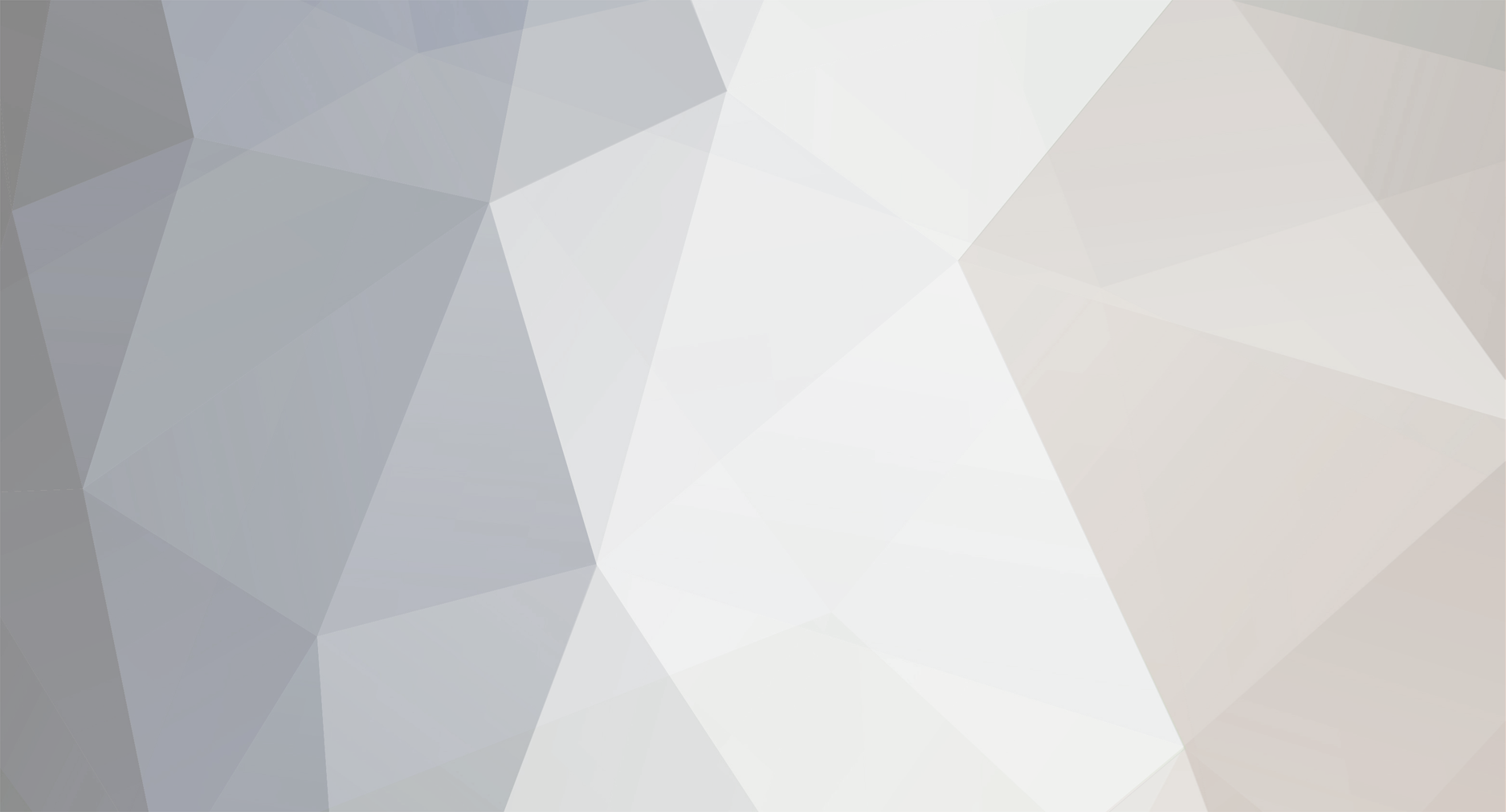
micah1701
-
Posts
613 -
Joined
-
Last visited
Posts posted by micah1701
-
-
neil beat me to it... but I typed all this out so I'm posting anyway
<?php foreach($name_of_your_array as $key => $val){ echo $key; // this is the name of the row to be updated echo $val; // this is the value of what needs to be updated $sql = "UPDATE tablename SET column_name = '$val' WHERE row_id_column_name = $key LIMIT 1"; echo $sql; } ?>
-
on line 17 (if I counted backwards correctly)...
if($result = 0){
needs to be:
if($result == 0){ //with 2 equal signs
Instead of checking if $result is equal to zero, you're re-assigning the value of $result to 0.
