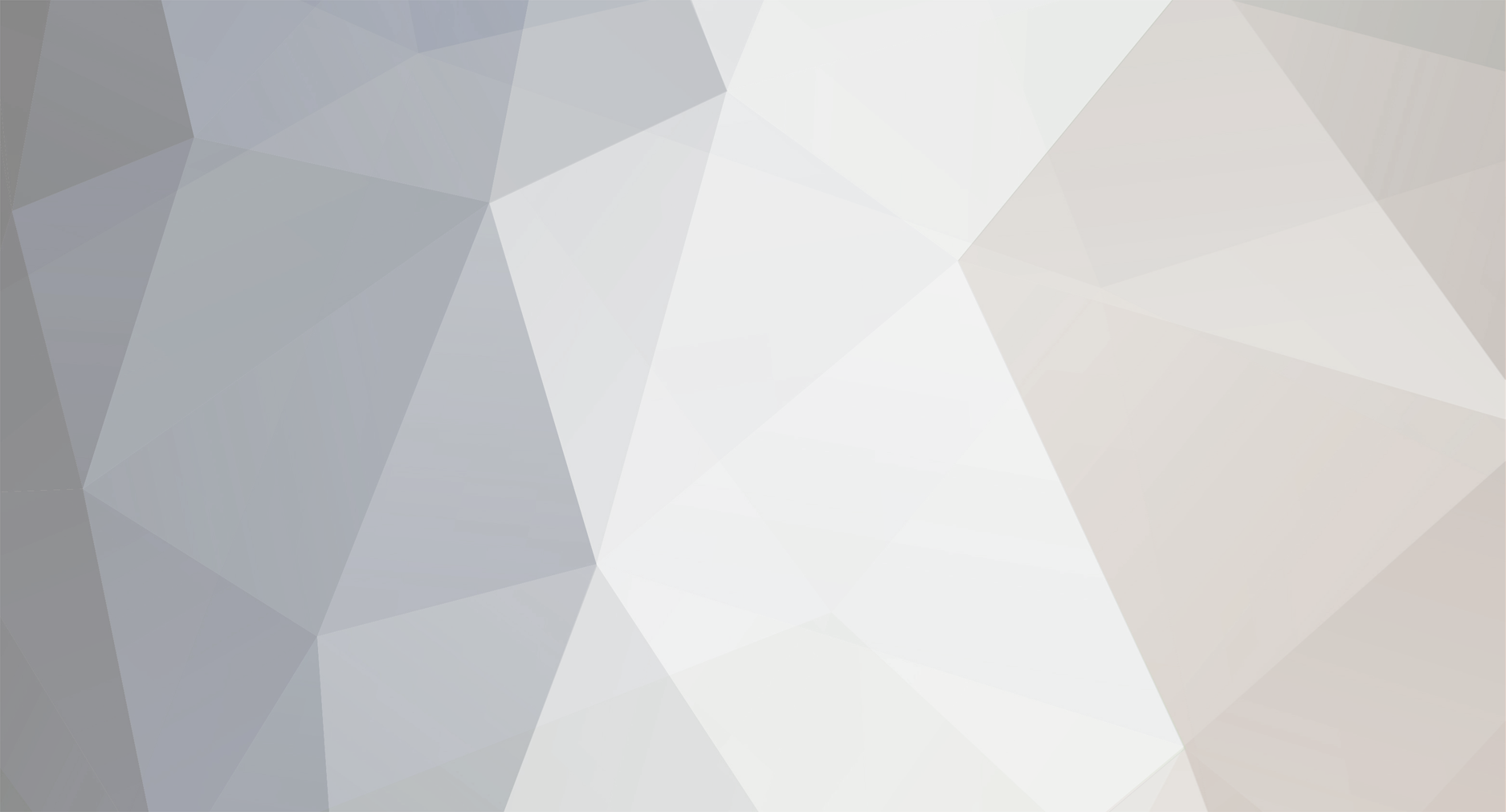
ober
-
Posts
5,327 -
Joined
-
Last visited
Posts posted by ober
-
-
You're going to have to use the mktime() function to add an hour. However, I suggest you figure out something in terms of server configuration otherwise you'll have problems when the time changes back.
-
You'll have to use something else to get that info. PHP is a SERVER SIDE scripting language and doesn't have access to those parameters. You MIGHT be able to grab the country via headers or IP translation, but PHP alone cannot get you all that information.
-
Chances are that you aren't going to solve this problem without using the GD library.
-
Well, it's to avoid holidays and to give people ample time to realize their mistakes. You can set something and not realize it was wrong and not have anyone report it to you for several days. I personally set my cushions at 3 weeks, which pretty much catches 99% of any major screwups. I have had cases where people came to me 3 months after a change was made and I didn't have the backups, but luckily our IT staff was able to go to tape-backups and retrieve it.
Hell, as far as code backups, I have stuff from almost 3 years ago. You never know when an old trick is going to work or you're going to need an example of how you did something.
Backups are a personal decision IMO, but you can't disagree that the more the better! It's a CYA (Cover Your Ass) policy that I try to follow.
