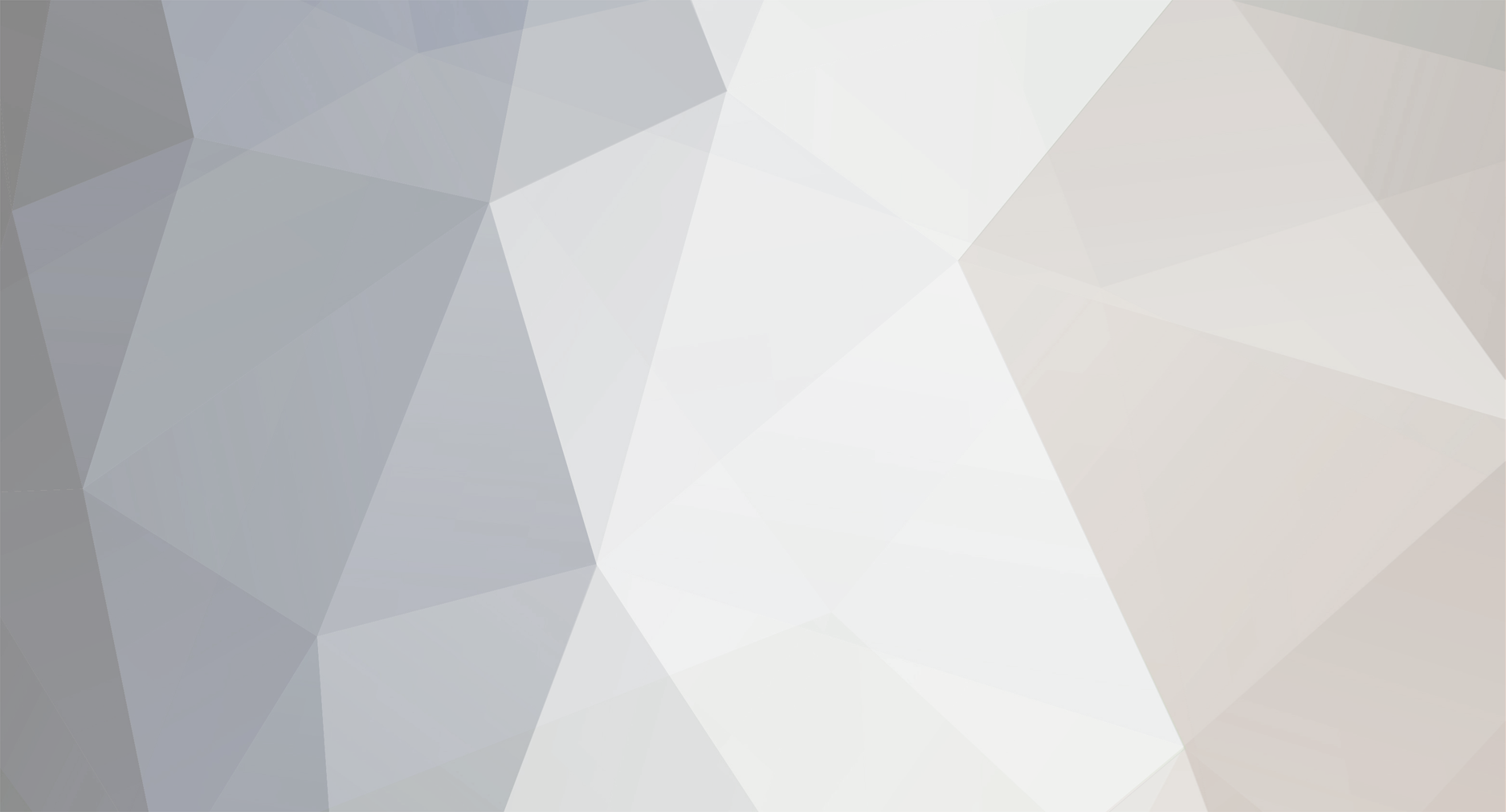
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
If each link is on new line then you can explode on the new line character $links = explode("\n", $_POST['your_text_field_name']);
-
Is it a good idea to htacess admin folder
wildteen88 replied to deansaddigh's topic in Apache HTTP Server
Provided your ip address is static then yes. There is nothing wrong with that. You'll want to look up on the Allow from directive Alternatively you could password protect your admin directive using http authentication. -
For demonstration I created two tables, users and users_info CREATE TABLE `users` ( `user_id` int(11) NOT NULL AUTO_INCREMENT, `name` varchar(20) NOT NULL, PRIMARY KEY (`user_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1; CREATE TABLE `users_info` ( `info_id` int(11) NOT NULL AUTO_INCREMENT, `user_id` int(11) NOT NULL, `item` varchar(100) NOT NULL, PRIMARY KEY (`info_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1; And some summy data INSERT INTO `users` (`user_id`, `name`) VALUES (1, 'name1'), (2, 'name2'); INSERT INTO `users_info` (`info_id`, `user_id`, `item`) VALUES (1, 1, 'item1'), (2, 1, 'item2'), (3, 1, 'item3'), (4, 2, 'item1'), (5, 2, 'item2'), (6, 2, 'item3'), (7, 1, 'item4'), (8, 1, 'item5'); And the PHP code $query = "SELECT u.user_id, u.name, ui.info FROM users u RIGHT JOIN users_info ui ON ui.user_id = u.user_id ORDER BY u.name"; $results = mysql_query($query) or die($query . '<br >' . mysql_error()); if($results) { while($row = mysql_fetch_assoc($results)) $data[$row['name']][] = $row['item']; foreach($data as $name => $items) { echo "<p><b>$name</b>: " . implode(', ', $items) . "</p>"; } }
-
Yes, join is the correct way. You need to add the logic within your while loop to stop if from outputting Both Smith all the time. If you require a code example I'll post one
-
I see you're using short tags (<? ?>). These tags are usually disabled by default. Your server may not have short tags enabled. It is best practice to use the full PHP tags syntax (<?php ?>). Also you should get into the habit of indenting your code. For example if( ) { while ( whatever) { // do something } } Indenting your code makes it much more readable. Also you can easily identfiy where each code block (code between { and }) starts and and end. Rather than if( ) { while ( whatever) { // do something } } Which makes it hard to read.
-
Basically that error usually means you have left of some closing braces }. Make sure your opening and closing braces matchup.
-
using a link to remove a record from a database????????
wildteen88 replied to son.of.the.morning's topic in PHP Coding Help
