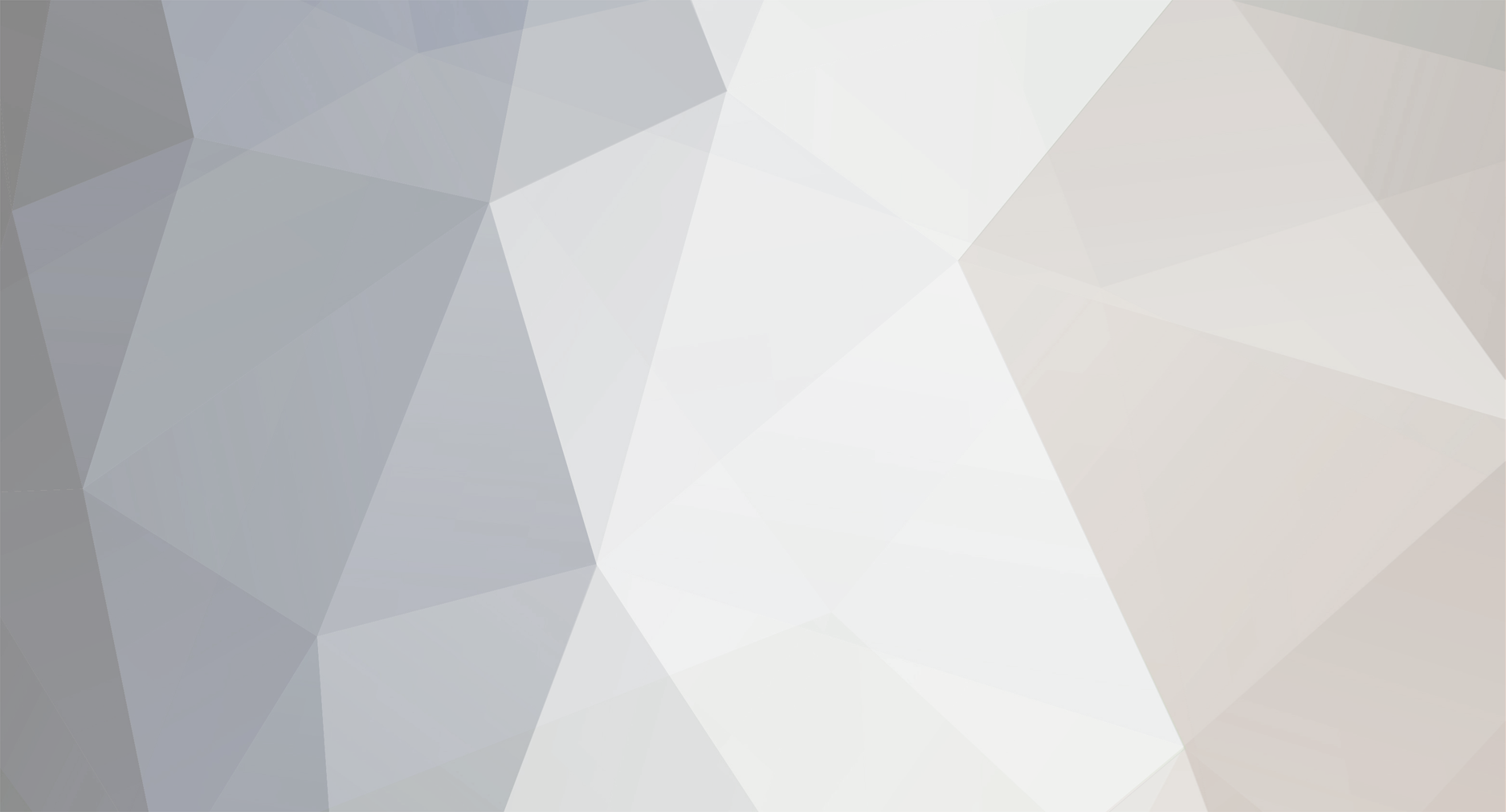
akphidelt2007
Members-
Posts
174 -
Joined
-
Last visited
-
Days Won
2
Everything posted by akphidelt2007
-
Proper Directory Structure And How To Loop Through It
akphidelt2007 replied to MockY's topic in PHP Coding Help
The way I typically store them is just a numbering system based on the autoid. Something like this... $increment = 100; $customerID = 1205; $int = intval($customerID/$increment); //the folder will now be 1200_1299 $folder = $int*$increment."_".(($int+1)*$increment-1); You can layer this if you expect 10s of thousands of customers or change the increment level to store more than 100 customers in a single folder. Otherwise, you just check if the folder exists and then store your file in there with whatever naming convention you want. Like customer_1205. Just one of many options. -
You are storing the php in a variable so there is no reason to need the php tags and to echo anything. <td style="padding:10px 14px 10px 7px; vertical-align:top; text-align:right;">'.WA_eCart_DisplayMoney($enersolmaCart, $enersolmaCart->DisplayInfo("Shipping")).'</td> At the end of the script you have to echo out $strMessage
-
Have you established a connection to the database? Try $query = mysql_query("SELECT * FROM templeagues WHERE templeague='$templeague'") or die(mysql_error());
-
Use a checkbox array... and add the database id as it's value. <input type='checkbox' name='ids[]' value='1'> //check $_POST ids if(isset($_POST['ids'])) { //loop through them foreach($_POST['ids'] as $id) { //$id is the id of the row selected. This is where you do whatever it is you want to record them for your basket } }
- 2 replies
-
- php
- postgresql
-
(and 3 more)
Tagged with:
-
Object Oriented Programming Or Traditional Coding?
akphidelt2007 replied to mostafatalebi's topic in PHP Coding Help
It's all good. The programming elite get on my nerves sometimes. Not everyone is an expert programmer and it seems they forget that. -
Object Oriented Programming Or Traditional Coding?
akphidelt2007 replied to mostafatalebi's topic in PHP Coding Help
