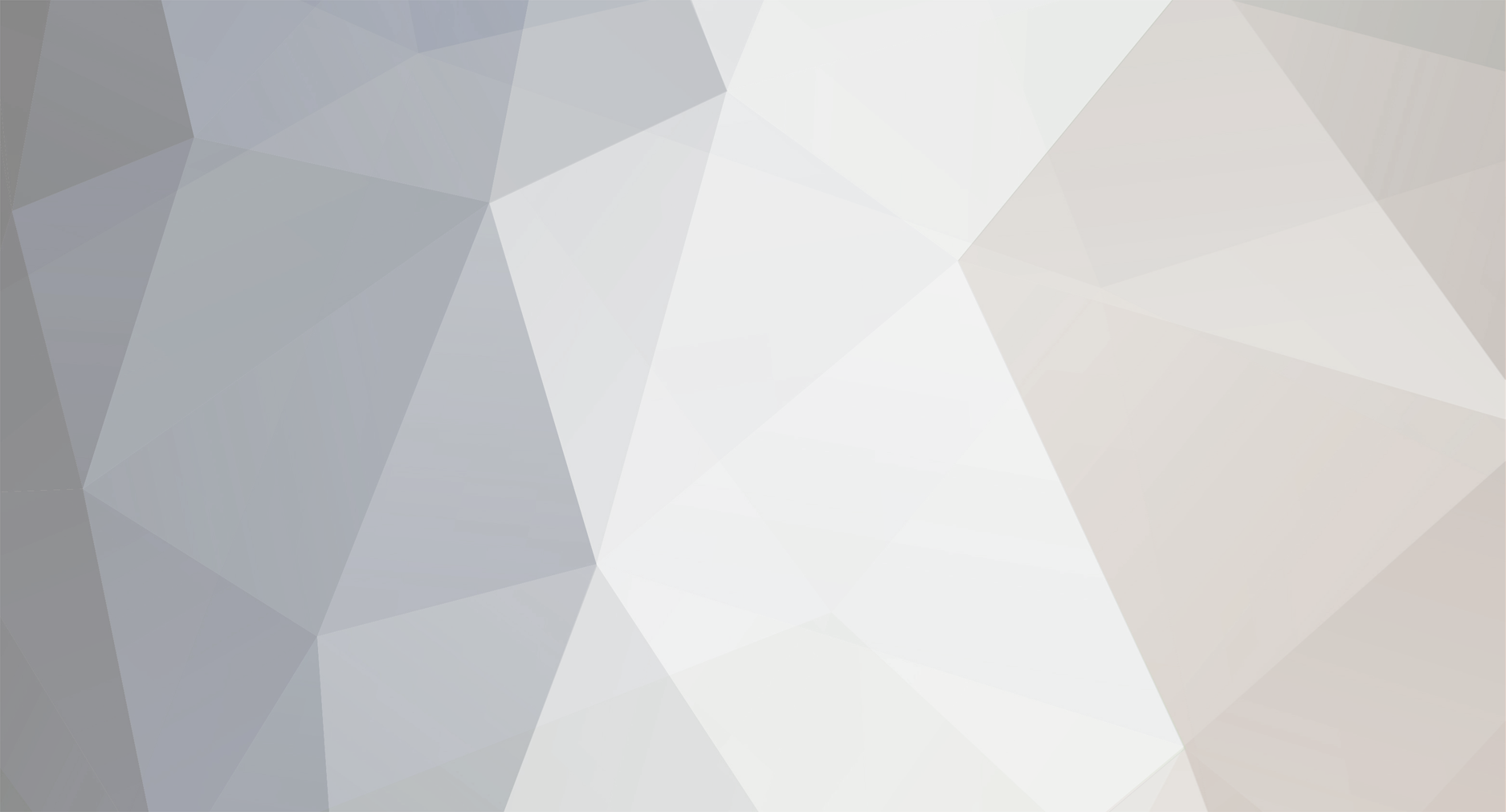
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
If you mean your columns are not aligning up it is because you are echo'ing a separate table for each row. You'll want to remove the table tags out of the loop: <?php //connect to database include'db.php'; //get all data from table $sql = "SELECT * from products"; $result = mysqli_query($mysqli, $sql) or die(mysqli_error($mysqli)); $display_block = "<table border=\"1\">\n"; //if authorized, get the values while ($info = mysqli_fetch_array($result)) { $ItemNo = stripslashes($info['Item_No']); $Man = stripslashes($info['Manufacturer']); $Cat = stripslashes($info['Category']); $Des = stripslashes($info['Description']); $Model = stripslashes($info['Model']); $Qty = stripslashes($info['Qty']); $Kw = stripslashes($info['Kw']); $Hours = stripslashes($info['Hours']); $Price = stripslashes($info['Price']); //create display string $display_block .= " <tr> <td>".$ItemNo."</td> <td>".$Man."</td> <td>".$Cat."</td> <td>".$Des."</td> <td>".$Model."</td> <td>".$Qty."</td> <td>".$Kw."</td> <td>".$Hours."</td> <td>".$Price."</td> </tr>\n"; } //displays string with data echo $display_block . '</table>'; ?>
-
[SOLVED] Sessions (session_register() vs $_SESSION[])
wildteen88 replied to hostfreak's topic in PHP Coding Help
before in order to create a session in the register_global's era you'd have to use session_register to register a session variable, as $_SESSION didn't even exist back then. There were no pre-defined variables as there is now to define a session without calling session_register. Now PHP has moved on and register_globals is now depreciated and so no need to use session_register. Some people may still use session_register because of old habits back in the register_global's era, or has read up on an old tutorial for using PHP sessions. -
No it gets the time from the server. If you want to get the clients time, ie the person visiting your site you can use javascript.