I didn't say change your ids to x. I meant replace the x with your records id number. <a href="<? mysql_query("DELETE FROM forum_question WHERE id='$id'"); ?>"> You can not pass PHP code within a link. Look at my code example. -
using a link to remove a record from a database????????
wildteen88 replied to son.of.the.morning's topic in PHP Coding Help
The link for deleting the record will be <a href="delete.php?id=x">Delete</a> change X to your records id. Now in delete.php you'd do if(isset($_GET['id']) && is_numeric($_GET['id'])) { $id = (int) $_GET['id']; // dont forget to connect to mysql $query = "DELETE FROM table WHERE id=$id"; mysql_query($query); } You can redirect a visitor using header. But you can only use the function before your output any text/html. -
What do you mean? Variables used within a query will not be unset. So no it shouldn't affect the $id variable To find out which query is causing the error . Change the or die(myql_error()); bits to include your queries, eg $checkenergy = "SELECT energy FROM dogs WHERE id=$dogyay"; $energylevel = mysql_query($checkenergy) or die($checkenergy . '<br />' . mysql_error()); That way you will know which query the error refers to and it'll stop use from asking silly questions.
-
Where is the variable $id defined?
-
How to get the first element in the Associative array.
wildteen88 replied to theITvideos's topic in PHP Coding Help
Thanks for the reply but this returns JPJ instead of 'Joe' How? You sure you typing the code in properly. You should get Joe. -
Oh, I never knew that. Learned something new today.
-
How to get the first element in the Associative array.
wildteen88 replied to theITvideos's topic in PHP Coding Help
Huh? You using that snippet in in this loop? echo "<br/>"; foreach ( $character as $key=>$val ){ print "$key = $val <br>"; } You don't need the loop to echo out an item from an array. This is all you need $character = array (name=>"Joe", occupation=>"Programmer", age=>30, "Learned language "=>"Java" ); echo $character['name'] If $character was a multi-dimensional array (held more than one character) then you'd need to use the foreach loop. -
How to get the first element in the Associative array.
wildteen88 replied to theITvideos's topic in PHP Coding Help
To get the characters name, you'd use echo $character['name'] Or you can use array_shift. Eg $person = array_shif($character); echo $person; But that will remove the first element from the array. -
You'd compare the $row['manager_email'] with the string 'null' <td align="left">' . ((strtolower($row['manager_email']) == 'null') ? $row['manager_email'] : 'N/A') . '</td>
-
By NULL do you mean the data type null. Or do you mean the string 'null'? empty will work if the data type is null. Null stands for no value.