When you say comments like... "The problem in your application was not the lack of objects." "Do you understand what abstraction is?" "What you're talking about is abstraction, not OOP." You have absolutely no clue what my project even was or my understanding of programming and you are already throwing out your snobby little comments. What I'm talking about is not only abstraction. And abstraction is part of OOP. I have no clue what magical OOP you do. -
Object Oriented Programming Or Traditional Coding?
akphidelt2007 replied to mostafatalebi's topic in PHP Coding Help
Creating Tables Create Templates Creating Hierarchies Creating Dynamic Query Strings attached to filters and authentication Loading up files and being able to use all the previous created variables with out passing them along Loading and unloading templates Form Creation/Handling Loading AJAX files and JS Scripts on the fly Handling project specific problems like materials, scheduling, modules, resource loading, etc. I'm not saying this can't be done with procedurally... but I can say with 100% satisfaction that it is much easier with OOP and my efficiency and organization has gone up exponentially. Being able to create all the pieces to the site before outputing it makes it much easier to add and subtract the pieces you want. All I'm saying is you have absolutely no basis for what my project was, what my problems were. All I was doing was giving a poster some information based on my personal experience. There is no reason to always be a little programming snob. -
Object Oriented Programming Or Traditional Coding?
akphidelt2007 replied to mostafatalebi's topic in PHP Coding Help
I never said it was a lack of objects. I said the problems in my code made me understand how OOP could be beneficial to me. Sure I could have rewritten the code procedurally more efficient each time. But why do that when you can put all that work in to objects and reuse it for future projects. Nice try, but you fail. -
Object Oriented Programming Or Traditional Coding?
