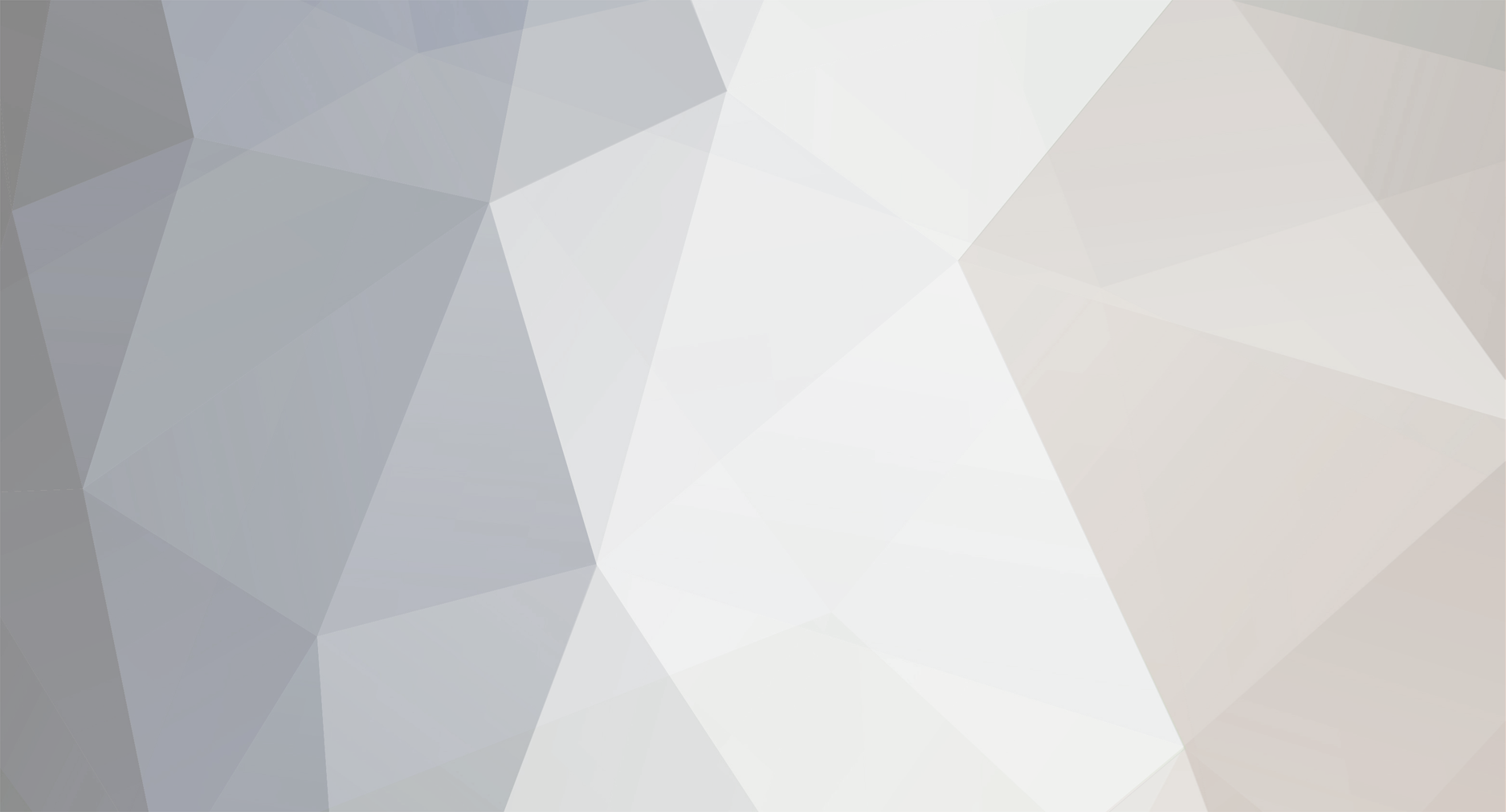
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
Changing a 'save as' dialog to save to server? [Code Inside]
wildteen88 replied to TRemmie's topic in PHP Coding Help
I assume the $pdf variable holds the data to be added to the .pdf file in which case you'd use file_put_contents function to create the .pdf file on your server. You wont need to use header to set the content type of the file. Example: // setup the path for the pdf file to be created $pdf_file_path = $_SERVER['DOCUMENT_ROOT'].'/pdfproject/'.$name; // check that the file doesn't exists first before creating it if(!file_exists($pdf_file_path)) { // file doesn't exists, so create the file. file_put_contents($pdf_file_path, $pdf); } -
Open your httpd.conf file and find the following lines: # First, we configure the "default" to be a very restrictive set of # features. # <Directory /> Options FollowSymLinks AllowOverride None Order deny,allow Deny from all </Directory> Add the following line before the </Directory> line: Allow from 127.0.0.1 localhost Now scroll down a bit further and comment out (add a # at the start of the line) the following lines highlighted in bold: Save the httpd.conf and restart Apache Apache will only accept connections from you. It'll reject any other users (remote computers)
-
You should use $_GET not $_GET_VARS
-
How to pass a value to multiple PHP programs?
