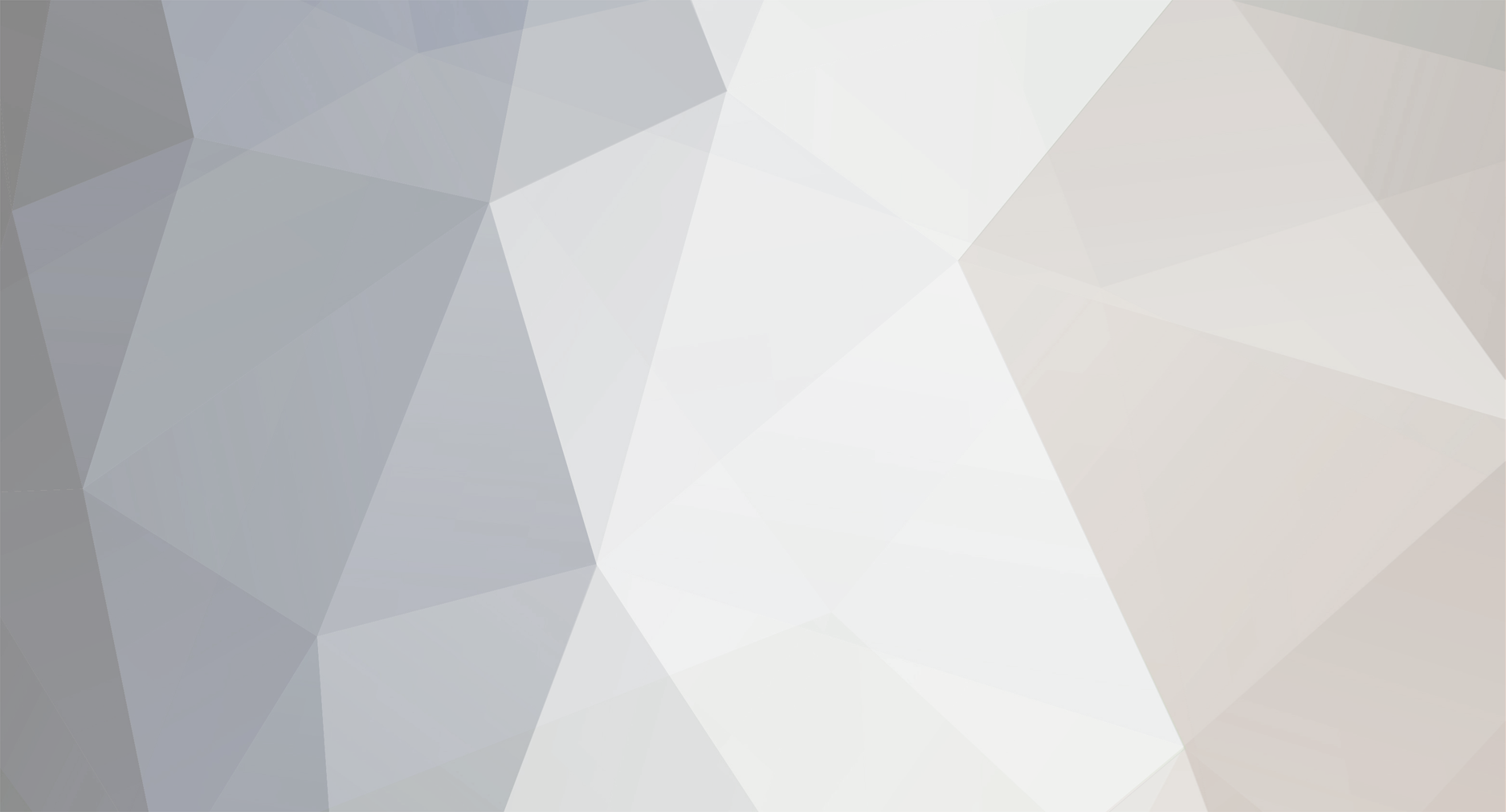
simcoweb
Members-
Posts
1,104 -
Joined
-
Last visited
Everything posted by simcoweb
-
[SOLVED] can't spot the goof causing this error...