akphidelt2007 replied to mostafatalebi's topic in PHP Coding Help
It might be best to do version one procedurally. OOP works best when you can understand the benefit of it. And there is no better way to get an understanding of the power of OOP than writing out an application procedurally. So right now if you are asking what you "should" use... than OOP probably would not make your life any easier. My first application that was ever actually used in the work place was written procedurally and worked for over two years. Now, it was a heaping mess and I had to act like Sherlock Holmes to figure out where and how to make changes... but it still worked. But this process made me understand exactly what power OOP can bring to your application development. Some of us non programming computer science elitist need to get a job done... and without having the proper background in understanding OOP, you just learn trial by fire. -
Object Oriented Programming Or Traditional Coding?
akphidelt2007 replied to mostafatalebi's topic in PHP Coding Help
As long as your client is happy it doesn't matter how you write it. BUT, that means if there is a problem in the future and it takes you days to pile through a mess of procedural code than your client probably will not be happy. If you write it neat and tidy and you have good documentation of how the site works than there is nothing wrong with how you write it. Just in my personal experience, OOP makes all the tedious, redundant work easy and let's you get to the meat of the code and all the fun stuff right away... which also greatly improves your efficiency. -
Adding Custom Function To An Object
akphidelt2007 replied to akphidelt2007's topic in Javascript Help
So I'm still trying to put the pieces together. Here is more of a clear picture of what I want. $this->ajax->loadScript( $(function() { var tbl = new Tablev2('beta'); tbl.init({'mysqlTable':'msr_data'}); //right here, I want to add the ability to calculate the cost based on the unit_cost and qty //I'd like to do something like this tbl.addFunctionSomeHow(function() { $('#qty').blur(function() { alert(this.activeCell.attr('id')); }.bind(this)); }.bind(this)); }); ); [/Code] This is literally just an example of what I want to do. I want to be able to have access to this so I can manipulate the table via the custom function. Do not worry about the actual validity of the function above. I just types it up for a broad example of what I'm trying to accomplish. Thanks again. -
Ok, I really should know this by now and I'm in a time crunch so I thought I might just ask to see if someone can point me in the right direction. So I build a table class in jquery. What I want now is to be able to handle custom functions that come about for the individuals tables I create. What I do now is have a customChange function and just use switch() to find the keyword and then run the function. But this is mighty inefficient and I have to constantly go in to the object and add the single custom instance. So for example, say on one table when the Qty is changed I want the Cost column to autocalculate and trigger a warning if the cost is above a certain value. I load up my javascript like this... $this->ajax->loadScript(" $(function() { var tbl = new Tablev2('beta'); tbl.init({'mysqlTable':'msr_data'}); tbl.ajaxDD('tid',{'text':'abbr','tbl':'budget_cats','locked':true}); tbl.ajaxDD('mid',{'text':'description','values':'mid','tbl':'modules','width':400,'numChars':2,'locked':true}); tbl.ajaxDD('sid',{'text':'description','values':'old_pav_sid','tbl':'systems','width':400,'numChars':2,'locked':true}); tbl.ajaxDD('manufacturer',{'text':'manufacturer','tbl':'msr_data','width':200,'numChars':1}); tbl.ajaxDD('line_description',{'text':'line_description','tbl':'msr_data','width':600,'numChars':2}); tbl.customChangeEvent('sid','getBudget'); tbl.customChangeEvent('per_unit_cost','calculateCost'); tbl.loadCustomMysql('tid',{'tbl':'budget_cats','matchField':'abbr','returnField':'bid','int':1}); tbl.loadCustomMysql('sid',{'tbl':'systems','matchField':'old_pav_sid','returnField':'sid','int':1}); }); "); How can I type up the function within the $this->ajax->loadScript function so I do not have to actually manipulate the object but still use the variables within the object? The way I do it now is through the tbl.customChangeEvent() and then I just write the function in the class itself. I want to be able to write the function on the script that is creating the table. Any help will be greatly appreciated. Thanks
-
Next Number In Sequence Following Specific Value
akphidelt2007 replied to dlebowski's topic in PHP Coding Help
I would just do a query and loop through it to get the next number. For example $lotNumber = 100; //this gets all lot numbers between 100 and 200 $qry = "SELECT lotNumber FROM lots WHERE lotNumber BETWEEN $lotNumber AND ".($lotNumber + 100)." ORDER BY lotNumber"; $result = mysql_query($qry); while($row = mysql_fetch_array($result)) { if($row[0] != $lotNumber) break; else $lotNumber++; } [/Code] Now the next available number is $lotNumber and just insert the value in to your db -
Trigger Onblur Event Even With Very Fast Clicking?
