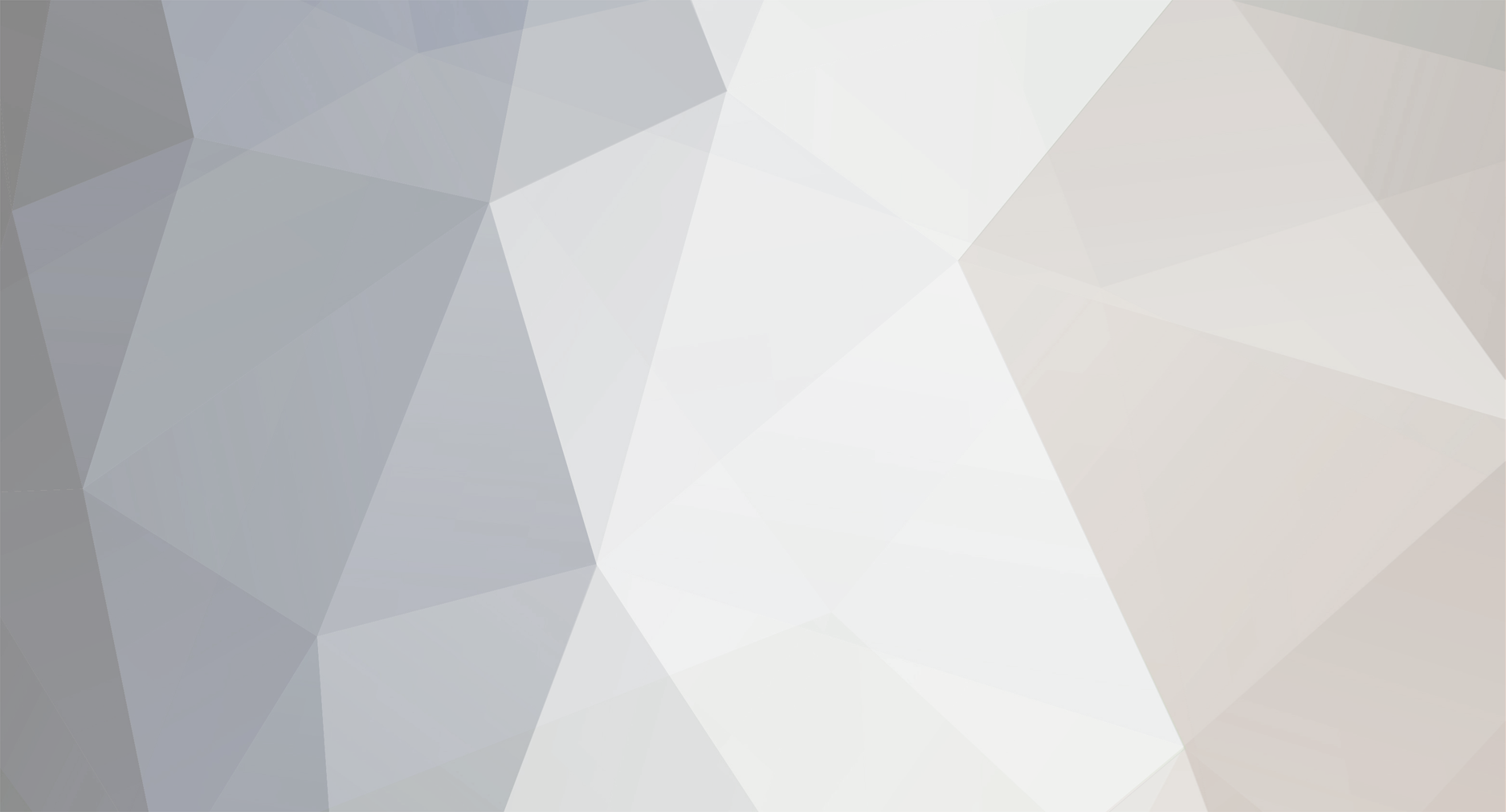
NotionCommotion
Members-
Posts
2,446 -
Joined
-
Last visited
-
Days Won
10
Everything posted by NotionCommotion
-
Pass PHP callback to constructor
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thank you all! Given more thought, I totally agree that I shouldn't use a constructor to do something. If the class only has one purpose, why make it happen using a constructor, and instead I should just use a simple function. Agree? Jacques1 suggestion of using the observer pattern has merit, and I will probably do so. Still curious why you all feel anonymous callback functions are such a bad idea? The whole purpose of this script is to respond to a jQuery plugin, and typically JavaScript/jQuery often uses anonymous callback functions for fairly sophisticated requirements. When configuring the server script, it would maybe be nice to follow the same design pattern as when configuring the jQuery plugin. -
Pass PHP callback to constructor
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thanks Scootstah, My original question dealt with with how to keep the base class intact and add additional script to update the database, and not how to implement a router/controller. Maybe, however, I need help on that part as well. You stated earlier: Also, for the record, constructors should only be concerned with setting up the class... and not actually doing anything. Originally I was planning on putting the controller just for tasks associated with this particular class in the constructor and implementing the appropriate task based on a GET or POST value. Why shouldn't the constructor do something other than set up a class? -
Pass PHP callback to constructor
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thanks Jacques1, Guess that makes sense, but will need to give it a bit more thought. Why do you think doing so is more appropriate than something like the following: new myImageCropper($arr); class myImageCropper extends imageCropper { public function update() { parent::update(); //SQL to update database goes here } } -
Pass PHP callback to constructor
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
I never heard of observer pattern before and am reading up on it. How do you envision it being used? Thanks -
Pass PHP callback to constructor
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Hello Scootstah and Requinix, I replied to Jacques1 give some context on what I am attempting to accomplish. Does your advise change? Thanks -
Pass PHP callback to constructor
