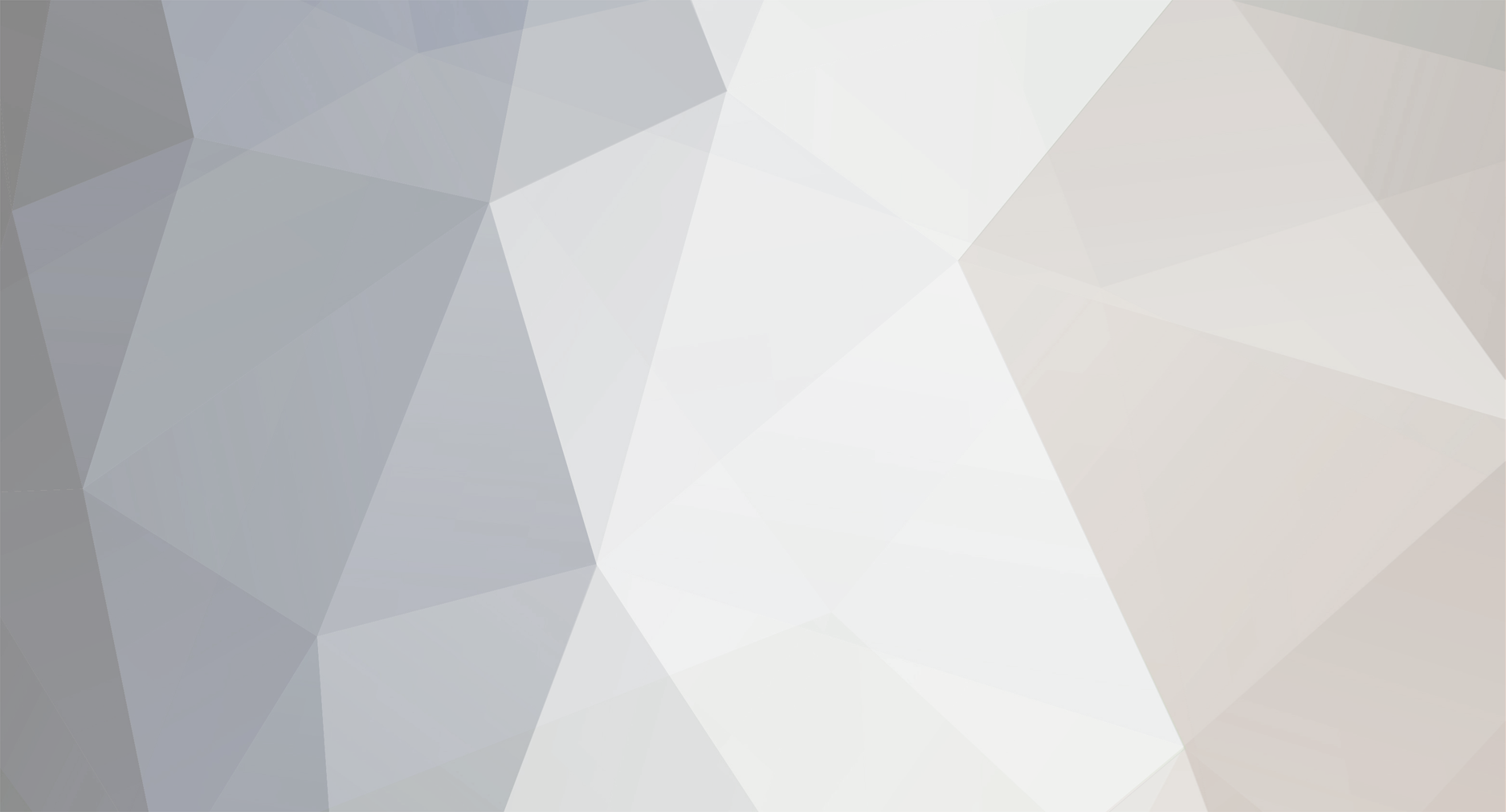
bsmither
Members-
Posts
213 -
Joined
-
Last visited
-
Days Won
3
Everything posted by bsmither
-
/cro1/cRIO01_live.csv (error entry) still does not match /crio1/cRIO01_csv (code) Might there be an issue with PASV?
-
$Post Multiple Variable from a Single <option>
bsmither replied to jperez260's topic in PHP Coding Help
A somewhat harder way, that is, if you want all the desired data to come in on the form submit, is to concatenate everything into one string: $sender_name . "|" . $sender_email to populate the HTML, then explode("|", $_POST)- 5 replies
-
- variable
- multiple variables
-
(and 1 more)
Tagged with:
-
In my experience: Module 'gd' already loaded in Unknown on line 0 means that PHP has attempted to load this module from either the PHP.INI file (the GD or GD2 extension, although the error message might be little different on startup errors) or from a ZendGuard or ionCube loader file that might be using the dl() command. The fact that there is no known file or line number means (IMO) that PHP wasn't running a human-readable script. I think the version of PHP may be prior to 5.3, and this is only a warning. PHP won't crash or exit because something is asking PHP to load this module when it is already loaded. So, I think your time outs are not caused by this situation.