EDIT: OH, and you're welcome for the comments... you're always very thorough and I hope I gave you the same experience. -
Well, I'll explain how I manage my stuff. All my connections are setup in my header file that I include with all my files. The connection is opened at the top of the page and then I have a class that I use to run the actual queries. I never close the connection, it just drops off automatically. So I never really write a "open connection" call in my pages. It's always just there. You obviously can't avoid calling queries within your pages, so you have to do that stuff.
-
Anytime.
-
Technically, you don't even have to close the mysql connection, but your second method is definately the better of the two. There is no problem with leaving the connection open for the entire page. Subsequent query calls are just going to reuse the existing connection.
-
[code]<?php
if (isset($_POST['view']))
{
// view!
}
elseif(isset($_POST['edit]))
{
// edit!
}
elseif(isset($_POST['new']))
{
// new!
}
else
{
// show data
}
?>
[/code] -
Can you show us your code? Specifically how you use the includes? And is the code that you hardcode with the same code as in the files you include?
-
I don't really notice many changes.... still looks simple and uncontained... if that's a word.
-
Awesome work, and the re-ordering definately works like a charm now!
I'd say that's one of the best admin pages I've seen in a while. Clean, efficient, and very easy to use!
And I think the rule of thumb for backups is at least 2 weeks.
Oh, I did have one more complaint... I don't see the point in the "Admin Home", unless you're going to add more content to it. If all it is going to say is "use the nav on the left to make your edits"... then I think you can drop it. -
Welcome to the forums!
Your "completion" message is always going to show up because you run it no matter what happens with the results.
You need to do this:
[code]<?php
$result = @mysql _query($query);
if($result)
echo '<strong>Information updated for $name_first $name_last<br><a href="./add_talent.php">Return</a> to add new Talent</strong>'
else
echo "There was a problem with your update: " . mysql_error();
?>[/code]
As a side note, you can reduce the following:
[code]<?php
$ child_id=$_POST["child_id"];
$name_first=$_POST["name_first"];
$name_last=$_POST["name_last"];
$age=$_POST["age"];
$singer=$_POST["singer"];
$dancer=$_POST["dancer"];
$actor=$_POST["actor"];
$model=$_POST["model"];
$gender=$_POST["gender"];
$height=$_POST["height"];
$weight=$_POST["weight"];
$eye_colour=$_POST["eye_colour"];
$hair_colour=$_POST["hair_colour"];
$hair_length=$_POST["hair_length"];
?>[/code]
to this:
[code]<?php
extract($_POST);
?>[/code]
It does the same thing. -
Keep in mind that some people are forced to do this if they don't have access to the web server configuration files.
-
Right, but you guys are missing the point. Javascript cannot detect when a user closes the browser window. You're going to have to use shocker's method of checking update times.
-
Your method sounds fine, but your plotting can probably be simplified: [a href=\"http://www.advsofteng.com/\" target=\"_blank\"]http://www.advsofteng.com/[/a]
-
Well... it's kind of hard for us to tell. I don't see where you're setting $url, but then again I don't know anything about the function you're calling either. The rest looks good enough. Have you tried to run it?
-
Welcome to the forums!
I think you're taking the long way to solve your problem. You don't need to use arrays so extensively. That is why you have a database and you should really learn the power of the database instead of using multi-dimensional array manipulation.
When you run your query, it should be setup like this:
[code]<?php
$query = "SELECT * FROM table";
$result = mysql_query($query) or die(mysql_error());
// the following tells you how many records were returned by the query
$numrows = mysql_num_rows($result);
echo "There are $numrows records in my database!<br/>";
// the following creates an array of a single record and consecutive calls
// using a while loop will fill the array with the next record
while($row = mysql_fetch_array($result))
{
echo $row['column_name1'];
echo $row['column_name2'];
// or alternatively
extract($row);
echo $column_name1;
echo $column_name2;
}
?>[/code]
You can also refine your query to look for specific items using WHERE clauses.
I hope this helps. -
This is not a PHP question, and you cannot post links to your own computer. If you want to ask a layout question, ask it in the HTML or CSS board. Thread closed.
-
Welcome to the forums!
Cookies are stored on the user's pc. Sessions are saved on the server. -
You need a if/else:
[code]<?php
if(isset($_POST['view']))
{
// do whatever
}
else
{
// show data
}
?>[/code]
You can add more to that if you want certain things to happen if something else in the post is set. Just add an "else if(isset(whatever)). -
www.ajaxfreaks.com
That's a sister site of this one and probably the better place to ask it. -
Then why isn't something in the query?!?!?
-
To all concerned: this is a Javascript question. I have moved it here because of that. I have also renamed the thread because that's a ridiculous title and provides no meaning. joecooper, you're being warned.
-
First of all, using an ampersand in a fieldname is NOT a good idea. Talk about asking for trouble.
Secondly, if Product_Cat_ID is an auto_increment field, you can leave it out entirely. It'll fill itself in. If it's not an auto_increment field and you've specified that it cannot be empty/null, it's not going to accept it.
Help!
in PHP Coding Help
Posted
1) in the onchange event of the select box, you call a javascript function that recalls the page with the ID of the user selected and runs the PHP to fill the boxes.
2) You use AJAX to do the dirty work.
Both require Javascript. If you want to avoid Javascript, you'll have to use 2 different forms. One with just the select box and one to deal with the info in the other fields once they get filled in.