simcoweb replied to simcoweb's topic in PHP Coding Help
Aha! Thank you! Sometimes when you stare at it for too long you can't spot the little things. I was concentrating on ; and }'s mostly. Thanks! -
My eyes are bugging out trying to spot the error causing this Here's the code: <?php // include our config files include 'db_config.inc'; // Turn on magic quotes to prevent SQL injection attacks if(!get_magic_quotes_gpc()) set_magic_quotes_runtime(1); // start processing if (isset($_POST['submitted'])) { $errors = array(); // validate the form input if (empty($_POST['Name']) || empty($_POST['Email']) || empty($_POST['Phone'])) { $errors[] = "<font face='Verdana' size='2' color='#FF0000'>Your name, email address and daytime phone number are required. Please complete those fields and resubmit.</font>"; } else { $name = mysql_real_escape_string($_POST['Name']); $phone = mysql_real_escape_string($_POST['Phone']); $email = mysql_real_escape_string($_POST['Email']); } // validate email address format if ($email) { if (!eregi("^[_a-z0-9-]+(\.[_a-z0-9-]+)*@[a-z0-9-]+(\.[a-z0-9-]+)*(\.[a-z]{2,3})$", $email)) { $errors[] = $email. " is not a valid email address. Please enter a valid email address.<br/>"; } } else { // run query $address = mysql_real_escape_string($_POST['Address']); $city = mysql_real_escape_string($_POST['City']); $state = mysql_real_escape_string($_POST['State']); $zip = mysql_real_escape_string($_POST['Zip']); $country = mysql_real_escape_string($_POST['Country']); $moving = mysql_real_escape_string($_POST['When_moving']); $where = mysql_real_escape_string($_POST['Where_moving']); $price = mysql_real_escape_string($_POST['Price_range']); $bedrooms = mysql_real_escape_string($_POST['Number_bedrooms']); $sqft = mysql_real_escape_string($_POST['Sqaure_feet']); $comments = mysql_real_escape_string($_POST['Comments']); $date = date("F j, Y, g:i a"); $sql = "INSERT INTO buyers ( Name, Email, Phone, Address, City, State, Zip, Country, When_moving, Where_moving, Price_range, Number_bedrooms, Square_feet, Comments, date) VALUES ('$name', '$email', '$phone', '$address', '$state', '$zip', '$country', '$moving', '$where', '$price', '$bedrooms', '$sqft', '$comments', '$date')"; $results = mysql_query($sql) or die(mysql_error()); if (mysql_affected rows($dbc) == 1){ // Send the E-Mail send_mails(); // redirect to confirmation page header("Location: confirmation.htm"); } else { header("Location: error.htm"); } } } ?> I've commented out line 50 which is send_mails(); function to see if it was something a step ahead of line 48. But that didn't fix it. Line 48 is: if (mysql_affected rows($dbc) == 1){
-
Trying to figure out why this login page doesn't work
simcoweb replied to simcoweb's topic in PHP Coding Help
Still looking for an answer to this riddle -
Trying to figure out why this login page doesn't work
simcoweb replied to simcoweb's topic in PHP Coding Help
I didn't post that part as it's a piece of the overall error checking. Here it is: if (isset($_POST['submitted'])) { $errors = array(); require_once ('db_config.php'); // validate first and last name fields are completed // run validation for username if (eregi('^[[:alnum:]\.\'\-]{4,30}$', stripslashes(trim($_POST['Username']))) ) { $user = mysql_real_escape_string($_POST['Username']); $query = "SELECT Username FROM users WHERE Username = '$user'"; $result = @mysql_query($query); $num = @mysql_num_rows($result); if ($num> 0) { $errors[] = '<font color="red">The username you have chosen has already been taken, please try again.</font>'; } else { $username = mysql_real_escape_string($_POST['Username']); } } else { $errors[] = '<font color="red">Please provide a valid username between 4 and 30 characters.</font>'; } // validate password if (!empty($_POST['Password1'])) { if ($_POST['Password1'] != $_POST['Password2']) { $errors[] = '<font color="red">The 2 passwords you have entered do not match.</font>'; } else { $password = $_POST['Password1']; } } else { $errors[] = '<font color="red">Please provide a password.</font>'; } // check to make sure email is valid format if (!eregi('^[a-zA-Z]+[a-zA-Z0-9_-]*@([a-zA-Z0-9]+){1}(\.[a-zA-Z0-9]+){1,4}', stripslashes(trim($_POST['Email'])) )) { $errors[] = '<font color="red">Please provide a valid email address.</font>'; } else { $email = mysql_real_escape_string($_POST['Email']); } // check other required fields if (empty($_POST['Email']) || empty($_POST['Phone']) || empty($_POST['Zip'])) { $errors[] = '<font color="red">Your email address, phone number and zip code are required. Please complete those fields and re-submit.'; } else { $phone = mysql_real_escape_string($_POST['Phone']); $zip = mysql_real_escape_string($_POST['Zip']); } -
