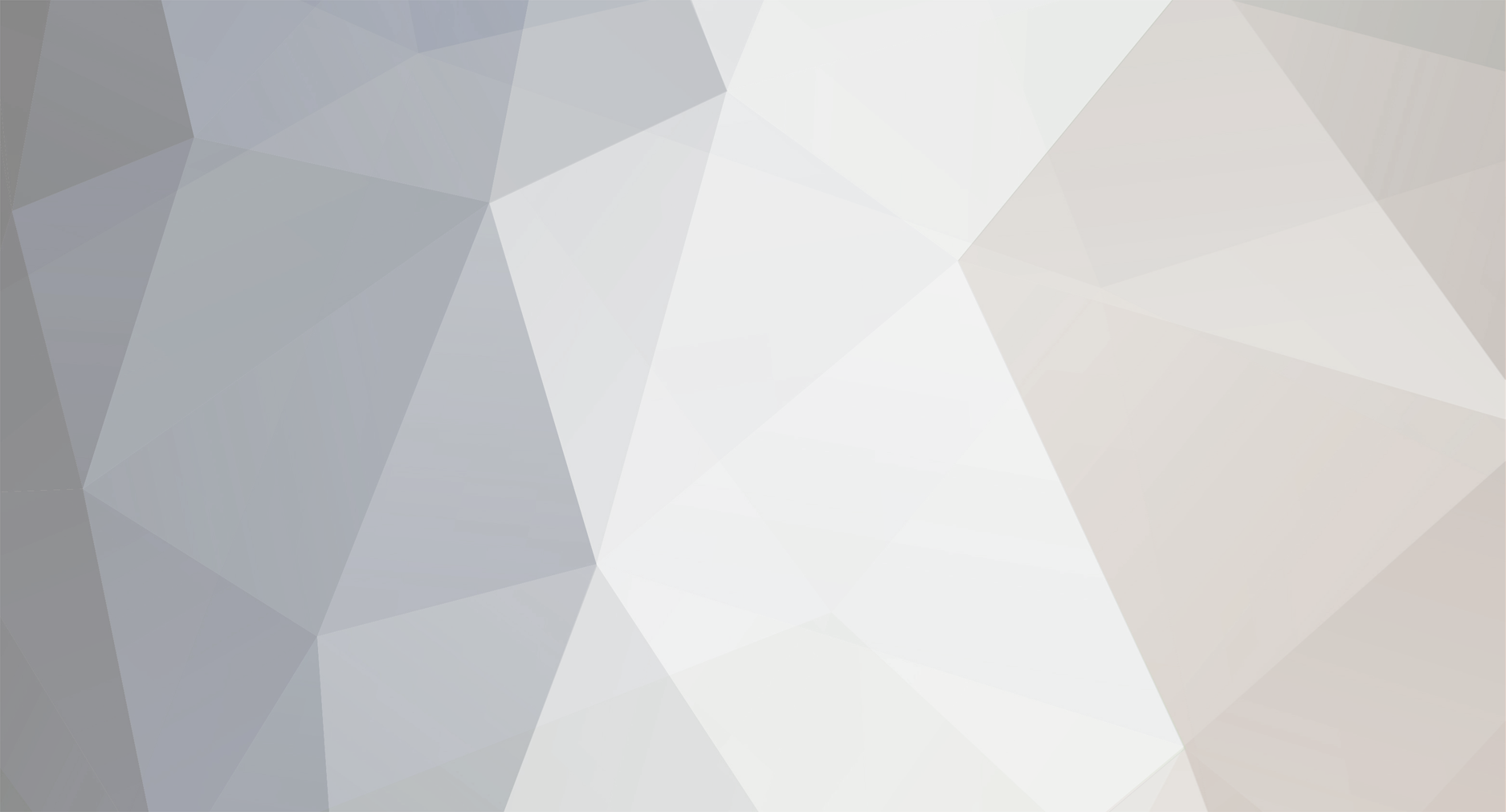
keeB
Staff Alumni-
Posts
1,075 -
Joined
-
Last visited
Everything posted by keeB
-
Break everything in to a 'piece' of the puzzle. <?php class AlbumDao { private $dbconnection; public function getImageByName($dir) { //find an image in a certain directory; } public function getImageById($id) { //select * from images where id = id } public function initialize($albumName) { // select * from album a // inner join images i on i.album = a.id // inner join videos v on v.album = a.id // where album.short_tile = '$albumName' } public function setDbConnection(DatabaseConnection $dbconnection) {$this->dbconnection = $dbconnection;} } class Album { private $name; private $title; private $dir; private $dao; public function __construct($name) { $this->name = $name; $this->init(); } private init() { $this->dao->initialize($this->name); } public function getImageList() { // some helper functions which return a list of Image objects } public function getVideoList() { // some other helper functions } public function setDao(AlbumDao $dao) {$this->dao = $dao} } class Image { private $filename; private $caption; public function __construct() { } public function draw() { // echo <img src .... } } ?> I just wrote this object diagram as I went along, there could be some oversights, but this is how I would start.
