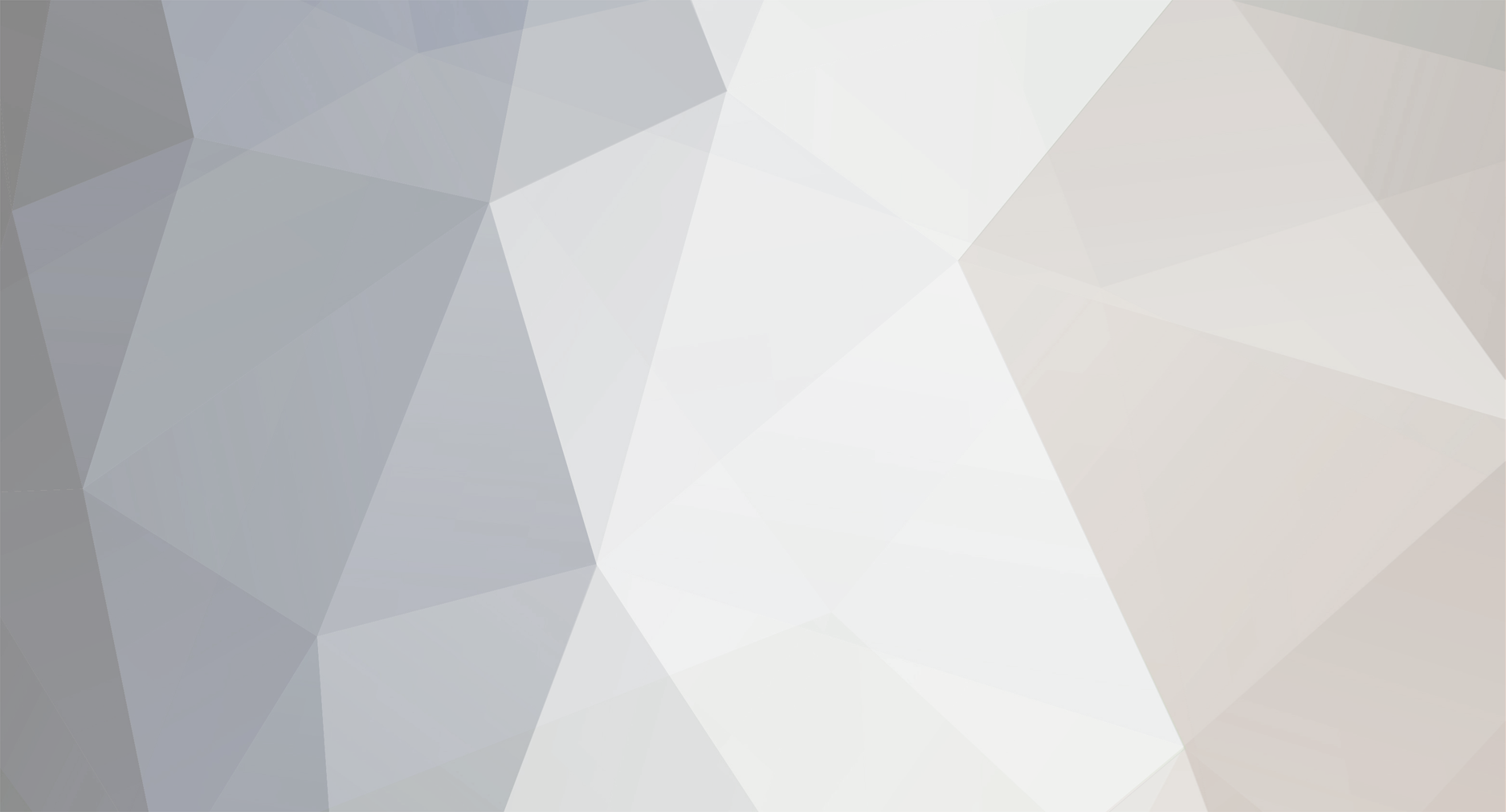
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
What's the difference between Zend_Db_Table and Zend_Db_Table_Abstract ?
