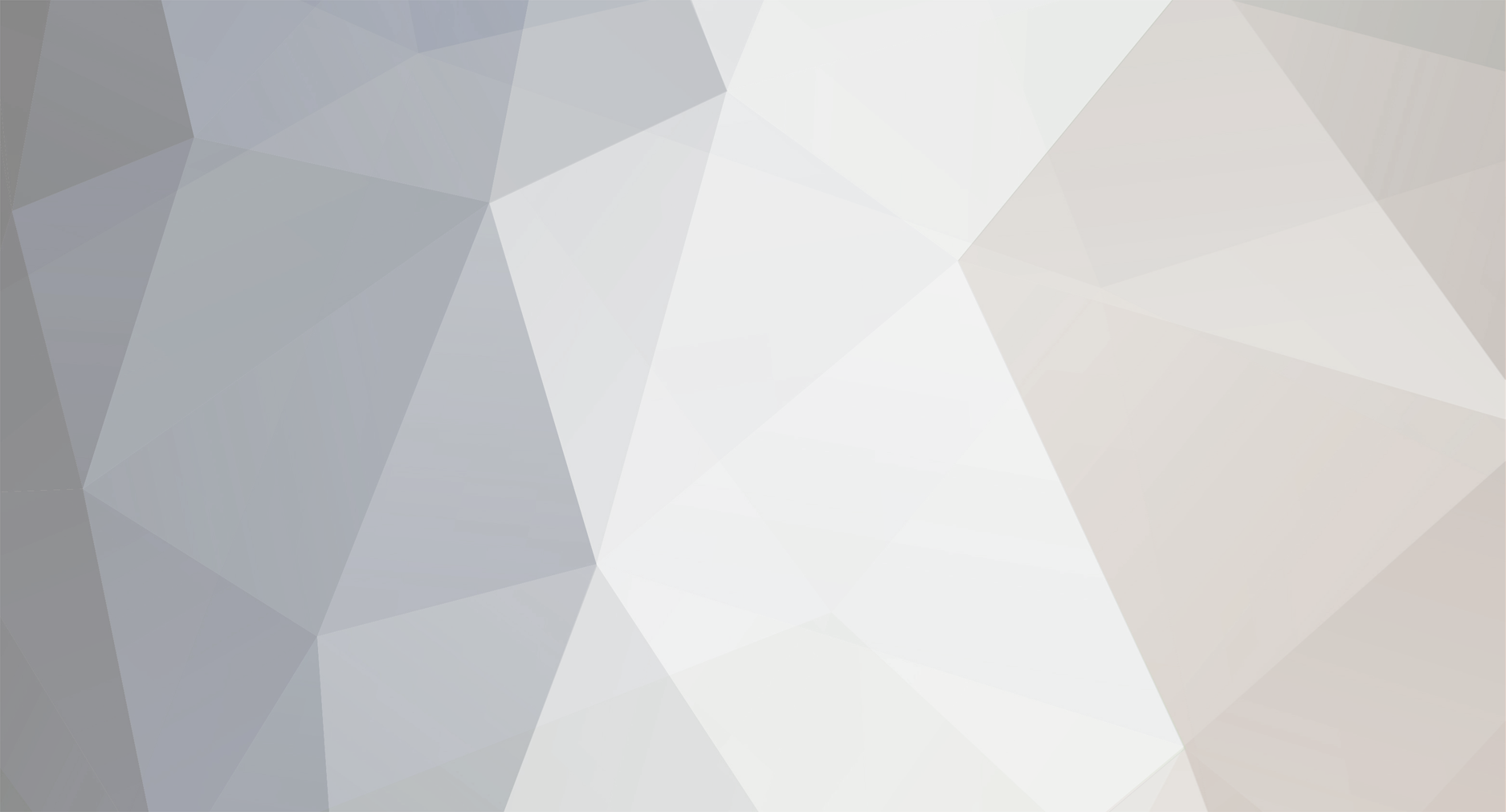
Christian F.
Staff Alumni-
Posts
3,072 -
Joined
-
Last visited
-
Days Won
18
Everything posted by Christian F.
-
Hmm... Wouldn't something like this work? function FindPerms ($Settings) { $Perms = 0; foreach ($Settings as $Options => $Number) { $Perms += pow ($Number, $Options); } return $Perms; }
-
If you take a look at line 10 in your code of the first code block, you should be able to spot it right away.
