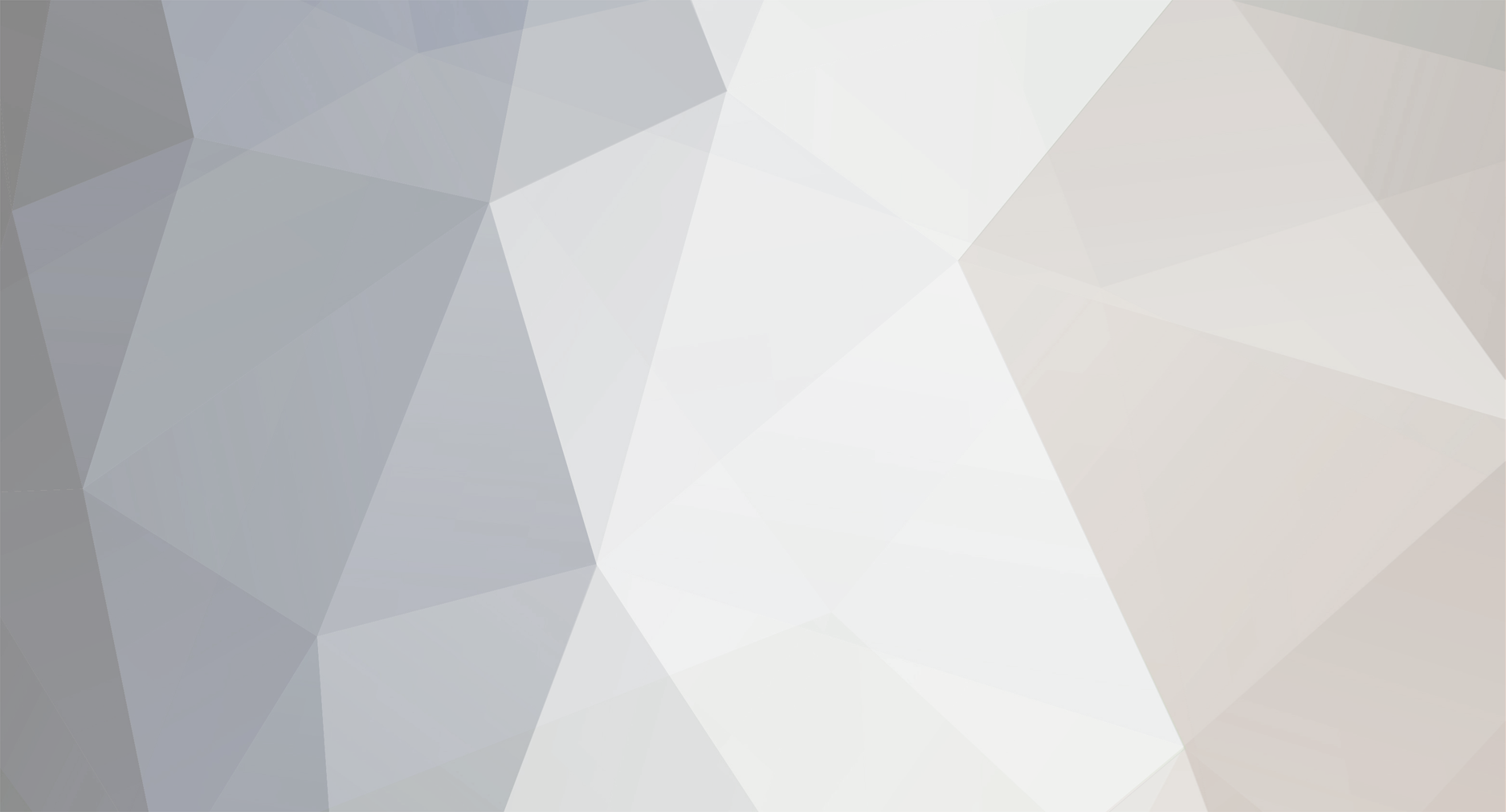
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
Try the attached code below. It'll load each user into their own separate array, example: [user1] => Array ( [0] => 0 [1] => 1 [2] => 2 ) [user2] => Array ( [0] => 132 [1] => 133 [2] => 134 ) The code: <?php $data = file("data.txt"); foreach($data as $line) { $text_line = explode("|",$line); foreach($text_line as $text) { list($user, $col1, $col2, $col3) = array_map('trim', explode(":", $text)); $users[$user] = array($col1, $col2, $col3); } } echo '<pre>' . print_r($users, true) . '</pre>'; ?>
-
I didn't spot this earlier, but when use the MySQL PASSWORD function. This will generate a password hash the length of 41 characters. The way you have your database setup the the support_password field only holds 32 characters. So when you run your query MySQL is trying match a password like the following (passed in the query): *94BDCEBE19083CE2A1F959FD02F964C7AF4CFC29 To this (stored in the database) *94BDCEBE19083CE2A1F959FD02F964C Obviously those two strings will never match. Try setting the support_password field to a char length of 41 instead of 32. If you change the length of the support_password field you'll have to reset all the password stored within that field too.