-
use a "flag" when looping through your results.
if the flag is set to the current date in the loop, don't show the div again. Otherwise, show the div and update the flag for the next time through the loop.
<?php // ...all the stuff in your while loop if($last_date_displayed != date("d F Y",strtotime($created)) ){ $last_date_displayed = date("d F Y",strtotime($created)); echo '<div>'.$last_date_displayed.'</div>'; } echo '<div style="width:631px;height:auto;clear:both;">'; echo '<div style="width:86px;height:20px;float:left;text-align:right;padding-right:14px;'.$fcol.'">'.$nfrom.'</div>'; echo '<div style="width:427px;height:auto;float:left;padding-right:14px;'.$fcol.'">'.$message.'</div>'; echo '<div style="width:90px;height:25px;float:left;clear:right;color:#999999;">'.$created.'</div>'; echo '</div><div style="width:631px;height:14px;clear:both;"> </div>'; } ?>
-
you could make an array of the keys and values you want, like this:
<?php $letters = array(0 =>"a",1=>"b",2=>"c",3=>"etc"); ?>
then if you have 4 numbers, like 3102 you would just do:
<?php $alpha_string = $letters[3].$letters[1].$letters[0].$letters[2]; echo $alpha_string; ?>
-
are you trying reload the page every hour? if so, you need to use a client-side script such as javascript, not PHP which runs server side. Your script will load once and then output the static text to the browser and nothing else will ever happen unless some script running in the users browser (not on your server) tells the browser to reload the page.
-
per kenrbnsn point, just take the excel file they give you and resave them as CSV or Tab-Delimited files.
-
if you just want to change the URL from ?id= to ?name= then all you need to do in your PHP code is change $_GET['id'] to $_GET['name']
or am I not getting what you're trying to do?
-
to turn 2 into 02 check out the str_pad function: http://us.php.net/manual/en/function.str-pad.php
$month = 2;
$month = str_pad($month,2,0,STR_PAD_LEFT);
echo $month; // returns: 02
-
Wow, Thanks Alex. Thats perfect. I've never used the callback function with a preg_replace. Very nifty! Thanks for showing me something new
-
I've used regex a bit in the past with great success but I'm a little stumped on how to do something.
I basically need to do a preg_replace withing another regex.
I Need to replace the spaces between words with under_score characters, but only for spaces within quotes, not for all the spaces in the string.
In other words, I need this:
Here is a long "test string that" also contains quotes. It actually has "multiple quotes"to look end up looking like this:
Here is a long "test_string_that" also contains quotes. It actually has "multiple_quotes"could someone point me in the best direction?
thanks!
-
you've gotta watch your apostrophes.
drop the single quotes around 'myusername'
VALUES (Null,'$_SESSION[myusername]','$_POST[logdate]','$_POST[type]','$_POST[totaltime_mins]', '$_POST[total_distance]','$_POST[notes]')";
-
Thanks premiso, that was the answer I was looking for!
-
This isn't so much a coding question as a theory/best-practices question, but I wasn't sure where else to post it.
A while back I built an application for a client of mine which grabs some finance data from yahoo! and parses the numbers to give him some stock information. I used something like $data = file("http://finance.yaho.com/whatever?my=paramaters"); and all worked fine until the other day. He called me in a panic that his whole site was crashing and it was my application's fault.
I quickly realized that the problem was that his host, Network Solutions, turned off the allow_url_fopen directive without informing him. I told him to tell them to turn it back on... of course, they wouldn't do that because he's on a shared IP with a thousand other websites and they now consider it a security risk.
They recommend I just use cURL instead, which I did and all is well with the world again.
But it leaves me wondering, how is cURL any safer then using file()? Both grab info from 3rd party sites.
-
In light of my last post, I added a php sleep() call to function to purposely slow it down by several seconds and it still worked fine - showing there's no correlation between jQuery/javaScript and the speed it takes PHP to process the .get() request (which I suspected).
Thus, this must be a CakePHP issue. Topic Closed. Thanks for letting me think "out loud" by posting this here.
-
UPDATE: The PHP script that I'm linking to (in my example it was "updateSessionArray.php" has been running inside a CakePHP invornment.
I moved it out of cake and, not only does it run faster for all ajax actions but it seems to be working fine now. When running in Cake it was taking a full 1 to 3 seconds to get my results back but now its near instant.