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Hi Jacques1. Long time no see. Hope all is good. Maybe no problems with the code. At my day job now and wrote it without trying it. I've never tried to do something like this, and didn't know I was close. What am I trying to do? I wrote a little jQuery plugin which allows the user to select an image file on their local PC and then select the area of the image which they want, and the file and desired area coordinates are sent to the server. It also allows the user to delete the previously uploaded image and replace the target with a default image. The JavaScript is similar to: $('.target').imageCropper('/path/to/server/script.php?task=doSomething'); To accompany the plugin, I also wrote a PHP class which has four public methods, and the method to execute is based on $_GET or $_POST['method_to_execute']. init(). The configuration of the plugin is all defined serverside and the plugin gets the configuration settings from the server when it is initialed. upload(). Uploads the image and saves it in a temporary folder. update(). Crops and resizes the image and moves it to a permanent folder. delete(). Deletes the previously image which is stored in the permanent folder. For the update() and delete() methods, I wish to allow some other custom functionality such as updating the database. The PHP configuration would be something similar to the following: public function doSomething() { $arr=array( 'path_to_save'=>'bla/bla/bla', 'ratio'=>2, 'default_image'=>'/bla/default.png', 'allowed_extentions'=>array('png','jpg'), 'other_stuff_to_override_default_settings'=>'bla', 'save'=> function($col1,$col2) { // call back to save filename in database $stmt=$this->pdo->prepare('UPDATE mytable SET col1=?, col2=? WHERE id=?'); $stmt->execute($col1,$col2,$this->id); }, 'delete'=>function() { //As required } ); new imageCropper($arr); //Based on $_GET or $_POST['method_to_execute'], execute the appropriate method. } Make sense? -
How do I pass a callback function to a constructor, and be able to use variables from both the parent class and new class? The sample code below will hopefully make my question more clear. Thank you <?php $controller=new controller(); $controller->doSomething(); class controller { public $pdo; public $id=333; public function __construct() { $this->pdo= new PDO ('mysql:dbname=testdb;host=127.0.0.1', 'dbuser', 'dbpass'); } public function doSomething() { $arr=array( 'a'=>123, 'b'=>321, 'save'=> function($col1,$col2) { $stmt=$this->pdo->prepare('UPDATE mytable SET col1=?, col2=? WHERE id=?'); $stmt->execute($col1,$col2,$this->id); } ); new myClass($arr); } } class myClass { public function __construct($options) { // Do some stuff... $col1=111; // Assume $_POST['col2_value'] is equal to 222 $options['save']($col1, $_POST['col2_value']); //Execute 'UPDATE mytable SET col1=111, col2=222 WHERE id=333' } }
-
Implementing a simple audit system
NotionCommotion replied to NotionCommotion's topic in Application Design
Thanks scootstah, I've considered embracing something like Symfony2 for a while, but have not yet jumped in. Maybe some day soon? I agree it is good to store the actual change set if one is serious about audits. I am on the fence whether I even do anything, thought maybe something very simple is better than nothing. Good point about foreign key integrity. -