-
[SOLVED] Printing the contents of each file in a directory
wildteen88 replied to ghr's topic in PHP Coding Help
Do you want PHP to read and display all files contents within a directory or just specific files? I am not sure what you are trying to do. Prehaps you'll want to use a database instead. -
YOu can drop all the session_register calls. The session_register function has been depreciated. You can just use $_SESSION['my_sess_var'] = 'value' instead. To create/edit a session variable. Also in order set/call session variables you must use $_SESSION. Using $_session, $_Session or $session is not the same.
-
relvent topics and better search
wildteen88 replied to nathanmaxsonadil's topic in PHPFreaks.com Website Feedback
Please don't post suggestions about how the forum functions. The forum is not developed by us. If you want a feature changed/added please recommend it to the SMF Team over at simplemachines.org There is a new version of SMF, SMF2.0, coming out soon will have an updated search. -
Your php setup most probably has a setting called magic_quotes_gpc. This setting will automatically escape quotes within _GET, _POST and _COOKIE data What you'll want to is: print"<font color=\"#000000\" Font size=\"3\" Font face=Arial >" . stripslashes($Text12) . "</font>";
-
What you'll want to do instead is use something like the following when including files: include $_SERVER['DOCUMENT_ROOT'] . '/example/example/file.php'; When using that you are using full local path instead of a relative one. As for a shortened version of Jesirose's quotation from the manual, it means this: If you use a URL Wrapper (eg: http://) when including a file PHP wont inherit the parent file's variable scope. Instead the included script will get parsed separately from the parent. The parent will receive just the result from processing the the script. Example: <?php $text = 'Hello World'; include 'http://www.mysite.com/include.php'; ?> <?php echo '<b>' . $text . '</b>'; ?> The result would be: Notice: Undefined variable: text in path/to/include.php on line 3 This is because include.php is being processed separately from the parent.php, and so include.php doesn't inherit the global scope from the parent. However if you used a file path for the include instead in parent.php, eg: include $_SERVER['DOCUMENT_ROOT'] . '/include.php'; The output would be: Hello World
-
Change this block of code: if (mysql_num_rows($result) == 1) { // the user id and password match, // set the session $_SESSION['db_is_logged_in'] = true; // after login we move to the main page header('Location: support_main.php'); exit; } else { $errorMessage = 'Sorry, wrong user id / password / pin'; header('Location: index.php'); } To the following to see what is happening: if (mysql_num_rows($result) == 1) { /*// the user id and password match, // set the session $_SESSION['db_is_logged_in'] = true; // after login we move to the main page header('Location: support_main.php'); exit;*/ echo 'Query return 1 result!'; } else { /*$errorMessage = 'Sorry, wrong user id / password / pin'; header('Location: index.php');*/ echo 'Query did not return just 1 result. Query returned ' . mysql_num_rows($result) . ' results.<br />'; } I have commented out the existing code. What do you get now. The rest of the code looks fine, this includes the query too. I have a feeling that its to do with the if/else block at the end.
-
You have to fill in that form manually. phpBB install wont fill in the form automatically for you. As for the script path field not being filled in automatically it is because you have placed all phpbb files and files in the root of easPHP's www folder (eg: C:\easyPHP\www), whereas on the phpBB knowledge base they place all phpBB files and folders in side a another folder called phpBBtest within the easyPHP www folder (eg: C:\easPHP\www\phpBBtest). Thats why the screenshot shows /phpBBtest/ in the Script Path field. it doesn't matter where you place the files and folders for phpbb within easyPHP's www folder it'll still work. You should fill in the form field as the image shows in the knowledge base, changing the Database Name filed to the database you created in phpMyAdmin (the database you created was called web).You'll also want to setup the Administrator username and password so you can login to phpBB and manage your board, the username and password can be anything you want.
-
When using escape characters use double quotes and not single quotes. However you may aswell use nl2br to do what you are trying to accomplish. However only use nl2br when you get the data out of the database not when you insert the data into the database.
