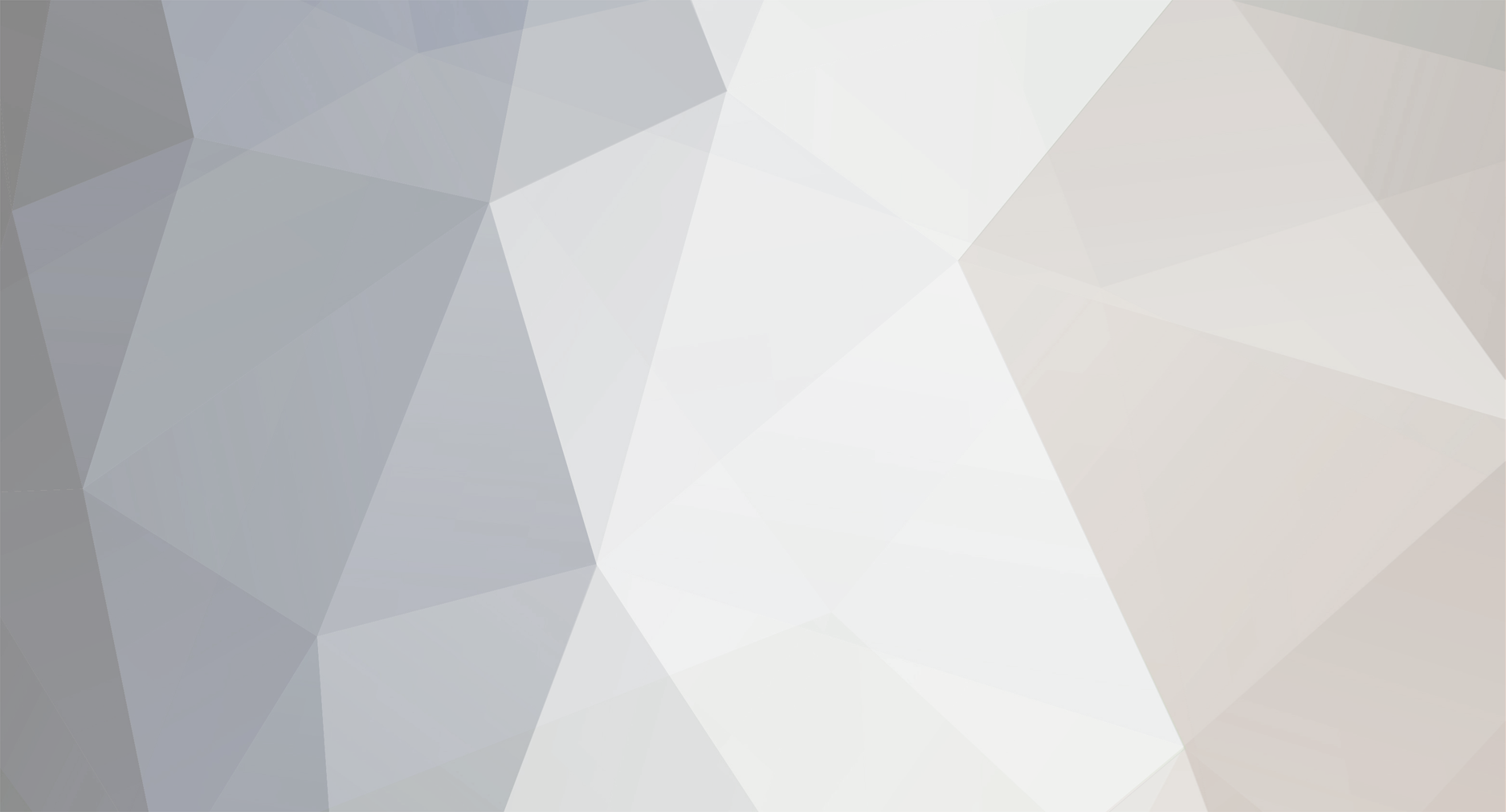
DaveyK
Members-
Posts
289 -
Joined
-
Last visited
-
Days Won
1
Everything posted by DaveyK
-
The issue is very basic, really. <input type="password" size="25" name="user_password" placeholder="Password" /><br /> if (isset($_POST["user_login"]) && isset($_POST["password_login"])) { Find the difference. Some other fundamental notes: 1. The variables you are using are not escaped. Look in mysql_real_escape_string() and prevent mysql injection. 2. You dont have to run a while loop if you only return a single row. 3. Do you really want to store the password in a session? 4. Do you really want to store the password instead of a HASH of the password? 5. You echo something and then you kill the page, without a link. Not a great user experience. 6. I dont know if you are missing it, but make sure you have turned on error_reporting by writing error_reporting(-1); after session_start(). A good way to prevent this in the future is to do something like <?php // Login Script if (isset($_POST["user_login"]) && isset($_POST["password_login"])) { var_dump($_POST); die(); This basically just checks if the variables you enter are correct, without actually doing anything else. Simplify everything as much as possible when debugging, at least you wont be confused. The issue requinix () pointed out was valid too, though.
