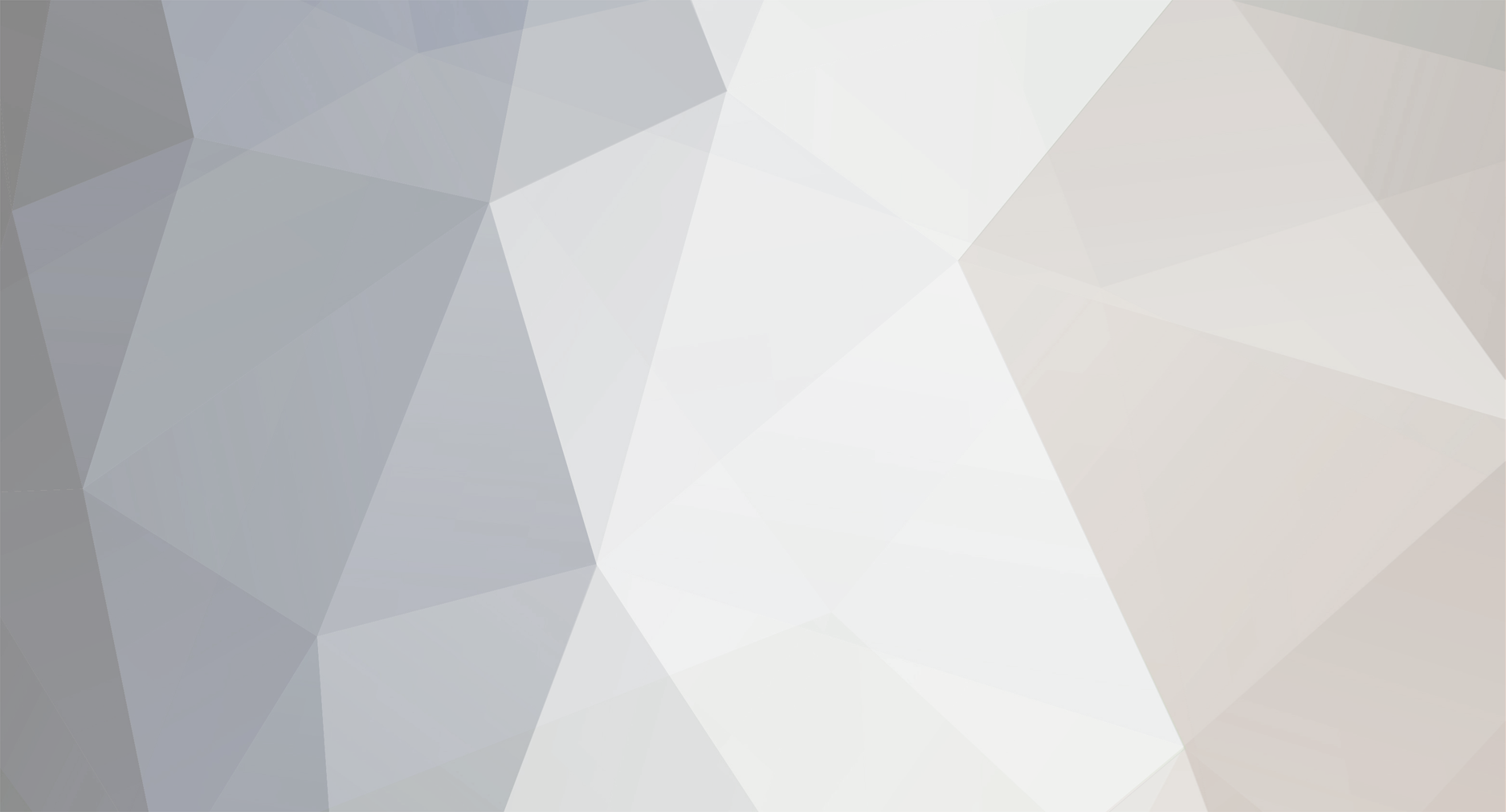
DFulg
Members-
Posts
27 -
Joined
-
Last visited
Everything posted by DFulg
-
Try this
-
how can i give text HTML formatting after a usser subits a form
DFulg replied to Baxt01's topic in PHP Coding Help
Make sure your error_reporting is set to E_ALL or -1 and display_errors is set to 'On' or 1. You should be receiving some kind of error. In any case, add some debugging: $fp = fopen('top100stats.txt', 'r'); //open the file read-only to verify that it exists. if ( $fp === false ) { //cannot open the file echo "Error while attempting to open the file."; } else { // file opened successfully, display message echo '<h2>File exists!</h2>'; } -
Okay, let's take a step back here. As much as I love spaghetti, I don't enjoy trying to read spaghetti code. 1. These two lines $image = new cmsImages; $image->storeFormValues( $_POST ); call the class construct twice. storeFormValues() is not needed, instead it should read. $image = new cmsImages( $_POST ); 2. The image information is stored in $_FILES not $_POST, which is why file_get_contents($_FILES['file']['tmp_name']) holds the correct data. 3. Read my first post in this thread because I state the reasons why your logic is flawed. 4. But since it seems like you don't want to listen to reason and just want an answer, your construct should read something like this: public function __construct( $post_data = array(), $file_data = array() ) { if ( isset( $post_data['img_id'] ) ) $this->img_id = (int) $data['img_id']; if ( isset( $post_data['img_name'] ) ) $this->img_name = preg_replace ( "/[^\.\,\-\_\'\"\@\?\!\:\$ a-zA-Z0-9()]/", "", $data['img_name'] ); if ( isset( $file_data['file'] ) ) $this->img_blob = file_get_contents($file_data['file']['tmp_name']); then you would pass the construct its arguments like so: $image = new cmsImage( $_POST, $_FILES ); I feel so dirty writing this.
