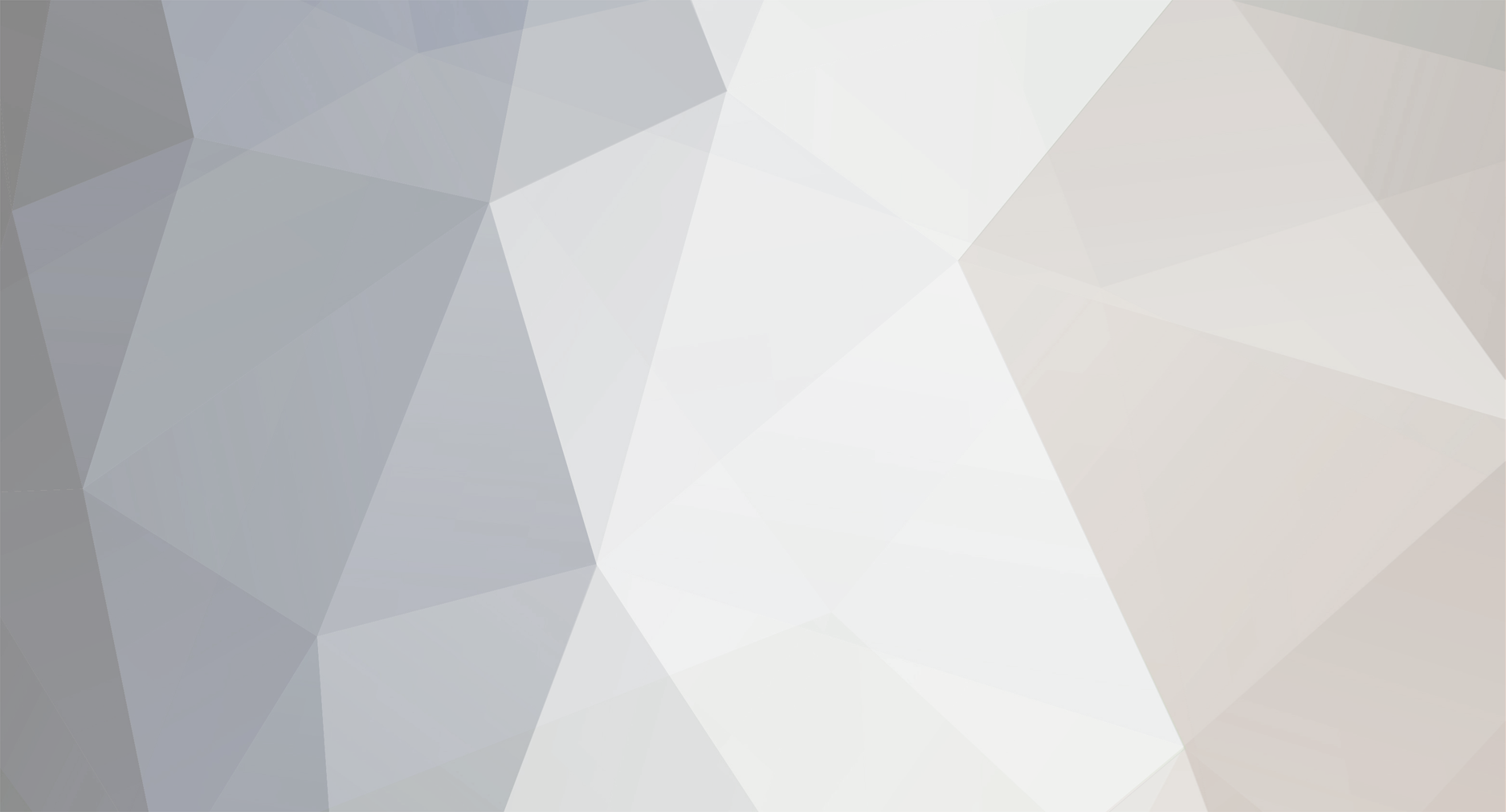
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
With windows use two \\ like so: [i]mkdir("files\\$userdir\\$username", 0777);[/i] Currently PHP is escaping the $ sign on your variables so it creating folder called 'files$userdir$username' it is not parsing the variables. Now with the double backslahes PHP will not escape the $ sign. Also chnaging the CHMOD settings to 0777 is completly usedless on Windows as it doesnt use CHMOD.
