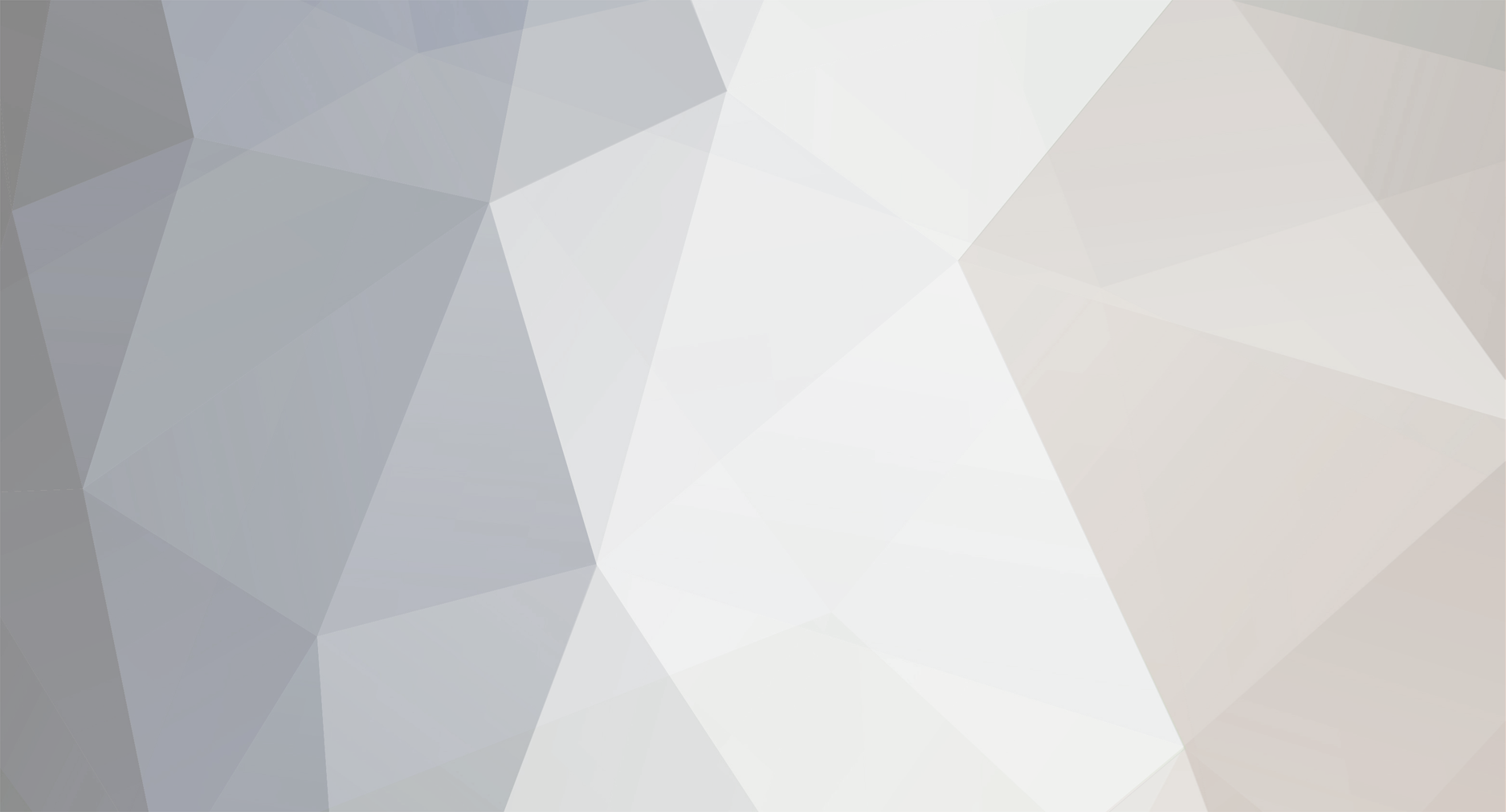
White_Lily
-
Posts
531 -
Joined
-
Last visited
-
Days Won
1
Posts posted by White_Lily
-
-
Hi, I am trying build a system in which users can send messages to eachother.
The problem im having is that the script is having problems inserting the message into the database and i cant figure out why.
