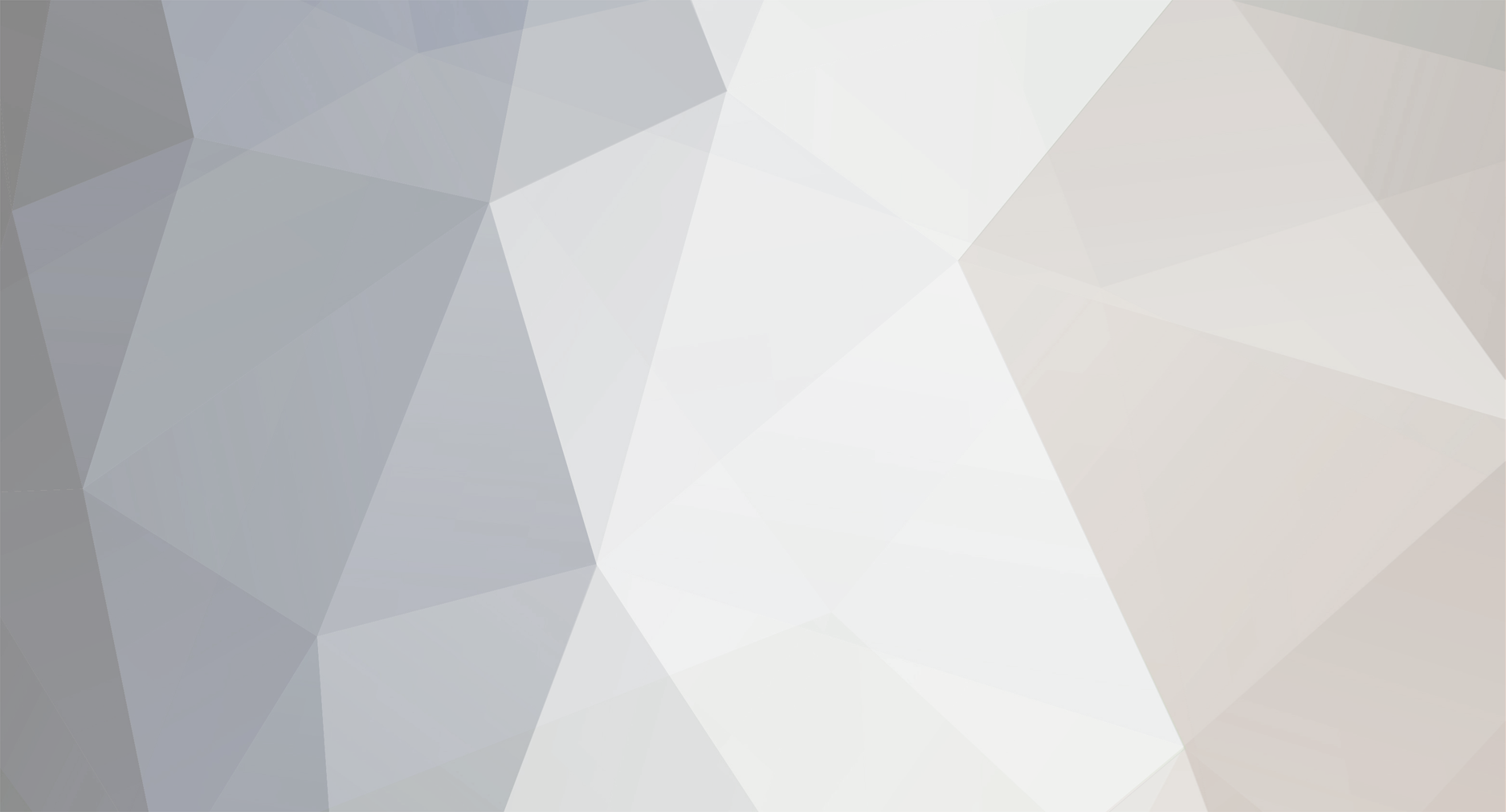
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
Form Validation Data, where should I put it?