-
It seems you are using PHP's "short open" tags. Your PHP configuration file must specifically allow for that. If it does not, then: <? stuff ?> will be ignored by PHP. It will also be ignored by the browser because angles denote an HTML tag, not content to be shown. But since a question mark is not a proper name of an HTML tag, the browser won't know what to do with it, except to ignore it. Thus, but phrases get displayed. For now, try to always use the normal PHP open tag: <?php stuff ?>
-
This: http://www.sparklenshinecs.com/index.php?content=paginas&cat=7 is a URL encoded variant of this: http://www.sparklenshinecs.com/index.php?content=paginas&cat=7 The character & is encoded to its html entity equivalent & Technically, when a script wants to paste a URL into the HTML output, such as the value of a text type form element, validation will agree with the encoded variant. Note that the browser will display all the HTML entities as their single character version. So, I think whatever script is creating these URLs, it is mistaking the intended place in the HTML: actual links, such as for images, or informational areas. Or, whatever is scanning your site for SEO-ness, is not discriminating between the two purposes. When PHP splits the querystring into the GET array, there will be $_GET['amp;cat'] that equals '7'. When your script tries to use $_GET'cat'], which is not set, your query will fail. Not having tested for the response from the database for a possible bad query - a boolean false (you are probably assuming the result from the mysql_query() call will always be a valid resource), the mysql_fetch_array() call will throw an error.
-
2 arrays with simplexml load string failing
bsmither replied to NetMonkey's topic in Third Party Scripts
Feel free to ask specific questions. "It don't work" is not specific. "If I lose line 42, the first array should be fine." Yes. And also for line 43. Odds are good this array will get populated somewhere earlier in the script. You don't want to empty it. -
2 arrays with simplexml load string failing
bsmither replied to NetMonkey's topic in Third Party Scripts
Line 6 assigns a populated array to $query_vals. Line 42 assigns an empty array to $query_vals. Line 18 starts a function but you are not calling it. Line 44 is a complete statement, but is followed by a brace that usually groups statements to be executed via a program flow construct. Line 45,46 are program flow constructs with nothing to do. Line 49 is the closing brace to line 44, but line 44 is not a program flow construct. -
Assuming you get the user/pass fixed: WHERE user_username = $username Please consider using apostrophes to surround string values. WHERE user_username = '$username'
-
Make absolutely sure you have the <tr>, <td>, </td>, and </tr> sequence correct. Also, browsers know to start another row when the sequence </tr><tr> is found. A few browsers (some versions of IE in particular) will collect all extraneous HTML that should not be between the table parts close/open tag sequences, and show them above the table. Thus, all the <br/> tags, being outside of cells, will be collected and 'displayed' above the table.
-
$Username = safe($_POST['Username'] . ""); $Username = safe($_POST['Username']) . ""; Either statement. Let's see what happens.
-
var_dump() gives you nothing...? If the unlikely result of var_dump() gives nothing if $Username is not set, please prepare the variable by doing this: $Username = $_POST['Username'] . ""; This will force $Username to equal an empty string if the POST variable is not set.
-
It looks like you have inadvertently incorporated a BASIC string function in the key expression for $row. So, assuming what you get with $row['fm_auction'] is something like auction2013 and you just want the 2013 part, try: echo substr($row['fm_auction'],-4);
-
Yes, something like that. Since you are echoing stuff to the browser, let's try: var_dump($Username); if ($Username == ""){ Let's let PHP tell us what it thinks of $Username.
-
Can we assume the statement: if ($Username == ""){ has $Username holding the sanitized value of $_POST['Username']? And etc.?
-
Yes, and it may be complicated to parse the results. Using cURL (or if that fails, fsockopen), you can have PHP issue a request to another site. Typically it is to one of that site's API methods where the return is no more, no less, than what you exactly need. But if you get back an entire web document, your PHP will need to parse out the data you want (called 'scraping' a page).
-
It is. I was not able to make a number of adjustments to my profile until after some time or number of posts had passed.
-
Let's start with $a being what we start with, $b being what we want. $b = array(); foreach ($a as $value) { $b[$value['sno']] = $value['result']; } print_r($b);
-
"mysql_fetch_array() expects parameter 1 to be resource, boolean given" Without looking at your code, in my experience, 99% of the time the mistake is assuming that the database server will always return a recordset for any given query. A good programmer must always code for the possibility that the database server will return false (which is a boolean value). So, look very carefully at your query on line 41 and make sure you think it should return some records. As of now, it isn't. It's returning false. Something is wrong with the query. The other 1% is from other sloppy coding mistakes.
-
You tried unset() specifically how? Was: foreach($a as $key => $value) { print_r($key = $value); echo "<br/>"; } Now try: foreach($a as $key => $value) { unset($a[$key]['num1'], $a[$key]['num2']); print_r($a[$key]); echo "<br/>"; } According to the PHP manual for foreach(), $value is a copy from $a. In order to change $value that is actually part of $a, you would need: foreach($a as $key => &$value)
-
::::---- Problem with login php mysql script ----::::
bsmither replied to MachineGamer's topic in PHP Coding Help
This part of the code: "<p color='#ff0000' style='margin-right: 10px;'".$login_error."</p>" does not have the right-angle to close the <p> tag. Try: "<p color='#ff0000' style='margin-right: 10px;'>".$login_error."</p>" -
Encode table in a class or use a database?
bsmither replied to dennis-fedco's topic in PHP Coding Help
Are you expecting there to be more to the class than just this array of values? If not, a simpler lookup table function would suffice. I am not seeing any kind of established pattern, so creating a math function would not work. Therefore, hard-coding the lookup table is probably best: $result = array( 'A1' => 4, 'B1' => null, 'C1' => null, 'A2' => 5, 'B2' => 5, 'C2' => null, 'A3' => 6, 'B3' => 7, 'C3' => 9, ... 'A12' => 7, 'B12' => 8, 'C12' => 11, 'A13' => 8, 'B13' => 9, 'C13' => 13, ); return $result[trim((string)$col) . trim((string)$row)] + $x;