ignace replied to colap's topic in Frameworks
There is no difference Zend_Db_Table extends Zend_Db_Table_Abstract. You should always extend from abstract classes, not concrete classes. -
ActiveRecord is a common pattern to use for simple projects. You could abstract the ActiveRecord and use a Repository to actually retrieve/store the ActiveRecord's. $repo = new UserRepository(); $user = $repo->find(1); $user->firstname = 'John'; $user->lastname = 'Doe'; $repo->store($user); This way the ActiveRecord doesn't need to know about the DB table structure or have a DB connection. A possible implementation for find() could be: public function find($id) { // get the record from DB $record = new ActiveRecord($this->_columns); $record->populate($rowData); return $record; }
-
class Cube { private $cubes = array(); public function addCube(Cube $cube) { $this->cubes[] = $cube; return $cube; } public function addCubes(array $cubes) { foreach($cubes as $cube) { $this->addCube($cube); } return $this; } } $cube = new Cube(); $cube->addCube(new Cube())->addCube(new Cube())->addCubes(array(new Cube(), new Cube(), new Cube(), new Cube())) print_r($cube); You could take a look at the Composite Pattern
-
$combobox = $form->addElement('Select', 'mycombobox'); You can read more about forms at the Zend_Form documentation
-
I like it alot. You certainly took my advice on explaining what minecraft is. I would add some in-game items to the website (opacity 10% background bottom-right, top-left) so that the visitor gets familiar with how the game looks and gets inspired to read more about Minecraft.
-
As you probably know the Waterfall methodology does all A&D up front. RUP is an Agile process like eXtreme Programming that uses Artifacts. If you do all your planning up front you are using the Waterfall methodology instead of a true Agile process. In an Agile methodology you perform your planning at the start of an iteration, this is mostly done on a white-board in group, sometimes someone is assigned to translate the thoughts/designs on the white-board to an appropriate/official paper format as documentation for later use. However not many do so and instead use the tests as guides for new programmers, as a test should clearly state how a class is used. A principle many adhere to, with concerns to documentation, is only create the Artifact if it ADDS VALUE.
-
Too much session and database reading? (general info request on coding)
ignace replied to Kiluad's topic in PHP Coding Help
You shouldn't have to worry unless you are absolutely sure you will make the 1M visitors/day (exaggeration intended) mark in that case you will need to adjust your app architecture to be able to scale-up/out and make your app so that certain pieces/areas of the app can be moved to a different server -
Ah, OO Analysis & Design my favourite topic Wether you want to develop your app in OO or not is up to you. Just remember you don't abstract away too much too soon in the process, otherwise your app although yet small will be overwhelming and too complex early on in the process. Since you have Sequence Diagrams you also should have a clear idea about the code, since a Sequence Diagram is actually code documentation on paper. Implement these classes and make sure you have a clear set of Unit-Tests to verify that they work. PS All Artifacts in RUP are optional.. except the code Only add aditional Artifacts if they ADD VALUE
-
You could do this with normal replication where you would pick one website that would act as a master and all 69 others would be slaves of that master. This of course assumes that: 1) There is only 1 website that edits prices 2) You have full control over the MySQL setup which may not be the case if they are all shared hosted Otherwise you could pick one website as a host or buy an additional server that will act as the database for all 70 websites. The advantage being that you can buy additional (or use less popular websites to act as a back-up) MySQL servers and add a load balancer. MySQL Replication
-
1) SELECT account_type FROM userdata WHERE manager_id = $managerID 2) SELECT projects.* FROM projects JOIN userdata ON projects.agent_id = userdata.account_type WHERE manager_id = $managerID Do the above queries give you what you need?