Here is the code form that the user fills out:
<?php echo '<div id="pronav">'; echo '<ul>'; echo '<li><p>Nav to go here.</p></li>'; echo '</ul>'; echo '<div class="clear"></div>'; echo '</div>'; echo '<img src="'.$GLOBALS["nav"].'images/vertical-rule.png" />'; echo '<p>'.$u.'</p>'; echo '<form action="" method="POST">'; echo '<table border="0" style="width:auto;">'; if(!empty($senderr)){ echo '<tr>'; echo '<td colspan="2"><div class="error">Error: '.$senderr.'</div></td>'; echo '</tr>'; }if(!empty($sendpass)){ echo '<tr>'; echo '<td colspan="2"><div class="pass">Success: '.$sendpass.'</div></td>'; echo '</tr>'; } echo '<tr>'; echo '<td>To:</td>'; echo '<td><input type="text" name="sendto" id="sendto" class="input" /></td>'; echo '</tr>'; echo '<tr>'; echo '<td align="top">Subject:</td>'; echo '<td><input type="text" name="sendsubject" class="input" /></td>'; echo '</tr>'; echo '<tr>'; echo '<td>Message:</td>'; echo '<td><textarea name="writemsg" id="sendmsg" class="input" style="height:200px;"></textarea></td>'; echo '</tr>'; echo '<tr>'; echo '<td colspan="2"><input style="float:right;" type="submit" name="sendmsg" class="buttons" value="Send" /></td>'; echo '</tr>'; echo '</table>'; echo '</form>'; ?>
Here is the script that inserts the message:
//Send Message $sendmsg = $_POST["sendmsg"]; $to = $_POST["sendto"]; $subject = $_POST["sendsubject"]; $message = $_POST["writemsg"]; if(!empty($sendmsg)){ if(empty($to) || empty($message)){ $senderr = "Please fill out the needed fields in order to send a message. (To: & Message:)"; }else{ $date = date('Y/m/d H:i:s', time()); $putmsg = insert("messages", "id, title, content, to, from, date", "1, '$subject', '$message', '$to', '$u', '$date'"); if($putmsg){ $sendpass = "You have successfully sent your friend a message."; }else{ $senderr = "Failed to send message to your friend. Please try again later.<br><br>".mysql_error(); } } }
Here is the error I am recieving:
Error: Failed to send message to your friend. Please try again later.
You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'to, from, date) VALUES (1, 'Testing Subject', 'Testing message', 'White_Lily', '' at line 1
Any help would be appreciated.
-
Sorry for the code being non-indented(?)
For some reason this forums text-editor removes indenting when you click submit :/
Thanks Psycho I will look at that list of things to do and see what happens
-
Hi I am trying to adjust my registration form because another web developer in the company said that all my empty() checking was making the form checking slow, and to put them into a foreach array with the same message for each failed field check.
I tried to do this however i have come accross a parse error that makes no sense to me.
Here is my code:
<?php if($_POST["submitReg"]){ $validateArray = array("name" => "text", "email" => "email"); foreach($validateArray as $key => $value) { if($value == "text"){ $$key = htmlspecialchars(mysql_real_escape_string($_POST[$key])); }else{ $$key = mysql_real_escape_string($_POST[$key]); } if(empty($$key)) { $msg3 = "Please fill in all fields of this form."; } } $regName = htmlspecialchars(mysql_real_escape_string($_POST["name"])); $regUsername = htmlspecialchars(mysql_real_escape_string($_POST["username"])); $regPassword = htmlspecialchars(mysql_real_escape_string($_POST["password"])); $regREpassword = htmlspecialchars(mysql_real_escape_string($_POST["rePassword"])); $email = mysql_real_escape_string($_POST["email"]); if(preg_match("^[a-zA-Z0-9_.-]+@[a-zA-Z0-9-]+.[a-zA-Z0-9-.]+$", $_POST["email"]) === 0){ $msg3 .= "Please enter a valid email."; }else{ if($regPassword != $regREpassword || $regREpassword != $regPassword){ $msg3 .= "The password fields didn't match."; }else{ $check = select("*", "members", "username = '$regUsername'"); $assoc = mysql_fetch_assoc($check); if($regUsername == $assoc["username"]){ $msg3 .= "That username has been taken, pick another."; }else{ $regPassword = sha1($regPassword); $id = uniqid(); $register = insert("members", "name, email, username, password, user_level, id, ban", "'$regName', '$email', '$regUsername', '$regPassword', 1, '$id', 0"); if($register){ $newsTitle = "New member registered!"; $cont = $regUsername." has just joined Fusion Forums!<br>"; $cont.= "Check out his/her profile:<br><br>"; $cont.