akphidelt2007 replied to akphidelt2007's topic in Javascript Help
Thanks for your replies. Drongo, I actually ended up with your advice by pure accident. When a user was clicking in to the text box it would create another text box, so I built that flag to make sure that an active box was already present and if it was then skip the code process. That ended up doing the trick. Thanks again -
Ok, so here is my little dilemma. I have a table that acts as a spreadsheet and allows the user to click a cell and pops open an input box. And when a blur event is triggered on the input box it runs some ajax to submit the data in to the database and then closes the input box and adds that value to the cell. My problem is, if I click really really fast on multiple cells, multiple input boxes pop up and the blur event is not triggered. Is there a way to handle this type of action. I know it's nitpicky, but I can just imagine some of our engineers clicking too fast and getting this little bug. Please let me know if there is anything I can do about this. Thanks in advance!
-
Removing A Select Drop Down Onchange And/or Onblur
akphidelt2007 replied to akphidelt2007's topic in Javascript Help
This is my bad, I did not bind correctly and the problem was happening within the code itself and not the logic. Sorry for wasting your guys time! -
Ok, so here's my problem. I have a table class built in javascript using jquery. What I want to add to the class is select box functionality. I have been using ajax search type functionality which has worked, but now, I want to add an actual select box. So when a user double clicks on a cell, a select box pops up in the cell. When a user changes the select box it is easy and I can run a change event to submit the data to my db and update the cell. This also gets rid of the select box. My problem now, is the hypothetical event that a user double clicks the cell but does not change anything in the select box. What would be a possible strategy to allow the onchange event to trigger but also allow an "onblur" event to happen if the user does not select anything. I don't need all the coding, just a theory or help from anyone who knows how to do this. Thanks in advance
-
I personally use a query building class to build dynamic query strings and a static database class to execute my queries. As you saw in the example I gave you, that is what the "DB::returnArray()" is. I don't know all the super programming jargon for these concepts, but I will try to explain them the best I can. What I do, that has worked for me the past 2+ years, is to kind of build a maze with a prize at the end (the display). And then pretend you are the user. What is the first thing you need to do when a user comes to the door to enter the maze? And remember there is only one door. In this ridiculous analogy that door for me is index.php (most of the time). I do not set up the site where it has .com/file1/file2/lastFile.php. I use GET variables to do my file finding for me. So for instance this would be index.php?firstPage=file1&secondPage=file2&display=lastFile. Of course that's not my actuall GET keys and logic... but you get the picture. This way there is only one entrance to your site. And if you ever need to change the code for what you need to do when a user enters the site, there is only one location to have to do this. And if anyone tries to enter a hidden door to the site, you make sure they are punished!! I do this by setting a constant in the index.php that only gets created if they enter that door. Then every file checks to make sure this constant is set. If it is not, I know they are trying to break through a trap door. So then I check the users authentication, set up the sessions, get all the necessary backend logic done before the user starts his path. Then I give that user a class (I call it the Brains). As the user goes through the maze, I gather information and store it in this class (well there isn't really a different class for each user, it's the same class, but you get the point). So if the user goes to the Admin door. Before they can enter, they have to go through another security checkpoint. So pretend they are trying to get to the accounts section to change a users e-mail address. This accounts section is within the admin controller. So my url for example would look something like this .com/index.php?c=admin&b=accounts. C is what I consider the controller, and b is the brain or logic within that controller. So I first go to the admin.controller.php file. That is the one door to get in to the admin section. There is only one file for this. So if that file contains a header or a navigation