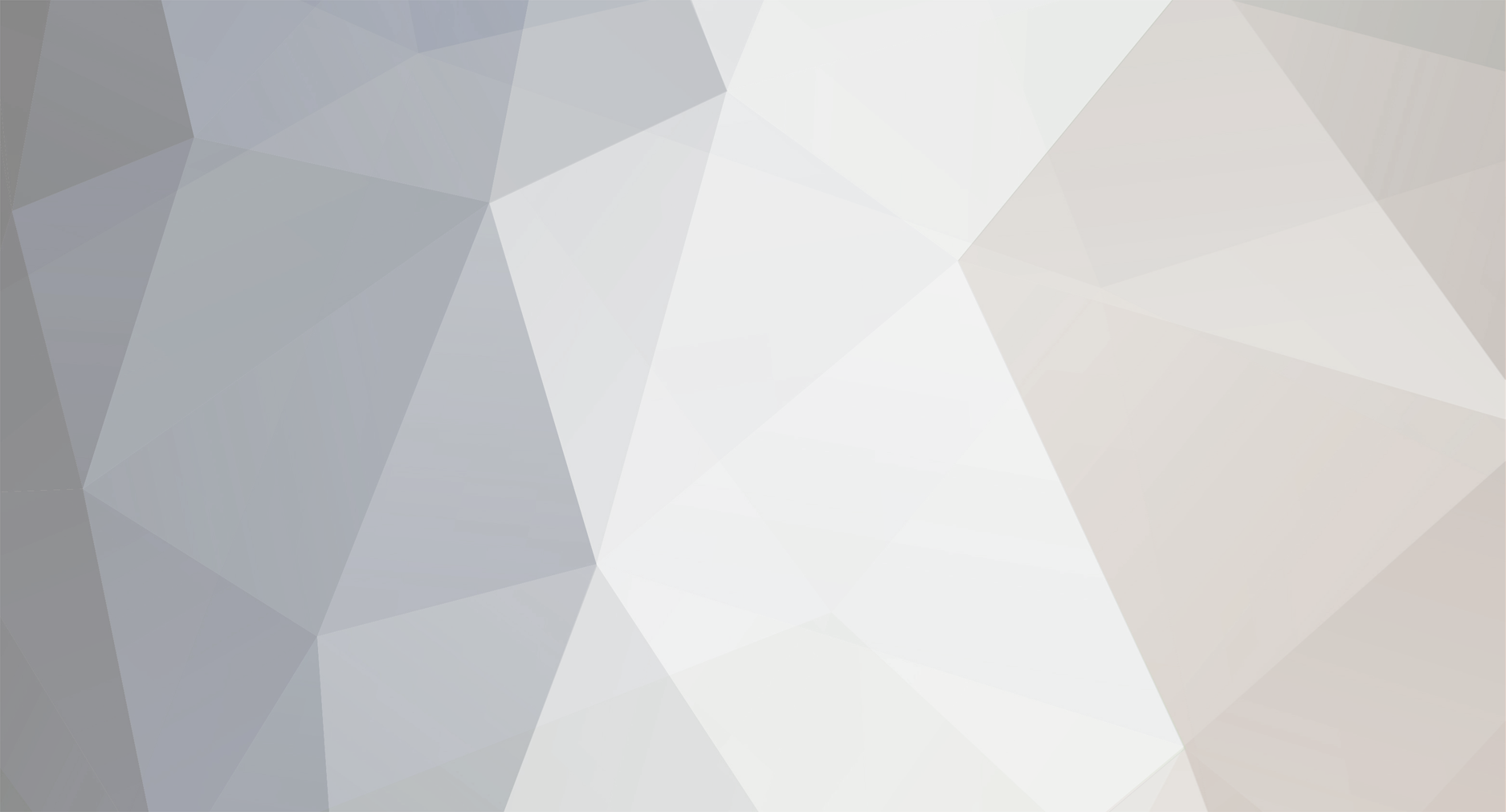
NotionCommotion
Members-
Posts
2,446 -
Joined
-
Last visited
-
Days Won
10
Everything posted by NotionCommotion
-
Providing that one has the ability to modify the server, is there any reason to use mysqli anymore?
