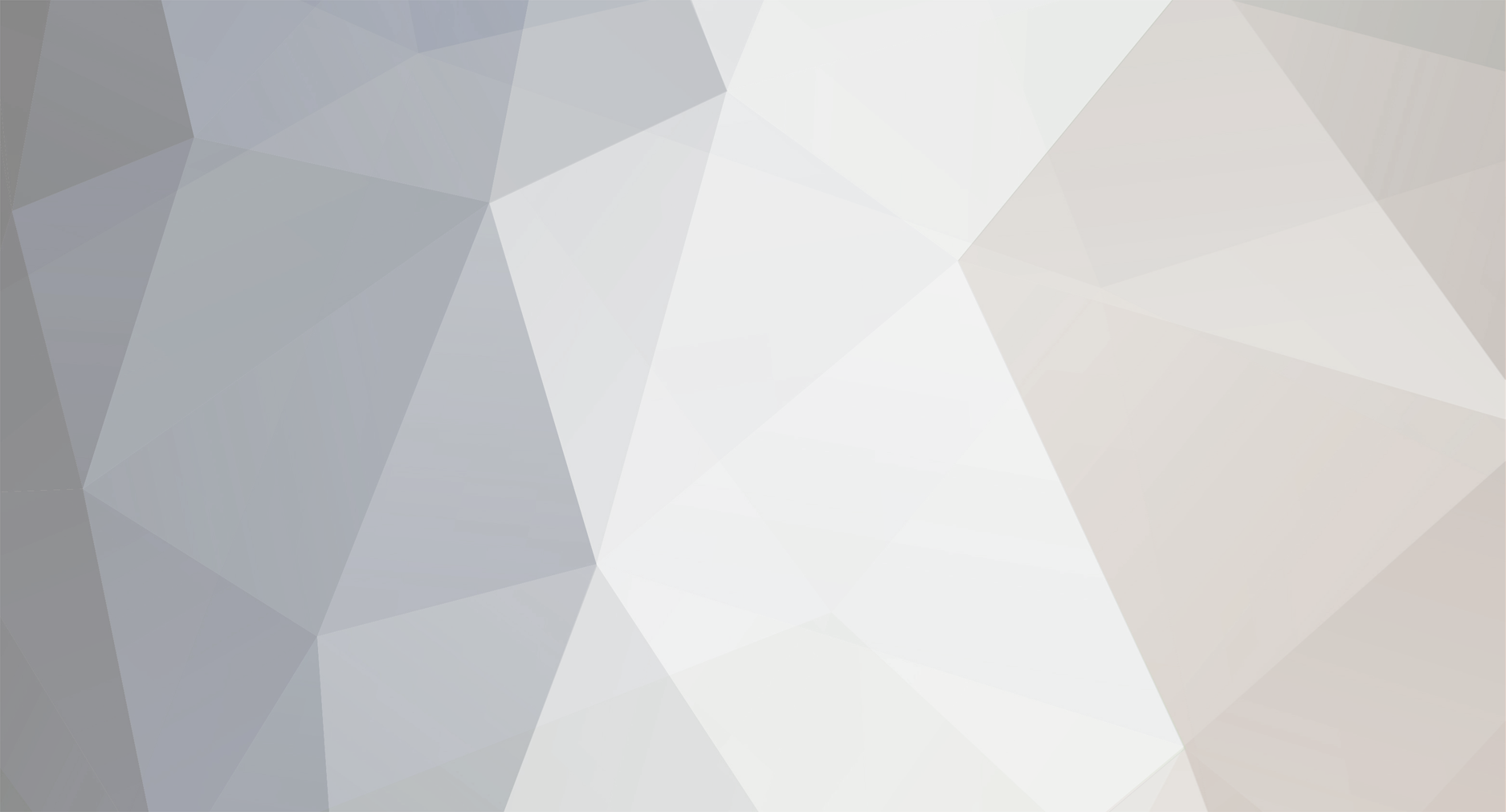
micah1701
-
Posts
613 -
Joined
-
Last visited
Posts posted by micah1701
-
-
Yeah thats how i would do it. That whay the only thing paypal needs to send your back is the ID of the member when the paiement is done.
agreed, in fact, in your database table, you don't even need a "paid" or "unpaid" status. Just have a field for the transaction ID. If the field is blank in your database, you know the status is "unpaid"
-
eeek. that doesn't sound like a good database schema. within a year you'll have 365 tables in your database.
you really just need a table with a few fields, like:
ID (the incremental integer row identification number)
date (the date that a court will be booked)
start_time (the time that the court will be booked)
end_time (the time that this booking ends)
court (the court name - or better yet, the ID value to a court listed in another table of "courts")
user (the user who has booked the court - or again, the ID value to a list of users and uses in another table)
then, you can query your database any way you want.
