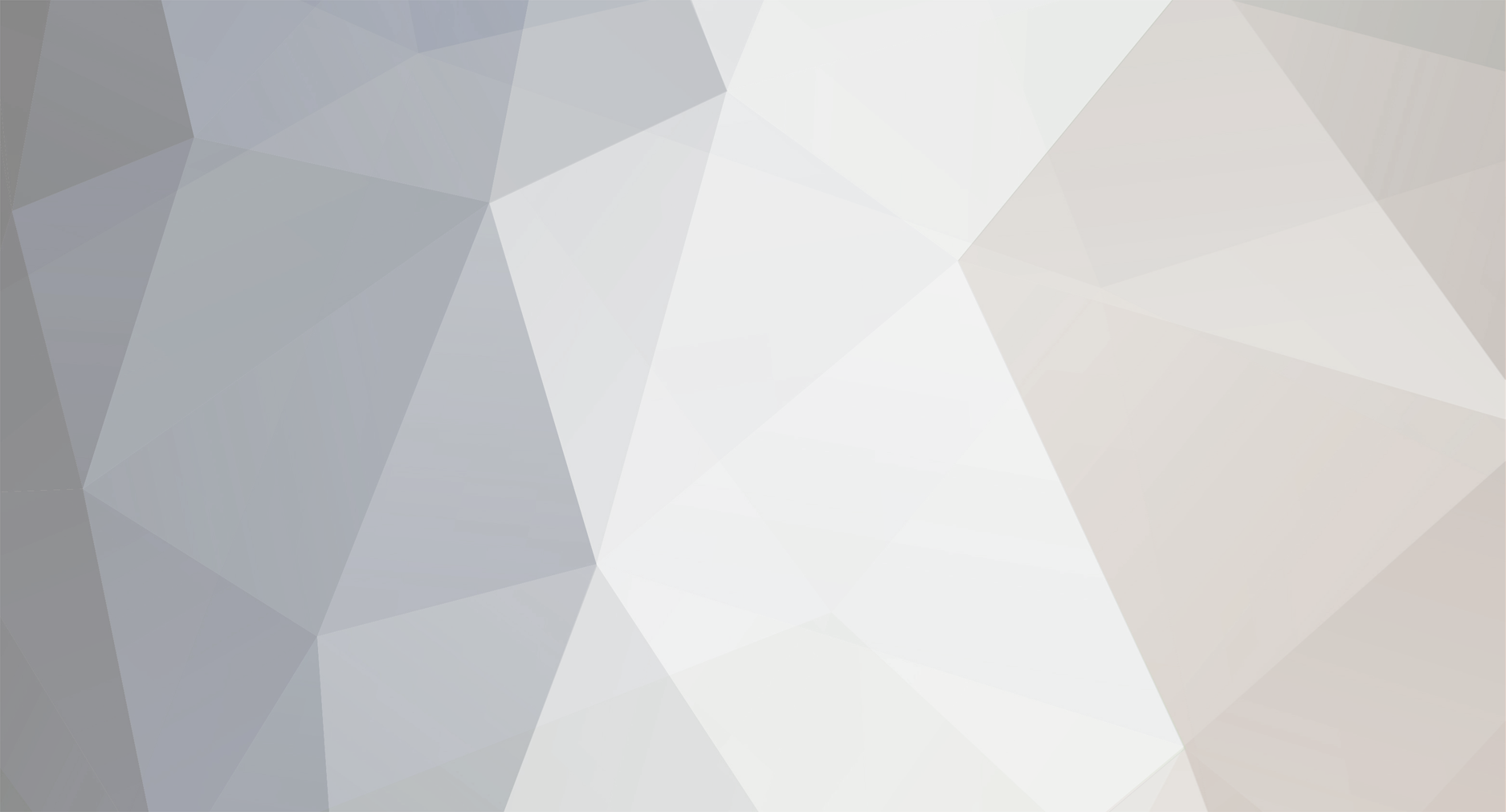
White_Lily
-
Posts
531 -
Joined
-
Last visited
-
Days Won
1
Posts posted by White_Lily
-
-
Before thinking about how people are going to pay for the item(s) you really need to get an actual site built with the products and categories listed. I suggest just sitting down and thinking about what pages you are going to have on your site what the content of those pages are going to be, and figure out a design. Then come back and ask where to go from there.