-
Help with sockets and ReactPHP
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Do you know of any good classes to handle application authentication and Newline-delimited JSON (or something similar)? -
To reiterate what Barand said, use prepared statements. I know it is just another thing to learn and that you already know the way you are doing it, however, they are easy and secure, and once you do start using them, you will wonder why you didn't start earlier. There are multiple ways to implement them, but 99% of the time I just use associated arrays with :whatever placeholders (first example) or unassociated arrays with ? placeholders (second example). If there are just a few pieces of data, I use ? placeholders, otherwise I use : placeholders. <?php //You should first validate your data before inserting it. I feel it is sometimes okay to use the DB for some validation, however, others will disagree. $data=['id'=>$_POST['id'],'fname'=>$_POST['fname'],'lname'=>$_POST['lname'],'email'=>$_POST['email'],'phone'=>$_POST['phone']]; $stmt=$db->prepare("UPDATE clients SET fname=:fname, lname=:lname, email=:email, phone=:phone WHERE id=:id"); $stmt->execute($data); // or $data=[$_POST['fname'],$_POST['lname'],$_POST['email'],$_POST['phone'],$_POST['id']]; $stmt=$db->prepare("UPDATE clients SET fname=?, lname=?, email=?, phone=?WHERE id=?"); $stmt->execute($data);
-
Help with sockets and ReactPHP
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Kicken, If bi-directional communication is required, I too was thinking that websockets is likely the way to go as I really, really don't want to develop my own and with the wide attention placed on it, expect it is rather robust. My understanding (correct me if I am wrong) is websockets was developed likely specifically for browser clients to remote server bi-directional communication, right? That being said, it probably still would be a good choice for server-to-server bi-directional communication, agree? Jacques1, I would have hoped that reactphp would have implemented some of this application authentication. I do agree that HTTP seems like the best choice "when" it could be used, and otherwise fall back to some more low-level solution. Thanks for both your help. -
Help with sockets and ReactPHP
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Bummer Perfect! But what if sender sends the data, but receiver gets blown up? Sorry, but I had several typos regarding this part. Sender gets local data and sends it remotely. If the data is not received remotely, it stores it locally so it can be sent remotely later. If the stored data is finally delivered, it deletes the local data. I still need to know that it was received. Yes, HTTP. My "big" data can be sent via HTTP as client is behind a firewall and will know the IP which it will be sent to. My only need for sockets is for the server to send the client little data. You think it makes sense to use HTTP/cURL for all client to server communication? Sorry, disregard this as I am sure I haven't provided enough information to make an informed recommendation, but I (now) think this is the way to go. This would also provide headers for confirmation of reception. -
Help with sockets and ReactPHP
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thanks Jacques1, but not what I wanted to hear. The "confirm valid JSON" approach seems a little prone for errors. First, it needs to smell at the start like JSON. Okay, easy enough. But then the concatenated string needs to be JSON, or the concatenated x N strings need to be JSON. I see things getting exponential really quick! In regards to the newline/length prefix, same concerns that I responded to Kicken. As far acknowledgement, that client/sender holds data, sends it to the server/receiver, and if received, doesn't attempt to send it any more and even deletes the data. As such, I think it is important to know that it is received. Maybe I shouldn't use low level sockets for this and instead use curl? My understanding is curl is just sockets but with more overhead protocol. -
Help with sockets and ReactPHP
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thanks Kicken, Helped fill a few, few, and few gaps of mine. Yea, reading the TCP protocol is on my bucket list. I really need to do so. A while back, I played with Wireshark, but need to do more. Regarding this ACKnowledgement packet, does react and/or PHP get this information? Wouldn't this provide confirmation that something was received? Your comments about needing to resend data, buffering, drain, etc makes sense. You are correct that I didn't not implement drain/etc as at the time I did not understand such a concept existed, but will implement them. As far building a protocol and implementing things like "\r\n\r\n" and "$length\n$json", really? I for one seem to like home building my own stuff, but I don't think I should do so. Is what I am attempting to do so really so unique? Many clients need to initiate communication to a server, and the server must be able to receive data from the clients as well as send data to a specific client. I would expect at least a spec and even several implementation classes exist to do so. Thanks again PS. 2016 kind of sucked, and wishing all a good 2017! -
