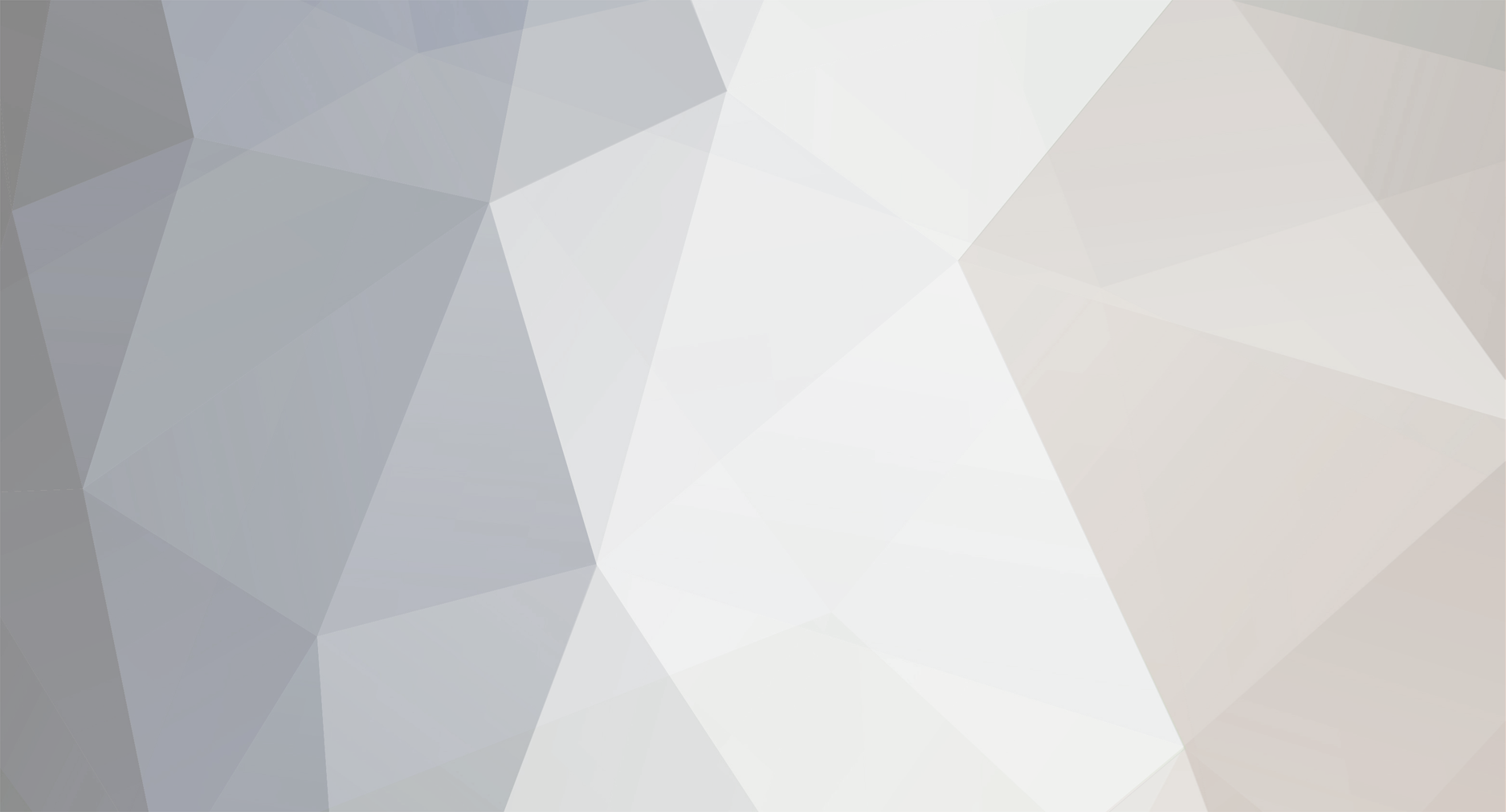
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
Your query is completely wrong for altering the users password $queryreg = mysql_query("INSERT INTO users VALUES WHERE username='$_SESSION['username']' ('','','$newpassword','','','','','','','','','')" When modifying records within the database you'll want to run an UPDATE query. An INSERT query is used for adding new records to the database. So to update the password your query with be $query = "UPDATE users SET password='$newpassword' WHERE username='{$_SESSION['username']}"; $result = mysql_query($query); if($result) { // successfully changed the password } else { // password wasn't changed. }
-
Make sure this line echo implode(' •', $links); Is outside of the while loop.
-
See my reply in your previous thread here http://www.phpfreaks.com/forums/index.php?topic=331263.msg1558873#msg1558873
-
You could do $links = array(); while($row=mysqli_fetch_array($query)){ $links[] = '<a href="viewlistings.php">' . $row['name'] . '</a>'; echo implode(' •', $links);
-
Need help on connecting to mysql database from php.
wildteen88 replied to boyyo's topic in PHP Coding Help
gizmola meant using the function mysqli_error to find out why your query is failing, Checkout the snippet gizmola posted Notice the use of the mysqli_error function. What is the error message? -
$plans need to be wrapped in (single) quotes within your $query_Fleet string. However you could do the above in one query using a simple join SELECT p.PlanetName, p.PlayerName, f.FleetName, f.Status FROM Planets p LEFT JOIN Fleet f ON (p.PlanetName = f.PlanetName) WHERE p.PlanetName = '$colname_Planet'
-
Example code $string = 'problem is an obstacle, impediment, difficulty or challenge, or any situation that invites resolution; the resolution of which is recognized as a solutionquestion raised for inquiry, consideration, or solution proposition in mathematics or physics stating something to be done'; $word = 'inquiry'; $word_pos = strpos($string, $word); // get the position of the word $startPos = $word_pos - 20; // 20 characters to the left of the word $endPos = strlen($word) + 40; // 20 characters to the right of the word $new_string = substr($string, $startPos, $endPos); echo "...$new_string...";
-
There are many functions for manipulating text. The two functions you'll want to use for your problem would be strpos and substr. Have a readup on those two functions and see if you can work it out.