= "View Profile"; $newsCont = $cont; $newMem = insert("news", "news_title, news_content, username", "'$newsTitle', '$newsCont', '$regUsername'"); if($newMem){ $to = $email; $subject = "Fusion Forums - Account Confirmation"; $message = "Hello! You have recently registered to Fusion Forum's.<br><br>"; $message.= "This is a confirmation email, below you will find your account details along with a Unique ID.<br><br>"; $message.= "In order to activate your account you must first enter the ID into the text field that follows the link at the end of this email. Your details are as follows:<br><br>"; $message.= "<table>"; $message.= "<tr>"; $message.= "<td><strong>Name:</strong></td>"; $message.= "<td></td>"; $message.= "<td>".$regName."</td>"; $message.= "</tr>"; $message.= "<tr>"; $message.= "<td><strong>Email:</strong></td>"; $message.= "<td></td>"; $message.= "<td>".$email."</td>"; $message.= "</tr>"; $message.= "<tr>"; $message.= "<td><strong>Username:</strong></td>"; $message.= "<td></td>"; $message.= "<td>".$regUsername."</td>"; $message.= "<tr>"; $message.= "<tr>"; $message.= "<td><strong>Unique ID:</strong></td>"; $message.= "<td></td>"; $message.= "<td>".$id."</td>"; $message.= "<tr>"; $message.= "</table><br><br>"; $message.= "Please follow this link in order to activate your account (opens in your default browser):<br>"; $message.= "<a href='http://www.janedealsart.co.uk/activate.php?id=".$id."'>Activate Account</a>"; $from = "noreply@janedealsart.co.uk"; $headers = 'MIME-Version: 1.0' . "\r\n"; $headers .= 'Content-type: text/html; charset=iso-8859-1' . "\r\n"; $headers.= "From: ".$from; mail($to, $subject, $message, $headers); $done = "You have successfully registered to Fusion Fourm's.<br>"; $done.= "We have sent you an email with a confirmation code on it,"; $done.= " when you go to confirm your account you will need this code in order to be able to access the forum's."; $msg2 .= $done; }else{ $msg3 .= "Sorry, we could not register your account details, if this persists contact the webmaster. "; } }else{ $msg3 .= "Sorry, we could not register your account details, if this persists contact the webmaster. "; } } } } if($msg2){ echo '<div class="success">Success: '.$msg2.'</div>'; }else{ if($msg3){ echo '<div class="error">Error: '.$msg3.'</div>'; } echo '<form action="" method="POST">'; echo '<label>Full Name:</label>'; echo '<input type="text" class="field" name="name" />'; echo '<div class="clear"></div>'; echo '<label>Email:</label>'; echo '<input type="text" class="field" name="email" />'; echo '<div class="clear"></div>'; echo '<label>Username:</label>'; echo '<input type="text" class="field" name="username" />'; echo '<div class="clear"></div>'; echo '<label>Password:</label>'; echo '<input type="password" class="field" name="password" />'; echo '<div class="clear"></div>'; echo '<label>Again:</label>'; echo '<input type="password" class="field" name="rePassword" />'; echo '<div class="clear"></div>'; echo '<input type="submit" class="button" name="submitReg" value="Register" />'; echo '<div class="clear"></div>'; echo '</form>'; } ?>
Here is the error:
Parse error: syntax error, unexpected $end in /home/sites/janedealsart.co.uk/public_html/inc/regCheck.php on line 135
I am slightly confused as i did a search for the variable $end and it does not exist within the code...
However this is line 135:
?>
Any ideas as to why this is would be appreciated.
(I'm not posting here to find out how vulnerable this form is to some types of injection, i just want to sort this error out.)
-
if
http://www.example.com/images/file-name.png
doesn't work,
then try:
echo '<img src="'.$_SERVER["DOCUMENT_ROOT"].'/images/file-name.png" />';
-
I will clear my cache and see what happens. doe this mean that i will have to write a seperate query for the stuff i wanted under the while loop?
-
error reporting is always on when im building a site, and it is showing no errors.
The search box is on a different page, and the script that does all the checks for different forms on the site is in a different page to.
-
I don't think there is because a different page uses the same query (but for 2 different tables) and that works fine
-
the assoc line will be used later after the message listing, plus, ive taken that line out because i also thought it would be the problem, but nothing changes.
