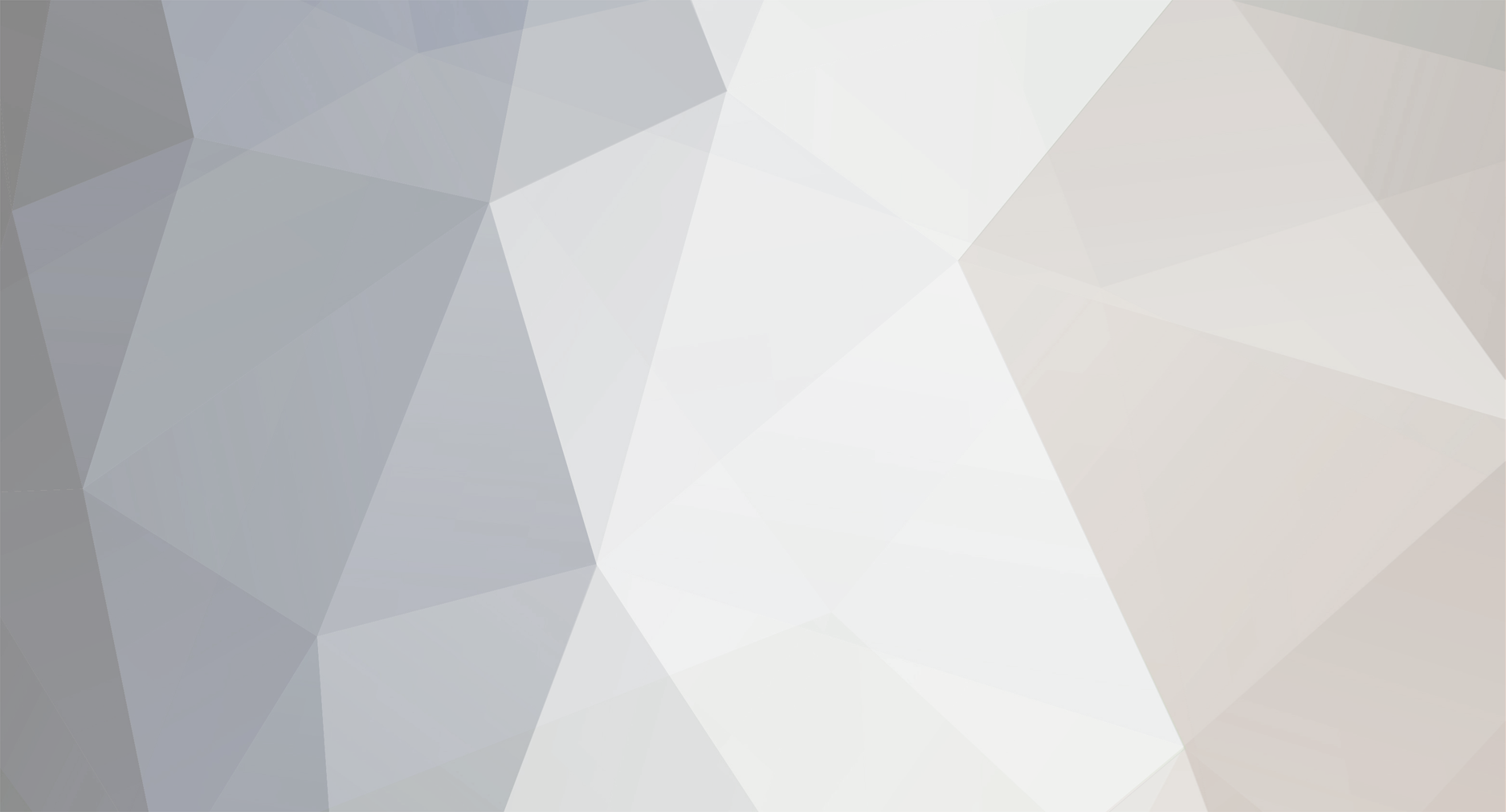
DaveyK
Members-
Posts
289 -
Joined
-
Last visited
-
Days Won
1
Everything posted by DaveyK
-
I dont understand what you are trying to say :/ the entire $structure array is dynamic. It stores a lot of info on all the cards/tiles that should be displayed on the page.
-
You can actually store the path to the image in a database and have both the random number as a file name AND a display title if you need it. Also, the name of the image is defined by $RandomNumber.
-
Turn on error reporting. I think its: error_reporting(E_ALL); ini_set("display_errors", 1);
-
I am building an app where the content of that page is defined by "cards" or tiles. Regardless, I store the title to that page in the database. For, say, a user profile I COULD store "User profile" as the pge title, but instead I want to show the actual username. So, in the database, I store a string that I can replace. Something along the lines of :name. So on the page itself, I make the PHP recognize that string and let it find the variable to match, which means I have to dig deep into an array which holds all of the cards and the relevant information. I could try to move the actual info much higher in the array, but I am always required to find the variable inside an array... CASE: I want a dynamic page header where the database is (obviously) rather static. ":name" should be replaced to "Davey Kulter" where that name (which is SURPRISINGLY my name) is stored inside an array.
-
Do you have error reporting turned on? If not, it would be wise to turn that on. for one, you are missing semi colons. (';') on the echo's and using var_dump() instead of echo is much better in cases like this.