So my original problem still stands (as I'd like to keep the PHP page in the cake framework) but I think this helps narrow down the problem to one of two things:
Either 1) Its either CakePHP -- either putting some sort of limit on the number of requests or perhaps trying to cache results?
or 2) its a jQuery/JavaScript issue having to do with the amount of time it takes to process the .get() function while waiting for a long response.
Any thoughts in light of this revelation?
-
the PHP snippet you inserted should create a string that looks like the text in your old Array that you had to create mannually.
after the sinppent of PHP, you need to echo out that text into your javascript like:
rndimg = new Array(<?php echo $fileList ?>);
After you've made that change, refresh your browser and look at its source code. The javascript Array() should look like the old one that you had to type manually.
-
I have a function in Javascript that uses jQuery's .get() function to tell my PHP script to update a $_SESSION value. it looks something like this:
function updateSession(key,value){ $.get('updateSessionArray.php',{key:key,value:value}); }
(the PHP code is inconsequential and works fine but I'll post it here so you get what I'm doing, it looks something like:
<?php $_SESSION[$_GET['key']] = $_GET['value']; ?>
This all works fine. When I call something like updateSession("username","Joe"); in JavaScript the $_SESSION array is updated and everyone's happy.
Here's where my problem starts
When I try to call the function multiple times at once it doesn't work. for example, I have a function that needs to update the $_SESSION several times, sort of like this:
function createUser(){ updateSession("username","joe"); updateSession("favorite_color","blue"); updateSession("foo","bar"); }
But when i try this, the original function only runs once - sometimes twice. So in the above example, the username would be set to joe and the favorite_color would be set to blue but foo would not be set to bar. Or perhaps it would but color wouldn't be set to blue. Or Only the username gets set.
It would seem that once the function is busy running (and possibly waiting for a reply) it won't run again. Is that the correct behavior? and if so, is it a javaScript specific behavior or a jQuery thing. It seems odd though as, I'm not asking for it to wait for a response. I just want it to trigger the function to run independently multiple times.
I originally wrote this script without jQuery and I never had a problem before. I admit though that I'm no expert so maybe I'm missing something? Am I just not wrapping my head around the way this should work?
Any thoughts or comments would be appreciated.
-
use opendir() and readir()
something like this...
... all your javascript upto the point where you create your array... then... <?php $dir = bloginfo('template_url') ."/Images/SplashImages/"; // Open a known directory, and proceed to read its contents if (is_dir($dir)) { if ($dh = opendir($dir)) { while (($file = readdir($dh)) !== false) { $fileList.= "'".$dir.$file."', "; } closedir($dh); } } ?> rndimg = new Array(<?=$fileList ?>); .... rest of your javascript
-
what does your javascript look like? eg,
function openPopup(){
//whats here ???
}
right now, your onSubmit is calling that function but it must be failing so instead, the form is just being submited to the action in the HTML and opening in a new window (because you have the target set to do so)
-
I think thats a browser thing. certain browsers (like IE 7+) will always display the address in a pop up box. I for one like that my browsers tells me the URL of the page I'm viewing
you may want to try another workaround like making a fake pop-up (a hidden div styled to look like a new window layered above your content that you un-hide at the appropriate time)
-
PHP doesn't know any of that stuff. PHP runs SERVER SIDE, meaning on your server not on the client-side. You need javascript or some other client-side language to get information about the browser.
-
<pre> <?php print_r($_SERVER); ?> </pre>
enter that and behold all the info!
-
as the above said, use ob_start()
your code is working fine, but it would seem some thing else is being output to the browser before the header("Location: "); call. Nothing can be sent to the browser before a header();
-
I'm new to frameworks and the whole model view control thing so please go easy on me...
I have a page that is included() inside a view. In this page, I have a function() that check needs to do a mysql query that needs to know the database name which I keep in a variable. Apparently though, I can't just declare it as a variable any more?
<?php $db = "database"; function sql(){ global $db; return "database name is: ".$db." ta-da!"; } echo sql();// returns: database name is: ta-da!
why is this?
while ($i <= 100)
in PHP Coding Help
Posted
the point thorpe was touching on is "Sever-side" verse "Client-side" scripts. PHP runs on the server and the entire script is executed before its data is sent to the client-side, aka your browser. If you want to have things happen on your browser's screen after the page has loaded from the server, you need to use a client-side script just as javascript.