-
One page System...linking Artists to specific albums
ignace replied to littlea5h's topic in PHP Coding Help
If you have the money, here's the car: http://www.youtube.com/watch?v=ElS9BKSsezw @OP All these items are too small to put on a specific page (except the lyrics that is) I would go for an approach where you have multiple selects next to each other and that users can click through the several selects to find the lyrics they need: <style>.lw200 { float: left; width: 200px; }</style> <form action="" method="post"> <div class="lw200"> Catalogue: <select size="20" onchange="fillArtistSelect(this.options[this.selectedIndex].value)"> <option>A</option> <option>..</option> <option>Z</option> </select> </div> <div class="lw200"> Artists: <select size="20" onchange="fillAlbumSelect(this.options[this.selectedIndex].value)"></select> </div> <div class="lw200"> Albums: <select size="20" onchange="fillSongSelect(this.options[this.selectedIndex].value)"></select> </div> <div class="lw200"> Songs: <select size="20" onchange="enableSubmit()"></select> </div> <input type="submit" name="Find Lyrics" disabled="disabled"> </form> It's also recommended you refer them to a lyrics page where the above form is also present (and filled in according to their last search so that they can find multiple lyrics without much hussle). -
Derived from your code: $total += $_SESSION['itemqty'][$key] * $_SESSION['itemprice'][$key]
-
Your website should be more then a few links. Start with why did you create this website? What is your goal? Do you want to create a community website for Minecraft fans? If it is then you should allow people to sign up and get involved, but the website should also intrigue people that are not yet familiar with Minecraft and be able to learn about Minecraft. Learn about Information Architecture
-
Always think in reusable components, here's an example derived from your code: function do($name) { $image3 = do1($value, $name); $filename_3 = basename($image3); $rawdata = do2($image3); do3(Mage::getBaseDir('media') . DS . 'import/'.$filename_3); } function do1($value, $name) { return $value->getElementsByTagName($name)->item(0)->nodeValue; } function do2($image3) { $ch = curl_init($image3); curl_setopt($ch, CURLOPT_HEADER, 0); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_BINARYTRANSFER,1); $rawdata=curl_exec($ch); curl_close($ch); return $rawdata; } function do3($filename_3) { $fp = fopen($filename_3,'w'); fwrite($fp, $rawdata); fclose($fp); } The above code won't run but the important thing about this code is that it shows the principles applied. If you have a big chunk of code, look for distinct tasks executed by your code and wrap each into a function.
-
I like the .com version better. What I don't like about your website is: - Giant buttons instead of proper navigation - The main site hovers, or seems to hover. Because the ad is underneath or seems underneath it feels like an annoying pop-up. - Don't call your landing page, landing page. - What is your website about? What's minecraft?
-
PHP-based indexing and search implementation
ignace replied to kristo5747's topic in PHP Coding Help
Zend_Search (as in Zend framework?) is free to use as is the entire framework. -
Go Raichu!
-
You need to add an exit after header like so: $referer = $_SERVER['HTTP_REFERER']; if ($referer != 'http://digitalpixels.co.uk/portfolio') { header('Location: http://digitalpixels.co.uk/404/') ; exit(0); } if ($referer != 'http://digitalpixels.co.uk/windscreen-repair-company') { header('Location: http://digitalpixels.co.uk/404/'); exit(0); }
-
Can this PHP code be valid: $4bears = $bears->getFirst4();
ignace replied to ansharma's topic in PHP Coding Help
This question raised my curiosity as to Why can't variable names start with numbers? Apparently because: -
Can this PHP code be valid: $4bears = $bears->getFirst4();
ignace replied to ansharma's topic in PHP Coding Help
Yes but only in the form: ${4xyz} = 5; You also need to access it in the same way: print ${4xyz}; As a side note: You should avoid writing variables like that. -
No. An array is not a persistent storage device: Everytime the user would reload the page, the quantity would be reset to it's original value. Why do you want to use an array instead of a DB?
-
You should use return because it's possible you may want to modify the data before it's send to the browser. That said, a class can echo stuff (eg Zend_View "renders" the HTML by calling echo)
-
A few ideas I had the last few weeks: YAML 2 Class, custom Zend_Form class builder instead of having to manually type in those Decorator's... custom Zend package builder.. (there has been one around but never really worked for me)
-
The 3th script is better. Less code that can do the same job is better, everything else is just noise. Your script does not call the parser instead on each request it's invoked and "parses" your script to machine-readable code (so called op-code) and executes it.