-
help needed with changing td background with css and php
wildteen88 replied to spindriftmed's topic in PHP Coding Help
Now you have changed your links to ?p=home. So now the php block at the top of your page will not work! Stop changing your url variables and your code will work! Try the following code at the top fo your script: $strClassType = 'defaultPage'; if(isset($_GET['pagename'])) { $intPageType = strtolower($_GET['pagename']); switch($intPageType) { case "home": $strClassType = 'homePage'; break; case "portfolio": $strClassType = 'portfolioPage'; break; default: $strClassType = "defaultPage"; } } Make sure your links are set to ?pagename=YOUR_PAGE_NAME -
sessions are separate from users. sessions are not shared through users connected to the site. Every time someone connects to your site they get assigned a unique session id.
-
Remove is_ from is_empty time for me to go to bed I think.
-
Recopy the suggested code again. I didn't copy it correctly, just edited my post after you read it
-
Just another bug I found. Another line I forgot to change in save_cart.php is this line: if($_POST[$prdnumber]=='0') it should of been: if($_POST[$prdnumber]['qty'] == '0' || is_empty($_POST[$prdnumber]['qty'])) If you update a product with a quantity of zero and update the cart, the product does not get removed from the list of ordered products. Glad I helped.
-
Sorry about did a typo, that line should be: $selected = ($_SESSION['cart'][$prdnumber]['shoesize'] == $shoe_size) ? ' selected="selected"' : null; Missed out the equal sign.
-
Try the following: foreach($_SESSION['cart'] as $prdnumber => $prddetails) { extract($prddetails); //$orderid = mysql_insert_id(); $detail = get_book_details($prdnumber); $query = "delete from order_items where orderid = '$orderid' and prdnumber = '$prdnumber'"; $result = mysql_query($query); $query = "insert into order_items values ('$orderid', '$prdnumber', '$shoesize', ".$detail['price'].", '$qty')"; $result = mysql_query($query); } return $orderid; } Also make sure you have corrected the bug I mentioned in my post above, about the sticky shoesizes
-
Ohh, looks like my codes now working! However I have just noticed a bug for the shoe sizes drop downmenu where the last size in the in the menu gets selected no matter what value you select in the dropdown. This is because I didn't unset the $selected variable in the display_cart function. Change this block: if($_SESSION['cart'][$prdnumber]['shoesize'] == $shoe_size) { $selected = ' selected="selected"'; } to this: $selected ($_SESSION['cart'][$prdnumber]['shoesize'] == $shoe_size) ? ' selected="selected"' : null;
-
Try: function calculate_price($cart) { // sum total price for all items in shopping cart $price = 0; if(is_array($cart)) { $conn = db_connect(); foreach($cart as $prdnumber => $prddetails) { extract($prddetails); $query = "select price from products where prdnumber='$prdnumber'"; $result = mysql_query($query); if ($result) { $item_price = mysql_result($result, 0, 'price'); $price += $item_price*$qty; } } } return $price; } You may get the same error for calculate_items too, if you do just change the foreach loop to how I have it above (including the extract function too). Also you'll want to change the bottom of save_cart.php ( if($_SESSION['cart'] && array_count_values($_SESSION['cart'])) ) to the following too: if(isset($_SESSION['cart']) && (count($_SESSION['cart']) > 0))
-
Hehe... I missed a line. The following line in save_cart.php: //if not, you add the new item to the cart $_SESSION['cart'][$prd_id] = 1; Should of been: //if not, you add the new item to the cart $_SESSION['cart'][$prd_id]['qty'] = 1;
-
woops. I placed the semi-colon in the wrong place, Use: // display_cart($_SESSION['cart']); echo '<h1>Session Data</h1>'; die( '<pre>' . print_r($_SESSION['cart'], true) . '</pre>');
-
Umm, looks like the session isn't being rebuilt properly. Change the following line in save_cart.php: display_cart($_SESSION['cart']); to this: // display_cart($_SESSION['cart']); echo '<h1>Session Data</h1>'; die( '<pre>' . print_r($_SESSION['cart'], true) . '</pre>' This will break your code. But it's only temporary. Its just to see how the session is being populated.
-
help needed with changing td background with css and php
wildteen88 replied to spindriftmed's topic in PHP Coding Help
These two lines: if(isset($_GET["page"])) { $intPageType = $_GET["page"]; Should be: if(isset($_GET["pagename"])) { $intPageType = $_GET["pagename"]; You are using ?pagename in your url's to identify the page and not ?page -
Did what? Run install.php? What part didn't work? Make sure MySQL is started and that Apache (or whatever webserver easyphp installs) is running/setup properly.