wildteen88 replied to VinceGledhill's topic in PHP Coding Help
You'd validate your data just before you save it in the database. So add it in between the lines if(isset($_POST['submit'])) { and //connect to database -
You'll want to name your radios buttons as question[$id], eg <input type="radio" name="question[<?php echo $id; ?>]" value="A"/>A. <?php echo $option1 ?><br /> <input type="radio" name="question[<?php echo $id; ?>]" value="B"/>B. <?php echo $option2 ?><br /> <input type="radio" name="question[<?php echo $id; ?>]" value="C"/>C. <?php echo $option3 ?><br /> <input type="radio" name="question[<?php echo $id; ?>]" value="D"/>D. <?php echo $option4 ?><br /> Now $_POST['question'] will be a multi-dimensional array containing all the users answers to the quiz Now in score.php you logic completly wrong here. Variables do not get passed from page to page. So the variable $answer will not exits in this page. What you will have to do in score.php is connect to your database. Run an SQL query and grab all the answers and place them in an array. // get all answers and add them to an array $query = 'SELECT autokey, answer FROM questions'; $result = mysql_query($query); while ($row = mysql_fetch_assoc($result)) { $question_id = $row['autokey']; $answer = $row['answer']; $question_answers[$question_id] = $answer; } Now we have the array of answers. We can now use a loop and compare the users answers. Like so // loop through the posted ansers and compare foreach($question_answers as $question_id => $question_answer) { if(isset($_POST['question'][$question_id]) { $user_answer = $_POST['question'][$question_id]; if($user_answer == $question_answer) { echo "<p>Your answer was correct for Q{$question_id}!<p>"; } else { echo "<p>Your answer was incorrect for Q{$question_id}.<p>"; } } else { echo "<p>You didn't give an anser for Q{$question_id}.<p>"; } }
-
use [0-9] character set or just \d when looking for numbers. You also need to set your pattern delimiters too. <?php $rep['find'] = '/<<!--{rep_([0-9]+)}-->>/e'; $rep['replace'] = "\$reputation->postRating('$1')"; $html = preg_replace($rep['find'], $rep['replace'], $html); ?> You'll notice I used the e pattern modifier, Otherwise the number will not be passed to your postRating function.
-
Possible to insert a space between a lowercase and capital letter?
wildteen88 replied to Jeffro's topic in PHP Coding Help
Does it display like that in the actual rss feed? If it doesn't then maybe you did something when parsing the rss feeds in your code. But anyway you can use regex. Something like this $str = 'ThisIsMyTestString'; $str = preg_replace('/([a-z])([A-Z])/', '$1 $2', $str); echo $str; -
use trim on $found[1] variable.
-
Parse error: syntax error, unexpected T_STRING in ... Help
wildteen88 replied to Jnerocorp's topic in PHP Coding Help
You're getting that error due to you not wrapping the query within quotes. This is how line 54 should read $result = mysql_query('SELECT * FROM p8_subscribers') or die(mysql_error()); -
Possible to insert a space between a lowercase and capital letter?
wildteen88 replied to Jeffro's topic in PHP Coding Help
Could you provide an example. Not quite following your there. Why the need to add a space between a lowercase letter and uppercase letter? -
Yes that is fine. That is perfectly valid syntax.
-
Maybe use file instead. File load each line into an array, Eg $lines = file('file.txt'); $lines[0] will be line1 $lines[1] will be line 2 etc. You can loop through the lines with a foreach loop $lines = file($this->_file_name); foreach($lines as $line) { // skip lines with spaces if(trim($line) == '') continue; preg_match('/^(.*?)=(.*?)$/', $line, $found); $this->_values[$found[1]] = $found[2]; print_r($this->_values); }
-
I think gizmola is referring to the configuration of Apache. Apache is an http server which MAMP installs (MAMP stands for Mac, Apache, MySQL and PHP). He was suggesting changing Apaches default configuration of DOCUMENT_ROOT from Users/user1/Documents/DEV/++htdocs to Users/user1/Documents/DEV/++htdocs/00_MYyWebsite That way your WEB_ROOT paths will be the same on your Mac and website. Therefore there will be no need for the constant WEB_ROOT Another way would be to setup your project as a virtualhost, so rather than going to ttp://localhost/00_MyWebsite/ you'll instead have a url say http://localhost.mywebsite/ or http://local.mysite.com/ or whatever url you'll want to use. This is the approach I'd take when dealing with multiple projects. Sorry, If I have just confused you again.
