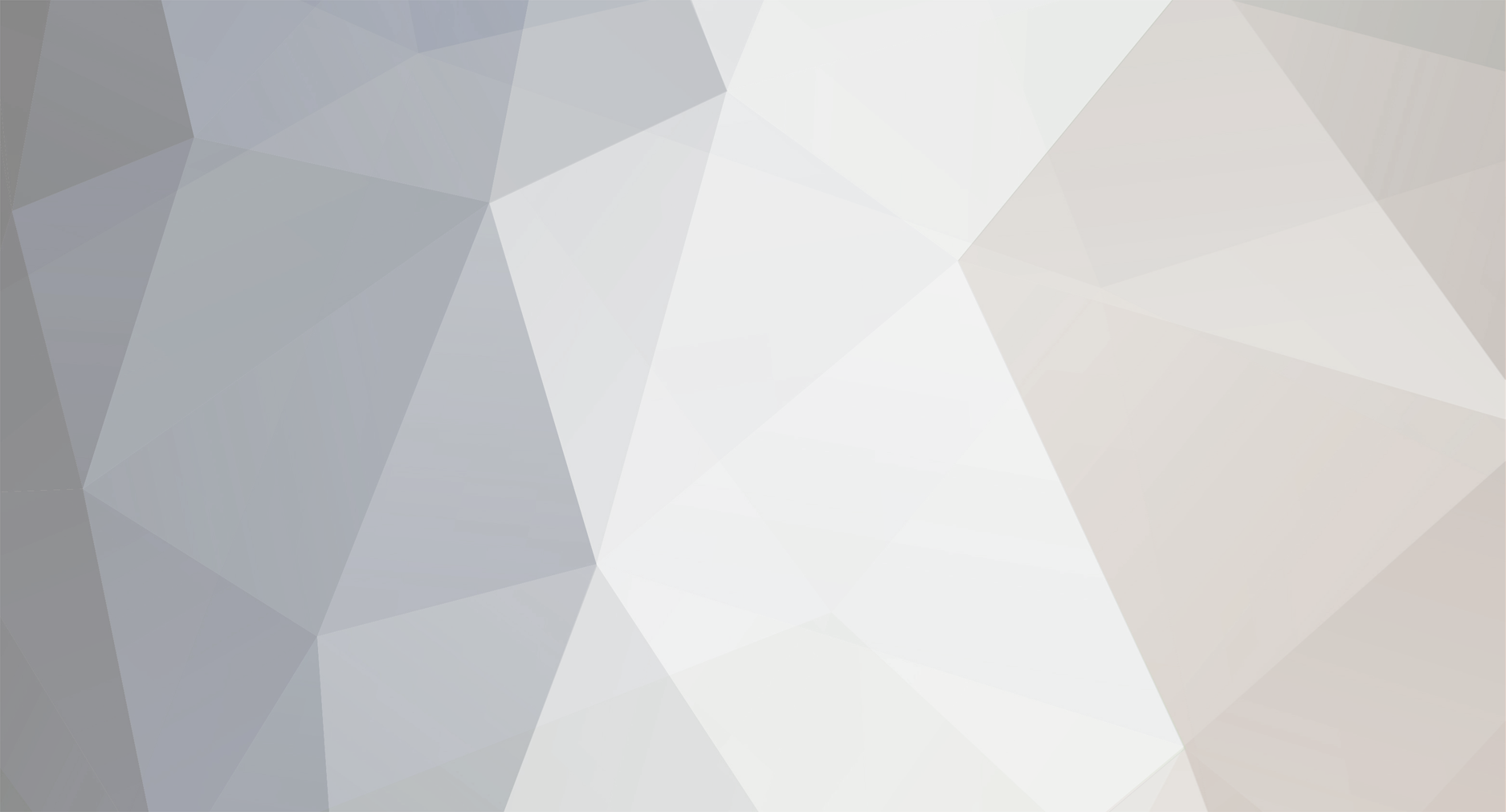
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
cooldude wow! Your script is over complicated. Your code can be simplified down to just: <?php $file1_words = file('file1.txt'); $file2_words = file('file2.txt'); echo '<h2>File1 Words</h2>'; foreach($file1_words as $word) { if(!in_array($word, $file2_words)) { echo '<b>' . $word . "</b> (<i>match</i>)<br />\n"; } else { echo $word . "<br />\n"; } } echo '<h2>File2 Words</h2>'; foreach($file2_words as $word) { if(!in_array($word, $file1_words)) { echo '<b>' . $word . "</b> (<i>match</i>)<br />\n"; } else { echo $word . "<br />\n"; } } ?>
-
Try the following instead: <?php $fields = array( 'txtFirstName', 'surname', 'txtAddress1', 'txtAddress2', 'postcode', 'txtCountry', 'txtTelephone', 'txtMobile', 'txtEmail', 'txtproduct', 'brochure', 'radContactby' ); $errors = null; foreach($fields as $field) { if(!isset($_POST[$field]) || empty($_POST[$field])) { $errors++; } } if($errors > 0 && !is_null($errors)) { echo '<center>Sorry, all the fields must be filled out.</center>'; } else { $txtFirstName = $_POST["txtFirstName"]; $surname = $_POST["surname"]; $txtAddress1 = $_POST["txtAddress1"]; $txtAddress2 = $_POST["txtAddress2"]; $postcode = $_POST["postcode"]; $txtCountry = $_POST["txtCountry"]; $txtTelephone = $_POST["txtTelephone"]; $txtMobile = $_POST["txtMobile"]; $txtEmail = $_POST["txtEmail"]; $txtproduct = $_POST["txtproduct"]; $brochure = $_POST["brochure"]; $radContactby = $_POST["radContactby"]; // rest of your code here } ?>
-
To display an array you'll want to use print_r: $array = array($nsubject); echo '<pre>' . print_r($array, true) . '</pre>';
-
Check against the $_POST variables themselves. Eg: if(!empty($_POST["txtFirstName"]) || !empty($_POST["surname"]) || !empty($_POST["txtAddress1"]) || !empty($_POST["txtAddress2"]) || !empty($_POST["postcode"]) || !empty($_POST["txtCountry"]) || !empty($_POST["txtTelephone"]) || !empty($_POST["txtMobile"]) || !empty($_POST["txtEmail"]) || !empty($_POST["txtproduct"]) || !empty($_POST["brochure"]) || !empty($_POST["radContactby"])) { $txtFirstName=$_POST["txtFirstName"]; $surname=$_POST["surname"]; $txtAddress1=$_POST["txtAddress1"]; $txtAddress2=$_POST["txtAddress2"]; $postcode=$_POST["postcode"]; $txtCountry=$_POST["txtCountry"]; $txtTelephone=$_POST["txtTelephone"]; $txtMobile=$_POST["txtMobile"]; $txtEmail=$_POST["txtEmail"]; $txtproduct=$_POST["txtproduct"]; $brochure=$_POST["brochure"]; $radContactby=$_POST["radContactby"];
-
use htmlentities: <?php echo htmlentities('<?php echo "This is what i\'m trying to do!"; ?>'); ?>
-
What editor are you using? Have you tried echo'ing $file. Just because your editor doesn't highlight the function doesn't mean it exists. I guess you are using Dreamweaver or something. Dreamweaver is not a proper PHP editor so dont trust any feed back it gives. You are always better of running your code through PHP to see if something works or not.
-