-
How can I write CSS with PHP? - I tried and i have lost styles
DaveyK replied to jarv's topic in PHP Coding Help
Use Less. It supports variables and you can just compile it on your machine using one of several free less compilers (SimpLess, WinLess, etc.) You can use variables. Its great. -
You say its not working. What if you do a console.log() and then check it in firebug. Also, Im pretty the php needs to return a JSON object in order for the JS to read it
-
Warning: mysql_num_rows() expects parameter 1 to be resource
DaveyK replied to ZeTutorials101's topic in PHP Coding Help
You shouldnt have to include the file in the function as long as its included on the file that calls the function. -
What ist he value of $result['profilepic'] before trim() and what is it after? Use var_dump() please. also, why are you doing this $profile = trim("".$result['profilepic'].""); instead of $profile = trim($result['profilepic']);
-
I'm just gonna use this since it makes most sense to me. I understand that using an ORM could be better but I simply don't have the time to learn that right now. It would take way too long to implement. Thanks for the help guys
-
Okay, I can see that this might be advantageous if you implement it from the beginning, but I am writing an admin panel to a site and I only just started using PDO sql where the normal site uses MySQL. Implementing an ORM like doctrine or propel is a step to far for me. I am only just getting used to PDO and having to learn that ORM as well will set me back way too long. The thing I find interesting in your post tho is that $loadEnrollments=false. What do you do with that variable to alter the sql? This was my original question.
-
I see. I had to google search a bit but I think I get it. At this point I manually write all queries (and columns) I need in seperate functions/methods. I just dont know any better. Regarding the fetchInfo() method question: Yes, information. If that were a function, I would make it return all information, something like: id, user name, email, status, avatar and possibly more info based on the users data. I just simply write an sql query and return the data. Regarding the rest. I am terribly sorry but I just dont follow. First: $user = $em->find('User', 1); print $user->getEmail(); what is $em ?! I think you are assuming I know what this is, while in face I dont have the slighest clue. $from = new User(); $from->setEmail('[email protected]'); $to = new User(); $to->setEmail('[email protected]'); $messageSender->sendMessage($from, $to, 'Je hebt een banaan in je oor!'); Again, I dont understand the use of this. First of all, no one likes a banana in ones ear. Second, you already have the $from variable, so why would you add it to a class and then fetch it from the same class. What am I missing here?! Also, "without needing a database". Do $from and $to just magically appear? Unless you fetch from a form (get, post) or from a session or something, that information is already likely fetched from a database. here is an example of my User.class.php file. class User { private $logged_in_user_id; public function __construct ($user_id = false) { $this->logged_in_user_id = $user_id; } public function fetchInfo ($user_id = false) { if ($user_id === false) { $user_id = $this->logged_in_user_id; } global $pdo; $user_id = intval($user_id); $statement = $pdo->prepare("SELECT `id`, `email`, `status`, `password`, `salt` FROM `users` WHERE `id` = ?"); $statement->execute(array($user_id)); return $statement->fetch(PDO::FETCH_ASSOC); } public function setLoggedInUser ($user_id) { $this->logged_in_user_id = $user_id; } } I assume that its about 100% between "class User {" and "}" is wrong, but please keep in mind that im just doing what I think works and I am coming here because I want to improve my code and expand my knowledge, so please help me...
-
How doesnt it? I need a function/method to return me the info I need to display the user profile I am currently viewing. In normal php, I would just use a fetch_user_info () function. Would you mind clearifying and showing me how it SHOULD be done rather than critizing how it shouldnt be done. I dont really understand the advantage of this. How do you set the user id then. and how do you get the info out of the method if you dont return it?! I dont know OOP and its all just me trying to do some stuff without anyone helping me. Ive never been schooled in PHP OOP so forgive me for not understanding this. EDIT: I replied inside the quote. Not too bright.