Help with sockets and ReactPHP
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Jacques1, I really wish you would just write my damn code for me and stop trying to teach me. Well, not really, but sometimes... I do really appreciate all the help I have received on this forum, and have learned a great deal. "You may have to repeat the sending procedure multiple times..." You being me/PHP or TCP and/or the operating system? When you say "and acknowledged by the receiver", which method? I would have expected it to be write(), but per https://github.com/reactphp/stream#methods-1, write only returns true if the buffer is not exceeded, and else false. How do I know that it has been received? Okay, I understand what you are saying regarding sending chunks and waiting for the drain event. But just to make sure, this is just to stay within the buffer, right? I am still not sure how the receiver deals with knowing when it has received all the data and can interpret it as JSON. I suppose I can concatenate the incoming text stream and check if it is valid JSON, however, I hope this is not the way to do so. PS. I was a couple months behind on the reactphp code on the Pi, and after updating composer, now I witness the same 65,536 buffer as the Centos box -
Help with sockets and ReactPHP
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thanks Jacques1, After driving a bit and thinking it over, I better realized that sockets deal with streams of TCP segments. Are each of these segments sent individually, and might go through different Internet routers to eventually get to their destination, and is that why they might be received in a different order? But receiving data in arbitrary order seems pretty useless. Somehow it must be reassembled after received in some intelligent order. I would think the reactphp class would handle this, and am wondering if something like $conn->on('end', function($data) {…}); instead of 'data' can be used? Also, after looking over your referenced documentation, write($data) will return false only if the supplied $data which might need to be buffered exceeds the internal buffer size, and it has nothing to do whether the receiving machine actually received the data. Do you think I will need to implement my own homemade acknowledgement protocol where after the receiving receives the data issues another stream back saying it has been acknowledged? This sounds a bit challenging as I wish to remain asynchronous and wouldn’t want to use some blocking function, and again would have expected the class to somehow implement this. Thanks again -
Help with sockets and ReactPHP
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
A couple other observations. While $connect->write() returns false on the Pi returns for packages 2,048 and greater, Centos does at 65,536 and greater. Sounds like a configuration issue. Still, the question remains what the function returning true or false really means, and how the client can know whether the server received the data. Also, big packages are always broken up in smaller packages of around 1 to 2K. So, with my small data transfers, I just witnessed this every now and then. Somehow, the server script needs to determine which packages go together and to combine them -
Check if variable is a two element unassociated array
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
A little off topic, so started a separate post. -
I am trying to communicate between two machines using sockets and the http://reactphp.org/ framework. For now, the client is just sending data and I know I can use cURL, but will later need the server to initiate communication, so please just assume I want to use sockets. The data transferred is below is just a string of dots, but will eventually be JSON. I ran the below client2.php script on a Raspberry Pi (192.168.1.210) and the below server2.php script on a Centos box (192.168.1.200), and got the results shown under TEST 1. I then swapped the two machines (and swapped the value of $ip in each), and got the results under TEST 2. Questions... Why am I loosing data? Is my loop rate two fast (1 