Do you mean rowCount()? http://php.net/manual/en/pdostatement.rowcount.php
-
I have found that implementing audit trails gets complicated and big quickly. I would like to take a simpler approach for a PHP application. Instead of logging content before and after the change, I would like to only log who and when a change was made to a given record in a table, and not the before or after values or even what columns were changed. My thoughts are something like the following: Use PHP to store the logged on users ID in a MySQL session. Set up a trigger whenever any column in a given table is changed, and store the table name, PK of the modified/deleted record, and timestamp in another table called audits. Ideally, I wouldn't store the actual table name as this would make it more difficult to ever changing table names (which hopefully is never necessary). Is there a systems MySQL table (i.e. used by the MySQL database) which could be used? In regards to storing the PK of the added/modified record (deletes will just be tagged as deleted) in the audits table, the only way I think I can use the DB to enforce foreign key integrity is creating a bunch of of one-to-one tables between audits.id and the audited table, and will probably just use the application to enforce. I would appreciate comments whether this approach is feasible and any recommended changes. Thank you
-
Well, I feel rather silly. Thanks for the help!
-
How can I cleanly and efficiently modify the following script so that I do not have to define the empty $user array twice? public function editRecord(){ $id=isset($_GET['id'])?$_GET['id']:null; if(is_null($id)) { $user=array('id'=>0,'firstname'=>'','lastname'=>'','username'=>'','access_id'=>10); } else { $sql='SELECT id,firstname,lastname,username FROM users WHERE id=? AND sites_id=?'; $stmt = $this->db->prepare($sql); $stmt->execute(array($id,$this->site->id)); if(!$user=$stmt->fetch(PDO::FETCH_ASSOC)){ $user=array('id'=>0,'firstname'=>'','lastname'=>'','username'=>'','access_id'=>10); } } $this->displayPage(__DIR__.'/templates/editRecord.html',array('user'=>$user,'access'=>$this->getList('access'))); }
-
In selectionTest.php, add the following to the top of the page. echo('<pre>'.print_r($_POST,1).'</pre>'); You see your input values? Note that project_id is likely not shown, so you will need to add it as a hidden input in your form. Now you need to save them in the database. $stmt=$conn->pdo('INSERT INTO user_project(first_name) VALUES(?) WHERE id=?'); $stmt->execute(array($_POST['first_name'],$_POST[' project_id']));
-
While you "could" use a different URL for different users, it is not recommended. Instead, why not just display different content from the same URL based on the user's logon status as defined by a session?