-
That worked. Thanks
-
I am manually parsing an HTML doc and want to read each row of a table. The HTML I want to gather looks like this: <tr class=mbrow1> <td align=center><img src="/images/boards/closed.gif" border=0 alt="Locked thread"></td> <td>Pinned: <a href="/tool/post/scbbbc/vpost?id=1038138">Message Board Policies - *Please Read*</a></td> <td><a href="/tool/view/mb/profile/scbbbc/Kodiakjo" title="View profile">Kodiakjo</a></td> <td align=center>787</td> <td align=center>0</td> <td> <table cellpadding="0" cellspacing="0" border="0" width="100%" class=mbrow1><tr> <td>04/06/06 at 10:35 AM by <a href="/tool/view/mb/profile/scbbbc/Kodiakjo" title="View profile">Kodiakjo</a></td> <td align="right" nowrap> <a href="/tool/post/scbbbc/vpost?id=1038138&trail=#1"><img src="/images/boards/page.gif" border="0" alt="Go to last post" title="Go to last post"></a></td> </tr></table> </td> </tr> The regex I have so far is: "<tr class=mbrow\d+>(.*)</tr>" That appears to be grabbing the first instance of mbrow1 and grabbing the LAST </tr> How to make it grab first instance of </tr> ?
-
[SOLVED] "must be an instance of string, string given", wait what?
keeB replied to jordanwb's topic in PHP Coding Help
You can still do it with a string, fyi Pretty sure this works <?php function lol (string $a) { echo $a; } $mystr = (string) "hello, world!"; lol($mystr); ?> -
Discuss - should classes ever call existing objects?
keeB replied to deadonarrival's topic in PHP Coding Help
That's so stupid to include queries in any place but an encapsulated object. Your client code should NOT CARE where the data comes from, so it can easily be switched out in case requirements change, the implementation changes, whatever. Testing a single instance of an object INSTEAD of the entire client is so key when you re-visit an app(or portion of an app) you haven't worked on in a while. You can program however you'd like, but NO THANK YOU. If you never have to maintain or change your software, then god bless you and congrats. -
Singleton abstract DB class, with mysql, etc extends...
keeB replied to Naez's topic in PHP Coding Help
Define the interface.. <?php interface Database { function connect($connectionString, $userName, $password); function select_db($db); function query($q); function num_rows(); function next(); } ?> Write a (concrete) implementation (wrote this a while ago, could be improved upon): <?php @include_once("database.interface.php"); @include_once("sql.exception.php"); class mysql implements Database { private $connection; private $resultSet = NULL; function mysql($host, $userName, $password) { if(!extension_loaded("mysql")) { throw new SQLException("MySQL not loaded"); die(); } try { $this->connect($host, $userName, $password); } catch (SQLException $e) { die($e->message()); } } public function connect($host, $userName, $password) { $this->connection = @mysql_connect($host, $userName, $password); if (!$this->connection) { throw new SQLException("Unable to connect." . mysql_error(), 1); } } public function select_db($database) { $selected = mysql_select_db($database); } public function query($q) { $this->resultSet = mysql_query($q); if (!$this->resultSet) { throw new SQLException("Error executing SQL statement. Here's the error: " . mysql_error()); return false; } return $this->resultSet; } public function next() { //nothing yet } public function num_rows() { if($this->resultSet != NULL) { return mysql_num_rows($this->resultSet); } throw new SQLException("No rows"); } } ?> Write an Abstract Factory (singleton which gets a concrete implementation based on context) <?php class DatabaseFactory { public static function create( DatabaseConfiguration $request){ switch ($request->type()) { case 'mysql': return new MySQL($request->host(), $request->uname(), $request->pwd()); default: throw new InstanceNotFoundException("Database type not supported"); } } } ?> -
This is an OOP forum not a teach the man terrible programming habit forum Globals ARE bad. I don't care if your favorite package uses them, they're bad. I also don't care if your global is an array of values. That's worse. I'm still not getting what needs to be configured. I can't help you with a good design if you don't tell me when you're trying to do. I agree here.
-
Someone didn't pass highschool math
-
require_once is the way to go. __autoload is one of those things that is good on paper but goddamn is it hard to debug if issues arise.
-
Shouldn't Admin be a type of User?