Trying to figure out why this login page doesn't work
simcoweb replied to simcoweb's topic in PHP Coding Help
Ok, fixed the code for the login form in regards to the errors in the mysql query. Now, new issue. It's not recognizing the username/password combo generated by the registration form. I'm pretty sure it has something to do with how it's creating and handling the MD5 password. Not 100% on that but seems to be the place to look since I don't see how it can't match up with an unencrypted username. Here's the code from the 'register' script that generates the MD5 and insertion: // if no errors if (empty($errors)) { // create random activation code $a = md5(uniqid(rand(), true)); $date = date("F j, Y, g:i a"); $firstname = mysql_real_escape_string($_POST['First_Name']); $lastname = mysql_real_escape_string($_POST['Last_Name']); $sell = mysql_real_escape_string($_POST['Need_to_sell']); $preapproval = mysql_real_escape_string($_POST['Preapproval']); $have = mysql_real_escape_string($_POST['Have_Realtor']); $contact = mysql_real_escape_string($_POST['Contact']); $current = mysql_real_escape_string($_POST['Current_Realtor']); $when = mysql_real_escape_string($_POST['How_soon']); // run query to insert data $query = "INSERT INTO users (First_Name, Last_Name, Email, Username, Password, Phone, Zip, Need_to_sell, Preapproval, Have_Realtor, Contact, Current_Realtor, How_soon, active, date) VALUES ('$firstname', '$lastname', '$email', '$username', SHA('$password'), '$phone', '$zip', '$sell', '$preapproval', '$have', '$contact', '$current', '$when', '$a', '$date')"; // check results $result = mysql_query($query) or die(mysql_error()); Here's the login query to match it: // check for form submission if (isset($_POST['submitted'])) { $errors = array(); if (empty($_POST['Username']) || empty($_POST['Password'])) { $errors[] = "<h3>Error!</h3><p><font face='Verdana' size='2'>You must complete the username and password fields. Please try again"; } else { $username = mysql_real_escape_string($_POST['Username']); $password = md5($_POST['Password']); // validate username and password against the database $sql = "SELECT * FROM users WHERE Username = '$username' AND Password ='$password' AND status='1' "; $results = mysql_query($sql) or die(mysql_error()); if(mysql_num_rows($results) > 0) { $_SESSION['searchlog'] = $_POST['searchlog']; header("Location: search.php"); exit; } else { $errors[] = "<h3>Error!</h3><p><font face='Verdana' size='2'>An error has occurred for one of the following reasons:<p>Your username and password did not match our database. Please check your username and password you have on file and try again.<p>You have not activated your account. An email was sent to you when you registered that requires you to click on an enclosed link to validate your email address and registration. Check your email and follow the instructions. In some cases this email may be diverted to your spam or junk box by accident."; } } } -
Trying to figure out why this login page doesn't work
simcoweb replied to simcoweb's topic in PHP Coding Help
Heh... one silly * missing. Didn't notice it Well, that's why I have all you guys and your eyes! Now I just have to figure out why it's not recognizing the username/password that I know is in there. Probably something with the MD5 encryption where i'm not checking it right. -
Trying to figure out why this login page doesn't work
simcoweb replied to simcoweb's topic in PHP Coding Help
Well ain't that a hummer. I took your image idea there, lewis987, and now at least I get an error. Before I got nothing. The error is a MySQL error: You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'FROM users WHERE username = 'barfbag' AND password ='ea02465f625de29a9dfb11f1b3e' at line 1 the password is MD5 encoded as you probably noticed. Not sure what would be causing this error unless I have to reference the password field also as MD5 in the query. Anyone? On the image issue, I seem to now recall that you have to name the image something special in order for it to work. Anyone have some info on that? -
