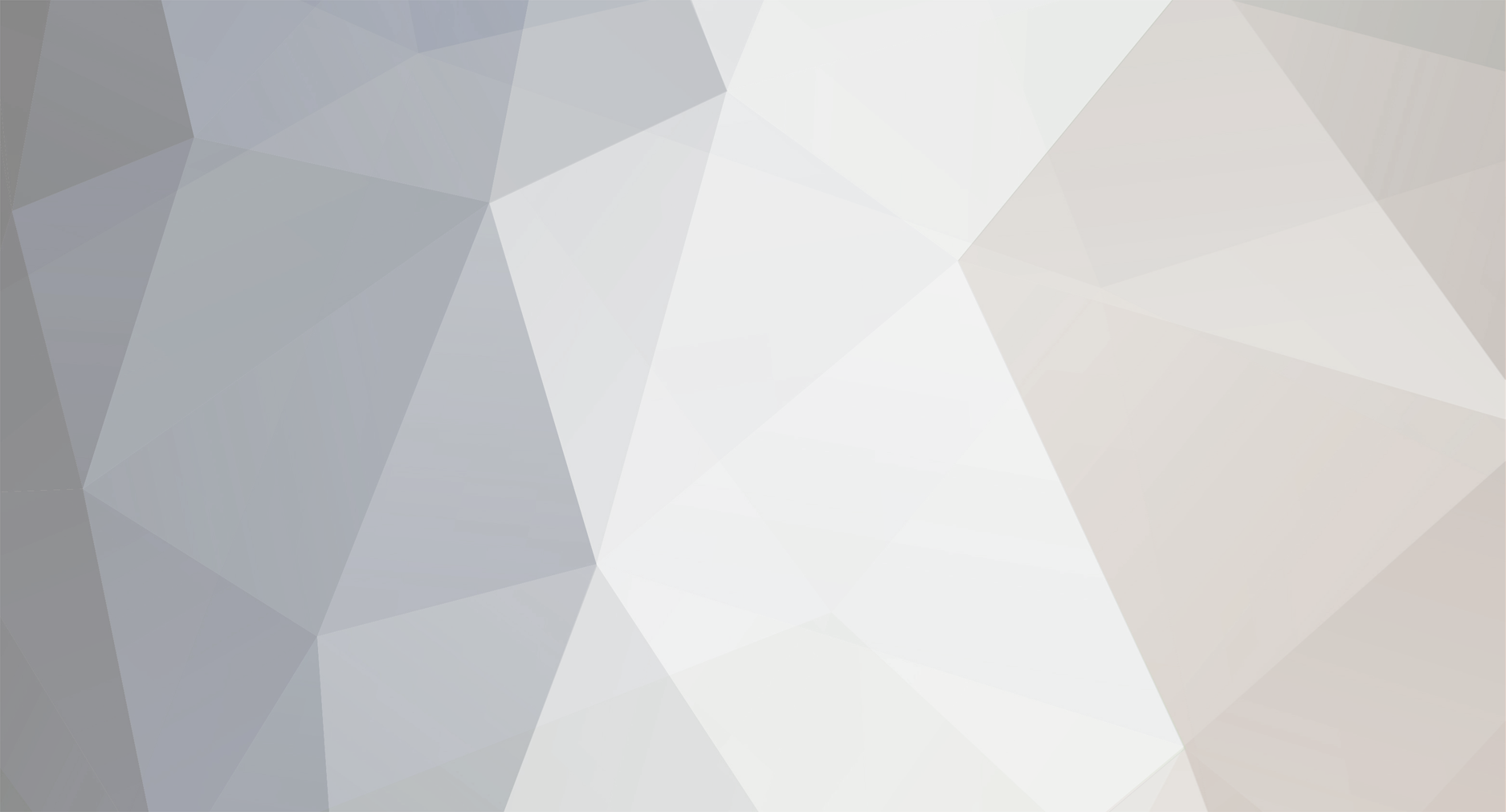
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
If you only want the hour and minutes displayed you can just drop of the seconds part like so: $time = '12:45:00'; list($hours, $minutes, $seconds) = explode(':', $time); echo $hours . ':' . $minutes; // 12:45
-
How are you echo'ing the constant? Make sure you don't wrap the constant in quotes eg: echo 'SITE_TITLE'; // OR echo "SITE_TITLE"; The constant has to be on its own eg: echo SITE_TITLE; Prehaps check to see if the constant SITE_TITLE is defined first before using it: if (defined('SITE_TITLE')) { echo SITE_TITLE; } else { echo "'SITE_TITLE' does not exist"; }
-
How do i pass array as an argument for a function ?
wildteen88 replied to jd2007's topic in PHP Coding Help
Code works fine for me, using PHP5.2.x here. I noticed you are using the private keyword here for initiating variables. Make sure you are not using PHP4. PHP4 does not support the visibility keywords (private, protected and public), use var in replacement: var $arr=array(); var $mystr; What results do you get? EDIT: Didn't see the replies above Even if the you pass the produceStr function an array for the $arr argument $arr will still be an array, without 'array' before $arr. -
I have not had much experience with IIS but if i remember you will want to add index.php to the default documents box within your websites properties. Look at 2nd image down for what I am referring to here.
-
Use nl2br when you fetch the data from the database, eg: $row['my_row'] = n2br(row['my_row']);
-
Also make sure display_errors is enabled too.