-
A while back, I used a singleton static class to share my database connection in various classes. Effectively, it was a global variable. I've since wanted to change my approach, and eliminate most if not all global variables. I am thinking something like the following. Any problems with this approach, or anything I should do to improve? Thanks $db=new PDO("mysql:host=localhost;dbname=dashboard;charset=utf8mb4",'myusername','mypassword',array(PDO::ATTR_EMULATE_PREPARES=>false,PDO::MYSQL_ATTR_USE_BUFFERED_QUERY=>true,PDO::ATTR_ERRMODE=>PDO::ERRMODE_EXCEPTION,PDO::ATTR_DEFAULT_FETCH_MODE=>PDO::FETCH_ASSOC)); $bla1=new bla1($db); $rs1=$bla1->getIt(); $bla2=new bla2(array('db'=>$db,'x'=>123)); $rs2=$bla2->getIt(); class bla1 { private $db; public function __construct($db) { $this->db=$db; } public function getIt() { $rs=$this->db->query('SELECT 1'); } } class bla2 { private $db; public $x; public function __construct($arr) { foreach($arr as $key=>$value) { $this->$key=$value; } } public function getIt() { $rs=$this->db->query('SELECT 2'); } }
-
Please review template parser
NotionCommotion replied to NotionCommotion's topic in Application Design
Hi Scootstah, I agree that Twig is great, but don't want most of the functionality; only replacing placeholders with different content. Also, I am just curious whether there are any mistakes in my approach. Please take a look and comment. Thank you -
Please review template parser
NotionCommotion replied to NotionCommotion's topic in Application Design
Hi Zane, Thank you for your response and thank you for moving my post to Application Design. In hindsight, I fully agree my original post was cryptic at best, and I should have given more context. Please let me start over. I wish to allow partially trusted users to easily create their own HTML templates using the "back end" application. They would be allowed to upload a CSS file, images, and a HTML file which will define the basic structure. My application would then take their HTML template file and convert it to a PHP file. When viewing their sites front end, my application would generate the HTML using the newly created PHP template, and populating it with various information or HTML blocks of code. In regards to being "partially" trusted, they are trusted not to implement XSS exploits, but I do not wish to give them access to the server other than described above. A template engine such as Twig is a possibility, however, I don't need (or want) all the features of Twig in the template. So, given the script described in my original post, is there anything a user could put in template.html which could give them access to the server or execute a PHP function? Thank you -
Please review template parser
NotionCommotion replied to NotionCommotion's topic in Application Design
I'll take it the lack of responses represents significant flaws. I will quick requesting comments. -
Please review template parser
NotionCommotion replied to NotionCommotion's topic in Application Design
Maybe not "review" is the right word. I am not looking for a detailed review, just whether the approach has gaping security liabilities. Any comments would be much appreciated. Thank you -
PHP and cURL - Multiform request with file and text
NotionCommotion replied to blackcell's topic in PHP Coding Help
Sorry, no direct answer. But instead of sending the file, maybe send the URL of the file, and have the responded get it? -
Please review template parser
NotionCommotion replied to NotionCommotion's topic in Application Design
Please? -
Who is legally responsible for XSS vulnerabilities?
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Or maybe the guy who sold the ladder that was used to build the house. -
Users directed to a page dependent on user level
NotionCommotion replied to coppens's topic in PHP Coding Help
May I ask why you are creating different versions of script for different PHP versions? I suppose this makes sense if you are creating a framework for others to use, but expect this is not the case. There also appears to be a bunch more superfluous script which doesn't appear to relate to providing a different home page for users of different access level. If it is a book or tutorial you are following, I would suggest you find another. Try to keep it simple. When the user logs on, store their ID in a session. When you page is accessed, get the user's access level from the DB, and use a switch statement or some other means to display the content desired. -
Please review template parser
NotionCommotion replied to NotionCommotion's topic in Application Design
Zero views after around 12 hours? It is not that bad. (EDIT. That is odd. Shows up as zero views, I add a post, and it changes to 62 views?) Also, it is only around 50 lines of code and not too much. By the way, template.html should be considered suspect and data (i.e. array('color'=>'red','dog'=>'ONE','name'=>'Burt') ) should be considered safe. Thanks -
Trying to pass variables to landing page after login
NotionCommotion replied to jygrnmny's topic in PHP Coding Help
Do you have errors enabled? Also, add "traps" along the way so you know you are executed the expected code. Use var_dump() as applicable. A couple of specifics... Login.php Is $user object created in inc/config.php? If not, it doesn't exist. Agree with requinx that $_POST['memberID'] and $_POST['firstname'] shouldn't be in the form. If they should be, then they should also be used in the verifyaction query $user->login(), else they should be obtained from the DB. Where is members.php? LANDING PAGE. Why set session with variables that are not defined? html page Not sure what you are trying to do.