I'm using a simple 2 field form 'Username' and 'Password' and 'submitted'. It's checking against the database for existence, etc. etc. but nothing happens. Even with the errors. If I submit leaving the fields blank the errors don't display. It just refreshes the page. Hmmm. Code: <?php session_start(); // Seattle Viet Homes customer login include 'db_config.php'; // check for form submission if (isset($_POST['submitted'])) { $errors = array(); if (empty($_POST['Username']) || empty($_POST['Password'])) { $errors[] = "<h3>Error!</h3><p><font face='Verdana' size='2'>You must complete the username and password fields. Please try again"; } else { $username = mysql_real_escape_string($_POST['Username']); $password = md5($_POST['Password']); // validate username and password against the database $sql = "SELECT FROM users WHERE username = '$username' AND password ='$password' "; $results = mysql_query($sql) or die(mysql_error()); if($results == 1) { $_SESSION['searchlog'] = $_POST['searchlog']; header("Location: search.php"); exit; } else { $errors[] = "<h3>Error!</h3><p><font face='Verdana' size='2'>Your username and password did not match our database. Please check your username and password you have on file and try again.<p><a href='login.htm'>Click Here</a> to return to the login page."; } } } ?> Form code: <fieldset><legend>Customer Login</legend> <form method="POST" action="<?php $_SERVER['PHP_SELF']; ?>" name="searchlog"> <span lang="en-us"> </span> <input type="text" name="Username" size="16" value="" style="border: 1px solid #666666; padding: 2px"><br> <span lang="en-us"> </span> <input name="Password" size="16" value="" style="border: 1px solid #000000; padding: 2px"> <br> <span lang="en-us"> </span> <input border="0" src="images/login.gif" name="submitted" width="40" height="16" type="image"> <br> <input type="hidden" name="searchlog" value="searchlog"> <font face="Verdana" size="1"><span lang="en-us"> </span>Not registered? <a href="register.php">Click here!</a></font> </form> </fieldset>
-
[SOLVED] repost: need to display image within if/else statement
simcoweb replied to webguync's topic in PHP Coding Help
Cool! Yeah, once I saw the full code you had set a value for that variable so it made sense to use it since $row['importance'] had been assigned to it. Glad we got it! Be sure to mark as Solved! -
Not sure if anyone can help. This is an Xcart shopping cart that out of the blue started throwing this error without any changes in the config or files.
-
[SOLVED] repost: need to display image within if/else statement
simcoweb replied to webguync's topic in PHP Coding Help
This is a tricky one. Ok, let's try this. Since you're assigning the 'row' results to variables let's try using that in your 'if' statement. if ( $importance == 1 ) { -
[SOLVED] repost: need to display image within if/else statement
simcoweb replied to webguync's topic in PHP Coding Help
But your WHERE clause is narrowing it down to one entry: WHERE id = '$_GET[id]' If the id's are unique then you're only going to pull one person from that table. -
[SOLVED] repost: need to display image within if/else statement
simcoweb replied to webguync's topic in PHP Coding Help
You don't need to make it specific as long as you either name that field in the query, use the * for all, or.. name it in the WHERE clause. Since i'm not 100% sure on what your form is doing I can see from the query that you're passing the 'id' via the url and using that to select the proper item from the database. Now, if you were to add AND importance='1' to it then the screening would be done in the query. Then use an if/else to display if the rows come back showing true: if($result == 1) { echo "blah blah"; } else { echo "no love here"; } Something like that. -
[SOLVED] repost: need to display image within if/else statement
simcoweb replied to webguync's topic in PHP Coding Help
I think we need to look at your query for this. There query should contain a WHERE clause related to that field and the value. Once it pulls the correct data then the 'while' loop can be tweaked to display it. The if/else will probably need a tweak or two depending. Post your query code. At least we figured out it's not setting the value. -
I found the issue. In the !eregi line I refer to the $_POST['email'] when it should be 'Email' (upper case E). So, no more eregi error but there's another issue that i'll have to post in the other forum. Thanks!
