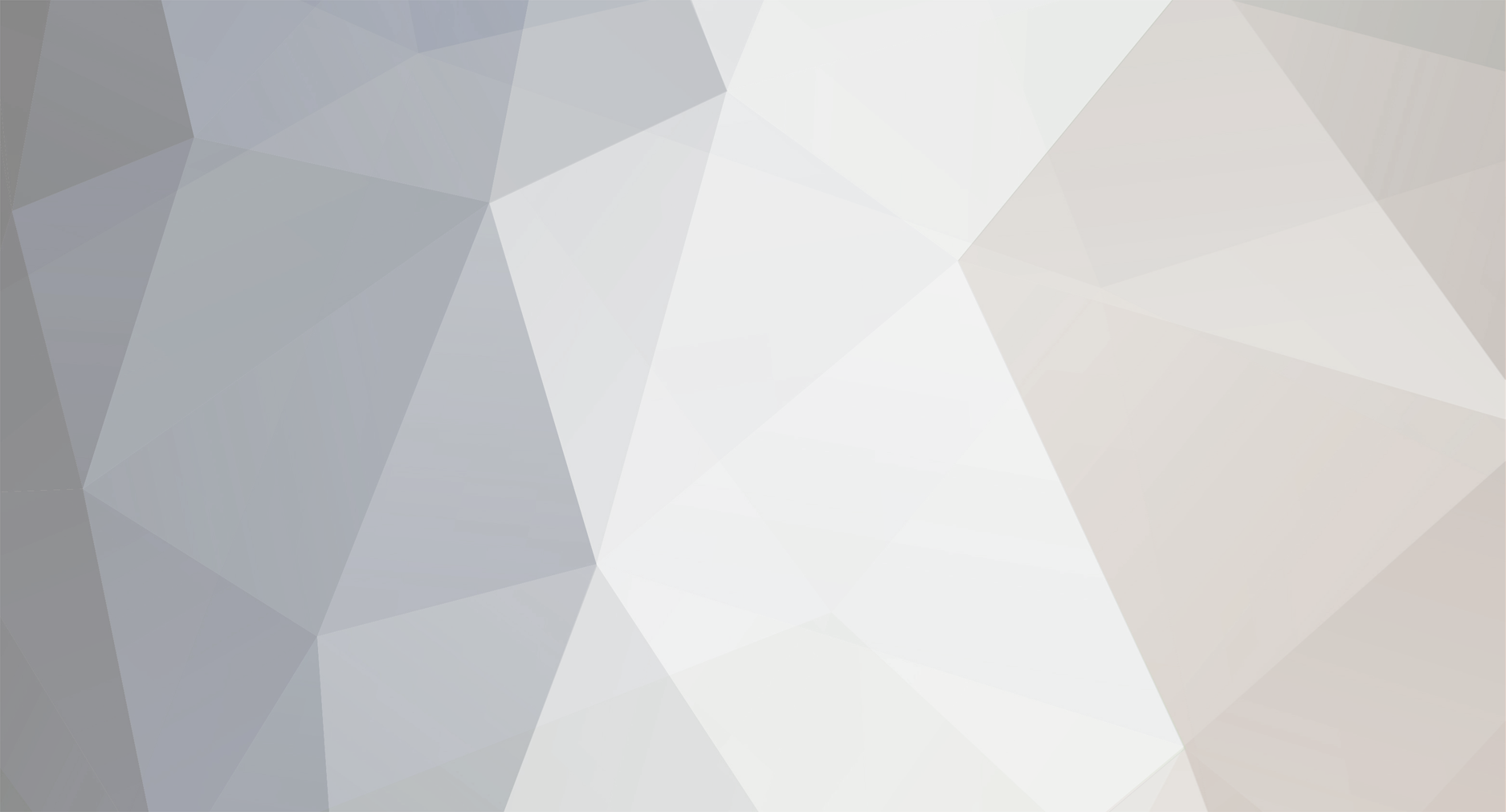
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
As far as i know, you can't. Some googling seems to confirm my thought that javascript is the way to go. My javascript ain't great and i dont have the time right now to play around with it, otherwise i'd put this together for you. You'll need a few things though: 1.) Call a function with the body onLoad attribute that will do two things: firstly, check the URL to see if the current scroll position should be altered (see point 3); and set a timeout to call another function after x number of seconds. 2.) Have this function work out where the scroll bar is (see here for a function that'll do that for you) 3.) Reload using javascript, appending the scroll position to the URL (yourpage.php?scroll=whatever) p.s. Welcome to the forum!