-
now i want to rewrite a friendly url
wildteen88 replied to deansaddigh's topic in Apache HTTP Server
Add / before thumb-circle-active.png -
now i want to rewrite a friendly url
wildteen88 replied to deansaddigh's topic in Apache HTTP Server
You should only need to apply this to the links/images in school_details.php. However there is no harm in doing this for all the links on your sites. -
now i want to rewrite a friendly url
wildteen88 replied to deansaddigh's topic in Apache HTTP Server
You will want to begin all your links with a / too. The same applies to your images etc. -
Change the rewrite rules to RewriteRule ^([a-z]{2})/([a-z0-9_-]+)/?$ $2.php?lang=$1 [NC,L] RewriteRule ^([a-z]{2})/?$ test.php?lang=$1-89 [NC,L] I have limited the first match to only two characters. You may also want to add these lines RewriteCond %{REQUEST_FILENAME} !-f RewriteCond %{REQUEST_FILENAME} !-d After this line RewriteEngine On
-
now i want to rewrite a friendly url
wildteen88 replied to deansaddigh's topic in Apache HTTP Server
Change this <link href="style.css" rel="stylesheet" type="text/css" media="screen" /> to <link href="/style.css" rel="stylesheet" type="text/css" media="screen" /> When the url is http://www.languageschoolsuk.com/school_details/Bath_Academy the web browser is trying to load the stylesheet in http://www.languageschoolsuk.com/school_details/style.css. It is thinking school_details/ is a directory which we know it isn't. Adding the / in front of style.css tells the browser to load the file from the root of the url eg http://www.languageschoolsuk.com/style.css -
Try the following rules RewriteRule ([a-z]+)/([a-z0-9_-]+)/? $2.php?lang=$1 [NC,L] RewriteRule ([a-z]+)/? index.php?lang=$1 [NC,L] Will map site.com/en/dir/ to site.com/dir.php?lang=en Will map site.com/en/ to site.com/index.php?lang=en
-
Streamlining Dreamweaver generated PHP code
wildteen88 replied to MargateSteve's topic in PHP Coding Help
Here is some example code of how you'd output the results with just one query and a while loop. $query = "SELECT * FROM player_season, players, player_positions WHERE date_left > '2011-07-01' AND player_season.season = '104' AND player_season.player = players.player_id AND player_positions.player_position_id = players.position ORDER BY player_positions.position_order, players.position, players.surname ASC"; $results = mysql_query($query); if($results) { if(mysql_num_rows($results)) { $last_player_postion_id = 0; while($row = mysql_fetch_assoc($results)) { // when the players positon id changes output a new heading if($last_player_postion_id != $row['player_position_id']) { echo '<h1>' . strtoupper($row['position']) . '</h1>'; $last_player_postion_id = $row['player_position_id']; } echo '<h3>' . $row['surname'] . ', ' . $row['firstname'] . '</h3>'; } } else { echo 'No results!'; } } -
now i want to rewrite a friendly url
wildteen88 replied to deansaddigh's topic in Apache HTTP Server
Ideally it is best to not have any spaces within your urls. When you are creating the link for the school $name = $row['name']; echo '<h2 class ="schoolname"><a href="school_details/'.$name.'">'.$row['name'].' (click for details)</h2></a>'; You should convert the spaces within the school name to underscores $name = str_replace(' ', '_', $row['name']); echo '<h2 class ="schoolname"><a href="school_details/'.$name.'">'.$row['name'].' (click for details)</h2></a>'; Now you urls will be like site.com/school_details/Bath_Academy Now. In school_details.php when use $_GET['id'] you'll need replace the underscores back to spaces. Like so $school_name = str_replace('_', ' ', $_GET['id'] You will also need to change this part of your query WHERE school.school_id =" .$school_id; to WHERE school.name = '$school_id'"; As you are now passing the school name to the query and not the schools id.