-
I have a search box inside the "inbox" area of my website, i want it so that when the user types in the username of the person that sent a message to them it should be able to count the number of results, and show them in a table of results.
However the problem im facing is that when you search for a message the result counter returns the correct value (say... 2), while the table only shows 1 result. I can't see or think for the life of me what is wrong with the code below...
<?php include "inc/scriptstart.php"; $title = "Fusion Social | "; $page = "Inbox"; $site = $title.$page; session_start(); $u = $_SESSION["user"]; $dir = $_SESSION["dir"]; if(empty($u) || empty($dir)){ header("Location: index.php"); } $searchquery = $_GET["query"]; include "inc/check.php"; ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "[url="http://www.w3.org/TR/html4/loose.dtd"]http://www.w3.org/TR/html4/loose.dtd[/url]"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1"> <?php include "inc/scripts.php"; ?> <title><?=$site?></title> </head> <body> <div class="header"> <div id="header"> <ul> <li><a href="<?=$GLOBALS["nav"]?>profile.php">Profile</a></li> <li><a href="<?//=$GLOBALS["nav"]?>#">Friends</a></li> <li><a href="<?=$GLOBALS["nav"]?>logout.php">Logout</a></li> </ul> <div id="search"> <?php if($searcherr){ echo '<div class="error">Error: '.$searcherr.'</div>'; } ?> <form action="" method="POST"> <table border="0"> <tr> <td><input type="text" name="define" id="name" class="searchField" /></td> <td><input type="submit" name="search" value="Search" class="buttons" /></td> </tr> </table> </form> </div> <div class="clear"></div> </div> </div> <div class="content"> <div class="main"> <div class="leftcol"> <h2><?=$page?></h2> <img src="<?=$GLOBALS["nav"]?>images/vertical-rule.png" /> <div class="clear"></div> <?php $results = select("*", "users, messages", "users.username = messages.to AND messages.to = '$u' AND messages.from LIKE '%$searchquery%'") or die(mysql_eror()); $numsearch = mysql_num_rows($results); $getsearch = mysql_fetch_assoc($results); echo '<p>Results Found: '.$numsearch.'</p>'; echo '<img src="'.$GLOBALS["nav"].'images/vertical-rule.png" />'; echo '<div class="clear" style="height:30px;"></div>'; echo '<table border="0">'; echo '<th width="1%">Senders Avatar</th>'; echo '<th width="25%">From</th>'; echo '<th width="25%">To</th>'; echo '<th width="25%">Options</th>'; if($numsearch > 0){ while($getfill = mysql_fetch_array($results)){ echo '<tr>'; echo '<td width="1%">'; if(empty($getfill["avatar"])){ echo '<img src="'.$GLOBALS["nav"].'images/avatar.png" />'; }elseif(!empty($getfill["avatar"])){ echo '<img src="'.$getfill["avatar"].'" />'; } echo '</td>'; echo '<td width="25%"><p>'.$getfill["from"].'</p></td>'; echo '<td width="25%"><p>'.$getfill["to"].'</p></td>'; echo '<td width="25%">'; echo '<a href="#">View Message</a>'; echo '<a href="#">Delete Message</a>'; echo '</td>'; echo '</tr>'; } }elseif($numsearch == 0){ echo '<tr>'; echo '<td colspan="4">'; echo "<p>There are currently no messages to view!</p>"; echo '</td>'; echo '</tr>'; } echo '</table>'; ?> </div> <img src="<?=$GLOBALS["nav"]?>images/home-divider.png" /> <!--<div class="rightcol"> <h3></h3> <?php// ?> <img src="<?//=$GLOBALS["nav"]?>images/vertical-rule.png" /> </div>--> <div class="clear"></div> </div> </div> <div class="footer"> <p>Copyright © <?=date("Y")?> Fusion Social</p> </div> </body> </html>
Any Ideas?
-
line 261 is the while loop near the bottom
Tip: copy the code into notepad++ or dreamweaver, then you will find line 261...
-
when using joins you need to use mysql_fetch_array() not mysql_fetch_assoc().