wildteen88 replied to jr8rdt's topic in PHP Coding Help
You should use $_GET['id_chart'] You'd use this var in a.php b.php c.php and d.php You wont be able to retrieve data you pass to a.php from another page. -
When echo'ing/print'ing variable you do not need to use quotes or parentheses. Either of the following is fine: <?php echo $var; // no need to use quotes when echo'ing a variable on its own echo "<b>$var</b>"; // variables are parsed in strings which start with double quotes. echo '<b>'. $var . '</b>'; // variables are not parsed in strings which start with single quotes. Instead you can use concatenation. ?> NOTE: echo and print are the same. print was derived from Perl
-
HELP! - Is it possible through .htaccess
wildteen88 replied to prakash's topic in Apache HTTP Server
I do not understand what you are trying to do? The .htaccess file is used to override server side settings which will apply for your site only. You cannot use a .htaccess file to modify code already within a html file. -
HELP! - Is it possible through .htaccess
wildteen88 replied to prakash's topic in Apache HTTP Server
You cannot affect other sites with a .htaccess file. Any settings in the .htaccess will affect your site only. -
You are limiting the query to return only 1 result. This is why you are getting only one result. Remove LIMIT 1 from the query.
-
I was close just needed to tweak the query. try: <?php $WalkNo = $_GET['WalkNo']; $query = "SELECT w.WalkerNo, m.MemberRef, m.Forname, m.Surename, w.DateJoined FROM members m, walker w WHERE w.MemberRef=m.MemberRef AND w.WalkerNo='$WalkNo'"; $result = mysql_query($query) or die('Query error!<br />Error:' . mysql_error() . 'Query: <pre>' . $query . '</pre>'); if(mysql_num_rows($result) == 0) { print "No Participants exist"; } else { ?> <table class="join" cellspacing="0" width="861"> <tr> <td class="Header">WalkNo</td> <td class="Header">Member Ref</td> <td class="Header">Forename</td> <td class="Header">Surname</td> <td class="Header">DateJoined</td> </tr> <?php while ($account = @mysql_fetch_assoc($result)) { ?> <tr> <td class="Body"><?php echo $account["WalkNo"]; ?></td> <td class="Body"><?php echo $account["MemberRef"]; ?></td> <td class="Body"><?php echo $account["Forename"]; ?></td> <td class="Body"><?php echo $account["Surname"]; ?></td> <td class="Body"><?php echo $account["DateJoined"]; ?></td> </tr> <?php } ?> </table> <?php } ?>
-
Id' change this: function getProperty($key){ if(!empty($this->properties)){ return $this->properties[$key]; // <-- line giving the notice } } to: function getProperty($key) { if(is_array($this->properties) && isset($this->properties[$key])) { return $this->properties[$key]; } }[/code Do note that the $_REQUEST superglobal contains both $_POST, $_GET and $_COOKIE data.
-
Use: <?php include'connection.php'; if(isset($_GET['bin_data']) && !empty($_GET['bin_data'])) { $file = $_GET['bin_data']; echo "<p>Deleted $file<br />\n" . 'You\'ll be redirected to Home Page after (4) Seconds<p>'; echo '<meta http-equiv="Refresh" content="4;url=/marlon/photo/thumbs/delete_pic_tn.php?bin_data=' . $info['bin_data'] . '">'; } else { echo "Error deleting $file"; } ?> When using variables in strings use concatenation. Also to use double quotes in strings which start with double quotes escape them, ge: echo "A string with \"double quotes\" in"; // OR echo 'A string with \'single quotes\' in'; // Another example echo "A string with 'single quotes' and \"double quotes\" in"; Notice the pattern?
-
The following line has incorrect syntax: if ($_POST['first'] == !"" && $_POST['date1'] == !"" && $_POST['date2'] == !"") you should use != rather than == ! Also you dont have a field called first in your form. I believe you meant $_POST['userid'] rather than $_POST['first']. Corrected code: if ($_POST['userid'] != "" && $_POST['date1'] != "" && $_POST['date2'] != "")
-
As I said earlier it'll be easier to use JOIN's. With a JOIN you can query multiple's tables at once. Can you post your table structure for the members and walkers table here and tell me what fields you want to retrieve from the two tables. EDIT: Try the following code instead. I had a guess at it: <?php $WalkNo = $_GET['WalkNo']; $query = "SELECT w.WalkerNo, m.MemberRef, m.Forname, m.Surename, m.DateJoined FROM members m, walker w WHERE m.MemberRef=w.MemberRef AND w.WalkerNo='$WalkNo'"; $result = mysql_query($query) or die('Query error!<br />Error:' . mysql_error() . 'Query: <pre>' . $query . '</pre>'); if(mysql_num_rows($result) == 0) { print "No Participants exist"; } else { ?> <table class="join" cellspacing="0" width="861"> <tr> <td class="Header">WalkNo</td> <td class="Header">Member Ref</td> <td class="Header">Forename</td> <td class="Header">Surname</td> <td class="Header">DateJoined</td> </tr> <?php while ($account = @ysql_fetch_assoc($result)) { ?> <tr> <td class="Body"><?php echo $account["WalkNo"]; ?></td> <td class="Body"><?php echo $account["MemberRef"]; ?></td> <td class="Body"><?php echo $account["Forename"]; ?></td> <td class="Body"><?php echo $account["Surname"]; ?></td> <td class="Body"><?php echo $account["DateJoined"]; ?></td> </tr> <?php } ?> </table> <?php } ?>
-
Using mysql_result is a very long winded way of getting results (IMO). By using Thorpe's code you can do away with mysql_result and just use the $row array to access your results. $query = 'SELECT * FROM table'; $result = mysql_query($query); while ($row = mysql_fetch_assoc($result)) { // display contents of the $row array echo '<pre>' . print_r($row, true) . '</pre>'; } To access a field from the row you'd use $row['field_name_here'] allows for much cleaner code.