-
[SOLVED] repost: need to display image within if/else statement
simcoweb replied to webguync's topic in PHP Coding Help
If it's 'bypassing' the if then that probably means that condition is not being met. Only way to find out is to echo that to see if there's actually a value set. I think this will work and if displays the value of that field then the value is being set. Otherwise, if it's not then nothing is matching up with 1: <tr> <? if ( $row['importance'] == 1 ) { $test = $row['importance']; echo $test; echo "<td colspan='3'>".$img."</td>"; } else { echo "<td colspan='3'>not so important.</td>"; } ?> </tr> also, I have image set as : $img= "<img src='images/mymage.gif' width='25' height='25' alt='myimage'>"; the field in MySQL is named 'importance' and some of the values in that field = 1. That is when I want the image to display. <? if ( $row[10] == 1 ) { echo "<td colspan='3'>".$img."</td>"; } else { echo "<td colspan='3'>not so important.</td>"; } ?> -
Hey, thanks for the post. And the description! It helps to learn it. Unfortunately it's still throwing an error even with that modification. I'm wondering if there's a way to have the error display exactly what is causing the issue so we can narrow down what segment of the regex is not being fulfilled. Anyone?
-
This email field validation is showing the error message that the email is not valid. I can't see where/why it would decline to validate this email address: bozotheclown@hotmail.com Here's the code: // check to make sure email is valid format if (!eregi('^[a-zA-Z]+[a-zA-Z0-9_-]*@([a-zA-Z0-9]+){1}(\.[a-zA-Z0-9]+){1,2}', stripslashes(trim($_POST['email'])) )) { $errors[] = '<font color="red">Please provide a valid email address.</font>'; }
-
[SOLVED] repost: need to display image within if/else statement
simcoweb replied to webguync's topic in PHP Coding Help
Post the code you're using now. -
I like to do it like this: $sql = mysql_query("SELECT * FROM shopping_products ORDER BY product_id"); $numrows = mysql_num_rows($sql); if($numrows == 0){ echo "There are no results available."; } else { echo "<table><tr><td>WAZZA!</td></tr>"; // this is outside the loop while($row = mysql_fetch_array($sql)) { echo "<tr><td>THAT'S RIGHT!</td></tr>"; } echo "</table>"; // this is outside the loop }
-
[SOLVED] repost: need to display image within if/else statement
simcoweb replied to webguync's topic in PHP Coding Help
Good stuff to know, frost. -
[SOLVED] repost: need to display image within if/else statement
simcoweb replied to webguync's topic in PHP Coding Help
I believe your other question was answered by Mr. Sheen about the if/else statement. -
[SOLVED] repost: need to display image within if/else statement
simcoweb replied to webguync's topic in PHP Coding Help
Just a quick question for my own edification. Array values always start with [0], as i've been told. Therefore, wouldn't the f15 position be [9] instead of [10]? -
Change to this: <?php if ($priv === true) { echo "<a href="post_topic.php">update_banner</a>"; } ?>
-
Fresh pair of eyes needed please! PHP/SQL prob
simcoweb replied to bladechob's topic in PHP Coding Help
Ok, quick question. You have just one variable, $hcg=$_POST['hotelclientgroup']; but about 30 fields. You need to set the values for each of those. Where is that being done? If there's no errors being thrown then something is being inserted. Most likely just the auto-incremented id field and the 'hotelclientgroup' field since that's the only variable being given a value in the $_POST.