-
Umm.... Isn't that basically the same as the code I posted earlier! Did you know includes behave differently when using a url as the path rather than a local file system path. include 'http://thingsihateaboutyou.net/michelle/membersgear/config/config.php'; If you have variables in config.php that you may want to use in members.php then they won't be accessible. That is because when using a url with incude it will only return the output of that script rather than the source code.
-
Can your issue more clearly. I do not understand what you're tying to do
-
How to Detect an Upload from Youtube?
wildteen88 replied to techsandwhichyt's topic in PHP Coding Help
Yes that is possible with the youtube api. Have a read of the youtube api for PHP. -
One major problem you have is you're calling session_start after output has been taken place. You can only call session_start() before any output has been taken place, otherwise sessions will fail to work. nav.php <html><body> <?php session_start(); What you need to do is clean up your code. In nav.php remove the <html><body></body></html> tags. There is no need to add these HTML tags for every PHP file you include, just the main php file. Which I guess is changepassword.php Remove session_start() from nav.php and add this line as the very first line in changepassword.php <?php session_start(); ?> That should sort your session issue out. Next your code can be cleaned up a bit. There is no need for this if if ($submit) { As you have already checked whether the form was submitted a couple of lines before. So you can remove that. These four if ($oldpassword&&$newpassword&&$reppassword) { if ($newpassword==$reppassword) { if (strlen($newpassword)<26) { if (strlen($newpassword)>6) { can be written as two if($newpassword == $reppassword) { if(strlen($newpassword) > 6 && strlen($newpassword) < 26) { ... rest of your code here } else { echo 'Password must be between 6 and 26 chracters'; } } else { echo 'Your passwords do not match!'; } Next, This can be all done within the update query $query = mysql_query("SELECT * FROM users WHERE username='$username'"); while ($row = mysql_fetch_assoc($query)) { $dbpassword = $row['password']; } if ($oldpassword==$dbpassword) { $queryreg = "UPDATE users SET password='$newpassword' WHERE username='$username'"; die("Din kode er nu ændret. <a href='home.php'>Tilbage til start</a>"); } So the above can be written as just $queryreg = "UPDATE users SET password='$newpassword' WHERE username='$username' AND password='$oldpassword'"; $result = mysql_query($queryreg); // check that the query successfully executed if(!$result) trigger_error("MySQL Error:". mysql_error() . "<br /> Query: $query", E_USER_ERROR); // check whether a row was updated if(mysql_affected_rows() == 1) { echo 'Password has been reset'; } else { echo 'Password has not been reset, probably due to username/password are incorrect' } With your code now cleaned up it should run as you expect it to. However you should look into encrypting your users passwords. Storing passwords as plain text is not very secure.
-
Why are you needing to add the numbers 50 (or whatever X is) all the way down to 1 to your database?
-
@Jnerocorp the change you made will not make a difference. Variables within double quotes is perfectly fine. Have a read of this tutorial for working with dates in PHP and MySQL.
-
Yes you can have story/$cominngDynamicString call story.php?read=$cominngDynamicString but what mod rewrite will not do is modify your existing links within your files. You will have to edit your code so your links uses the new url. RewriteEngine on RewriteRule ^story/([a-z0-9]+)$ story.php?read=$1 [NC,L]
-
Yeah you're making this more complicated than needs to be. Set the link to the members area back to memebers.php As it is members.php only displays the contactname for the user that is logged in. What are you trying to do in members.php?