-
I have just downloaded ZPanel and I would not advise you using it. It is full of bugs. Nothing to do with your server. Poorly coded.
-
You'd only be able to use the following url: http://www.url.com/?page=pagename If you have a index.php file in the root of your website. If you don't have an index.php file you'll have to specify a file, so http://www.url.com/?page=pagename is the same as http://www.url.com/index.php?page=pagename When you have a index.php file in the root of folder/your sites root that file will always get called if not file is requested.
-
You should still look in to using mysql_real_escape_string as pocobueno1388 suggested. Your code is prone to SQL Injection attacks, without it.
-
There is no connection between PHP and ruby and rails. Ruby on Rails is completely different language. Do a search on ruby on rails if you do not know what it is. Moved to miscellaneous board.
-
Give your tables/images unique id's. Then you use javascript (rather than PHP) to show/hide a particular table/image using the id. Have a search in Javascript help forum for scripts for showing/hiding elements on a page. There has been many posts for such scripts.
-
If you only want access numbers 6 through to 9 to show a link to office.php just use a simple if statement: // if access greater than or equal to 6 and is less than or equal to 9 // echo link to office.php if($access >= 6 && $access <= 9) { echo " <a class=\"sidelink\" href=\"../office.php\">The Office</a><br>"; }
-
Provide the full/relative path to the file you want to get the filesize off. The file doesn't have to be in the same directory as the script.
-
What do you mean by "connect"? What does the form collect. What are trying to do.
-
This line in upload.php: $fileuploaded = 'upload/' . $file_name . '.swf'; Should be: $fileuploaded = 'upload/' . $userfile_name . '.swf';
-
What does the following bit of code display: $catid = $_GET['cat']; // gets the category id form the url echo $catid; //display current category Prehaps the query is supposed to be: $othercat = "SELECT * FROM categories WHERE id != '$cur_cat'";
-
Ohh! Sorry did a few errors in my code. Try the following: view.php <html> <head> <title>View Flash</title> </head> <body> <?php require "dbconnect.php"; if(isset($_GET['id']) && is_numeric($_GET['id'])) { $id = $_GET['id']; } //selects database row with passed flashid $query = "SELECT * FROM flash WHERE flashid='$id'"; $result = mysql_query($query); if(mysql_num_rows($result) == 1) { $row = mysql_fetch_assoc($result); //declare variables $views = stripslashes($row['views']); $score = stripslashes($row['score']) . ' / 10'; $title = stripslashes($row['title']); $author = stripslashes($row['author']); $email = stripslashes($row['email']); $website = stripslashes($row['website']); $height = stripslashes($row['height']); $width = stripslashes($row['width']); $description = stripslashes($row['description']); $userfile = stripslashes($row['userfile']); $date = stripslashes($row['date']); $category = stripslashes($row['category']); $flashid = stripslashes($row['flashid']); echo'<font size="4"><strong>Movie Stats</strong></font><br> <strong>Title: </strong>' . $title . '<br> <strong>Author: </strong>' . $author . '<br> <a href="mailto:' . $email . '">Email</a> | <a href="' . $website . '" target="_blank">Website</a><br> <strong>Category: </strong>' . $category . '<br>" <strong>Date Added: </strong>' . $date . '<br> <strong>Views: </strong>' . $views . '<br> <strong>Score: </strong>' . $score . "<br>\n"; //make rate form so user can rate submission (we will create the rate.php file later) echo '<form name="form1" method="post" action="rate.php?id=' . $flashid . '"> <div align="center"> <select name="rate" id="rate">'; for($i = 0; $i <= 10; $i++) { echo "\n <option value=\"$i\">$i</option>"; } echo "\n" . ' </select> <input type="submit" name="Submit" value="Rate"> </div> </form> <p><font size="4"><strong>' . $title . '</strong></font></p> <div align="left" style="text-decoration:underline;font-weight:bold;"> Author\'s Discription:<br />' . $description .' </div>'; //echo link that opens movie in new non-resizeable window echo "<br>\n<a href=\"javascript:void(0)\" onClick=\"window.open('$userfile','miniwin','toolbar=0,location=0," . "directories=0,status=1,menubar=0,scrollbars=0,resizable=0,width=$width, height=$height')\">"; //checks