second)? I've changed it to 3 seconds, and it happens less but still happens. I suppose I can add some sort of checksum to the data, but surely doing so isn't required with TCP? Why am I receiving more packets than I am sending? They seem to add up to the correct amounts that are being sent (so maybe this addresses my Question 1). How does the server "know" which packets need to be combined? It is JSON, and half a JSON string doesn't mean much. Why doe the Pi not return true for $connect->write($package) when the string is 2048 or greater? What does this really mean as you can see the other machine is still getting the data. How can the client "know" that the server received a package of data? Don't know if I wish to ask this, but what else am I doing wrong with my implementation? Thanks! client2.php <?php // Client require 'vendor/autoload.php'; use React\EventLoop\Factory; use React\Socket\Connection; ini_set('display_errors', 1); ini_set('display_startup_errors', 1); error_reporting(E_ALL); set_time_limit(0); $ip='192.168.1.200'; $loop = Factory::create(); $server = @stream_socket_client("tcp://$ip:1337", $errno, $errstr, STREAM_CLIENT_ASYNC_CONNECT); echo 'Connection successful!' . PHP_EOL; $socket = new Connection($server, $loop); //$socket->bufferSize = 65536; $connect=$socket; $socket->on('data', function($data) { echo "on data: $data" . PHP_EOL; }); $socket->on('close', function() { echo "on close" . PHP_EOL; }); $loop->addPeriodicTimer(1, function() use ($connect) { static $counter=2040; $package=str_repeat('.',1*$counter). PHP_EOL; $counter++; $size=strlen($package); if($connect->write($package)) { echo "Message Sent ($size)". PHP_EOL; } else { echo "Message Not Sent ($size)". PHP_EOL; } }); echo 'Start Client ...' . PHP_EOL; $loop->run(); server2.php <?php // Server require 'vendor/autoload.php'; use React\EventLoop\Factory; use React\Socket\Server as SocketServer; use React\Http\Server as HttpServer; ini_set('display_errors', 1); ini_set('display_startup_errors', 1); error_reporting(E_ALL); set_time_limit(0); $ip='192.168.1.200'; $loop = Factory::create(); $socket = new SocketServer($loop); $socket->on('connection', function($conn) { $conn->on('data', function($data) { $size=strlen($data); echo "on data: ($size)" . PHP_EOL; }); $conn->on('close', function($conn) { echo "on close" . PHP_EOL; }); $conn->on('error', function($conn) { echo "on error" . PHP_EOL; }); }); $socket->listen(1337,$ip); $loop->run(); ******************************* TEST 1 (Pi as client and Centos as server) ******************************************* michael@raspberrypi:/var/www $ php client2.php Connection successful! Start Client ... Message Sent (2041) Message Sent (2042) Message Sent (2043) Message Sent (2044) Message Sent (2045) Message Sent (2046) Message Sent (2047) Message Not Sent (2048) Message Not Sent (2049) Message Not Sent (2050) ^C michael@raspberrypi:/var/www $ [Michael@devserver react]$ php server2.php on data: (2041) on data: (2042) on data: (2043) on data: (1448) on data: (596) on data: (1448) on data: (597) on data: (1448) on data: (598) on data: (2047) on data: (2048) on data: (1448) on data: (601) on data: (2050) on close ^C [Michael@devserver react]$ ******************************* TEST 2 (Centos as client and Pi as server) ******************************************* [Michael@devserver react]$ php client2.php Connection successful! Start Client ... Message Sent (2041) Message Sent (2042) Message Sent (2043) Message Sent (2044) Message Sent (2045) Message Sent (2046) Message Sent (2047) Message Sent (2048) Message Sent (2049) Message Sent (2050) Message Sent (2051) Message Sent (2052) Message Sent (2053) Message Sent (2054) Message Sent (2055) Message Sent (2056) Message Sent (2057) ^C [Michael@devserver react]$ michael@raspberrypi:/var/www $ php server2.php on data: (2041) on data: (2042) on data: (2043) on data: (2044) on data: (2045) on data: (2046) on data: (2047) on data: (2048) on data: (2049) on data: (1448) on data: (602) on data: (2051) on data: (2052) on data: (2053) on data: (2054) on data: (2055) on data: (2056) on data: (2057) on close ^C michael@raspberrypi:/var/www $
-
Check if variable is a two element unassociated array
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
I give, I give Thanks guys, I will go back to a more sensible approach. I actually do have a problem with the amount of content. Trying to make it marginally smaller, however, is surely only a band-aid and not a fix. -
If you wish to use SQL to make these modifications and not use any PHP, you should have asked this question in the MySQL (or whichever) forum. I expect you can do so using http://dev.mysql.com/doc/refman/5.7/en/regexp.html.