-
How to Detect an Upload from Youtube?
wildteen88 replied to techsandwhichyt's topic in PHP Coding Help
Not sure what you mean here. Are you wanting to detect when a new video has been added to youtube? Maybe have a look into using the youtube api. -
I assume you're setting the username session variable when a user is successfully logged in? But you unset this variable when they are not logged in. If that is the case then you can easily change the visibility of your links with this block of code <?php if (!isset($_SESSION['username'])): display links for users that are not logged in <?php else: ?> display links for users that are logged in <?php endif; ?> So if the user signs in as bob you want the user to go to bob.php? Why are you creating separate php files for each logged in user?
-
You will have to build the array of settings from the results of the query, Like so // get all settings from database $query = "SELECT `name`, `status` FROM `settings`'"; $result = mysql_query($query); // now add all settings to an array // we'll call it $settings while($row = mysql_fetch_assoc($query)) { $name = $row['name']; $status = $row['status']; $setting[$name] = $status; } // display the status of the setting named some echo $setting['something'];
-
First make sure your <form></form> tags and submit button are outside of your while loop. Otherwise only one profile will be updated. I'd place the opening form tag before this line echo "<table width=\"896\" border=\"0\" cellspacing=\"0\" cellpadding=\"0\">"; The submit button and closing form tag should be placed just before the closing php } ?> Also if you have multiple userids then you'll want to change this line <input type=\"text\" name=\"userid\" id=\"userid\" value='".$row['userid']."'> to <input type=\"text\" name=\"userid[]\" id=\"userid\" value='".$row['userid']."'> Notice the square brackets. If you didn't add that then only the last profile that is associated with that userid will be updated. Now your form should submit all profiles to be edited.
-
Looking at the code in your first post, It could be down to you using 3 nested foreach loops when running your query. foreach ($_POST[user] as $key => $value) foreach ($_POST[userid] as $key => $value1) foreach ($_POST[email] as $key => $value2){ ... Why the need for 3? From I see you only need to use one. Having nested foreach loops you're just asking for trouble. foreach ($_POST[user] as $key => $value) { $userid = $_POST['userid'][$key]; $email = $_POST['email'][$key]; ...
-
Because your logic is not quite right when you're logging in the user. This is how I'd proccess the login <?php // check form has been submitted if(isset($_POST['submit'])) { // grab username/password // sanitize username and encrypt password $username = mysql_real_escape_string($_POST['username']); $password = sha1($_POST['password']); $connect = mysql_connect("localhost","root", "") or die ("Couldn't Connect!"); mysql_select_db("userlogin", $connect) or die("Couldn't find db"); // comprare username AND password within the query. $query = mysql_query("SELECT * FROM users WHERE username='$username' AND password='$password'"); // check that there was a match if (mysql_num_rows($query) == 1) { // grab the data, no need for a while when only one record was return from the query $row = mysql_fetch_assoc($query); // get the data, no need to grab username/password as we already have those $dbscore = $row['score']; $dbdclty = $row['dclty']; $dbid = $row['id']; $dbnewdclty = $row['newdclty']; // set the session vars $_SESSION['username'] = $username; $_SESSION['password'] = $password; $_SESSION['id'] = $dbid; $_SESSION['PreviousScore'] = $dbscore; $_SESSION['dclty'] = $dbdclty; $_SESSION['newdclty'] = $dbnewdclty; // display success message echo ("You Successfully Logged In!"); } else { // no records returned either invalid user or password was wrong echo("Invalid username/password provided"); } } ?> add your login form here As your're using sha1() encryption make sure your passwords are stored as the encrypted form too. You should also make sure your password field is set to VARCHAR with atleast 42 characters (as that is length of a sha1 string). If its set to anything different the query with fail regardless of using the correct username/password.
-
You will be far better of learning joins. Joins will allow you to get data from more than one table within a single query (as long as your data relates to each other). Having to manage three separate loops to piece together the data is not worth doing and just makes things much harder. The join I provided earlier should work with your ships table too, Example query SELECT p.PlanetName, p.PlayerName, f.FleetName, f.Status, s.ShipName FROM Planets p LEFT JOIN Fleet f ON (p.PlanetName = f.PlanetName) LEFT JOIN Ships s ON (f.FleetName = s.FleetName) WHERE p.PlanetName = '$colname_Planet'