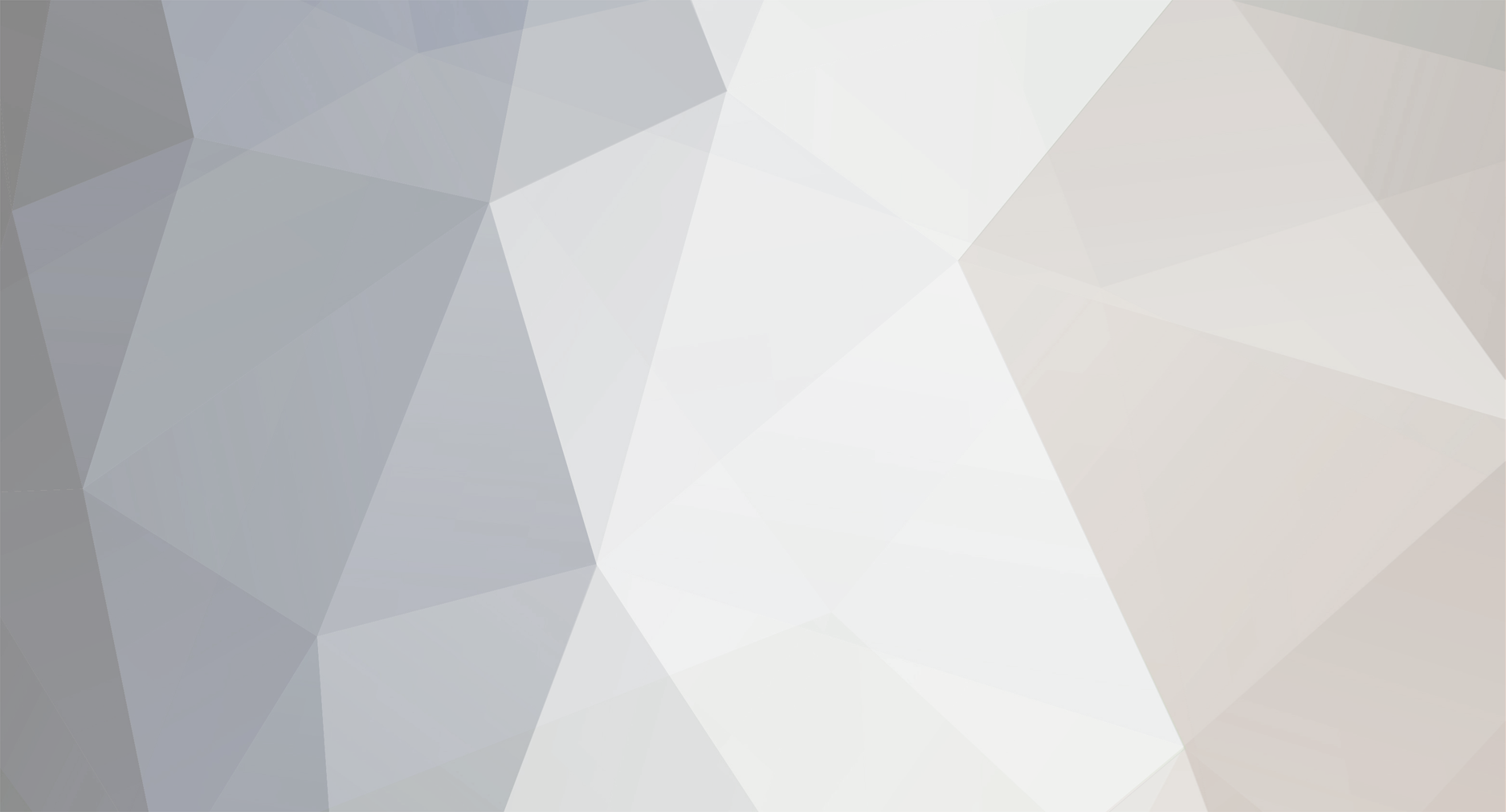
simcoweb
Members-
Posts
1,104 -
Joined
-
Last visited
Everything posted by simcoweb
-
Write a script for the 'delete' parameters and then make a link to it using the id in the url. Like this: <a href="delete.php?id=$id">Delete</a> Then on the delete page you'd need: $id = $_GET['id']; $sql = "DELETE FROM tablename WHERE id='$id'"; $results = mysql_query($sql) or die(mysql_error());
-
Fresh pair of eyes needed please! PHP/SQL prob
simcoweb replied to bladechob's topic in PHP Coding Help
There's some repetitious elements in these: dbconnect(); // add new relationship $hcg=$_POST['hotelclientgroup']; if(!empty($hcg)){ $sql2="INSERT INTO client_pref_venue VALUES ('','$hotelid','$hcg','$pegsCode')"; if(!$insert=mysql_query($sql2,$conn))echo mysql_error(); } dbconnect(); // add to venuemain db $hcg=$_POST['hotelclientgroup']; if(!empty($hcg)){ $sql4="INSERT INTO venue_main VALUES ('','$hotelclientgroup','$hotelid','$name','$groupname','$address1','$address2','$address3','$town','$county','$tel_main','$postcode','$country','$tel_res','$tel_conf','$fax_main','$fax_res','$fax_conf','$email_main','$email_res','$rating','$type','$region','$special','$rooms','$parkins','$airport','$airportdist','$motorway','$motorwaydist','$railway','$railwaydist','$notes','$acctel','$accfax','$accemail','$currency','$cancpol','$terms','$extpark','$parkdist','$free','$website','$graphic','$pagabflag','$kcflag','$cceflag','$kcpagabflag','$description','$blacklisted')"; if(!$insert=mysql_query($sql4,$conn))echo mysql_error(); } Try this. See my notes included: dbconnect(); // add new relationship $hcg=$_POST['hotelclientgroup']; if(!empty($hcg)){ $sql2="INSERT INTO client_pref_venue VALUES ('','$hotelid','$hcg','$pegsCode')"; if(!$insert=mysql_query($sql2,$conn))echo mysql_error(); } // dbconnect(); //only need this once // add to venuemain db // $hcg=$_POST['hotelclientgroup']; // don't need this as it's already declared if(!empty($hcg)){ $sql4="INSERT INTO venue_main VALUES ('','$hotelclientgroup','$hotelid','$name','$groupname','$address1','$address2','$address3','$town','$county','$tel_main','$postcode','$country','$tel_res','$tel_conf','$fax_main','$fax_res','$fax_conf','$email_main','$email_res','$rating','$type','$region','$special','$rooms','$parkins','$airport','$airportdist','$motorway','$motorwaydist','$railway','$railwaydist','$notes','$acctel','$accfax','$accemail','$currency','$cancpol','$terms','$extpark','$parkdist','$free','$website','$graphic','$pagabflag','$kcflag','$cceflag','$kcpagabflag','$description','$blacklisted')"; // if(!$insert=mysql_query($sql4,$conn))echo mysql_error(); // let's change this to below $results = mysql_query($sql4) or die(mysql_error()); if(!results) { echo "something is wrong with the data insertion."; } } -
