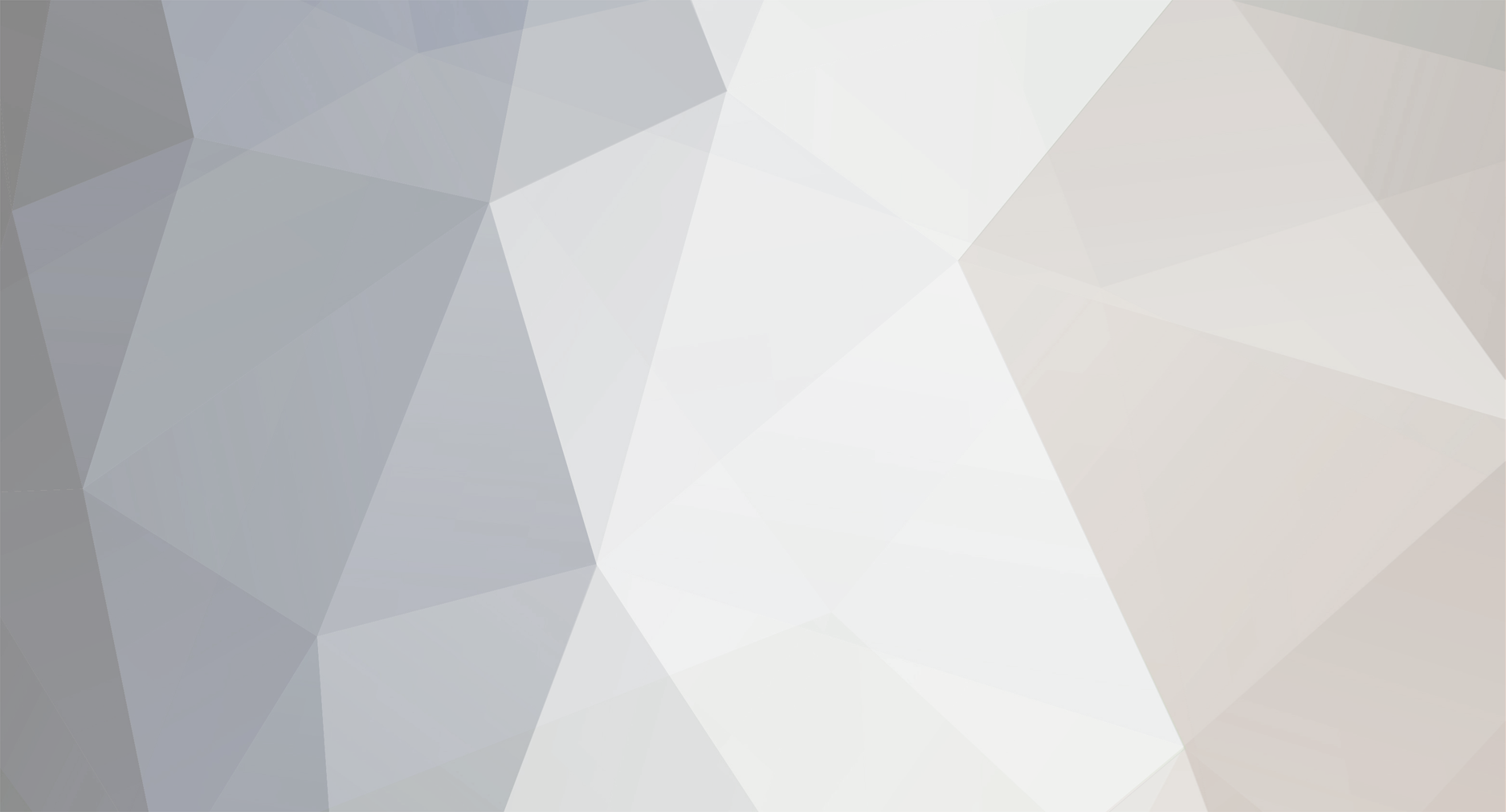
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
Look into using the GROUP BY clause and the GROUP_CONCAT function.
-
I guess you have a form setup like so <form action="" method="post"> Username: <input type="text" name="username" /><br /> Password: <input type="text" name="password" /><br /> <input type="submit" name="submit" value="Login" /> </form> And an array of users $authUsers = array ( 'Joe' => 'pass1', 'Sam' => 'pass2', 'Bob' => 'pass3' ); To see of the entered username/password is valid you'd do if(isset($_POST['username'], $_POST['password'])) { $user = $_POST['username']; $pass = $_POST['password']; if(isset($authUsers[$user]) && $authUsers[$user] == $pass) { echo '<h1>Success! You are a valid user</h1>'; } else { echo 'Sorry but the username/password are incorrect'; } }
-
Your host most probably doesn't accept remote database connections. You will be better of extracting your database(s) from your host and importing them locally.