-
Okay thank you.
-
Post the code for the whole loop and then we can help you properly.
-
I was wondering how i would go about writing information to an excel file with php...
say i had data such as:
Refernce ID Product Name Price
PR56709 Product 1 £10.00
PR14820 Product 2 £25.00
How would i use PHP to enter data like that into an excel file?
I can't seem to be able to find anything useful on this using google...
Any help is appreciated.
-
Depends, what do you have already?
-
That site appears to supply the code for me, where's the learning factor in that? If there is maybe a simple tutorial you know, that would help me.
-
No, as i said at the beginning and end of my last post I want to write the script MYSELF not have someone else to write it for me, because then i would have to pay them, and i dont feel like being that generous. so in actual fact you are 0% clear. All I wanted to know was 1) is it possible (to which you replied yes and said it is not easy) 2) How would i go about it? Thats all I would have needed to know.
EDIT: Psycho, I'm not to bothered how long really, it's more of a personal project rather than a client thing. But my boss said that it could be added to our list of functionalities if I could get it working.
-
Okay. All i want to do is write a script that takes a screenshot of the website url that is entered by a user and that screenshot being saved to a directory and output to the user if the save was successful, thats all I want to do, i don't want to go through some other external site. I want to be able to write the script for it myself.
-
I meant is it possible for me to code the script rather than use some random site that you need to pay for?
-
Hi, I was wondering if it is possible to have a script locates a website that has been entered by a user, takes a screenshot of it, saves it as a .jpg in a specified directory and then outputs that screenshot to the user?
If this is possible could anyone maybe give me some links to sites that could help? I have searched the internet to very little success.
Thanks - Lily
-
Why do I have the feeling he posted some sort of school assignment? LOL.
There is a freelancing section on this forum near the bottom of the main page. Post there and people will name their price.
-
You have either written the error message wrong or you have not posted all the code, according to notepad++ and dreamweaver, there are only 397 lines, not 401.
-
For starters your msql preventing methods (mysql_real_escape_string()) etc, are almost useless as on the very first query you run you are putting the raw value of input straight into mysql without any protection on it at all.
Assign all $_POST values a unique variable at the top of your validation, this will make your script easier to read and edit later on.
I also don't believe you posted the entire scripts as in the second section of code you specify $password = md5($salt, $password); when the $salt and $password variables dont seem to exist within that block of code.
-
That is not the only way to do php validation on any type of form, there are maynt ways in which forms can be validated.
For example:
$u = mysql_real_escape_string($_POST["username"]); $p = mysql_real_escape_string($_POST["password"]); if(empty($u) || empty($p)){ //The empty() functions checks to see if the given $_POST has any type of value in it. $error_msg = "The username or password fields are empty"; }else{ //continue validation }
Another:
$compare = mysql_query("SELECT username FROM members WHERE username = '$u'"); $check = mysql_fetch_assoc($compare); if($check["username"] != $u){ //Checks to see if the username the user entered matches any members that have already registered. $error_msg = "the username provided does not match any registered users"; }
-
sessions only help if you can actually sign in in the first place lol. Plus if the password is hashed with md5() or any other method you should not just be able to copy and paste the password. This suggests that you don't fully understand what your script is doing, so start by searching particular functions in google and php.net, this should help you to understand the functions that your script is using.
Also, we cannot help you solve your coding problem unless you actually post the code that you are using or the code that is causing the problem (prefferable to post whole page of code since the problem may not lie within the error line specified.)