file_get_contents does exist! What version of PHP do you have? file_get_contents is available from PHP4.3.x. If you have a version earlier than this version you're better of upgrading PHP.
-
[SOLVED] About the RATING in [phpfreaks.com]
wildteen88 replied to watthehell's topic in Miscellaneous
The rank you get is related to your post count. Read this sticky to see how many posts you'll need to get to the next rank. -
[SOLVED] Defieing Variables [Unsolved][Help]
wildteen88 replied to chizley's topic in PHP Coding Help
Use == on your expressions and not a single equal singe. == means comparison = means asignment if($noa = Description){ should be if($noa == 'Description'){ -
Use explode on the string that contains the numbers. $string = '12.56.79.35.93.22'; $num = explode('.', $string); echo $num[0]; // 12 echo $num[1]; // 56 echo $num[2]; // 79
-
Your code is not correct. Try: <form action="#" method="post"> Package: <select size="1" name="package"> <?php $A = $B = null; if(isset($_POST['submit'])) { switch ($_POST['package']) { case '50': $A = ' selected="selected"'; break; case '75': $B = ' selected="selected"'; break; } } ?> <option value="50"<?php echo $A; ?>>Premium Package RM50 (with RMXX talktime)</option> <option value="75"<?php echo $B; ?>>Premium Package RM75 (with RMXX talktime)</option> </select><br /> <input type="submit" name="submit" value="Submit" /> </form>
-
A good example is always the PHP manual. Can't go wrong there.
-
[SOLVED] GARGH! function defies all logic!
wildteen88 replied to dj-kenpo's topic in PHP Coding Help
Place: print "dd2-$orig_filename - $new_name -$count<br />"; print "2- will return $count<br />"; Within your else statement. If its outside the else it will be executed every time the function gets called. <?php function file_name_int_increment($orig_filename, $new_name,$array, $count) { print "<p>if exists-$new_name $count "; if(array_key_exists ($new_name, $array )) { echo 'TRUE<br />'; $count++; $new_name = $orig_filename.'['.$count.']'; print "file_name_int_increment($orig_filename, $new_name, $array, $count);</p>"; $count = file_name_int_increment($orig_filename, $new_name, $array, $count); } else { echo "FALSE<br />$orig_filename - $new_name - $count<br />will return $count</p>"; } return $count; } ?> -
disk_total_space does not calulate a size a of a directory. It only calculates the size of the hard drive or a partition within a drive. private_guy I wrote this function a while ago: <?php function folder_size($path, $folder=null) { if(!isset($dir_size)) { $dir_size = 0; } $folder_path = $path . $folder . '/'; $handle = opendir($folder_path); $ignore = array('.', '..'); while ( false !== ($file = readdir($handle))) { if (!in_array($file, $ignore)) { if(!is_dir($folder_path . '/' . $file)) { $dir_size += filesize($folder_path . '/' . $file); } elseif(is_dir($folder_path . '/' . $file)) { $dir_size += folder_size($folder_path, $file); } } } closedir($handle); return $dir_size; } $folder_size = folder_size('./file'); echo 'Total folder size = ' . number_format(($folder_size/1024/1024), 2) . ' MB'; ?> This will calculate the file size of a given directory.