-
Change this line: $st->bindValue( ":imageFile", $this->img_blob, PDO::PARAM_LOB); To this: $st->bindValue( ":imageFile", $imageFile, PDO::PARAM_LOB); and it should work fine, although you shouldn't be doing this in the first place. We see your class structure but not the call to the class, show that as well, the problem appears to be the data that you are sending to the class.
-
A couple things I notice: 1. As mentioned, it's generally a bad practice to store blob images inside of a database. Instead, store the images on the server and the image paths in a database. 2. the uploadImage() method should only be concerned with, you guessed it, uploading an image, not establishing a connection to the database and querying data. You should look into the idea of using utility classes to help abstract these tasks.
-
PHPFreaks has a tutorial on basic pagination available: here
-
mkdir("path/to/dir", 0755);
-
Super nested ifs - Better as switches? Multiple logic expressions
DFulg replied to brentman's topic in PHP Coding Help
Include all of the falsy logic in one statement: if ( $number == 5 || $letter == 'a' || $option > 2 ) { //false block } else { // true block } much more readable and logical -
No. include 'connect.php'; include 'main.php'; $id = $_SESSION['id']; $page = "http://localhost/page1.php"; $sql = "UPDATE math SET latestpage=$page WHERE id='$id'"; mysql_query($sql) or die("MYSQL Query Failed : " . mysql_error()); $result3 = mysql_query("SELECT * FROM html WHERE id='$id'") or die("MYSQL Query Failed : " . mysql_error()); while($row3 = mysql_fetch_array($result3)) { $latestpage=$row3['latestpage']; echo $latestpage; Note: mysql is deprecated and will be removed in a future version of PHP. Use mysqli or PDO instead.
-
Where is your debugging code?
-
Make sure that the visibility of __construct() is private not public. The purpose of a singleton in this case is so that one and only one database connection will be established, having a public construct breaks that functionality.
-
Do you have both error_reporting set to E_ALL and display_errors set to 'On'?
-
How to upload multiples images and strore link on database
DFulg replied to carlitoway's topic in PHP Coding Help
If you would like to do this using strictly markup and PHP, I suggest taking a look a this and this. Note that the "multiple" attribute is not supported in IE or Opera. -
I think that this is a good idea, however you have to consider that IE still represents the majority of users and I do not believe that chunking is supported. I also believe that chunking should be considered after the host provider has been consulted since it is more of a bandaid then a solution.
-
I do not believe that chunking is supported by IE and if that is the case, imo that is not a viable option. Your option is also assuming that the OP knows basic Javascript/JQuery. OP, imo you should start by contacting your host provider and see if the issue can be resolved, if it cannot, switch to another provider.
-
Your best bet is to contact your host provider and work with them on this.
-
Typically with a search script, you will only display a certain amount of rows using the LIKE keyword and use pagination to navigate to the next set of rows. $sql = "SELECT field FROM table WHERE field = 'value' LIMIT 0,10"; There is a tutorial on pagination on this site I believe, although I'm not sure exactly where I saw it. Do not use $_SERVER['PHP_SELF'] as a forms action, as this is a security risk because the value can be tampered with. Passing the action attribute an empty string is fine.
-
1. Don't be afraid to use both HTML and PHP in tandem, right now you have spaghetti code. 2. Using the error suppression operator (@) is a bad practice as it promotes sloppy coding without the proper error handling. 3. It's a good practice to store your database connection in a variable for proper error handling instead of invoking it inside of another function. 4. I recommend against using MYSQL as it has been deprecated and will no longer be used in a future version of PHP. I recommend using either MYQSLi or PDO.
-
Error: No input file specified when sending email
DFulg replied to Maher_Nabeel's topic in PHP Coding Help
This error indicates that semail.php either does not exist, or is not in the same directory as the file that contains the HTML form. -
I believe jcbones nailed the issue on the head, the conditional statement when checking if the file exists is incorrect, and really is not needed here since file_put_contents along with the FILE_APPEND flag already checks if the file exists, and appends the data instead of overwriting if it does. The LOCK_EX flag is more of a precautionary flag and should not fix the issue here, however it should be used if you are expecting heavy traffic in that file. I also don't quite understand the hashing logic here, and as jcbones already stated, your code is most likely in a loop and seeing all of the relevant code will help us pinpoint the problem.
-
Again, I urge you to use prepared statements in MYSQLi or PDO instead of MYSQL, which is now deprecated. Also again, never insert user data directly into a SQL statement as this is a security risk. Always properly sanitize data before insertion.
-
My mistake, this will not work unless null is specified upon insert time since PHP treats null values differently then MYSQL. You can however do this using a prepared statement in MYSQLi or PDO which should be what you are using anyway. Below is pseudo code to illustrate an example usage: $stmt = $mysqli->prepare("INSERT INTO table (field1, field2) VALUES (?, ?)"); $stmt->bind_param('ss', $field1, $field2); $field1 = "string1"; $field2 = null; $stmt->execute();
-
So you are purposefully pointing the OP to bad practices? Don't be overly defensive, ignace is just trying to help. I don't think it's the best idea to point a new programmer to OO right away, he probably has no idea what he is looking at in your example. However, OP, trq has pointed you to a good authorization interface that I can think you can manage to implement if you go through the documentation.