panel... I throw it in to my brains class storing it as a template. I also populate the variables that are needed for this navigation panel. Say you permissions set up where certain users can only access certain doors within the admin section. Then I store those variables, and move on to the next logic portion. That would be the accounts section. I do the same thing here. Then if everything checks out, all the security checkpoints pass, the path leads to the finish line. I then take all the information I gathered during that path and create the final display. There is no including html files during the maze. That happens at the very end once you KNOW that the user has a chance to see this. This is just a simple analogy, but you get the picture. During this process you can handle all sorts of different errors, relay messages to the user, etc, etc. So that is one benefit of OOP is that you can store things across files. So when I load up the accounts file. I do something like $this->loader('brains.accounts'); //Now, then the brains class includes this file within the class. So now I can use everything I've gathered already within the accounts file. //So the accounts.brains.php file I can use $this->auth->doSomething(); //or $this->get('post.postKey'); So it really makes life a lot easier when there is only one path the user can go through and you collect all the data throughout that path before you use that data to create the actually display of your site. Now that I reread this, I realize it's a pretty bad analogy... but I'm going to post it anyway, lol. Good luck.
- 12 replies
-
Stick it in your html... <?php if(isset($_SESSION['user_id'])) { ?> Welcome | Log Out <?php } else { ?> <form action="login.php" method="post"> <p> <label>Username</label><input type="text" name="username"> <label>Password</label><input type="password" name="pwrd"> <input type="submit" name="submit" value="LogIn"> </p> </form> <?php } ?> That's just quick and dirty but hopefully you get the drift.
-
It's great if you plan on doing multiple projects. Then if you get more advanced and can build your own framework you can whip out the backbone of sites instantly without going through the painstaking process of starting from scratch. All the meat rests in your code. For instance, my projects typically involve lots of tables of data. So I created a table class... and instead of having to do all the looping through data and building the html... I can do this. $data = DB::returnArray("SELECT id,mid,description,tenrox_id FROM modules"); $tbl->packets( Array('key.mid','right','w.15','title.MID','class.testClass') ,Array('key.description','w.65','title.Description') ,Array('key.tenrox_id','title.Tenrox ID') ); $tbl->loadData($data); $this->vars['tblHTML'] = $tbl->Buffer(); That right there is a basic example but builds the entire table, loops through the data, pulls the data from my database class and stores it in a variable for later use where I insert it in to my templates. And if I ever need to change something up in my tables for a project, I just have to go to the class and make one change... rather than going to every file that has a table and making the change. It's not necessary at all, but once you learn the power of it, it becomes something you can't live with out. It makes everything more efficient, manageable, and useful. Your clode becomes cleaner, you can automate a lot of uncessary typing and you can link your entire system up that makes it very easy to add pages and manage pages. It seems useless at first because you can get by with just using functions and coding yourself, but trust me... once you go OOP you will never go back.
- 12 replies
-
It will not show up if it is not clicked so you can easily do... $allow_dupes = isset($_POST['allow_dupes']) ? 1 : 0;
-
Well than that can't be it. Show the updated delete_old_prof_img function.
-
Well most likely in another one of your function you are updating the database. Because if you put that code in to your delete old prof function you would not end up with the resource id. What I'm guessing is change_prof_img is doing the update/insert and coming up with the resource id.
-
Just for future reference if you ever want to put a value in to the auto-increment field without rewriting the auto-number or causing a duplication error, use NULL with out quotation marks as your value.
-
Oh I didn't read all the way through, apparently you did try using $old_path and you came up with the resource id. Try this. $old_path = mysql_query("SELECT `prof_img` FROM `users` WHERE `user_id` = " . (int)$user_id); $old_path = mysql_num_rows($old_path)==0 ? false : mysql_result($old_path,0);