-
Hi, i have an inbox system where by people can create and send messages to different, the user can see the list of messages that they have and they can also search for specific messages from certain users.
i have written this:
<?php $results = select("*", "users, messages", "users.username = messages.to AND messages.to = '$u' AND messages.from LIKE '%$searchquery%'") or die(mysql_error()); $numsearch = mysql_num_rows($results); $getsearch = mysql_fetch_assoc($results); echo '<p>Results Found: '.$numsearch.'</p>'; echo '<img src="'.$GLOBALS["nav"].'images/vertical-rule.png" />'; echo '<div class="clear" style="height:30px;"></div>'; echo '<table border="0">'; echo '<th width="1%">Senders Avatar</th>'; echo '<th width="25%">From</th>'; echo '<th width="25%">To</th>'; echo '<th width="25%">Options</th>'; if($numsearch > 0){ while($getfill = mysql_fetch_array($results)){ echo '<tr>'; echo '<td width="1%">'; if(empty($getfill["avatar"])){ echo '<img src="'.$GLOBALS["nav"].'images/avatar.png" />'; }elseif(!empty($getfill["avatar"])){ echo '<img src="'.$getfill["avatar"].'" />'; } echo '</td>'; echo '<td width="25%"><p>'.$getfill["from"].'</p></td>'; echo '<td width="25%"><p>'.$getfill["to"].'</p></td>'; echo '<td width="25%">'; echo '<a href="#">View Message</a>'; echo '<a href="#">Delete Message</a>'; echo '</td>'; echo '</tr>'; } }elseif($numsearch == 0){ echo '<tr>'; echo '<td colspan="4">'; echo "<p>There are currently no messages to view!</p>"; echo '</td>'; echo '</tr>'; } echo '</table>'; ?>
the problem that i am having is the $numsearch is returning "2", whereas the while loop is only displaying one message...
any ideas?
-
Hi, this is the .htaccess file that i wrote for the last website i built:
Options +FollowSymLinks RewriteEngine On RewriteCond %{HTTP_HOST} !^www\.example\.co\.uk [NC] RewriteRule ^(.*)$ http://www.example.co.uk/$1 [R=301,L] RewriteCond %{REQUEST_FILENAME} !-d RewriteCond %{REQUEST_FILENAME}\.php -f RewriteRule ^([a-zA-Z0-9_-]+)$ $1.php RewriteRule ^product/([a-zA-Z0-9_-\s\'\,\(\)\.]+)$ product.php?product=$1 RewriteRule ^category/([a-zA-Z0-9_-\s\'\,\(\)\.]+)$ category.php?category=$1 RewriteRule ^category/([a-zA-Z0-9_-\s\'\,\(\)\.]+)/price/([0-9]+.[0-9]+)-([0-9]+.[0-9]+)$ category.php?category=$1&lower=$2&upper=$3 RewriteRule ^category/([a-zA-Z0-9_-\s\'\,\(\)\.]+)/([0-9]+)$ category.php?category=$1&pageNo=$2 RewriteRule ^page/([a-zA-Z0-9_-\s\'\,\(\)\.]+)$ page.php?page=$1 RewriteRule ^search/([a-zA-Z0-9_-\s\'\,\(\)\.]+)$ search.php?search=$1 RewriteRule ^search/([a-zA-Z0-9_-\s\'\,\(\)\.]+)/([0-9]+)$ search.php?search=$1&pageNo=$2 RewriteRule ^reset-password/([0-9]+)/([a-zA-Z0-9_-]+)$ log.php?id=$1&authCode=$2 RewriteRule ^orders/([0-9]+)$ orders.php?pageNo=$1 RewriteRule ^view-order/([a-zA-Z0-9_-]+)$ view-order.php?id=$1 RewriteRule ^view-order/([a-zA-Z0-9_-]+)/([a-zA-Z0-9_-]+)$ view-order.php?id=$1&authCode=$2 RewriteRule ^news/([0-9]+)$ News.php?pageNo=$1 RewriteRule ^news/article/([a-zA-Z0-9_-\s\'\,\(\)\.~\%\*]+)$ article.php?pageNo=$1 ErrorDocument 404 /404.php
it all works perfectly.
-
Ive been given a design that incorporates almost (if not all) columns in both tables... I don't particulary fancy writing a massive query...
-
I have a lot more fields than that, thats why im asking if you can do: users.*, messages.*
-
I need to get several different bits of information from 2 tables at the same time, but im slightly confused about how i go about this.
when you have:
<?php $query = mysql_query("SELECT users.username, messages.to FROM users, messages WHERE users.username = messages.to"); ?>
can you have:
<?php $query = mysql_query("SELECT users.*, messages.* FROM users, messages WHERE users.username = messages.to"); ?>
this is because i pretty much have to select everything from each table, but i dont want to have to write a stupidly long query to do it...