-
By vertically do you mean output the results as 1 6 11 2 7 12 3 8 13 4 9 14 5 10 15 rather than 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 Have look at my code example I posted in this thread http://phpfreaks.com/forums/index.php/topic,308888.msg1459408.html#msg1459408
-
Warning: implode() [function.implode]: Invalid arguments passed
wildteen88 replied to bex's topic in PHP Coding Help
I guess line 73 and74 is <?php $include_categories = ot_option('include_categories'); ?> <?php wp_list_categories('depth=4&title_li=&sort_column=menu_order&include='.implode(',', $include_categories)); ?></ul> implode expects the second argument to be an array. What does the function ot_option() return? -
Change RewriteRule . Track/%1? [NC,R,L] to RewriteRule . /Track/%1? [NC,R,L]
-
Try out the following RewriteEngine On RewriteCond %{ENV:REDIRECT_STATUS} ^$ RewriteCond %{REQUEST_URI} track\.php$ [NC,OR] RewriteCond %{QUERY_STRING} ip\=([^&]+)(&.*)?$ [NC] RewriteRule . Track/%1? [NC,R,L] RewriteRule ^Track/([a-z0-9.]+)/?$ track.php?ip=$1 [NC,L] RewriteRule ^Track/?$ track.php [NC,L] If you go to site.com/track.php it should redirect you to sitre.com/Track/ Submitting the form will go to site.com/Track/AA.BB.CC.DD
-
Try this instead <?php if(!isset($_POST['input'])) { echo ' <b>How many pieces of data do you have?</b> <form action="" method="POST"> <input type="text" name="input"> <input type="submit" name="submit" value="Submit"></form><br/> <font size="2">Ex: 88.8,92.3,76.5 - That would be 3 pieces of data</font>'; } else { if(!is_array($_POST['input'])) $data = explode(',', $_POST['input']); // get data from first form else $data = $_POST['input']; // get data from secound form echo ' <b>Data Values</b> <form action="" method="POST">'; $i = 0; foreach($data as $number) { echo ++$i . ': <input type="text" name="input[]" value="'.$number.'" /><br />'; } // work out the average $dataSum = array_sum($data); $average = $dataSum / $i; echo ' <b>Average:</b>: ' . $average . '<br /> <input type="submit" name="submit" value="Recalculate Average" /> </form>'; } ?>
-
If your forms submit method is POST, then you'd get the selected size from the $_POST['size'] variable $size = $_POST['size']; To assign the chosen size to the session you'd do $_SESSION['size'] = $size; To get the size from the session you'd use the $_SESSION['size'] variable.
-
<?php if(!isset($_POST['input'])) { echo ' <b>How many pieces of data do you have?</b> <form action="" method="POST"> <input type="text" name="input"> <input type="submit"></form><br/> <font size="2">Ex: 88.8,92.3,76.5 - That would be 3 pieces of data</font>'; } else { $data = explode(',', $_POST['input']); echo '<form action="" method="POST">'; $i = 0; foreach($data as $number) { echo ++$i . ': <input type="text" name="data[]" value="'.$number.'" /><br />'; } echo '</form>'; } ?>
-
If the user is inputting comma delimited list of data eg 88.8,92.3,76.5 Then you'll want to use explode to get each number in the list. $data = explode(',', $_POST['input']); Now $data will be contain array of each individual number, eg $data[0] will be 88.8, $data[1] will be 92.3 etc. Seeing as $data is an array you'd use a foreach loop to output each number within its own form field. echo '<form action="index.php" method="POST">'; $i = 0; foreach($data as $number) { echo ++$i . ': <input type="text" name="data[]" value="'.$number.'" /><br />'; } echo '</form>';
-
Dynamic form from array. or better solution ????
wildteen88 replied to Peterod's topic in PHP Coding Help
To generate the form you'd simply output your individual form fields within the while loop, For example <form action="" method="post"> <?php // results while ($row = mysql_fetch_array($query)): $id = $row['id']; ?> <p> Material: <input type="text" name="materials[<?php echo $id; ?>][name]" value="<?php echo $row['material']; ?>" /><br /> Qantity: <input type="text" name="materials[<?php echo $id; ?>][quantity]" value="<?php echo $row['quantity']; ?>" /><br /> Price: <input type="text" name="materials[<?php echo $id; ?>][price]" value="<?php echo $row['unit']; ?>" /><br /> </p> <?php endwhile; ?> <input type="submit" name="submit" value="Submit" /> </form> Now I have setup the form fields to be grouped together within the materials array. This will allow us to easily process the form results using a simple foreach loop eg foreach($_POST['materials'] as $material_id => $material_data) { extract($material_data); echo "Material #$material_id :: $name :: $quantity :: $price<br />"; } -
Using 2 mysql_connect() functions in 1 PHP project.