-
Well, firstly, you need to be ordering by the groups. You can then cycle through the results, outputing a new select box if the group name of the current row is different to the last row's group name. Try: <?php $query = mysql_query(" SELECT feature_product.feature_product_id, feature_product_name, feature_product_price, feature_group.feature_group_id, feature_item.feature_group_id, feature_group_name, feature_item_name, feature_item_price FROM feature_product, feature_group, feature_item WHERE feature_product.feature_product_id={$id} AND feature_group.feature_product_id=feature_product.feature_product_id AND feature_item.feature_group_id=feature_group.feature_group_id ORDER BY feature_group_name") or die(mysql_error()); $curr = ''; while($row = mysql_fetch_assoc($query)){ if($curr != $row['feature_group_name']){//last group isn't the same as the current one if($curr != ''){//we're not on the first iteration of the loop - end the last select box echo "</select> <br />\n"; } //output the new select box echo '<select="'.$row['feature_group_name'].'">';//not sure what name you wanted the select boxes to have? $curr = $row['feature_group_name'];//update the current group } //output the option for this row echo '<option value="'.$row['feature_group_name'].':'.$row['feature_item_name'] . '">'.$row['feature_item_name'] ."</option>\n"; } echo "</select><br />\n"; ?> You'll need to format the layout a bit, and you might need to change the names of the select boxes/options - i wasn't entirely sure what you wanted. Hope that helps.
-
Somehow i managed to miss off three semi-colons in that post. I noticed one and modified, but didn't see the other two. I must be tired! Try: <?php $sql = "select count(*),to_number from entries where lcase(smsc) like '%vodafone%' and logdate >='2007-12-01' and logdate <='2007-12-31' and action='Receive' and to_number in (55555,55556,55557,55558,55558) group by to_number;"; $result = mysql_query($sql) or die(mysql_error()); $nums[] = array(); while(list($count,$num) = mysql_fetch_row($result)){ $nums[$num] = $count; } echo $nums[55555]; ?>
-
I prefer to use an INT field to store the dates, so we can store them as a unix timestamp. <?php $now = time(); $next_week = strtotime('next week'); mysql_query("INSERT INTO tbl SET col1 = $now and col2 = $next_week") or die(mysql_error()); ?> You can then format the dates however you would like when you output them, using the date() function.
-
You got the short stick then? I know they say don't shoot the messenger...
-
I was wondering if it were something to do with strtotime(). I had a problem a while back where the code i posted worked perfectly for me, but not for whoever was asking for the help. I think at the time i assumed it was a difference in the strtotime() function in the different versions of PHP that we were running. If that were is that case (and it's certainly a big if) then i would imagine your code would work.
-
Did you read what i said? We're using an array, because variable names cannot start with a number. I added an echo at the end as an example
-
You shouldn't need to do any nesting. Just select the to_number along with the count. You'll also need to use an array, rather than the variables. A variable name cannot start with a number, so we cant have $55555. Try: <?php $sql = "select count(*),to_number from entries where lcase(smsc) like '%vodafone%' and logdate >='2007-12-01' and logdate <='2007-12-31' and action='Receive' and to_number in (55555,55556,55557,55558,55558) group by to_number;" $result = mysql_query($sql) or die(mysql_error()); $nums[] = array() while(list($count,$num) = mysql_fetch_row($result)){ $nums[$num] = $count; } echo $nums[55555]; ?>
-
No problem. Can you mark this as solved if you're all done?