-
problem sorted. now you just gotta figure out what to do with that array lol
-
theres your problem then , idont think the array has any values to output lol
-
do get the difference between an admin logging in and a normal member logging in look at my example of an if and elseif statement. so long as you change it to suit your code then it will work first time.
-
and plus if it doesnt work, try a different method? the one i posted is the one i use for my CMS, Forum, and other sites that i have built / am building.
-
The link works fine for me.
If you mean the edit-profile, then its because im still working on that file.
Same with the view-profile as well.
Thats true though some people learn by listening, some by reading, some by doing.
-
use == (equal to) not === (identical to). this is sometimes the problem with if else statements that i write aswell
-
try it and see, best way to learn what things do is to just play around with them.
-
if they have the same names then both forms would be submitted at the same time, like your suggesting i have developed a page with 2 forms.
http://janedealsart.co.uk/social/
to handle these to forms i had to give them seperate names.
<?php $register = $_POST["register"]; $fname = $_POST["fname"]; $lname = $_POST["lname"]; $uname = $_POST["uname"]; $pword = $_POST["pword"]; $rword = $_POST["pword_repeat"]; $gender = $_POST["gender"]; $login = $_POST["login"]; $username = $_POST["username"]; $password = $_POST["password"]; if(!empty($register)){ if(empty($fname) || empty($lname) || empty($lname) || empty($pword) || empty($rword)){ $regerr = "You must fill in the entire form."; }else{ if($gender == ""){ $regerr .= "You need to also select a gender, if you don't want to share this simply select 'Not Telling'."; }else{ if(strlen($uname) < 5 || strlen($uname) > 20){ $regerr .= "The username you entered is either to short or to long. They need to be between 6 and 20 characters long."; }else{ if(strlen($pword) < 5){ $regerr .= "The password you entered is to short. They need to be at least 6 characters long."; }else{ if($pword != $rword || $rword != $pword){ $regerr .= "The passwords do not match, re-enter them."; }else{ $pattern = '#^(?=.*\d)(?=.*[a-z])(?=.*[A-Z]).{6,}$#'; if(preg_match($pattern, $pword)){ $usercheck = select("username", "users", "username = '$uname'"); $ucheck = mysql_fetch_assoc($usercheck); if($ucheck == $uname){ $regerr .= "That username is already taken, try again."; }else{ $folder = $uname; $directory = $_SERVER["DOCUMENT_ROOT"]."/social/users/".$folder; $dbdir = $GLOBALS["url"]."/social/users/".$folder; if (!mkdir($directory, 0777, true)) { $regerr .= "Failed to create user directory."; }else{ $images = $_SERVER["DOCUMENT_ROOT"]."/social/users/".$folder."/images/"; if(!mkdir($images, 0777, true)){ $regerr .= "Failed to create user directory."; }else{ $uploads = $_SERVER["DOCUMENT_ROOT"]."/social/users/".$folder."/uploads/"; if(!mkdir($uploads, 0777, true)){ $regerr .= "Failed to create user directory."; }else{ $pword = sha1($pword); $newUser = insert("users", "first_name, last_name, username, password, gender, directory", "'$fname', '$lname', '$uname', '$pword', '$gender', '$dbdir'"); if($newUser){ $regpas = "You have successfully registered. You may login now."; }else{ $regerr .= "Could not register your details. Try again later."; } } } } } }else{ $regerr .= "Your password needs to have at least 1 capital letter and 1 digit in it."; } } } } } } } if(!empty($login)){ if(empty($username) || empty($password)){ $logerr = "You must enter the details you registered with before logging in."; }else{ $compare = select("*", "users", "username = '$username'"); $get = mysql_fetch_assoc($compare); if($username != $get["username"]){ $logerr .= "The username you entered is incorrect, try again."; }else{ $password = sha1($password); if($password != $get["password"]){ $logerr .= "The password you entered is incorrect, try again."; }else{ session_start(); $_SESSION["user"] = $username; $_SESSION["dir"] = $get["directory"]; header("Location: profile.php"); } } } } ?>
Sending A User A Message
in PHP Coding Help
Posted
Hmm, okay - that worked thanks! I didn't relize just putting ` around each column name made a difference...