wildteen88 replied to theITvideos's topic in PHP Coding Help
You can query more than one table per connection. You do not need to open another connection to query a different table. You may want to post your code here. -
One issue I see is in your form you're not setting any values for the sizes field <select size="1" name="size"> <option value="Small">Small</option> <option>Medium</option> <option>Large</option> <option>Extra Large</option> </select> You're deifing the labels but no values! For each option (<option></option>) you need to set a value. You've only set a value for the first option. To get the selected value you'd use either $_POST['size'] or $_GET['size'] (depending on your forms submit method). Also when setting session variables do not use the session_register function. session_register("size"); $size = $_SESSION['size']; To set a session variable you'd use $_SESSION['session_variable_name'] = 'some value'; To set thr size session you'd use $_SESSION['size'] = $size; Just remember to call session_start at the top of all pages that uses sessions.
-
You haven't constructed your query correctly. You have a missing ' at the end of your query However you probably don't need to to have the quotes wrapped around {$_SESSION['flyerID']}. If that variable only contains numbers. Only strings should be wrapped in quotes.
-
i need more of an understaning of the following...
wildteen88 replied to son.of.the.morning's topic in PHP Coding Help
$_FILES["file"]["error"] will contain an error code if their is a problem when uploading the file. All possible error codes are stated in the manual here http://php.net/manual/en/features.file-upload.errors.php $_FILES["file"]["size"] stores the files size of the uploaded file. The file size is measured in bytes. This operation here $_FILES["file"]["size"] / 1024 Is dividing (thats what the / stands for) the uploaded files file size by 1024 to convert the file size from bytes to kilobytes (There are 1024 bytes in a kilobyte (KB)) For more information on how PHP handles file uploads have read of the documentation here http://php.net/manual/en/features.file-upload.php -
Only strings should be wrapped in quotes. Change this $queyr = "select * from reference,users where reference.username=users.user_name AND reference.refid = '".$_POST['refid']."'"; to $query = "select * from reference,users where reference.username=users.user_name AND reference.refid = ". intval($_POST['refid']);
-
You'll want to look for some pagination tutorials, such as this one: http://www.phpfreaks.com/tutorial/basic-pagination The core function you'll need to be using is mysql_query. To alter records in mysql you'll use an UPDATE query. To retrieve records from the database you'd use a SELECT query. Here is a tutorial on basic database handling http://www.phpfreaks.com/tutorial/php-basic-database-handling If you're starting out with PHP then I'd stick with the procedural functions. When start to get confident you can move on to the MySQLi class. If you learn OOP you can either modify the MySQLi class to your own needs or create your own database class.
-
That error usually indicates there is a problem with your query. Change this $result = mysql_query("select * from reference,users where reference.username=users.user_name AND reference.refid = '".$_POST['refid']."'"); To $query = "select * from reference,users where reference.username=users.user_name AND reference.refid = '".$_POST['refid']."'"; $result = mysql_query($query) or trigger_error('MySQL encountered a problem<br />Error: ' . mysql_error() . '<br />Query: ' . $query); Run the code and post the error here
-
I believe suge is using the smarty template engine.
-
Using preg_match_all to get content between brackets?
wildteen88 replied to Andrew R's topic in PHP Coding Help
Try the regex pattern ~\(Age ([0-9]+)\)~ -
You can set a cookie with php using the setcookie function. Or you can use sessions
-
Parametrized Queries - Why are they not popular?
wildteen88 replied to bachx's topic in PHP Coding Help
This will all be depended on how efficient your code is and making sure your queries are optimised. Have a read off the PDO function list in the manual http://php.net/pdo However if you're using a MySQL database then it is best to use the MySQL Improved extension. -
This link will be more useful for how sessions work http://www.ruturaj.net/tutorials/php/how-sessions-work
-
Do you mean add bbcode tags (eg ) when the user clicks a button? For this you'll want to use javascript Or to parse bold to bold? For parsing bbcode you'd use regex $str = 'This is some [b]text[/b], bold and [b][u]underlined[/u][/b] [i]italics[/i], [b]bold[/b], [u]underline[/u]'; $str = preg_replace('~\[b\](.*?)\[/b\]~', '<b>\\1</b>', $str); $str = preg_replace('~\[i\](.*?)\[/i\]~', '<u>\\1</u>', $str); $str = preg_replace('~\[u\](.*?)\[/u\]~', '<u>\\1</u>', $str); echo nl2br($str);