-
charlie it'll display fine for you as you have a bigger screen resoulution than isaac_cm, looks at issac's screenshots they are using a screen res of 800 x 600. That is what I mean by the text.
-
[SOLVED] How many minutes till next hour?
wildteen88 replied to AbydosGater's topic in PHP Coding Help
Its called a ternary operator . Its an inline version of an if/else statement. The quoted line you posted above basically means this: if($cur_min == 59) { $mins_left = 0; // true } else { $mins_left = 60 - $cur_min // false } format of the ternary operator: (expression) ? TRUE : FALSE -
[SOLVED] I always get this error, I cant find the problem
wildteen88 replied to chmpdog's topic in PHP Coding Help
You had a load of curly braces missing in your code for the load_content function. Also I noticed you had defined a function called header. You cannot redefine a function already used by PHP. I renamed your header function to page_header instead. <?php function load_content() { if (isset($_GET['task'])) { switch($_GET['task']) { case 'login': include 'login.php'; break; case 'mostplayed': include 'mostplayed.php'; break; default: echo 'homepage'; break; } } else { // HOMEPAGE echo 'homepage'; } } function title() { include 'config.php'; if(isset($_GET['task']) && $_GET['task'] == 'login') { echo 'Login'; } elseif(isset($_GET['task']) && $_GET['task'] == 'register') { echo 'Register'; } } function navi() { include 'includes/nav.html'; } function page_header() { include 'includes/header.html'; } function page_footer() { include 'includes/footer.html'; } function css() { include 'include/cssjava.html'; } ?> -
Umm, this is completely incorrect: mysql_pconnect("$ mysql -u 112489 -h mysql.ict.school.edu.au -p 112489db") mysql_pconnect requires three parameters hostname, username and password in that order. Not a command line. This is what the above line should be: mysql_pconnect("mysql.ict.school.edu.au", "112489", "112489db")
-
[SOLVED] How many minutes till next hour?
wildteen88 replied to AbydosGater's topic in PHP Coding Help
Use date to get the current minutes and seconds. Then you can take the current minute away from 60 to get the remaining minutes, you can do the same with the seconds too. Heres an example. <?php // get the current minute and second using date $date = date("i:s"); // explode against the semi-colon to get the minute and seconds separately list($cur_min, $cur_sec) = explode(':', $date); // calculate remaining minutes and secounds $mins_left = ($cur_min == 59) ? 0 : 60 - $cur_min; $secs_left = 60 - $cur_sec; // echo the time remaining sentance echo 'There is ' . ($mins_left) . ' minutes and ' . $secs_left . ' seconds left until the next hour'; ?> -
its the text (Description Here) that is causing your input boxes to be spaced a part more than you want.
-
Looks like php is having a problem while reading the configuration in the php.ini. Go into the php.ini and enable a setting called display_startup_errors, by changing its default value Off to On. Save the php.ini try to startup Apache again. This time you should see error prompts being displayed if PHP is having a problem when loading.
-
Because you are using empty <td></td> tags. Your web browser will ignore empty table cells. What you should so is remove all empty cell tags and use the following CSS for your table (main_table class): .main_table { width: 50%; height: 100%; margin: 0 auto; /* Center table in middle of page, no need for empty table cells */ } Remove width: 50%; for .content. As for vertical alignment with css. There are many articles out there that show you have to, Heres one and many others
-
[SOLVED] GARGH! function defies all logic!
wildteen88 replied to dj-kenpo's topic in PHP Coding Help
You are not catching the return value when you call a new instance of file_name_int_increment within the if statement. Change this line: file_name_int_increment($orig_filename, $new_name, $array, $count); to: $count = file_name_int_increment($orig_filename, $new_name, $array, $count); -
[SOLVED] PHP not installing hmph
wildteen88 replied to Lokolo's topic in PHP Installation and Configuration
uninstall PHP from the installer. Then just download the zip binaries package from php.net rather than using the installer. Extract the contents of the zip to C:/php. Open up Apaches configuration file (httpd.conf) default path is something like C:/Program Files/Apache Foundation/Apache2.2/conf Add the following lines: # PHP5 LoadModule php5_module "C:/php/php5apache2_2.dll" PHPIniDir "C:/php" After the following line: #LoadModule ssl_module modules/mod_ssl.so Then scroll down and find the following line: AddType application/x-gzip .gz .tgz And then add the following after the above line: # PHP5 AddType application/x-httpd-php .php Save the httpd.conf and restart Apache. Open up notepad and type the following: <?php phpinfo(); ?> Save the following as test.php and save it to the following path: C:/Program Files/Apache Foundation/Apache2.2/htdocs (note: you can delete all existing files in this folder if you wish). Open up your web browser and go to http://localhost/test.php you should now see a page showing information about PHP. Apache is now configured to parse PHP scripts.