-
Replacing&extracting an item in a string
Christian F. replied to Shadowing's topic in PHP Coding Help
No, those two RegExps are not the same. One is looking for something that's preceded by one or more spaces, while the other is looking for everything that's not preceded by a character1 that is not a white space2. The one I posted includes support for sentences that start with @name, while the other does not. This means the sentence "@Christian is testing" would not get matched. Your second expression, however, is quite correct. 1The lack of any character satisfies this requirement, even if it can sound a bit convoluted. 2Yeah, I know. Double negatives suck in natural languages, but for RegExp they work quite nicely. As long as you can wrap your head around them... -
Ah, I misunderstood your requirement about "one hyphen only" a bit. Or, rather. I took it a bit too literally. In any case, the fix is simple. Just change the last question mark in the RegExp to a star. That'll change it from matching "0 or 1" to "0 or many"
-
Feedback/review on DBA class wanted.
Christian F. replied to Christian F.'s topic in Beta Test Your Stuff!
First of all, thanks for taking the time. Then to your remarks: [*]I've done this to ensure that there is no way there can be a naming collision, regardless of how the class is included. Also, it'll prevent collisions with other classes as well. Something I've picked up from Qt. Call it "Defensive Programming", if you like. [*]I'll look into this. Primary reason I've done as I've done is to be able to use the constants like the flags for "preg_match_all ()" and other built-in functions. [*]You're right, and I'll fix this. Thanks. [*]As noted in my first post, I'm not a too big a fan of throwing exceptions. Especially for what can be considered "normal" error conditions. I do, however, agree that relying upon a custom error function does increase the effort needed in order to import this. The first time, at least. Currently the error function used here is a part of my framework, and ties nicely into my template engine. Which works quite nicely, for me at least. That said, I'm not quite 100% satisfied with the current solution, and I'll take your comments into consideration. Will have a look at the Adapter pattern as well, see if it fits my intentions. Though, even if it does I won't be removing that first line. -
Replacing&extracting an item in a string
Christian F. replied to Shadowing's topic in PHP Coding Help
That RegExp is looking for a single tilde (~), followed by a single backslash (which doesn't have any effect, btw) and then one or more tildes. With the following tildes being stored in a sub group. Not quite what you're looking for, in other words. If you formalize (like I did above) the pattern rule you want to match, then you should find it a lot easier to translate it into RegExp. The "u" modifier is to make the RegExp UTF-8 compatible. You can read more about the different modifiers in the PHP manual. -
Tip: Look at the name of the function you're using. Should be quite informative when compared to the error message.
-
passing looped variables to a javascript when page created
Christian F. replied to turpentyne's topic in PHP Coding Help
Hehe, fun to know that there's always something new and exiting to learn though. Don't you agree? You'll see that you'll be just as good, if not better, once you've gotten 12 years of PHP development under your belt too. -
Replacing&extracting an item in a string
Christian F. replied to Shadowing's topic in PHP Coding Help
Check the PHP manual for "preg_replace ()" Though, that said you should be really careful with it. Otherwise you can end up with giving your users full access to run their own PHP code inside your system. -
Do all of the PHP parsing at the very top of the page, and then use variables to store the output. Doing it this way will ensure that you don't get the dreaded "headers already sent" error message, and it will make it a lot easier to write code. Once you've done that, it's just a matter of using following: header ('Location: new_file.php'); die (); Remember the "die ()" after a redirect, otherwise PHP will continue parsing the script and potentially create problems.