-
You don't use echo when define variables. Just remove the echo's and your code will work.
-
Best creating a function which you pass the number group (UB and -1) and the range (1600 and 1650) which will total up the range from UB -1 1600 to UB -1 1650 function calculate_range($cat, $bool, $range_min, $range_max) { global $number; $total = 0; for($i = $range_min; $i <= $range_max; $i++) { if(isset($number[$cat][$bool][$i])) { $total += $number[$cat][$bool][$i]; } } return $total; } // calculate range UB -1 1600 tp UB -1 1650 echo calculate_range('UB', -1, 1600, 1650);
-
The function does what you assume it does. Which is convert links written as normal text into html links, eg: www.google.com into <a href="www.google.com" target="_BLANK">www.google.com</a>
-
ERROR: MANUALLY CONFIGURE THE WEBSERVER
wildteen88 replied to taurus5_6's topic in PHP Installation and Configuration
I guess you are using the installer to install PHP. Before installing PHP make sure you have Apache installed and that is running. When using the installer it'll try to configure Apache automatically for you. I generally don't use the installer. I prefer a manual install myself. It is really a simple procedure to configure Apache with PHP. -
That line is commented out. If you uncomment it it'll echo out the contents of the $number variable, which will display how the array is formatted. Not sure what you mean by this question:
-
[SOLVED] auto_increment column has lost count and needs fixing!
wildteen88 replied to Edward's topic in PHP Coding Help
smallint has limit between 0 and 32767. For columns which use auto_increment I always use INT(11) Have a look at the following MySQL Cheat Sheet to see what each data type's limit/capacity. -
[SOLVED] Ugh - simple MySQL update query giving me trouble
wildteen88 replied to avillanu's topic in PHP Coding Help
Use mysql_error() in an or die clause after mysql_query: $result31 = mysql_query($query31) or die('Problem with Query:<br />' . $query31 . '<br />' . mysql_error()); Rerun your code. This time if there is a problem with your query an error message will be displayed. -
This line: $rundate = mysql_query($datequery) Should have a semi-colon at the end of it: $rundate = mysql_query($datequery); EDIT: Tyhe same applies to this line too: $date = date("m-d-y") Go through you code and make sure you have semi-colons at the end of lines
-
My code generates the following array, it wont 'overwrite' existing keys/values: Array ( [iB] => Array ( [0] => Array ( [2510] => -81219.00 [2640] => 495.37 [2890] => -43750.55 [2990] => -2300.00 ) ) [uB] => Array ( [-1] => Array ( [1229] => -4700.00 [1250] => 14100.00 [1410] => 20000.00 [1514] => 246892.00 ) [0] => Array ( [1229] => -4700.00 [1250] => 14100.00 [1410] => 20000.00 ) ) ) There is not a problem with my code. Its to do with how file.txt is formated
-
What is the space between each 'column'. Is it a tab? If its change this line: list($cat, $int, $id, $num) = explode(' ', $line_value); To this: list($cat, $int, $id, $num) = explode("\t", $line_value); I thought it was three spaces as this is what you have been posting with your examples for file.txt
-
From what I have a read it looks like the following should do the trick: $contents = file('file.txt'); foreach($contents as $line_value) { list($cat, $int, $id, $num) = explode(' ', $line_value); $cat = str_replace('#', '', $cat); $number[$cat][$int][$id] = trim($num); } //echo '<pre>' . print_r($number, true) . '</pre>'; echo $number['IB'][0][2510]; // returns -81219.00 // similarly $number['UB'][-1][1229]; will return 14100.00 These numbers are based on the numbers in your post here.
-
Something like this: $contents = file('test.txt'); foreach($contents as $line_id => $line_value) { list(, , $id, $num) = explode(' ', $line_value); $number[$id] = $num; } echo $number[2099] + $number[2641]; However with that code, it'll only store the last number for the following: #RES -1 5900 574.92 #RES 0 5900 6402.63 in the $numbers variable, it wont store both values for 5900
-
Or use trim prehaps: if ($val == trim($test)) { trim removes all whitespace characters before and after a string.
-
Doesn't your hosting package come with MySQL? I'm sure all Yahoo hosting plans come with MySQL from looking at this hosting plan chart Why don't you want to install AMP? Installing AMP locally on your PC while developing your PHP scripts can save you a lot of time as you don't have to upload your files to your site and test each time you make a changes. Also if you are starting out with PHP it is the best way to easily get started PHP.
-
Have you been restarting WAMP when you make any changes to the php.ini? You must restart WAMP in order for the changes you have made to come into affect.
-
Installing MySQL on local comp. to run a forum on my local comp.
wildteen88 replied to Wicked_one's topic in MySQL Help
To install phpbb you'll need three things Apache (a webserver), PHP and MySQL. You have to install Apache as PHP is a server side language. Your computer wont understand PHP, even though your have PHP installed. When you install and setup Apache all php files you request from http://localhost will get parsed by PHP. If you are new to installing AMP (Apache, PHP and MySQL) I'd recommend you to install WAMP from wampserver.com. Also the hostname will be just localhost -
If you want to see if something is divisible by three use the following: if($var%3 == 0) { }
-
No. Not unless you convert all $_GET variables eg ?abcvar=123 into session variables.
-
To remove spaces use a simple string replacement with str_replace, eg: $searchText = "123 4567 890"; $newSearchText = str_replace(' ', '', $searchText); echo $newSearchText;