-
You said you had access to your php.ini? You just open it up and find the line that says [i]allow_url_fopen = Off[/i] to [i]allow_url_fopen = On[/i] It may not state Off it might be set to 0 instead. Additonally you might be able to use the following in your script: [code]// enable url fopen ini_set("allow_url_fopen", "On");[/code]
-
Try this: [code]<?php include("dogs.inc"); mysql_connect($host,$username,$password) or die("Unable to connnect to mysql: " . mysql_error()); mysql_select_db($database) or die( "Unable to select database: " . mysql_error()); $query = "SELECT song_title FROM Songs"; $results = mysql_query($query) or die("Unable to perform query: <i>" . $query . "</i>: " . mysql_error()); while($row = mysql_fetch_assoc($query)){ echo "$row['song_title']"; } mysql_close(); ?>[/code] Try running your code again. Do you get any errors?
-
You are outputting text/html in your code before you use your header function. You cannot send any output before you use the header function. The following is output: [code]echo '<br>'.date("D, d M Y H:i:s O"); ?> <?php[/code]
-
stripslashes doesnt strip all slashes out of a string, it only strips slashes that is escaping certain characters: For example you had an apostrophe in your name, like so O'reily if you did this: O\'reily the backslash is escaping the apostrophe. Whn you use stripslashes on that name PHP will remove the slash, it'll also remove the slash if you had two backslashes. Such as \\ would be converted into \ If you want to remove slashes completely use str_replace like so: [code]<?php $str = "This //string\\had slashes /\ in\\ it!"; // Only strips out slashes that is escaping characters. echo stripslashes($str) . " - with stripslashes<br />\n\n"; // use str_replace to remove \ and / characters from our string // NOTE: two \'s was used below to stop PHP from escaping the quote mark $str = str_replace(array("\\", "/"), '', $str); echo $str . '- with str_replace'; ?>[/code]
-
Session sets a cookie, which only stores the session id. Or ifcookies are disabled it'll show the session id in the url, The session id is autormatically generated and is unique. The session data itself is stored in locatation specified in the php.ini file Also sessions are secure becuase in order for a malicous hacker to get the contents of the session, they have to be on the same computer to read the cookie which stores the session id. Plus sessions expire when the user closes his browser window or when the session expires. The session expirary time set in the php.ini. The only way to hack into someones session is by session fixation, explained above. [a href=\"http://www.oreilly.com/catalog/phpsec/\" target=\"_blank\"]this book[/a] explains in detail about session fixation.
-
It looks like you cannot specify a url in the parenthesesis, ie: file_get_contents("http://www.mysite.com/filename.php"); Try a relative path instead if the file is on your server. or see if you can turn on [b]allow_url_fopen[/b] setting in the php.ini. Also GoDaddy may have safe_mode trun one which may be causing this.
-
You'll want to lookinto nl2br function ie: [code]<?php $str = "this text\nhas line\nbreaks in\n it!"; // convert carriage returns into line breaks (<br />) echo nl2br($str); ?>[/code] Also if you want to only allow paragraph tags you can use a function called [a href=\"http://www.php.net/strip-tags\" target=\"_blank\"]strip_tags[/a]. For example: [code]<?php $str = "this text<p>is only <b>allowed</b></p>paragraphs!"; // strip all tags from $str but keep < p></p>! echo strip_tags($str, '<p>'); ?>[/code] Hope that helps.
-
Automate Php Script Execution By Time Interval On Windows
wildteen88 replied to thepip3r's topic in PHP Coding Help
For windows its called Scheduled Tasks I belive. -
Yes thats ok for the First one, now you need to do it for the next three if/else statements. Make sure you have $_POST['s1'] to $_POST['s2'] for the secound if statement, $_POST['s3'] to the third if statement and so forth. So your code looks like this: [code]if (isset($_POST['s1']) && !empty($_POST['s1'])) { //name of field 1 $MailBody = 'Name : ' . $_POST['s1'] . "\n"; } if (isset($_POST['s2']) && !empty($_POST['s2'])) { //name of field 2 $MailBody = 'Company : ' . $_POST['s2'] . "\n"; } if (isset($_POST['s3']) && !empty($_POST['s3'])) { //name of field 3 $MailBody = 'E-mail : ' . $_POST['s3'] . "\n"; } if (isset($_POST['s4']) && !empty($_POST['s4'])) { //name of field 4 $MailBody = 'Subject : ' . $_POST['s4'] . "\n"; }[/code]
-
I'd use sessions. That is the best secure method as the user is oblivious of any data being passed from page to page. It keeps the url tidy too.