-
Hey Freakers, I have a question for you. Its about PHP OOP. Basically, I am rewriting my users class and I have a method to fetch info (surprise). However, the amount or kind of info I require is different for some situations. Sometimes I only need basic info (id, email, avatar) and sometimes I need all of it (id, email, full name, avatar and other relevant information). The question here is, how do YOU guys do this. I always used seperate methods: <?php class Users { public function fetchInfo ($user_id) { // fetch user id, full name, email and other relevant info } public function fetchInfoMin ($user_id) { // fetch a small amount of required info } } ?> This works fine, but I use cases where the amount of info to be fetched is determined by method variables (often constans) and I wonder how that would work. I imagine something like this: <?php class User { const $fetch_all = 'fetch_all'; public function fetchInfo($user_id, $fetch_all = self::FETCH_ALL) { // set the sql based on the $fetch_all variable. } } ?> I wrote that out of the top of my head and frankly I have no idea how that would work, unless just for a lot of if statements changing the SQL and afterwards also the data manipulation on the function itself. How do you guys do this? What is best practise?
-
Change font on forum title with image picture (font picture)?
DaveyK replied to mostone's topic in Javascript Help
You either need to download the fotn and install it to the server or load it from another server (google does that, I think). Or you can use images. -
Best way to start of with Dynamic Web Development?
DaveyK replied to jackhard's topic in Applications
Not sure what it is you seek but that seems solid. I use notepad++ for editing... For SQL you should use the PHP class PDO_mysql() or mysqli() since mysql_* is deprecated as of PHP 5.5.0. Also, HTML5/CSS3/JavaScript are not backend, but front end ! Where do you want to get started? Looks and functionality are both equally important... -
This code would only work if every single user who signed up with the site actually has a PMA accoutn as well... More seriously, think SQL INJECTION. This is not the way to prevent it.
-
The $row variable you are using isn't set anywhere. After result you should do something like $row = mysql_fetch_assoc($result); Also, put code in code brackets, identified by the "<>" icon.
-
Thats not a good way to do it. First off, what would you like to achieve??
-
php mailer will send mail but not include content
DaveyK replied to bumpn's topic in PHP Coding Help
Also, it MIGHT actually send it but your inbox might just now show it. -
Yeah, I would definitely suggest using a COUNT. perform a COUNT on the question_id WHERE category_id = $id.
-
passing and retriving a dropdown box value to another page
DaveyK replied to alanl1's topic in PHP Coding Help
Please put your code in code blocks (identified by the "<>" icon on the top) and seperate them by file. Also, please only show relevant code. Looking at your code real quickly I noticed there is a difference in methods. Both <forms> have method="POST" but you are using $_GET['towerSelect']. might I suggest that you perform most of your PHP (including ESPECIALLY queries) above the HTML. This code is really hard to read. Also, is error reporting turned on? -
You should ask yourself what the kind of security you need. You can do whatever you want, adding hashes and encryptions as many times as you like: but does it add value? I would use the PHP function crypt().
-
Yes, you can do it. You can use a for() loop as you are using now, but I would suggest a foreach() which is much easier. <?php foreach ($_SESSION['foodTypes'] as $food) { echo $food . '<br>'; } ?> Thats basically what gets it done. Also, you STILL need to think about what you want to do with the data the moment you use the submit (the actual submit, not the add). That does not work at all right now. EDIT: if you want to know if the item is taken, and also if you want to make sure you dont have duplicates in your $_SESSION['foodTypes'] array, you need to use the in_array() PHP function. To keep duplicates from being added to the array: (...) else { if (!isset($_SESSION['foodTypes'])) { // if the session is not yet created, create it now $_SESSION['foodTypes'] = array(); } // check to see if the newly added food type is not already in the array if (in_array($_POST['foodType'], $_SESSION['foodType']) === false) { // The selected food item is not in the array // add the selected food item to total food array $_SESSION['foodTypes'][] = $_POST['foodType']; } } (...) Now if you also want to keep the selected food items from showing up in the <select> you need to do a bit more work. - First you need to store the items inside the select in an array. If you fetch them from a database, this is already the case. - Then you should use a foreach loop to display the <option>s (at this point you should have exactly the same HTML as you have right now, but dynamically generated by PHP) - Now for hiding certain items, you need to use the in_array() function to see if the current item in the foreach loop is also in the foodType session variable. If that is true, dont echo anything. If it is false, show the food as an option. I think that pretty much describes it. You need to convert this: <select name="foodType" id="foodType"> <option value="Labneh" selected="selected">Labneh - ????</option> <option value="Cucumber">Cucumber-????</option> <option value="Tomato">Tomato-??????</option> <option value="Lettuce">Lettuce-??</option> <option value="Cucumber Pickles">Cucumber Pickles -???? ????</option> <option value="Olive Oil">Olive Oil- ??? ?????</option> <option value="Cucumber without peal">Cucumber without peal - ????? ?????</option> <option value="Olive Black">Olive Black- ????? ????</option> <option value="Olive Green">Olive Green - ????? ????</option> </select> into something like this: <select name="foodType" id="foodType"> <?php foreach ( .... ) { // do something here } ?> </select> You should be able to make it work from what I described here.