-
-
Okay I have it sorted. I needed to use a file path instead of a URL, but DOCUMENT_ROOT didn't work, code below:
<?php $doc = "users/".$u."/uploads/"; if(!empty($_POST['imgsubmit'])){ if (isset ($_FILES['new_image'])){ $imagename = $_FILES['new_image']['name']; $parts = explode( '.', $imagename ); $extension = strtolower( $parts['1'] ); $new_filename = uniqid().".".$extension; $source = $_FILES['new_image']['tmp_name']; $target = $doc.$new_filename; move_uploaded_file($source, $target); $imagepath = $new_filename; $save = $doc.$imagepath; //This is the new file you saving $file = $doc.$imagepath; //This is the original file list($width, $height) = getimagesize($file) ; $modwidth = 166; $diff = $width / $modwidth; $modheight = $height / $diff; $tn = imagecreatetruecolor($modwidth, $modheight) ; $image = imagecreatefromjpeg($file) ; imagecopyresampled($tn, $image, 0, 0, 0, 0, $modwidth, $modheight, $width, $height) ; imagejpeg($tn, $save, 100) ; if(!empty($width)){ $imageavi = $doc.$imagepath; $changeavi = update("users", "avatar = '$imageavi'", "username = '$u'") or die(mysql_error()); if($changeavi){ echo "Avatar Changed!"; } }else{ } } } ?>
I hate PHP >_> lol
-
I think what he is trying to say is, is there some kind of plugin he can use on his website so that when a customer of his wants to talk with him all they have to do is log in and instant message him should he be online with either yahoo or gmail.
-
Scott, sorry if this sounds rude, but if there were values in $width and $height I wouldn't really be posting here. Simply because if there were values in both variables then my problem would be around the actual maths part itself, this means just reconsidering the logic of events in the equation.
AyKay47, I have used php.ini (this is where the original connection error came from) i have used mysql_error() on the select function, and have echoed all variables possible for values, the only variables that I am concerned about is the $width, $height, and the getimagesize() php function, as these don't have any values, or have the wrong values. I have also checked the path/url provided to getimagesize() and the image existed and shows in the browser.
-
Okay so i took jess' advice and used a path, the connection error disappeared but it is still not caluclating the width and height of the image.
The path i used is:
$doc = $_SERVER['DOCUMENT_ROOT']."social/users/".$u."/uploads/".$new_filename;
-
because each user has his/her own directory, it isnt just a single imagethats being calcuated, the image that is uploaded by the user could be 45px x 9px, or 165px x 164px
-
I copied and pasted the file path into the browser tab and the correct image came up, so other than that im not entirely sure what you mean?
-
Okay, so i have written a php calculation that determines what the margin left and top will be of a users avatar.
I have a slight problem in the way that the script can't find the image to get the size of it.
The Error:
Warning: getimagesize(http://janedealsart.co.uk/social/users/Guest/uploads/50a3c6fe2a699.jpg) [function.getimagesize]: failed to open stream: Connection refused in /home/sites/janedealsart.co.uk/public_html/social/profile.php on line 22
This is the code I have written for it:
$getbg = select("*", "users", "username = '$u'"); $bg = mysql_fetch_assoc($getbg); $pic = $bg["background"]; $propicmargin = $bg["avatar"]; if($propicmargin){ list($width, $height) = getimagesize($propicmargin); // <-- LINE 22 /*//Calculates Height of images that have an exact width of the box. if($width == 166){ $margin = 166-$height; $margin = $margin/2; if($margin < 0){ $margin = 0; } }*/ //Calculates Width & Height of small images to find the margin left & top. if($width < 166 && $height < 166){ $margin = 166-$height; $margin = $margin/2; if($margin <= 0){ $margin = 0; } $marginw = 166-$width; $marginw = $marginw/2; if($marginw <= 0){ $marginw = 0; } } $avatar = '<img src="'.$propicmargin.'" />'; }else{ $avatar = '<img src="'.$GLOBALS["nav"].'images/avatar.png" />'; }
Any Ideas? Folder permissions are 777.
The image exists and the file path is correct.
-
okay, so i decided to change the avatar on a completely new account, what i found is that 83px margin left and top is a set value, it does not appear to have anything to do with the calculations but something else :/
Sorry for this slight bother
Upload File Script - Amend It To Check Size?
in Third Party Scripts
Posted
You could make this fairly easy and try to learn how to create a file uploading system yourself. That way error checking would be much easier and you would be able to customise what type of files go in and out of your system.