Fresh pair of eyes needed please! PHP/SQL prob
simcoweb replied to bladechob's topic in PHP Coding Help
Is this throwing an error? -
Do you mean have it emailed to multiple addresses?
-
Another way: $sql= "SELECT username FROM user_table WHERE username='$user'"; $result = mysql_query($sql) or die(mysql_error()); if($result) { echo "In use. Try again."; } else { echo = "Not in use. Congrats!"; }
-
[SOLVED] Darn code's not doin' what I want it to do...
simcoweb replied to christofurr's topic in PHP Coding Help
thorpe, that's what I was trying to say -
[SOLVED] Darn code's not doin' what I want it to do...
simcoweb replied to christofurr's topic in PHP Coding Help
I can definitely see where you could shorten your code and make it more efficient. For two form fields you have an awful lot of code there Examples: Change the echo statements to an array of error messages that you can display. So instead of this: if (empty($_POST["nickname"])) { echo "You forgot to tell us what you want your nickname to be.<br />"; } You'd do something like this: <?php $errors = array[]; if(empty($_POST['nickname'])) $errors = "You did not enter a nickname. Please complete that field."; That way you can loop through all the errors at one time instead of individually. Conceivably someone could have to resubmit your form a half dozen times to get through all the errors one at a time. To loop them you'd insert this just above your form code: <?php if(!empty($errors)) { echo "<font color='red'><strong id='errorTitle'>One or more input fields on the form has not been completed</strong>"; echo "<ul>"; foreach ($errors as $value) { echo "<font face='Verdana' size='2' color='red'><li>$value</li></font><br/>"; } echo "</ul>"; } ?> That way it would list all their errors at once in a list format. Also, you can consolidate some of your 'if' statements. For example, instead of this: if (empty($_POST["nickname"])) { echo "You forgot to tell us what you want your nickname to be.<br />"; } else { echo "You requested for your nickname to be <b>" . $_POST["nickname"] . "</b>.<br />"; if (!ereg('([a-zA-Z0-9]+[:space:]*){1,33}', $_POST["nickname"])) { echo "Your nickname can only consist of alphanumeric characters (A-Z & 0-9) and spaces. Please check your input.<br />"; You might do this: if (empty($_POST["nickname"]) || if (!ereg('([a-zA-Z0-9]+[:space:]*){1,33}', $_POST["nickname"]))) $errors = "The nickname field was empty or did not match our requirements. Nicknames must be between 3 and 35 characters, contain letters A-Z, numbers 0-9 and can contain spaces. Please try again."; This means 'either or' fails and the error displays. -
Does it throw an error?