-
The secound error message is a result from the the first error message. If you sort out the file_get_contents error message then the email will be sent. Also when dealing with files, i'd do this: [code]// check whether file exists first if(file_exists("blockip.txt")) { // if it exists get the contents of the file $file_contents = file_get_contents("blockip.txt"); }[/code]
-
PLease note that PHP Help forum is for asking questions about PHP and for getting help with your scripts that you are developing. It is not for getting help with third party scripts that has been developed by someone else.
-
I would not advise you to stored session data in a cookie, as cookies are stored in the users tmp folder which anyone that uses the computer can access and read all cookie data. Where as a session are stored on the server, where the client can not read. You dont have to use what the book says for session. You can use sessions with its defualt behaviou which is saving the session data to a file, this already setup: [code]<?php session_start(); $_SESSION['userID'] = USER_ID_HERE; ?>[/code] Then whenever you need to access the userID session make sure you have session_start(); at the top your script and use $_SESION['userID'] to access the userID Some more advanced PHP programmers prefer to write thier own session handler, which is what your book is teaching you/telling you. However PHPs defualt session behaviour is fine. If you want to use cookies then you can use the [a href=\"http://www.php.net/setcookie\" target=\"_blank\"]setcookie function[/a]
-
You still havn't read my post correctly. Delete the following: [code]if ($s1 == ""){ //name of field 1 } else { $MailBody = "Name :$_POST['name']; }[/code] And replace the baove with this: [code]// check that $_POST['s1'] isset and that $_POST['s1'] is not empty if (isset($_POST['s1']) && !empty($_POST['s1'])){ //name of field 1 $MailBody = 'Name : $' . _POST['s1'] . "\n"; }[/code] Thats the correct way!
-
You have forgot to add a closing } after this: [code]elseif ($gender=='F') { $message='<b><p>Good Day , Madam!</p></B>'; } else { $gender=NULL; echo'<p><b>You Forgot to enter your gender!</b></P>'; } } // this was missing[/code]
-
Are you talking about how to play the game, or are you wanting to create a PHP Poker game? If you are aksing about how to play it then this is not the correct forum.
-
Prehaps having a read of [a href=\"http://www.phpfreaks.com/forums/index.php?showtopic=95443\" target=\"_blank\"]this thread[/a] may help.
-
Why not just update the current session the GUEST user is using? Rather than destorying the session and creating a new one? Someting like this: [code]<?php session_start(); if($_SESSION['logged_in'] != '1') { $_SESSION['user'] = "GUEST"; } else { // reset session as a blank array: $_SESSION = array(); //get user credentials // reset the session data $_SESSION['logged_in'] = '1'; $_SESSION['user'] = $username; } ?>[/code] Also session_destory clears the data in the session file and sets that session id as invalid. It does not delete the session. If you want the session files to be deleted automatically when they expire you'll want to look into Garbage Collection. Garbage collection is control by [i]session.gc_probability[/i] and [i]session.gc_divisor[/i]. Look these up over at [a href=\"http://www.php.net/session\" target=\"_blank\"]http://www.php.net/session[/a]. These settings can set using ini_set.
-
bilis_money and DaveLinger your posts have been deleted. bilis_money if you wish to chat to Barand please use the PM feature.
-
Still shouldn't have that big gap. That is defualt behaviour. Untill the page gets more content then it'll display correctly.
-
Pehaps they are using an old IPB security exploit. Prehaps upgrading the board agin to the latest release, IPB2.1.6 might help keep them obey. Also phpfreak. Should this of been posted in the Admin forum? And nice see you around too [img src=\"style_emoticons/[#EMO_DIR#]/smile.gif\" style=\"vertical-align:middle\" emoid=\":smile:\" border=\"0\" alt=\"smile.gif\" /]
-
You saying that this is a previous script from someone and that they didn't have session_start at the top of their pages? If thats the case then they most probably had setting called [b]session.auto_start[/i] enabled. This setting starts the session on every page request without having to use session_start().
-
And that isn't sending the email? Make sure you check that emails are not being sent to your Junk/spam folders as emails sent with PHP usually get treated as spam. Also make sure the server you are sending the email from has an SMTP server installed/configured. Otherwise no emails will be sent, regardless of what your if statement returns. Mail doesnt check the success of the email, it just checks that your have filled in the parameters correctly.