-
Then remove the where clause and use a while loop to display all the settings $query = "SELECT `name`, `status` FROM `settings`'"; $result = mysql_query($query); while($row = mysql_fetch_assoc($query)) { echo 'The setting <b>' . $row['name'] . '</b> is set to <b>' . $row['status'] . '</b><br />'; }
-
Probably due to the space just before images/ on this line <img class="logo" src="<?php WEB_ROOT ?> images/my_logo.png" width="220" /> Remove the space and your logo should work.
-
What data type have you set your id field to when creating your table? Make sure the id column is set to INT
-
Not sure what is wrong with your code but you could use regex for this. Like this pattern.
-
For getting status of the setting named some. Your query would be $query = "SELECT `status` FROM `settings` WHERE `name` = 'some'"; $result = mysql_query($query); $row = mysql_fetch_assoc($query); echo "the status of some is:" . $row['status'];
-
Yes that is correct. This is what $_SERVER['DOCUMENT_ROOT'] will be set to. You can confirm this by running echo 'My webroot path is: ' . $_SERVER['DOCUMENT_ROOT']; No, that is incorrect. netbeans will not alter your websites webroot. All netbeans did was add a folder to your webroot folder called 00_MyWebsite. This folder contains all files for your site (php/html/java scripts, images etc). As all your files are contained within this folder I'd consider setting a variable which sets 00_MyWebsite as the webroot. Like so <?php $MY_WEBROOT = $_SERVER['DOCUMENT_ROOT'] . '/00_MyWebsite'; ; When performing any includes we'll prepend this variable in front of our file paths. Like so <?php require_once($MY_WEBROOT . '/components/body_header.inc.php'); ?> Above code should now included body_header.inc.php successfully. That is because you used a relative file path, instead of an absolute file path. Above we're dealling with absolute file paths
-
You can use array_change_key_case to convert the keys to lowercase.
-
Small typo remove the ( from the end of this line $query = 'INSERT INTO blog_tags (post_id, tag) VALUES (';
-
Use explode. Example $tags = explode(',', $_POST['your_tag_field']; print_r($tags); To insert the tags into your tags table I'd do something like this $cat_id = (int) $cat_id; $query = 'INSERT INTO tags (cat_id, tag) VALUES ('; // dynamically build the query foreach($tags as $tag) { $tag = mysql_real_escape_string($tag); $query .= "($cat_id, '$tag'), "; } // clean the query up, remove the comma and space at the end of the query string $query = substr($query, 0, -2); mysql_query($query);
-
You capture the return value by assigning it a variable $myFuncResult = myfunction($name);
-
When making changes to httpd.conf make sure you restart Apache afterwards. The same applies to the php.ini too.
-
This will only work if PHP is installed as an Apache Module and that Apache is configured to read .htaccess files too.
-
You're not sanitizing your user input correctly. The hacker is using an attack known as SQL Injection to grab the passwords from your databases. If Trainer_ID is only supposed to contain a number you should check this before you use it. I'd do something like this to make surer Trainer_ID is actually a number if(isset($_GET['Trainer_ID']) && is_numeric($_GET['Trainer_ID'])) $Trainer_ID = $_GET['Trainer_ID']; Here we're checking to make sure the variable exists. Then we validate it to make sure it contains the data we're expecting in this case a number. If you do not sanitize your user input then you will be prone to these kinds of attacks. Have a read of this four part article for tips on writing secure PHP code.
-
Streamlining Dreamweaver generated PHP code
wildteen88 replied to MargateSteve's topic in PHP Coding Help
To concatenate the date into your string you'd do something like this echo 'your existing string here .... <strong>DATE OF BIRTH: </strong><br />'. date('jS F Y',strtotime($row['date_of_birth'])) .'<br /> .... the rest of the string'; For the if statement you'll want to end the current echo statement, then do your if/else statement outputting whatever it is and then start another echo statement. echo ' your existing string here .... <strong>HOME SPONSOR</strong><br />'; // end the current echo statement // now do the if/else statement if ($row['home_sponsor'] <> "") echo $row['home_sponsor']; else echo '<a href="http://www.margate-fc.com/content/commercial/sponsorship/shirt_sponsors.php">AVAILABLE - Click here for info</a>'; // now start another echo statement. echo '..... the rest of your string'; Or you can use a ternary operator (an inline if/else statement) echo ' your existing string here .... <strong>HOME SPONSOR</strong><br />' . ( ($row['home_sponsor'] <> "") ? $row['home_sponsor'] : '<a href="http://www.margate-fc.com/content/commercial/sponsorship/shirt_sponsors.php">AVAILABLE - Click here for info</a>' ) . ' ..... the rest of your string'; -
You may want to use fopen/fget instead?
-
Strings need to be wrapped in quotes <?php if ($players['Banned'] == 'Yes'){ Otherwise PHP will think you're using a constant. Which is explained in the error
-
mod_rewrite isn't a coding language as such. It is a module which extends Apaches functionality. There is not much to learn of mod_rewrite. Have a read of the Apache documentation on mod_rewrite for how it works. However you will want to have a good understanding of regular expressions too.