-
[SOLVED] help error after submit [need help urgently]
simcoweb replied to chris_rulez001's topic in PHP Coding Help
You need a closing } before the ?> tag. -
Use either of those. I use XAMPP but on XP. Make sure their version is up to date for Vista. It has all you need.
-
[SOLVED] I don't need Cialis or Viagra... how can I trim that out?
simcoweb replied to simcoweb's topic in PHP Coding Help
I love that you're modest, too! :) Ok, I had to make a couple of tweaks (dare I say corrections?) to your snippet but got this to work: <?php $words = "cialis,viagra,phentermine"; $wordList = explode(",", $words); $check = trim($_POST['comments']); if (is_spam($check, $wordList)) { header("Location: reject.htm"); // Note the header code inside the if.. echo $check . " has been flagged as spam<br />"; // echo "To boot them out place header code here!!!"; exit; } else { ?> where you had the 'echo "To boot them out place header code here!!!"; had to be moved up or deleted since it caused a header error. I also had to change this: echo $test . " has been flagged as spam<br />"; as $test wasn't used anywhere but $check was. I may just turn this into an $error that would be displayed on the reject page. Not sure, though, since giving the spammer a clue as to what the word(s) was may not be a good idea. Anyway, with the 'tweaks' and your simple function I tested it and was able to successfully get redirected if using the wrong words in the comments box (even though they've used the name field for that as well but primarily the comments box is the one they flood) and also was able to get the spam error message to echo but at the expense of not having it redirect. That's why i'll probably turn it into an error that would display on the next page. -
[SOLVED] I don't need Cialis or Viagra... how can I trim that out?
simcoweb replied to simcoweb's topic in PHP Coding Help
frost, you rock, dude. Ok, couple of questions. Because there's SOOOOOOOO many words that need to be checked and the prospect that the list would grow weekly I felt it best to utilize an external file or possibly even a database for your $words variable/array. 2nd, the string to check is unknown until they post. So, my original snippet had: $check = trim($_POST['comments']); Can that method work... and... would it be wise to take the 'comments' posted content and also explode it and compare the $words with the $check? -
[SOLVED] I don't need Cialis or Viagra... how can I trim that out?
simcoweb replied to simcoweb's topic in PHP Coding Help
Heh, well I figured i'd spent enough time on this so I just integrated a CAPTCHA setup into the form. Works fine and we'll see if it stops the bulk of the spammers. I'm sure it'll have an impact. Honestly it's not my preferred method but I needed something in the interim until the real method is debugged. Once I get through with it the spammers will get biatch-slapped. frost, I didn't just disregard your 'spam' checker. I'm looking at that as well and how I can adapt it. What it was missing was the rejection I want ( IF spam GETTHEBOOT ) which is what I was trying to work out in my original code but couldn't seem to get it to work. In regards to a 100% solution, there may be one today. But by tomorrow those spamjerks will have it figured out and a way to bypass it I would, though, like to continue to create the banned word functionality and when I do i'll post back here. Based on the number of views and the sheer number of sites having forms affected by spammers (one of our clients has a shopping cart with customer product reviews which we had to turn off due to spammers) it could be a nice bit of code to share. Any ideas are welcome. Thanks to all that posted! You guys rock! -
[SOLVED] I don't need Cialis or Viagra... how can I trim that out?
simcoweb replied to simcoweb's topic in PHP Coding Help
The CAPTCHA can prevent the bots but most of these are humans going right past it, unfortunately. A better way to discourage them is to see the look on their face when the form boots 'em out. After a dozen more tries they'll probably give up and go bug someone else. I'm trying to figure out why the code is not matching the words and executing properly. I'm not getting any errors but it's not working either in regards to comparing the entries in the form field with the banned words and exiting if found. -
[SOLVED] I don't need Cialis or Viagra... how can I trim that out?
simcoweb replied to simcoweb's topic in PHP Coding Help
Hi frost. But I didn't use 'Generic viagra' in the test. Just the word 'generic'. In the previous tests I used two words, 'viagra' and 'cialis'. So based upon those shouldn't it have displayed as follows?: generic viagra cialis levitra And, the golden question, why isn't it flagging the banned words and exiting? It's obviously matching up. But what happens is it continues with the 'else' code which is to add it to the database and send the emails. -
[SOLVED] I don't need Cialis or Viagra... how can I trim that out?
