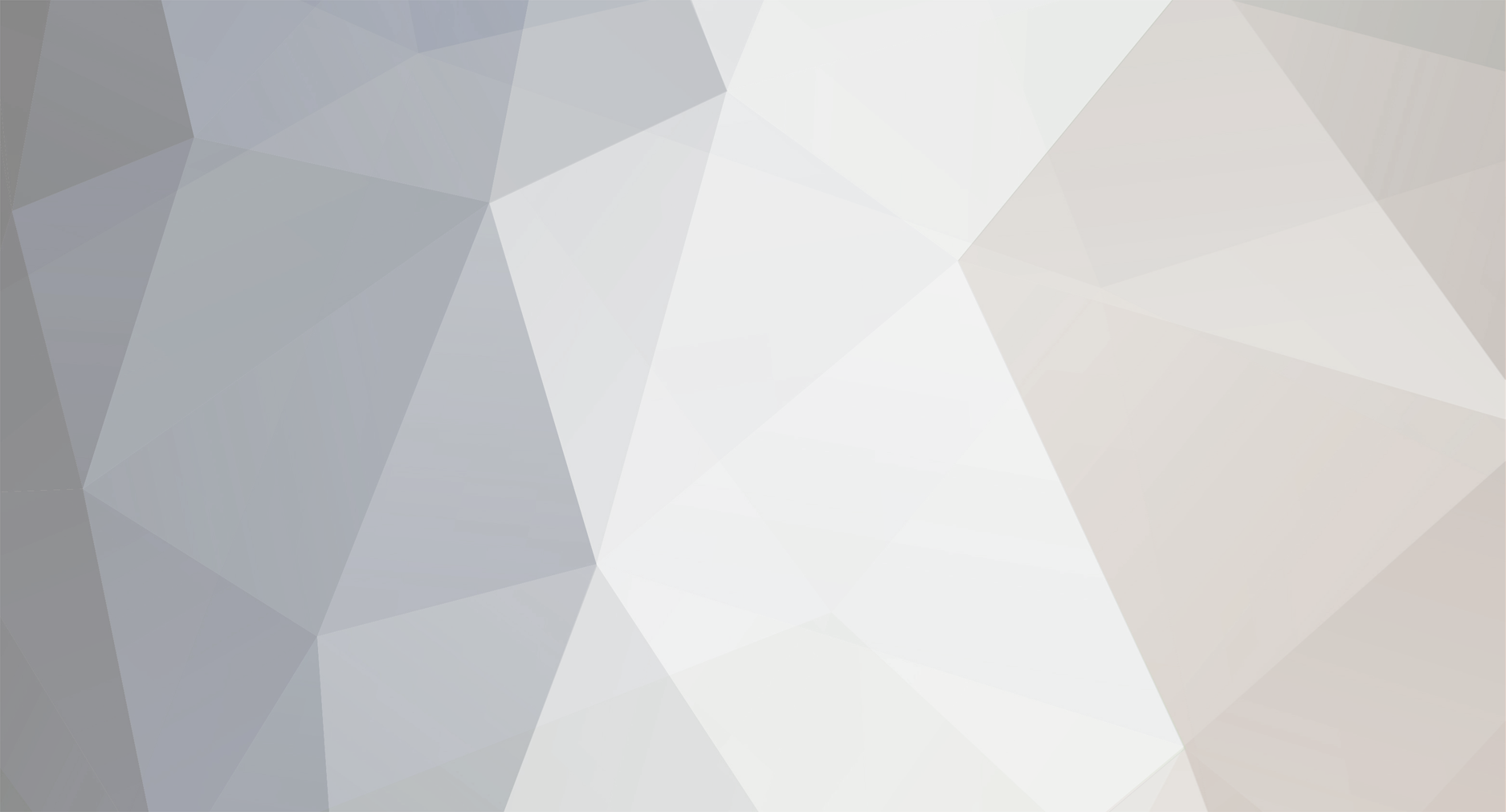
xyph
Staff Alumni-
Posts
3,711 -
Joined
-
Last visited
-
Days Won
1
Everything posted by xyph
-
You need to group conditionals when using a combination of AND and OR, or the engine will have to make assumptions.
-
I'd suggest Plupload. I've used it many times, and it's extremely customizable. They have a great default interface, but the API is powerful enough to create your own custom look and feel (assuming you know a little JS) with ease. http://www.plupload.com/example_custom.php
-
Curly braces? Dot notation? Seems more like JavaScript
-
You sure you're smoking cannabis? I've never heard of accuracy and efficiency being a side effect. Munchies and zoning out on the other hand?