-
OOP is language independent.. it's just a completely different way of thinking. The (benefit/downfall -- you pick) of PHP is it's loosely typed. Meaning, return values aren't static, but can be an int, string, array.. which can (potentially) be cause for confusion for 'beginner' programmers when they want to do things 'correctly.' While the code from that article can't be directly ported, things like Syntax and explanations can be understood. If you're new to this I don't expect you to understand all of it right away -- I have been at this for a while and I still don't understand everything he says Programming (especially in OOP) is practice. You don't get anywhere if you are set on doing it right because you learn at such a quick pace. Your style and preferences will evolve over time. Happy coding!
-
If it's db information I'll show you what you can do if you need a .properties file or something. Out with it!
-
He's asking for 2 dimensional (2D) arrays. Basically, you don't instantiate them All kidding aside, my preferred method is the following: <?php $d2 = array("dimension2" => "this is dimension2"); $d1 = array("dimension1" => $d1); ?>
-
Someone just recommended use of globals? You're crazy! 1) Objects properties should always be private 2) Globals are bad, mkay? Give an example of where this would be necessary and I will help you with the design.
-
Not possible. But it would be nice, eh? I've tried to do typecasting for custom objects before and it's not possible. The best solution for you, though, is to either write an Iterator, or.. have the User->__construct() method take a raw array and parse it.
-
What part is confusing and cannot be ported to PHP? I'll answer any questions you may have
-
Interesting idea. Is the background image customizable? If so I didn't notice it..
-
I'm going to quote Allen Holub twice in the same week. My goodness! http://www.javaworld.com/javaworld/jw-08-2003/jw-0801-toolbox.html Read this, love it, learn it. I would do what you want to do in the following fashion: <?php interface User { //common functions } class BasicUser implements User { // functions which need to be implemented } class BeefyUser implements User { //what makes the beefy user different } ?>
-
This has nothing to do with globals. You use :: outside of a class when it's a static object. Your second code example is wrong. It should be the following: <?php $auth=new Auth(); //Won't bother with passing construct arguments in the example if($auth->logged_in){ //User is logged in } else{ //Guest } ?>
-
interesting Why do you save the text as a file?
-
Yeah, I'm a huge vim fan. Haven't used any other text editor in years. While I am a huge fan of VI for editing... Managing 'large' projects can get a bit ridiculous. At least for me ;x
-
Feedback: Great entry in to the OOP world My last word of advice on this topic. You can't Do It Right from the beginning. Your class is fantastic. If you'll ever want to: reuse it, modify it , extend it,maintain it.. you'll then come to understand some of the limitations of your implementation and come to understand the reason I laid out the object above. Truth is-- you won't understand this unless you have to go through it (or unless you're formally taught and given feedback.) I've had to completely tear projects apart because I designed it poorly from the beginning. Thinking about design from the start and potential problems help alleviate this from the beginning
-
As you can see, I have broken down each part of the messaging system to encapsulate it in to different 'parts' These parts usually have a single or really simple piece of the puzzle, and it is the cooperation between these that give you the ultimate flexibility. We have templatized most of the messaging system for common goals which can be re-used and 'plugged' in elsewhere. Say you want your inbox to support UNIX file based MailDirs, an XML file, whatever. Your client code shouldn't care *where* this information is coming from or really what to do with it other than to tell it when to *do* something (like display itself.) This method of design allows for that flexibility.
-
<?php interface MailReaderDao { public function retrieve($user, $folder); } public class MessageList { #container for messages private $items = array(); public function add(Message $item) { $this->items[] = $item; } public function read($index) { return $this->items[$index]; } public function readBySubject($subject) { # find a message by subject } } public class Message { public function __construct($raw) { # parse the message object and provide } #read should take a parameter like where to draw itself public function display($where=null) { if (where=null) { #write to stdout, print $this->subject; print $this->sender; } else { # who knows, probably not necessary, you may not be writing to anywhere other than a #browser, but this is for the sake of using a pattern like MVC. } } } public class DatabaseMailReader implements MailReaderDao { private $db; # stores the database connection public function __contruct(DatabaseConnection $db) {$this->db = $db} #must define retrieve() method public function retrieve($user, $folder) { #return a messagelist object #db->query is an object which returns all results as an, normally you would #implement a factory (or something along those lines) which returns the MessageList #for you. We can pretend that this method would be plugged in to said Factory. $q = "select subject, sender from inbox where userid = " . $user->userId; $result = $db->query($q); $list = new MessageList(); foreach ($result as $message) { $mObj = new Message($message); $list->add($mObj); } return $list; } } ?>
-
If you're going for a completely OO centric way, this is what you do (please remember this is a prototype, i am not going to write the actual code) Writing the rest right now