-
I had been using influxdb 0.9.4.2. I am also using https://github.com/influxdata/influxdb-php as a PHP influxdb client. I never tried making an insert directly into the influx shell, so I don't know whether I would get the float/integer error. I've since upgraded to influxdb 1.1.1. I still get the float/integer error when using the influxdb-php client. I do not get the error when making an insert directly into the influx shell. Looks like I need a new influxdb client...
-
Check if variable is a two element unassociated array
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Originally, I used indexes, and my JSON looked like: [ {"timestamp":123321,"data":{"value":133.3,"units":"lbs"}}, {"timestamp":123324,"data":{"value":123.2,"units":"lbs"}}, {"timestamp":123327,"data":{"value":113.4,"units":"lbs"}}, .... {"timestamp":124329,"data":{"value":153.1,"units":"lbs"}} ] It just seemed like a lot of extra fluff when I could instead do: [ {"t":123321,"d":[133.3,"lbs"]}, {"t":123324,"d":[123.2,"lbs"]}, {"t":123327,"d":[113.4,"lbs"]}, .... {"t":124329,"d":[153.1,"lbs"]} ] Maybe, it isn't an issue, and I might as well improve the readability and go back to the first approach. But then again, if user requirements change in the future, maybe it would be nice to have to deal with less data. -
Thanks Jacques1, requinix, and Psycho, Yes, the version I am using will throw an error stating something like previous values were floats and the new value attempted to be inputted is an integer. The problem manifests when a value just happens to be 128.0 which PHP replaces with 128. I don't know whether influx just wants it to "look" like a float or really be one (and I am not sure this even makes any sense). I haven't been able to check the influx version I am using, but expect it is old. If it is, I will update it and hopefully this will be a mute issue. If it still is an issue (which I expect will not be), I will just iterate over the values on the receiving side and typecast as necessary.
-
Check if variable is a two element unassociated array
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
No, I've never dealt with BSON. It seems to have been originally developed for MongoDB, but maybe has applications outside of it? http://php.net/manual/en/function.bson-decode.php seems to be a bit premature. -
influxdb
-
Check if variable is a two element unassociated array
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
It is just a homemade JSON protocol transferred via sockets between two machines which I both control. I wish to limit the amount of network traffic by reducing the JSON string size. I plan on transferring something like the following, and need to retrieve the three values in the d arrays and interpret them based on their position in the array. I'm also concerned with their values, but need to first understand their meaning by their position. Make sense? Thanks [ {"t": 123321, "d": [123.41,4113.231,45234.123]}, {"t": 123324, "d": [143.41,4213.231,44234.123]}, {"t": 123326, "d": [142.41,4413.231,42234.123]}, {"t": 123329, "d": [153.41,4313.231,43234.123]} ] -
The receiving machine needs float values. I am in control of both the sending machine and receiving machine, and I wish to put as much of the processing on the sending machine. Since I am in control of both machines, I didn't plan on validating on the receiving machine, but in hindsight, not doing so is likely never a good idea. Please ignore. I will send JSON in whatever format PHP wishes to do, and still validate/typecast on the receiving machine.