When is Court 3 being used on Oct 25?
"SELECT `user` FROM table_name WHERE date = '2010-10-25' "
Is Court 3 availabe at 2:45 on October 25?
"SELECT `ID` FROM table_name WHERE date = '2010-10-25' AND start_time < 2:45 AND end_time > 2:45"
etc...
(and really, you don't need that `date` column either if the start and end time columns use a full date/time format)
-
I hate to be one of those people who writes a comment that doesn't answer your question.... but....
you're better off saving all the keywords in a database. If you used an array you would need to then convert it to a string and store it in a flat file somewhere on your site, then when you wanted to view the data, convert it back to an array and parse through it programatically. A database would be much easier and provide simple searchability as well.
-
Ok, so I didn't test any of this, but here is what I'm envisioning.
so on site 1:
<?php start_session(); $_SESSION['auth_token'] = rand(10000,99999); $_SESSION['username'] = "Joe_blow"; echo '<a href="http://www.site2.com/login.php?session_id='.$session_id.'&auth='.$_SESSION['auth_token'].'">click here to log in to site 2</a>'; ?>
on site 2, "login.php"
<?php session_start(); $get_session_data = file_get_contents('http://www.site1.com/pass_session.php?session_id='.$_GET['session_id'].'&auth='.$_GET['auth']); if($get_session_data == "fail"){ exit("ERROR: Could Not Log In From site1.com"); }else{ $_SESSION['username'] = $get_session_data; header("Location: /welcome-page.php"); } ?>
back on site 1, the page "pass_session.php" page, called from site2 in the above code, should look like:
<?php session_id($_GET['session_id']); // load the session session_start(); if($_GET['auth'] != $_SESSION['auth_token']){ exit("fail"); }else{ echo $_SESSION['username']; // or whatever other value you want to pass to site2. } ?>
Hope that helps!
-
the problem is that your PHP script has now just logged itself into the 2nd site, not your user's browser.
your better bet is, send the user to the 2nd site and pass a token (either as a $_GET or $_POST) that both sites recognize.
I'm no expert so some one probably has a better way to do this, but for example, you could send the user from site1 to site2 with site1's php SESSION_ID as the token. Then, once on site2, a php script runs a cURL script back to site1, sending along this session id (and probably some other authenticaion values to prevent XSS hacking) and receiving the session data from site1.
-
I might not be completely understanding what you're doing with the foreach loops inside a foreach loop - but I would think you could accomplish the same security check by using the in_array() function instead. somthing like:
<?php $page = "my-page"; $allowed = array("my-page","some-other-page","etc..."); if(!in_array($page,$allowed)){ exit("You do not have permission to view this page!"); } ?>
-
I recently noticed in an e-mail i received in my gmail account showed the line "Signed-by: domainname.com" along with the simplified header information. I became curious to find out more and learned a bit about DomainKey-Signatures and e-mail authentication. So now I want to implement this in my own mail sending php applications.
I have an app that uses the phpmailer class to send multi-part e-mails. I want to modify the headers so that email clients can validate the legitimacy of the mail they receive from my site.
So, where do I start? I have an SSL certificate for my site, do I need to use that at all?
Thanks for any help pointing me in the right direction
-
<?php $columns = array("category","manufacturer","model","type"); $where = ""; foreach($columns as $col){ if($_POST[$col] != ""){ $where.= "$col = '$_POST[$col]' AND "; } $where = $rtrim($where, " AND"); //trim off that last "AND" $sql = "SELECT * FROM sales $where"; ?>
-
wrapp all your search code in this:
if($_POST['category'] != ""){
// all your code here
}
then it will only run your code if the post "category" value is NOT blank
-
I don't believe you can modify the users settings in javascript, however, you may be able to use some CSS to help
in your page "shortview.php" add...
<style type="text/css" media="print"> body{page:landscape} </style>
and see if that helps.
-
With 1.x versions of CKFinder, I've had great success implementing the file browsing application by simply doing something like:
<button id="saveImage">Browse...</button> <script type="javascript"> $(document).ready(function() { $('#saveImage').click(function() { CKFinder.Popup( { BasePath : '/ckfinder/', SelectFunction : setImageFilePath, startupFolderExpanded: true }); return false; }); }); </script>
But swapping out version 1.4 for 2.0, this doesn't work and I get the error "CKFinder.Popup is not a function."
If I call CKFinder without jQuery using the standard code it works, so I know its there. And its finding the CKFinder object.
alert(CKFinder.version);
this returns "2.0.4" so I just don't get what I'm missing or doing wrong.