-
I need to access some variable inside an array, based on another variable... I know its really stupid and not that nice but lets just say thats what I need. What is the cleaner method then? Ive done the google research, but I mostly find how to echo an array or something :/
-
Werid csv encoding, strlen returns twice length it should
DaveyK replied to NeilWalden's topic in PHP Coding Help
CSV is comma seperated right. Can you verify IN THE FILE that the commas are not the issue here ? -
My first advice would be: try something! Or follow this link RTFM
-
Okay, I am experiencing a really weird where this gives different results: $str = 'structure[0]'; var_dump(isset($structure[0])); // bool(true) var_dump(isset($$str)); // bool(false) Does anyone know how this could be caused? Am I missing something?!
-
We are free to criticize the work you display as we find fit. You will only benefit from what we say. All points are valid to the displayed code althought not all are directly related to the subject at hand. Give yourself a break to learn from others who actually have something to share.
-
You should parse code in between the code tags, identified by the "<>" icon.
-
And cushion for comfort... There are plenty of tutorials on this... You can either store it in a database (which would make the most sense) or store it in a file. If you store it in a file, you could serialize or JSON encode an array and then decode it for full functionality. You can also store each entry on a new line. Whichever suits your need. Get creative, search the webs. Dont ask us to do your stuff for you.
-
Well, where to begin. - First, allow me to notify you that you shouldnt be using mysql. Use mysqli or PDO mysql instead. - Second, since you are using mysql, you need to prevent mysql injection and escape the variables. I cant see if you are doing that or not, but I hope you are. - if you want to understand your $_POST request better, you can echo it using: echo '<pre>' . print_r($_POST, true) . '</pre>'; - this line: mysql_affected_rows(); wont echo anything. You need to put echo in front of it. - dont use center tags. CSS can do that better and <center> tags seem really outdated. - Lastly, what in the world are you doing to store data in such a way? Perhaps you could normalize your data and store it in several databases? that should give some insight as to what the PHP is receving and it might help you determine what to do next
-
You PHP supported mysqli and PDO mysql, but not mysql() itself. That means your php is set up looking forward, rather than using old outdated mysql() stuff. mysql_* is deprecated as of 5.3.x (I think) and you shouldnt use it. Instead either use mysqli or PDO mysql, which is exactly the same query but the PHP around that query is different (they are both OOP). If you have any questions regarding mysqli() or PDO mysql, we are here to help obviously.
-
Sure. I am fairly new to PDO as well, so it might be wrong what I say here, but here it goes. PDO is a php library for connecting to a mysql database. That means it is OOP. You create a PDO connection by doing this code: $pdo = new PDO('mysql:host=HOST;dbname=DB_NAME;charset=utf8', USER_NAME, PASSWORD, array( PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION, PDO::MYSQL_ATTR_INIT_COMMAND => "SET NAMES utf8" )); $pdo->exec("set names utf8"); Just replace the CAPS by whatever is relevant to your site. HOST is like an IP address and DB_NAME is the database name and so forth. I am using an UTF-8 (Unicode) charset so I went overboard making sure verything is utf-8. You can remove that part if you want to. Say you have some query, like: SELECT * FROM users WHERE users.id = $id In PDO, you can do $pdo->prepare('SELECT * FROM users WHERE users.id = :id'); $pdo->bindParam(':id', $id); $pdo->execute(); $result = $pdo->fetch(); // this gives a single array Does that make sense?
-
So write code that allows him to without the security hazard ?
-
Yes, you can use mysqli() or PDO_mysql() to prepare a statement PRIOR to the for loop, and then you bind the param inside the loop and execute the query dont use mysql_*, always use mysqli() or PDO_mysql for php libraries if that is an option. HERE is an example for pdo: $pdo = new PDO (); $sql = "INSERT INTO ................"; $stmt = $pdo->prepare($sql); for () { $stmt->bindParam(':file_name', $name); $stmt->execute(); } this WONT work but will give you a general idea about what it might look like
-
I dont see why this wouldnt work: <?php $files = $_FILES['upload']; foreach ($files['name'] as $k => $file) { var_dump(move_uploaded_file($files['tmp_name'][$k], "user_images/{$username_entry}/{$file}")); } what does that do? EDIT: Oh, well in that case just ignore this post. You are welcome. It actually wasnt just the curly brackets. You made a typo here: move_uploaded_file($tmp_name, 'user_images/'.$username_entry.'/'. $name); You missed the red one.
-
is the page that displays the retrieved data UTF-8 (unicode) ? Otherwise, set it to UTf-8 in the php header() function and a HTML meta tag. Also, if you are storing that data also make sure that the connection to the database AND the database itself is unicode as well.
-
Try echoing the variables without actually moving the uploaded file. What do you get? <?php echo '<pre>' . print_r($_FILES, true) . '</pre>'; ?> Also, try something like this: move_uploaded_file($tmp_name, "user_images/{$username_entry}/{$name}");
-
Then try building a small website using MVC and an IDE before you take on the big fish
-
Dont use mysql_*. Mysql is deprecated as of 5.3.0 or someting and will not be supported in the future. Use either mysqli() or PDO_mysql(). You can use the exact same queries you would use in PMA. As for getting how many rows were affected, they all have different methods to get that info. Search for it on google, they all have it built in (even mysql()).
-
First, Its not in code tags. Dont include your file, but show relevant code in code tags identified by the "<>". Second, you dont tell us what the issue is, so no one is really going to bother. You cant just come on here and expect us to solve all your problems for you if you are not even trying.
-
Variables initialize everytime I go to a page
DaveyK replied to ady_bavarezu89's topic in PHP Coding Help
You realize PHP has a function that returns the session id? In addition, it is STORED in the current _SESSION... You are overcomplicating things. -
use quotes around the second variable as well? How in the world are you storing thing 0_0