-
If you follow that suggestion, make sure to have a solid system to check when a users premium ends. If you dont check that, they will remain premium for ever. Considering you will probably be storing premium subscriptions in a database, you can use that table to see if the user is still a premium member or not. The first option is easier, but the second is more accurate. Whatever you so desire
-
I know, just not giving any priority to as its more important that the OP first understands the basics of the script before adding conditionals.
-
I have this code so far, and it appears to work fine. <?php session_start(); if (isset($_POST['add'])) { // check if an option has been selected if (empty($_POST['foodType'])) { echo 'You need to select some food!'; } else { if (!isset($_SESSION['foodTypes'])) { // if the session is not yet created, create it now $_SESSION['foodTypes'] = array(); } // add the selected food item to total food array $_SESSION['foodTypes'][] = $_POST['foodType']; } // display the current food list (in a really ugly PHP way) echo '<pre>' . print_r($_SESSION['foodTypes'], true) . '</pre>'; } else if (isset($_POST['submit'])) { // check if the session exists, or if its empty if (!isset($_SESSION['foodTypes']) || empty($_SESSION['foodTypes'])) { echo 'No food in the list to submit!'; } else { // session exists and has content // process the array list here. // after the processing, empty the session $_SESSION['foodTypes'] = array(); } } ?> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Calorie Counter</title> </head> <body> <p>It's Breakfast </p> <p> <form action="" method="post"> <p>Keep on adding items:</p> <p> when you are done, click submit</p> <p> <select name="foodType" id="foodType"> <option value="Labneh" selected="selected">Labneh - ????</option> <option value="Cucumber">Cucumber-????</option> <option value="Tomato">Tomato-??????</option> <option value="Lettuce">Lettuce-??</option> <option value="Cucumber Pickles">Cucumber Pickles -???? ????</option> <option value="Olive Oil">Olive Oil- ??? ?????</option> <option value="Cucumber without peal">Cucumber without peal - ????? ?????</option> <option value="Olive Black">Olive Black- ????? ????</option> <option value="Olive Green">Olive Green - ????? ????</option> </select> <input name="add" type="submit" id="add" value="add"> </p> <input name="submit" type="submit" id="submit" value="Submit"> </form> </p> </body> </html> I somehow lost the special characters, but this works. It allows duplicated in the array and it doesnt do much on submit. How does it work on your end?
-
I get that, I just want to make sure there is already something that does. HOWEVER, since you choose to do it like this, lets roll What you need first a good starting point. This is your script without any backend to it. If you click "add", nothing happens. <?php session_start(); ?> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Calorie Counter</title> </head> <body> <p>It's Breakfast </p> <p> <form action="" method="post"> <p>Keep on adding items:</p> <p> when you are done, click submit</p> <p> <select name="foodType" id="foodType"> <option value="Labneh" selected="selected">Labneh - ????</option> <option value="Cucumber">Cucumber-????</option> <option value="Tomato">Tomato-??????</option> <option value="Lettuce">Lettuce-??</option> <option value="Cucumber Pickles">Cucumber Pickles -???? ????</option> <option value="Olive Oil">Olive Oil- ??? ?????</option> <option value="Cucumber without peal">Cucumber without peal - ????? ?????</option> <option value="Olive Black">Olive Black- ????? ????</option> <option value="Olive Green">Olive Green - ????? ????</option> </select> <input name="add" type="submit" id="add" value="add"> </p> <input name="submit" type="submit" id="submit" value="Submit"> </form> </p> </body> </html> Having a clean starting up is also important. You want two functionalities in the script: - Clicking add generates a list of the products someone has consumed - Clicking submit sends this list to somewhere You want to store this in a session, but first start off with capturing the data: if (isset($_POST['add'])) { if (empty($_POST['foodType'])) { echo 'You need to select some food!'; } else { if (!isset($_SESSION['foodTypes'])) { $_SESSION['foodTypes'] = array(); } $_SESSION['foodTypes'][] = $_POST['foodType']; } echo '<pre>' . print_r($_SESSION['foodTypes'], true) . '</pre>'; } Naturally, since this is processing code this is placed ABOVE the html, underneath the session_start(). If you now select something, the session should grow. EDIT: fixed typos. Sorry.
-
I am just guessing, but what does this do: date_default_timezone_set('Europe/London'); Also, on http://wwp.greenwichmeantime.com/info/current-time/ I found that the UK is on GMT in the winter but on BST in the summer.