-
You should be using AND, not a comma to separate the different conditions of the WHERE clause: <?php $result = mysql_query("SELECT * FROM product, product_attributes, product_options WHERE product.product_id={$id} AND product_attributes.options_id=product_options.product_options_id AND product_options.product_options_id=product_options_values_to_product_options.product_options_id AND product_options_values_to_product_options.product_options_values_id=product_options_values.product_options_values_name") or die(mysql_error()); ?>
-
[SOLVED] Use date() as html <input value ?
GingerRobot replied to br3nn4n's topic in PHP Coding Help
Yes. Unless you happened to have a constant called D which contained the string 'D'... -
[SOLVED] Over 100 Records = Constant Loop....???
GingerRobot replied to PHPNewbie55's topic in PHP Coding Help
How long does it take for the code to complete with 100 records? I personally think its much more likely to be an issue with the sheer number of records you're trying to use. You've a lot of while loops - each one is having to loop through 100x as much data with 10,000 records. One of the things that isn't going to be helping you is the sheer number of mysql queries. You do realise that you can update more than one field at a time? UPDATE tbl SET col1 = 'some value', col2 = 'something else' Looks to me like doing that would save you an awful lot of queries. -
Well, the query would be something like: UPDATE tbl SET col = col +5 Im not entirely sure if that's what you were asking though.
-
Yes, they are, for all intents and purposes, the same. I think most people have switched over to using the superglobals though. And i think you probably misread.
-
[SOLVED] Can't get multi values to return in a select list
GingerRobot replied to scott56hannah's topic in PHP Coding Help
The problem is that, because you can select mutiple products, it returns an array of selected products. Try: <?php $FirstName = $_POST['FirstName']; #assign value $LastName = $_POST['LastName']; #assign value $Address = $_POST['Address']; #assign value $Country = $_POST['Country']; #assign value $Mobile = $_POST['Mobile']; #assign value $Phone = $_POST['Phone']; #assign value $Email = $_POST['Email']; #assign value $Message = $_POST['Message']; #assign value $Product = $_POST['ProductSelected']; #assign value $To = "enquiry@xlautomation.com.au"; #recipient $Subject = "Contact Us Form Feedback from ".$Email; #subject #create the comments that will be sent as text in the message later on $Comments = "First Name :".$FirstName."\n"; $Comments .= "Last Name :".$LastName."\n"; $Comments .= "Address :".$Address."\n"; $Comments .= "Country :".$Country."\n"; $Comments .= "Mobile :".$Mobile."\n"; $Comments .= "Phone :".$Phone."\n"; $Comments .= "Email :".$Email."\n"; $Comments .= "Message :".$Message."\n"; $Comments .= "Products Selected :".implode(',',$Product)."\n"; $Attachment = $_FILES['ClientFile']; $FilePath = $_FILES['ClientFile']['tmp_name']; $FileName = $_FILES['ClientFile']['name']; $FileSize = $_FILES['ClientFile']['size']; $FileType = $_FILES['ClientFile']['type']; $FileError = $_FILES['ClientFile']['error']; #echo "FilePath ".$FilePath."\n"; #echo "FileName ".$FileName."\n"; #echo "FileSize ".$FileSize."\n"; #echo "FileType ".$FileType."\n"; #echo "FileError ".$FileError."\n"; #make sure the file is not greater than 500k in size if it is then report the Error page for file to big if ($FileSize > 500000) { header("Location: errors/ErrorFileSize.html"); exit(); } #create a bounday string $num = md5(time()); $bound_text = "Multipart_Boundary_x{$num}x"; $bound = "--".$bound_text."\r\n"; $bound_last = "--".$bound_text."--\r\n"; #set the header information before including the text and file details $headers = "From: website@xlautomation.com.au\r\n"; $headers .= "MIME-Version: 1.0\r\n"; $headers .