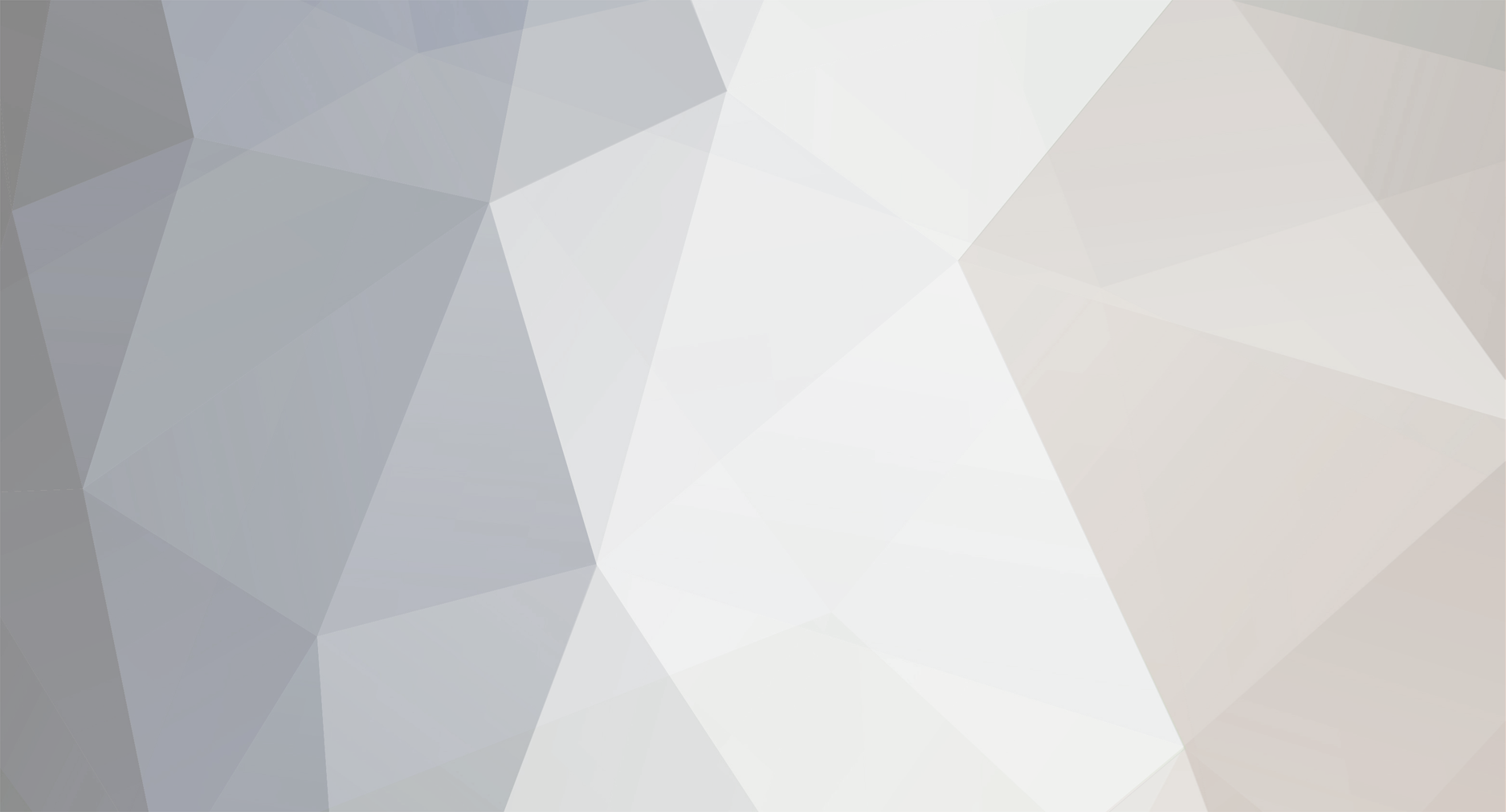
cmgmyr
-
Posts
1,278 -
Joined
-
Last visited
Never
Posts posted by cmgmyr
-
-
can you provide the script?
-
I seem to recall seeing that template before. Is there a point in getting critique on something you did not make?
...it looks like businessman's template that he used
@mreish - If you are not good at designing, why don't you hire someone to do that for you instead of using a template?
-
You can put it into a switch with functions.
-
you can use session cookies...or just cookies
-
www.maaking.com has a good membership script (simple) that will get you going in the right direction.
-
both are giving me 404 errors...and aren't both links the same? ???
-
much better, looks perfect in IE7
-
yeah thats what i'm still getting too. IE7
-
2 things...
1. It looks like you have your table set to 100% width (without looking @ your code). Change the width of the table to the same size as your layout.
or
2. use css with background-position
hope this helps.
-
o hey, I forgot about this one
Yes the blue is still a little bit too dark, and for the grey I was refering to your navication and also the box on your front page.
-
great article!
-
Ok, i've searched for a little while...I just need to change test.php to test.html
Thanks!
-
-
I like it too, good job and keep up the good work!
-
...wow
-
Add this to the top of the page:
<?php $mtime = microtime(); $mtime = explode(" ",$mtime); $mtime = $mtime[1] + $mtime[0]; $starttime = $mtime; ?>
and this to the bottom:
<?php $mtime = microtime(); $mtime = explode(" ",$mtime); $mtime = $mtime[1] + $mtime[0]; $endtime = $mtime; $totaltime = ($endtime - $starttime); echo "This page was created in ".number_format($totaltime,6)." seconds"; ?>
-
HAHA
thats too funny...you must have picked a bad spot or something, I know lots of hot girls that tan
-
no problem
-
<?php echo "Hello you are visiting this page: ".$PHP_SELF; ?>
-
try:
$sql = "SELECT date, type, event, action, comments FROM cancellations WHERE type = 'school' AND date = CURDATE() ";
-
you can take a post, then get a list of words from your DB, scan the post for each word and if you get any results then you can e-mail the post number or whatever you want to yourself
-
you should probably look into making a DB. This would be good for future use. I'm sure you would like to upgrade in the future or even sell you "system" to someone else...it would be pretty hard to keep track of 500+ users in a text file... (besides using a flat file DB...but thats still a DB)
-
when they sign up they will have to select their service provider from a list. When they submit the form, write all of the data into your DB. Then when you send out your "SMS" (email) you will have to search for the provider's info and add that onto the user's phone number.
-
Interesting 6th post I might add!
haha very true
Login Script
in PHP Coding Help
Posted
try something like this:
*** notice the hidden value in your login form
hope this helps