-
Change AllowOverride None in the following block in the httpd.conf to AllowOverride All # # AllowOverride controls what directives may be placed in .htaccess files. # It can be "All", "None", or any combination of the keywords: # Options FileInfo AuthConfig Limit # AllowOverride None Save the httpd.conf and restart WAMP server. Type you Mod_rewrite rules in an .htaccess file How to create an .htaccess file on Windows Open Notepad go to File > Save As. Navigate to C:\wamp\www in the Save In drop down menu. In the Filename box type .htaccess and select All Files from the File Type menu. Click Save and a .htaccess file is now created.
-
Thorpe's code should work fine? Sounds like you're using the mysql_result function.
-
try: <?php $dir = "./files/"; /*$find["/*"] = "<noedit>/*";*/ $find["\" = \""] = "\" = \"</NOEDIT><TRAD>"; $find["\"=\""] = "\"=\"</NOEDIT><TRAD>"; $find["\";"] = "</TRAD><NOEDIT>\";"; // Open a known directory, and proceed to read its contents if (is_dir($dir)) { if ($dh = opendir($dir)) { while (($filename = readdir($dh)) !== false) { if(preg_match('/\.strings$/sm', $filename )) // check if the file ends with .strings { $file = $dir . '/' . $filename; $str = file_get_contents($file); // reads entire file into a string $str = str_replace(array_keys($find), array_values($find), $str ); $str = "<OPEN><NOEDIT>\n" . $str . "\n</NOEDIT></OPEN>"; file_put_contents($file.'.html', $str); // adds .html to the filename } } closedir($dh); } } ?> I remove the need to have to following lines: // opens file to write to it $handle1 = fopen($file.".html", 'r+'); $opentags = "<OPEN><NOEDIT>\n"; fwrite($handle1, $opentags); // adds opening tags to the beginning of the file // opens file to write to it $handle2 = fopen($file.".html", 'a'); $closetags = "</NOEDIT></OPEN>"; fwrite($handle2, $closetags); // adds closing tags to the end of the file
-
If you host your database on your home computer it'll mean you computer will need to be on 24/7 and your site may become slow when doing SQL queries. I'd recommend looking for a new host which provides atleast as database as part of the basic hosting plan. If you still want to to host your MySQL database from your computer then you'll need to have a static ip address, ensure you enable port forwarding for port 3306 on your router (if you have one). Allow for remote connections on port 3306 in your firewall. Add your hosts IP address to MySQLs permissions table.
-
Look into using JOIN's
-
Then you'll need use a bit of javascript to submit the form once a user has selected an item from the list <form method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>"> <select name="selectElem" onChange="this.form.submit()"> <option>Filling</option> <option value="ham">Ham</option> <option value="cheese">Cheese</option> <option value="hamcheese">Ham and Cheese</option> </select> </form> <?php if(isset($_POST['selectElem'])) { echo $_POST['selectElem']; } ?>
-
Read my post above.
-
Is that all your code? If it is there is a few issues: 1. There is no submit button. In order for PHP to retrieve data from the form it needs to be submitted. 2. Your form method is set to post so you'll use the $_POST superglobal instead, eg $_POST['selectElemId'] 3. You should always check to see if user defined variables exists first before using them to prevent the "Underfined index notice" notices (these are not errors)
-
This has nothing to do with your php.ini htmlspecialchars_decode is a core PHP function. There is not an extension/setting in the php.ini to enable in order for PHP to recognise this function. Check that your PHP version is 5.1.0 or greater by running the phpinfo() function in a script. htmlspecialchars_decode() is only available from PHP5.1.0 or greater.
-
Make sure you are calling the function after you have defined it. It may be helpful if you provide some code too.
-
It would be helpful if you clearly state what you are trying to do. You say you have installed WAMP, I presume on your computer. What is the reason from having a remote website and using a MySQL on your local computer as the database?