simcoweb replied to simcoweb's topic in PHP Coding Help
Still looking for a fix on this As an experiment I submitted the form with the word 'generic' in the comments box (previous tests all used 'viagra' and 'cialis'). What happened that was different than previous tests is the keyword display showed two lines instead of one. Check it out: The 'debug' code is set to loop through all the keywords but for some reason that's not working: if (isset($_POST['Submit'])) { // our attempt to stop spammers $wordfile = file_get_contents('words.txt'); $notallowed = explode(",",$wordfile); $str = strip_tags(trim($_POST['comments'])); echo "String to Test against: " . $str . "<br />"; foreach ($notallowed as $keyword) { echo "Keyword: " . $keyword . "<Br />"; $keyword = trim($keyword); if(preg_match('/'.$keyword.'/i',$str)) { //header("location: reject.htm");exit; //Or whatever the logout url is } else { As another experiment I changed the 'words.txt' file to a comma delimited format. This is supposed to check against the banned words and, if found, exit the script. -
change: while ($row = mysql_fetch_array($results)) { to: while ($row = mysql_fetch_array($result)){ without the 's' on $result
-
[SOLVED] I don't need Cialis or Viagra... how can I trim that out?
simcoweb replied to simcoweb's topic in PHP Coding Help
That's pretty much what we're trying to do. But, the way it figures, I don't want to let someone keep trying if they typed in viagra, cialis, phentermine, etc. in their first attempt. That's a spammer. The goal is to get rid of them on the first try so they don't figure out a way to modify the spelling and get it through (ex: c1alis, c*i*a*l*i*s, etc. instead of cialis). The site is about foreclosure proceedings. Viagra has no place in that conversation (Hi, Mr. Realtor, I was taking some viagra today and remembered my house was in foreclosure...). I really want it to check/filter against the restricted words and if found it bounces their asses outta there before it submits the data to the database and emails the site owner with the results. We're close but need a tweak or two to get it there. Any ideas? -
[SOLVED] I don't need Cialis or Viagra... how can I trim that out?
simcoweb replied to simcoweb's topic in PHP Coding Help
wildteen, I tried that and it go t the same error message since there's another header function later in the code: -
[SOLVED] I don't need Cialis or Viagra... how can I trim that out?
simcoweb replied to simcoweb's topic in PHP Coding Help
*ahem*...the contents of the 'words.txt' file are not appropriate for young eyes. So, i'll post the 'edited' version Regarding the form, dohhhhhh.. honestly i'm not that dumb. The $_POST['comments'] form field was mislabeled. I corrected it and resubmitted. Here's the new message: So, it appears it's testing now but the Keyword: generic just shows the first line of the words.txt file. viagra is the 2nd line and cialis is the 3rd. -
[SOLVED] I don't need Cialis or Viagra... how can I trim that out?
simcoweb replied to simcoweb's topic in PHP Coding Help
frost, the key words are on separate lines. I changed the "," in the explode to \n and ran it: 'generic' is the first word/line in the words.txt file. It's still no exploding them all apparently. -
[SOLVED] I don't need Cialis or Viagra... how can I trim that out?
simcoweb replied to simcoweb's topic in PHP Coding Help
I get this: First time i've seen the header error. Would that have an effect on the script parsing up to that point? Line 95 is this: header("Location: download.php?actionflag=registered"); exit; Which is basically the last lines of the script which shouldn't even come into play IF the word filter did its job. That line is IF there's no errors and everything is a success. Apparently the word filter is getting pushed away, not working, etc. but it's in some way competing with the header() function. -
[SOLVED] I don't need Cialis or Viagra... how can I trim that out?
simcoweb replied to simcoweb's topic in PHP Coding Help
frost, i'll do some checking to see if it's reading the file. I would assume that if it wasn't then the script would just fail. Since it didn't, then I figured the next thing is the code is just not doing what it's supposed to... open the file, compare the words against the $_POST['comments'] content, declare any matches and do what's appropriate. Either it's clean or it's rejected. Does that part of the code make sense to the point it should work? -
[SOLVED] I don't need Cialis or Viagra... how can I trim that out?
simcoweb replied to simcoweb's topic in PHP Coding Help
grrrrrrr... ok, this is not working. After testing the form and inserting the forbidden key words 'cialis viagra' into the comments section it did NOT reject me and forward me to the proper location. Instead it went ahead and submitted the form and inserted the data into the database: if (isset($_POST['Submit'])) { // our attempt to stop spammers $wordfile = file_get_contents('words.txt'); $notallowed = explode(",",$wordfile); $str = strip_tags(trim($_POST['comments'])); foreach ($notallowed as $keyword) { $keyword = trim($keyword); if(preg_match('/'.$keyword.'/i',$str)) { header("location: reject.htm");exit; //Or whatever the logout url is } else { The logic appears sound, no errors and the word file is present with the forbidden words. Ideas? Help?