-
Here's how you'd do it with sessions. <?php session_start(); error_reporting(E_ALL); $domain=$_GET['domain']; $update=$_GET['update']; if ( isset($_SESSION['domains'][$domain]) && $_SESSION['domains'][$domain]['expire'] > time() ) { $quota_avail = $_SESSION['domains'][$domain]['quota_avail']; $quota_used = $_SESSION['domains'][$domain]['quota_used']; $bandwidth_avail = $_SESSION['domains'][$domain]['band_avail']; $bandwidth_used = $_SESSION['domains'][$domain]['band_used']; } else { // Get domain info $dom_return = explode("\n", shell_exec("sudo /usr/share/webmin/virtual-server/list-domains.pl --multiline --domain $domain")); // Strip out and save quota details $quota = array_values(preg_grep("/Server quota/", $dom_return)); $quota_avail_tmp = explode(":", $quota[0]); $quota_used_tmp = explode(":", $quota[1]); $quota_avail = $quota_avail_tmp[1]; $quota_used = $quota_used_tmp[1]; // Strip out and save bandwidth details $bandwidth = array_values(preg_grep("/Bandwidth/", $dom_return)); $bandwidth_avail_tmp = explode(":", $bandwidth[0]); $bandwidth_usage_tmp = explode(":", $bandwidth[3]); $bandwidth_avail = $bandwidth_avail_tmp[1]; $bandwidth_used = $bandwidth_usage_tmp[1]; $_SESSION['domains'][$domain]['expire'] = time() + 300; $_SESSION['domains'][$domain]['quota_avail'] = $quota_avail; $_SESSION['domains'][$domain]['quota_used'] = $quota_used; $_SESSION['domains'][$domain]['band_avail'] = $bandwidth_avail; $_SESSION['domains'][$domain]['band_used'] = $bandwidth_used; } ?> Simply handle any expiry times within the session itself.
-
So the design isn't completely daffy? Or am I not giving enough information to make it clear? Yeah, I guess it's easy enough to specify extra constant columns in the UNION.
-
PHP MySQL, pop up box when a new row is inserted in mysql database?
xyph replied to Lukeidiot's topic in Javascript Help
You need to run an AJAX call that grabs the id of the 'newest row' from the database. Run this every 5 seconds or so, and store the result in a JS variable. If the value that you fetch is different than the value you've previously stored, you know an update has happened. -
I'm wondering what you guys think would be the best way to accomplish this. It's more of a design question than any specific query. I have a client that I'm building a file-management system for. They require a user/group permission system, and it's a little archaic. I'll try to explain Files are 'placed' in a folder using an alias to that file. Any file can have an alias in any number of folders. Folders/subfolders are placed in 'cabinets' using an alias to that folder/subfolder. Any folder can have an alias in any number of cabinets or folders. Permissions are applied to aliases, not the files/folders themselves. Different aliases of the same file could have different permissions. Permissions can target either a user, or a usergroup. As of now, I have 4 tables to link user/group permissions to file/folders. user_to_file_permissions user_to_folder_permissions group_to_file_permissions group_to_folder_permissions I like this method, because I can easily keep foreign key constraints. I don't like it though because getting them all into a single query could be quite ugly (if possible). I'm guessing I'd use UNIONs and possibly some if cases to help determine which values came from which tables. In the case of conflicting permissions, the most permissive will be used. Is there a better way to do this? Should I provide more details? Ideally, I'd like to keep foreign key constraints available, but if it's not possible in a more robust or efficient system, I can program the clean-up manually
-
Foreach doesn't work this way. $sth->fetch(PDO::FETCH_ASSOC) will only be executed once. You want to use a while loop.
-
Preg_match line and break up parts of a line into variables
xyph replied to michael.davis's topic in PHP Coding Help
<?php $data = file_get_contents('http://www.srh.noaa.gov/productview.php?pil=OHXRWROHX'); $city = 'Nashville'; // NASHVILLE MOSUNNY 74 60 61 CALM 30.15R // BURNS* N/A N/A N/A N/A S1 N/A $expr = "%$city*?\s+ ([a-z/]+)\s+ (n/a|\d+)\s+ (n/a|\d+)\s+ (n/a|\d+)\s+ ([a-z0-9]+)\s+ (n/a|[0-9.]+[a-z])%ix"; preg_match( $expr, $data, $match ); print_r($match); ?> -
-
Deleting XML-entries with checkboxes echoed by foreach
xyph replied to Inquiescent's topic in PHP Coding Help
Is there any reason you're using an XML file for this? You'd be much better off using something like SQLite if you need a flat-file database. -
What happens if the search term includes a line-break? Or if the term is two words, and they happen to reside at the end of one line, and the start of the other?