And, I know I have jQuery working because I'm using it elsewhere in my app. In fact, when I do this
<textarea name="editor" id="editor">wysiwyg</textarea> <script type="text/javascript"> $('#editor') .ckeditor(function() { CKFinder.SetupCKEditor(this,'/ckfinder/'); }, { skin : 'office2003' } )}; </script>
it loads CKEditor, just without CKFinder (and gives the error "CKFinder.SetupCKEditor is not a function")
Any ideas on what I'm missing or doing wrong?
This has been driving me nuts all week and I've had very little luck with the documentation or searching other forums.
-
modify your security script with something like:
<?php session_start(); if(!isset($_SESSION['loggedin'])) { die(Access to this page is restricted without a valid username and password); } //add this: $url_parts = $_SERVER['PHP_SELF']; //returns "/path/to/current/page/username.php" $filename = array_pop('/',$url_parts); //returns "username.php" if ($_SESSION['username'].".php" != $filename){ die("You are not allowed to view another user's page"); } ?>
-
add
sleep(30);
at the end of the loop before it restarts to send the next message.
its running as a cron you said, so its not like you're waiting for your browser to load for 5 minutes to see a success result.
-
<?php if (isset($_GET['cat-id'])) { $id = $_GET['cat-id']; }else{ $id = 'your-default-category-id'; } ?>
-
here's what i do.
i have a search box with is a form that sends the search keywords as a get variable, so when the user hits search, it goes to something like: my-site.com/search?q=These+are+my+keywords
then in php i do something like:
<?php $words = explode(" ",$_GET['q']); $where = "WHERE "; foreach ($words as $word){ $where.= " column_name LIKE '%".$word."%' OR"; } $where = rtrim($where,"OR");// remove that last "OR" operator $sql = "SELECT * FROM table_name $where"; $result = mysql_query($sql); // ta-da ?>
-
I didn't look through all your code to see what else could be causing your problem but the first thing that sticks out is that in:
<?php function saveImage($chart_url,$path,$file_name) { if ( (!file_exists($path.$filename)) || (md5_file($path.$filename) != md5_file($chart_url)) ) { file_put_contents($path.$filename,file_get_contents($chat_url)); } return($filename); }?>
Notice the variable name in the functions parameters list is "$file_name" but in the rest of the function, you're looking for a variable named "$filename" (without the under_score).
Trying replacing "$file_name" with "$filename" and see if that helps. You may have more problems after that, but its a start
-
<a href="/path/to/your/file.doc">Download File</a>
-
closing the browser kills the session anyway. unless you're talking about cookies?
-
well, at what point in your code are you figuring out which user they are? Like, how do you know that they are user1 or user2?
it looks like the user name they're providing is their email address. (I'm assuming this because I see the line that says: $user_cond = "user_name='$user_email'"; )
so, assuming that the variable $user_email is how you are uniquly identifying the user, you would change the previously mentioned header line to:
<?php header("Location: ".$user_email.".php"); ?>
if the person enter their username as "user1" and now the variable $user_email = "user1" then the above header() function will forward them to a page called "user1.php"
if $user_email contains their actual e-mail address, then they'll be forwarded to a page like "email-name@domain.com.php"
-
towards the end of your code you have a line that reads:
header("Location: myaccount.php");
this is whats forwarding the user to the page "myaccount.php"
if you want each user to have their own page to go to, set this to something like:
<?php header("Location: ".$full_name.".php"); ?>
that said, this really isn't the best way to handle the issue.
you should create your myaccount.php to check for who the logged in user is and load content as needed.
-
sure, just format your output as a comma separated list and set your headers to output as a text file
<?php header('Content-Type: text/plain'); // some stuff to get your object of sql $results foreach($results as $row){ echo $row['column_1'].",".$row['column_2'].",".$row['column_3'].",."$row['etc']."\n"; }?>
-
you are correct that __FILE__ seems to be what's causing the problem:
The full path and filename of the file. If used inside an include, the name of the included file is returned. Since PHP 4.0.2, __FILE__ always contains an absolute path with symlinks resolved whereas in older versions it contained relative path under some circumstances.you need another way to pass the file's absolute path to the function containing the include.
like:
1)
<?php function options_page_fn($file_path){ include 'options_form.php'; } ?>
2) In your included file, change __FILE__ to $file_path.
3) call your function like this:
echo options_page_fn(__FILE__);
-
also, mysql_real_escape_string() only works inside the mysql_query().
so doing
this wont work:
$sql = "INSERT INTO table (col1) VALUES ('".mysql_real_escape_string("my text")."'"; mysql_query($sql);
but this will:
mysql_query("INSERT INTO table (col1) VALUES ('".mysql_real_escape_string("my text")."')");
someone correct me if i'm wrong
-
you might have some luck using the array_multisort() function
http://us2.php.net/manual/en/function.array-multisort.php#97766
PHP, MySQL and PayPal
in PHP Coding Help
Posted
sure thing, use mysql_insert_id() to retrieve the last inserted ID.