-
passing looped variables to a javascript when page created
Christian F. replied to turpentyne's topic in PHP Coding Help
The best thing to do is to do all of the PHP parsing, before you send out anything to the browser. Do that, and you'll see that your problem is suddenly a very, very simple one. BTW: I've fixed up your PHP part a bit, should give you some clues on how to write more maintainable code. <?php // CF: Using sprintf () and templates makes things a whole lot easier to read. $ExpandTemplate = <<<OutHTML <div id="%1\$s" style="width:350px;padding-top:20px; class="select-toggler" onclick="return showHide('%1\$s-expander');\"><img style="position:relative;top:-2px;" src="images/structural/red-plus.gif" />%1\$s</div> OutHTML; $ExpandImageTemplate = <<<OutHTML <div id="%1\$s-expander" style="float:left;padding-right:25px;display:none;" width="90">%2\$s <br><img src='%3\$s'></div> OutHTML; // and here's the loop I'm talking about. $Output = ''; while ($row = mysql_fetch_assoc ($result)) { if ($category != $row['component_category']) { $category = $row['component_category']; if (!$firstime) { $Output .= '</div><br><br>'; } else { $firstime = true; } //CF: Changed output to be stored in a temp variable, as well as adding output escaping to prevent XSS etc. $Output .= sprintf ($ExpandTemplate, htmlspecialchars ($row['comp_cat_name'])); // CF: Add the name of the JS variable to a comma-seperated list. $JSVariables = "var_" . htmlspecialchars ($row['comp_cat_name']). ", "; } //CF: Changed output to be stored in a temp variable, as well as adding output escaping to prevent XSS etc. $Output .= sprintf ($ExpandImageTemplate, htmlspecialchars ($row['comp_cat_name']), htmlspecialchars ($row['component_name']), htmlspecialchars (rawurlencode ($row['image_filepath']))); } ?> The rest, however, I'll leave for you to figure out yourself. Best way to learn, now that you've gotten a tip on what you need to do. Added: Of course, using jQuery makes things quite a bit simpler as well. Especially if both methods are combined. No need to reinvent the wheel, after all. Unless it's for learning purposes. -
Replacing&extracting an item in a string
Christian F. replied to Shadowing's topic in PHP Coding Help
Last question first, because I'm weird like that. Not quite, the $1 refers to the first matching sub group. In other words, the first pair of "clean" parentheses. In this case it's the character immediately preceding the @. Namely the beginning of the string or a whitespace character. Index "0" doesn't exists for replacement variables within RegExps. The rest of the string is unmatched by the RegExp, and as such is not affected by it. Variable replacement in RegExp can be quite useful, indeed. As for learning RegExp, I recommend RegExp.info. I consider it courteous, and a matter of professional pride, to write a site so that it'll be completely functionally working even without JS. You're welcome, glad I could help. PS: Fixed my name. -
passing looped variables to a javascript when page created
Christian F. replied to turpentyne's topic in PHP Coding Help
If I understand you correctly, you want the result of this line: $var{$row['comp_cat_name']} = "var_".$row['comp_cat_name']; To be printed out in the JS, where the following bit of code is? If not, where do you get "$variable1" from? var divs=[<?php echo "'".$variable1."'," and so on.... ?>]; Also, what exactly did you want to do with the first line of code? The "$var{}" bit doesn't make much sense, tbh. I suspect you might be a bit confused about the syntax here, possible mixing in the JS syntax..? If so, remember that PHP and the JS is completely separate. For all that PHP cares (which it doesn't in regards to the JS function you posted) the JS is just pure text. Like everything else that's not PHP. After all, all of the PHP code is processed by the server, before the result is sent to the browser. Which, in turn, is responsible to parse all of the text as HTML, CSS, JS or whatever else it happens to be. -
The following RegExp should do wonders, if I've understood your request correctly. $RegExp = '/^\\d+(?:-\\d+)?\\z/'; Since you've validating a phone number, you have to remember to bind the RegExp to the start (^) and end (\z) of the string. Otherwise I'll match as long as there's just a single digit in there somewhere. Regardless of how the rest looks like. To make sure you only get digits, after validating, just run "$number = str_replace ('-', '', $number[0])";
-
Seeing as you have this wonderful section here, I was hoping that I could get some knowledgeable people to help me look over a DBA layer class I've been working on? I'm looking for feedback on all aspects of it, and all constructive criticism would be highly appreciated. Whether it be negative or positive. So please don't be shy about commenting, as long as you can explain your position. In case it's not obvious it's meant as a fully interchangeable class for interfacing against a multitude of DBMS, without having to change anything other than the name of the file included. It doesn't, obviously, support all of the features. Nor will it ever do, but it should support the most used ones. If I've missed some, please let me know as well as why you think it's a necessary inclusion. You can find the class here: http://pastebin.com/tJYNiBKE And some examples on how it can be used: http://pastebin.com/aygrZJTa It exists primarily because of 4 reasons, in order: [*]I wanted to learn more about DBA, and what it takes to write a good class for it. [*]Other classes I've looked at didn't give me what I really wanted, either due to lack of features, too high usage complexity, not being DBA but DAA classes and so forth. [*]I had an old attempt, that didn't work as an abstraction layer for anything but the most simple of queries, and wanted to improve it. [*]And a little bit of "because I could." I also got asked "why not PDO, as it gives you the same". First and foremost it's because PDO isn't a DBA (database abstraction) class, but a DAA (data-access abstraction) class. They even mention it in the intro for PDO. So, it does not give me the same. There are a few other things that I don't like about PDO as well, such as the throwing of exceptions for everything (I blame MSStrings for my hate of this), the connection string and a few other caveats. Arguably most of these caveats I have are personal preference, I have to admit. Still, that doesn't change the fact that PDO isn't a DBA layer. PS: If anyone wants to use it for their own projects, the license is stated in the PHPdoc header for the class. Should anyone, for any reason, want to use it in a commercial project please let me know. I'm sure we can come to an agreement. PPS: I posted this on TheDailyWTF forum as well, though the response was rather lacklustre unfortunately.