-
b[0]->c is 128. How can I make json_decode return it as a float and not an integer? The only way that I am able to do so is hardcode 128 as either 128. or 128.0, but I don't wish to hard code it. Thanks <?php $json='{"a": 123, "b": [{"c":127.2},{"c":128},{"c":129.2}], "d":123.321}'; echo '<pre>';var_dump(json_decode($json));echo '</pre>'; $json='{"a": 123, "b": [{"c":127.2},{"c":128.0},{"c":129.2}], "d":123.321}'; echo '<pre>';var_dump(json_decode($json));echo '</pre>'; $json='{"a": 123, "b": [{"c":127.2},{"c":128.},{"c":129.2}], "d":123.321}'; echo '<pre>';var_dump(json_decode($json));echo '</pre>'; function display($c1) { $arr=["a"=>123,"b"=>[["c"=>127.2],["c"=>$c1],["c"=>129.2]],"d"=>123.321]; $json=json_encode($arr); echo '<pre>';var_dump(json_decode($json));echo '</pre>'; } $c1=128; display($c1); $c1=(float) $c1; display($c1); $c1=128.0; display($c1); object(stdClass)#1 (3) { ["a"]=> int(123) ["b"]=> array(3) { [0]=> object(stdClass)#2 (1) { ["c"]=> float(127.2) } [1]=> object(stdClass)#3 (1) { ["c"]=> int(128) } [2]=> object(stdClass)#4 (1) { ["c"]=> float(129.2) } } ["d"]=> float(123.321) } object(stdClass)#1 (3) { ["a"]=> int(123) ["b"]=> array(3) { [0]=> object(stdClass)#4 (1) { ["c"]=> float(127.2) } [1]=> object(stdClass)#3 (1) { ["c"]=> float(128) } [2]=> object(stdClass)#2 (1) { ["c"]=> float(129.2) } } ["d"]=> float(123.321) } object(stdClass)#1 (3) { ["a"]=> int(123) ["b"]=> array(3) { [0]=> object(stdClass)#2 (1) { ["c"]=> float(127.2) } [1]=> object(stdClass)#3 (1) { ["c"]=> float(128) } [2]=> object(stdClass)#4 (1) { ["c"]=> float(129.2) } } ["d"]=> float(123.321) } object(stdClass)#1 (3) { ["a"]=> int(123) ["b"]=> array(3) { [0]=> object(stdClass)#4 (1) { ["c"]=> float(127.2) } [1]=> object(stdClass)#3 (1) { ["c"]=> int(128) } [2]=> object(stdClass)#2 (1) { ["c"]=> float(129.2) } } ["d"]=> float(123.321) } object(stdClass)#1 (3) { ["a"]=> int(123) ["b"]=> array(3) { [0]=> object(stdClass)#2 (1) { ["c"]=> float(127.2) } [1]=> object(stdClass)#3 (1) { ["c"]=> int(128) } [2]=> object(stdClass)#4 (1) { ["c"]=> float(129.2) } } ["d"]=> float(123.321) } object(stdClass)#1 (3) { ["a"]=> int(123) ["b"]=> array(3) { [0]=> object(stdClass)#4 (1) { ["c"]=> float(127.2) } [1]=> object(stdClass)#3 (1) { ["c"]=> int(128) } [2]=> object(stdClass)#2 (1) { ["c"]=> float(129.2) } } ["d"]=> float(123.321) }
-
Check if variable is a two element unassociated array
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Validation of data received via JSON. -
Not positive about the SELECT query, but try something like the following. Also, look into prepared statements. <?php $ip = $_SERVER['REMOTE_ADDR']; $con=mysqli_connect("domain.com.mysql","domain_com","domain_password","domain_database"); $result = mysqli_query($con,"SELECT (NOW()-INTERVAL DAY > lastvisit) lastvisit FROM ipblock WHERE ip='".$ip."'"); $row = mysqli_fetch_array($result); if($row && $row['lastvisit']) { //The user has used the function within 24 hours, kill the script. echo "Come back in 24 hours"; exit; } else { // Looks clear, let them use the function $MyFunction = true; mysqli_query($con,"INSERT INTO ipblock SET lastvisit=NOW() WHERE ip='$ip' ON DUPLICATE KEY SET lastvisit=NOW()"); }