if submission is a movie or a game if ($category == 'Game/Interactive') { echo 'Play Game'; } else { echo 'Play Movie'; } echo '</a><br /><br />'; //Read/Write reviews link (opens reviews window) $query = "SELECT * FROM flash_reviews WHERE flashid='$id'"; $result = mysql_query($query); $numreviews = mysql_num_rows($result); echo "<a href=\"javascript:void(0)\" onClick=\"window.open('review.php?id=$id','miniwin','toolbar=0,location=0,directories=0,status=1,men ubar=0,scrollbars=0,resizable=0,width=470, height=510')\">Write/Read Reviews("; //prints number of reviews already written echo $numreviews . ")</a>"; $numreviews++; $viewquery = "UPDATE flash SET views = '".$views."' WHERE flashid=$id"; $viewresult = mysql_query($viewquery); } else { echo 'ID not found'; } ?> </body> </html> I also noticed a few syntax errors in portal.php too. portal.php <html> <head> <title>Flash Portal</title> </head> <body> <h1>15 Most Recent<h1> <?php require "dbconnect.php" ; //displays most recent flash using a while loop $query = "SELECT * FROM flash ORDER BY flashid DESC LIMIT 15"; $result = mysql_query($query); $i = 0; while ($row = mysql_fetch_array($result)) { //increases printed number for flash by one $i++; //declare varibles $score = stripslashes($row['score']); $title = $row['title']; $flashid = stripslashes($row['flashid']); //print flash submission echo $i .' - <a href="view.php?id=' . $flashid . '">' . $title . "</a>\n"; //tells if submission is excellent (score higher than 7.0), okay (score between 3.0 and 7.0), or bad (score lower than 3.0) if ($score <= 3.0) { echo'(Bad!)'; } elseif ($score > 3.0 && $score <= 7.0) { echo '(Okay)'; } elseif ($score > 7.0) { echo '(Excellent!)'; } echo "<br>\n"; } echo "<h1>15 Most Viewed</h1>\n"; //displays 15 most viewed flash $query = "SELECT * FROM flash ORDER BY views DESC LIMIT 15"; $result = mysql_query($query); $i = 0; while ($row = mysql_fetch_array($result)) { //increases printed number for flash by one $i++; //declare varibles $score = stripslashes($row['score']); $title = $row['title']; $flashid = stripslashes($row['flashid']); //print flash submission echo $i .' - <a href="view.php?id=' . $flashid . '">' . $title . "</a><br />\n"; } echo "<h1>Top 15</h1>\n"; //displays 15 top flash (ordered by highest score) $query = "SELECT * FROM flash ORDER BY score DESC LIMIT 15"; $result = mysql_query($query); $i = 0; while ($row = mysql_fetch_array($result)) { //increases printed number for flash by one $i++; //declare varibles $score = stripslashes($row['score']); $title = $row['title']; $flashid = stripslashes($row['flashid']); //print flash submission echo $i . ' - <a href="view.php?id=' . $flashid . '">' . $title . "</a>\n"; } //finish up page by adding end tags ?> </body> </html>
-
You will want to reinstall WAMP then. If the httpd.conf is corrupt, cant see how it did.
-
The array you pass it is empty.
-
Use a Where clause in your query: $cur_cat = mysql_real_escape_string($catid); $othercat = "SELECT * FROM categories WHERE category != '$cur_cat'";
-
Whats register_globals to do with not using your function?
-
wamp is a pre-configured package you can install, this will install, Apache, PHP and MySQL for you. You cannot install PHP on its own if you want to run your php scripts on your computer, unless you want to use the command line. PHP is a server-side programming language and needs to be run on a server. That is what Apache is, MySQL is a database. if you just want PHP. refer to thorpes post above.
-
only if register_globals is enabled. register_globals is now depreciated and is disabled by default as it can cause security exploits within your code. However some hosts do still keep this setting enabled for compatibility reasons for older (outdated) scripts.
-
Prehaps have a look at this post. The script does exactly as you want but it creates three different sized thumbnails, it can be easily changed so it creates just one thumbnail.
-
How is wv_scrores.txt formatted? Post a few example lines from that file. From the looks of it should insert all lines into the database.
-
I'm sure on is not supposed to be here: $popularProds = $db->select("SELECT ... on WHERE ..."); This is where your query is failing.