-
Same section, under 'More examples to demonstrate this behaviour:' // The following is okay, as it's inside a string. Constants are not looked for // within strings, so no E_NOTICE occurs here print "Hello $arr[fruit]"; // Hello apple // With one exception: braces surrounding arrays within strings allows constants // to be interpreted print "Hello {$arr[fruit]}"; // Hello carrot print "Hello {$arr['fruit']}"; // Hello apple It's not necessarily encouraged, but I can't find anywhere that it's directly discouraged. This will never produce an undefined constant error. It's an active feature of the language. <?php ini_set('display_errors',1); error_reporting(-1); $arr['foo'] = 'PHP'; echo "I love $arr[foo]"; // Output: I love PHP // No errors. ?> I actually write it in the curly syntax as well, I just find it hard to argue with someone who picks the 'cleaner' route, and ours definitely isn't that
-
Short answer, no. You can read 'chunks' of a file into memory at a time, and search within the chunk. If for some reason a match is split between chunks though, you may not get correct results.
-
INTOFILE would most likely be more efficient, but would be MySQL-specific. If you want to take full advantage of the abstraction PDO gives you, I'd bring the data into PHP and parse it there - you can easily do this with ANSI/ODBC standard code. If you only plan on using MySQL, and want to maximize efficiency around this, then use INTO OUTFILE
-
Then do it that way, I suppose? Or write a CLI-based script that accepts a flag/argument containing the row's ID and e-mails all your subscribers? Run this script after you've added a post, manually giving it the post's unique ID. I'm not sure if you can create a MySQL trigger that will run an outside application, that might be worth looking into as well.
-
generating unique password without going to db?
xyph replied to stijn0713's topic in PHP Coding Help
Multi-threaded software. Multi-core computers. It's very possible to do multiple things at the exact same time with modern computing. My understanding is that even with multithreading things are not truly happening at the same time. Only the processor is multithreaded and things like disk reading/writting and ram access are not. You're thinking extremely low-level. At a higher level, you can be both reading a file into memory, and writing from memory 'at the same time' in the sense that both actions start before either completes. Even at the low level, the limitation would only be hardware and OS operation. If you've only got 1 pipe, or the OS only knows how to use 1 pipe, you're restricted to that. So, in this same sense, unless you've specifically placed a lock on a shared resource, it's very possible for any given request to begin being processed while the server is in the middle of processing another. If this weren't true, race conditions wouldn't exist. -
That example is specifically targeted at classes designed to hold huge amounts of data, where a getter/setter for each mutable property could result in thousands of methods needing to be held in memory. In that case, it's better to have a generic getter/setter for all properties, rather than one for each or none at all, IMO.
-
Need suggestions for a user-specific content page
xyph replied to desigi's topic in Application Design
How would this differ from a web application? Are these smart phones capable of running apps, but not browsing a website? An amazing part about web-based applications are the fact that they are for the most part platform independent. There's no need to maintain both an Android and an iOS version, and you aren't alienating those who don't have smart phones. Time and resources will have to be spent regardless. -
So why are you manually entering the database information. If you're going as far as having subscribers and want to send out mass e-mails on updates, why not create a front-end to update the blog, and have it send the e-mails.
-
Where is it specifically discouraged? I thought this as well, but in the manual, there's a very specific example of it http://www.php.net/manual/en/language.types.string.php#example-78 and no mention of a 'preferred' or 'better' method. The quotes are only required when wrapping the array in curly-braces. I'm not attacking your advice, just wondering where it comes from. Overall, I agree with your methods and always use curly-braces+quotes for associative/string keys and was actually surprised when giving similar advice only to not find it in the manual. It's actually quite clean to leave the curly-braces/quotes out of the string, if possible <?php $arr['foo'] = 'bar'; // Mmm, super clean and 100% valid/supported echo "I sure love $arr[foo]"; // Still clean, but I think the above still has it beat echo "I sure love {$arr['foo']}"; ?> This is incorrect for string parsing. <?php // foo - Always evaluated as an associative array key echo "I sure love $arr[foo]"; // foo - Constant, assumed string if constant doesn't exist w/ notice echo "I sure love {$arr[foo]}"; ?>
-
This topic has been moved to Application Design. http://forums.phpfreaks.com/index.php?topic=364194.0
-
Need suggestions for a user-specific content page
xyph replied to desigi's topic in Application Design
I'm not quite sure what you're asking. Please be more specific with your issue. All I gather from this post is you want to learn PHP and web application design but don't know where to start.