= "Content-Type: multipart/mixed; boundary=\"$bound_text\""; #now include the text of the message before including the file $msg = "If you can see this MIME than your client doesn't accept MIME types!\r\n"; $msg .= $bound; $msg .= "Content-Type: text/plain; charset=\"iso-8859-1\"\r\n" ."Content-Transfer-Encoding: 7bit\r\n\r\n" .$Comments."\r\n\n" .$bound; #now set the file name for the function to return the file for sending $file = file_get_contents($FilePath); #now add the file to the message contents for sending $msg .= "Content-Type: {$FileType}; name=\"{$FileName}\"\r\n" ."Content-Transfer-Encoding: base64\r\n" ."Content-disposition: attachment; file=\"{$FileName}\"\r\n" ."\r\n" .chunk_split(base64_encode($file)) .$bound_last; #now ready to send the email if(mail($To, $Subject, $msg, $headers)) { header("Location: Thankyou.html"); } else { header("Location: errors/ErrorFileUpload.html"); } ?> I've used the implode() function to join all the elements together with a comma. -
You have to link to it. Thats the way it works. If you need to use other variables, either pass them in the URL, or set a session. You should have one php file, lets say captcha.php with your image generation code, and then you should use: <img src="captcha.php?foo=bar" />
-
In what way is it not pretty? As far as i can see, it's the only way to do it. Personally i'd also add in some error checking to return the first image if the current image is the last: <?php $sql = mysql_query("SELECT * FROM tbl WHERE image_id > $current_id ORDER BY image_id LIMIT 1") or die(mysql_error()); //not sure why you had a limit 2 previously? if(mysql_num_rows($sql) == 0){ $sql = mysql_query("SELECT * FROM tbl ORDER BY image_id LIMIT 1") or die(mysql_error()); } $row=mysql_fetch_assoc($sql); ?> This does, of course, rely on you passing the current id through the url.
-
Its a common misconception. You shouldn't be storing an online/offline status, because, as you've noticed, you run into issues if someone closes the browser. What you should be doing is storing a 'last active' timestamp. This will, of course, need to be updated on each page. When working out who is online, you'll select only those users who were last active in, say, the last 300 seconds (5 minutes).
-
As Ken alluded to, it could be a myriad of different things. Posting the code (please use - without the spaces) tags would be helpful, along with a fuller explanation of what happens when you try this on your computer. Do you get any errors? Blank page? Does everything 'appear' to be ok, but you dont get any data in the database? p.s. Welcome to the forum
-
How to store last 4 characters of a string.
GingerRobot replied to bsamson's topic in PHP Coding Help
SELECT * FROM tbl WHERE SUBSTRING(col,-4) = '7890' -
You need to use AND, not a comma: <?php $id = intval($_GET['product_id']); $result = mysql_query("SELECT * FROM product, manufacturer_image WHERE product_id={$id} AND manufacturer_image.manufacturer_id=product.manufacturer_id") or die(mysql_error());//always try adding an or die statement if you're having mysql troubles if(mysql_num_rows($result) > 0) { while($image = mysql_fetch_assoc($result)) { echo "<p align=\"center\" class=\"shipping-head\"><img src=\"$image[image]\" width=\"130px\">"; }} else { echo "Hey"; } ?>
-
[SOLVED] easy way to add 60 days to datetime in mysql to all rows?
GingerRobot replied to izbryte's topic in PHP Coding Help
Try: UPDATE tbl SET col = col + INTERVAL 60 DAY -
Edit: ignore. Being blind.
-
Thats not exactly going to work - you'd need the session id to access it! raven74 : Unfortunately, those are your options. You either pass it around in the URL or stick it in a cookie.
-
You can create word documents on the fly with php. See: http://web.informbank.com/articles/technology/php-office-documents.htm I've never done it myself though, and Im not sure how you would go about using that to create tables. But its worth a look.