-
Replacing&extracting an item in a string
Christian F. replied to Shadowing's topic in PHP Coding Help
You seem to have taken the replacement string from the right post, but the actual RegExp from the wrong. Here's the correct version: $_POST['message'] = "hey whats up @baal how are you doing. @paul bleh"; // Replace user references with links to profiles. $replace = '$1<a href="profile.php?name=$2" class="profile_name" id="$2">$2</a>'; echo preg_replace ("/((?<!\\S))@(\\S+)/u", $replace, htmlspecialchars ($_POST['message'])); Added "htmlspecialchars ()" for security's sake, to prevent XSS and CSRF. -
If you're thinking about a footer for each section, then just print it out before the header for the next. Just remember to save the previous line at the bottom of the loop first, so you can get the necessary data. Doing it that way means you don't have to detect twice, but can just refer to the previous line's data before starting on a new category. Something like this, in other words: while ($row = fetch_array ()) { if (if($category != $row['component_category']) { // Add a footer unless this is the first row, and thus the first category. if (isset ($prev)) { $output .= $prev['footer']."</p>"; } $category = $row['component_category']; $output .= "<p>{$category}<br>\n"; } $output .= "{$row['component_name']} <img src='{$row['image_filepath']}'><br>\n"; $prev = $row; } Simple and easy.
-
You're welcome.
-
Replacing&extracting an item in a string
Christian F. replied to Shadowing's topic in PHP Coding Help
Yes, you're wrong about that. "preg_replace ()" replaces all occurrences, as I've noted above. If you test the code I've linked you to, you'll see for yourself. -
Easiest way would be to save it as a .txt file, and include it as such: include ("http://www.server.invalid/source.txt"); That's a quick and dirty way of doing it, and perfect if you're not going to run it on the local server. If you want to make sure it's always updated, and can be run on the local server as well, then you need to do something like this: // At the top of "source.php" if (isset ($_GET['show_source']) && $_GET['show_source'] == 1) { die (file_get_contents (__FILE__)); } And then include it like this: include ("http://www.server.invalid/source.php?show_source=1"); However, you should note that if you do it like this then everyone will be able to open it up and read the source code as well. So only do this if you're absolutely certain that: [*]Latency times have no meaning, at all. [*]It is 100% safe to show the source code to the entire world. In the vast majority of times, you'll find that both of these will indeed make it ill adviced.
-
If you know the structure of the array, and that it always the same number of elements in the second dimension, then you're far better off skipping that second loop. Also, unless you need to use the data in another location, you don't need to save it in an interim array either. It's just a waste of time and memory. I'd do something like what Psycho posted above, if I were you. Only saving the completed table to a variable, instead of printing it outright.
-
As it's written now, yes. This is because you're making a HTTP call to the web server, which interprets the PHP code and sends out the resulting HTML. Just like a web browser. You're much better off copying said file to your local server, and including it properly. That way you don't have to wait for the HTTP response, which would increase the include time from nano seconds to milliseconds. Several thousand times slower, in other words.
-
Do you want to execute the PHP code in that file, or just get the resulting HTML? If it's the former: You cannot. At least not without editing the script to print out the actual PHP code, or changing it to a pure text file. In any case this is an extremely bad idea, as you give away complete control to your server. Not to mention the latency increase.. If it's the latter, I recommend a look at cURL.
-
Replacing&extracting an item in a string
Christian F. replied to Shadowing's topic in PHP Coding Help
Shadowing: All you need is the code I posted above, the last two lines to be accurate. Just replace "$string" in the "preg_replace ()" call with $_POST['message'] and you're set. As for the "$1" in the links, they're variables for "preg_replace ()" to insert the results of the capturing sub groups. Where the number is the same index you'd get if you'd use "preg_match_all ()" PS: You wouldn't happen to have all of this code wrapped in a function, would you? function test () { echo test2 (); function test2 () { return 2; } } test (); PHP Fatal error: Call to undefined function test2() in php shell code on line 2 -
Hehe, consider this another one of those useful forum netiquette tips: It's always appreciated when the OP, after figuring out the problem on his own, comes back and tells people what it was and how he solved it. That way, the thread might be useful for someone else as well.