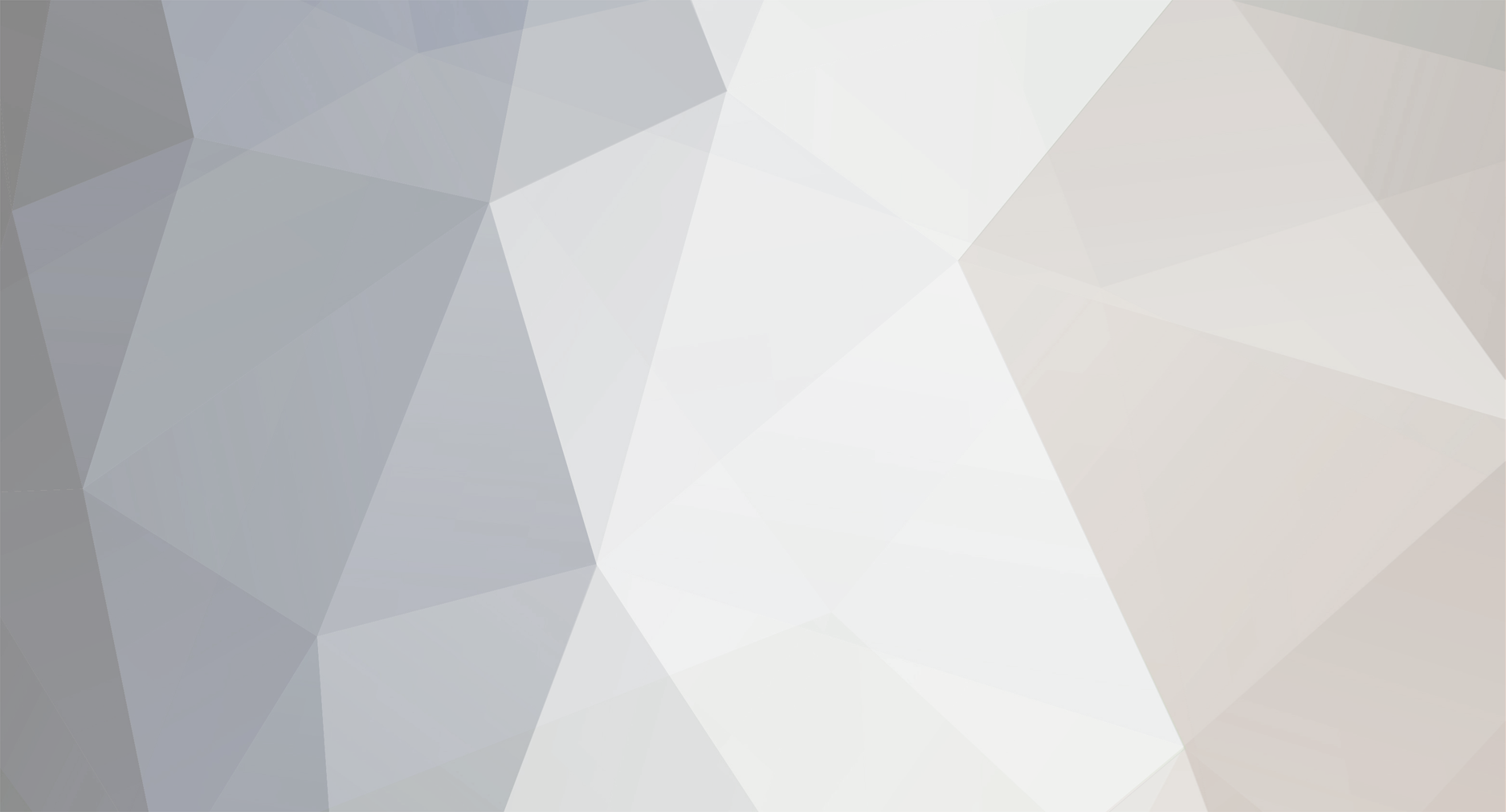
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
You need to check the return value of every call to mysql_query(). A simple way is like this: mysql_query($query) or die("Error in $query\n" . mysql_error());
-
As an aside, there is no need to extract($row) if you are going to explicitly set $id, $photo1 and $gender below. They both perform the same action (though the extract will set all variables, not just the ones you want).
-
Use this in place of all your queries: mysql_query($query) or die("Query failed: $query\n" . mysql_error()); That will tell you if any queries fail (probably for syntax reasons).
-
Try "echo $query;", and take a look at it. Also try adding this: ini_set('display_errors', 1); That'll make sure php tells you why it is stopping. If you're still having trouble, post your entire script here and we'll take a look
-
http://dev.mysql.com/doc/refman/5.1/en/fulltext-fine-tuning.html
-
The short answer is "You don't". You can do this to find out: $request_vars = array_keys($_REQUEST);
-
Alternatively, you could stop relying on the bug. How do you set your cookie and how do you access it? Can you show us the code?
-
There's no "GETFIELDS" .. just alter the url like this: $get_url = $bcURL . '?' . $data; Then get rid of the HEADER, GET and GETFIELDS lines. As GET is the default action, there is no need to specify that curl should use it.
-
Parsin URL from string then convert to hyperlink....
btherl replied to Sonic88's topic in PHP Coding Help
When does it work and when does it not? Give us a few examples and we should be able to fix it -
[SOLVED] Help! Code error is stumping me!
btherl replied to techiefreak05's topic in PHP Coding Help
Try printing out the pattern you are matching, as well as the string you are matching against. They must match exactly. -
It's ok, sprintf is confusing That's normal. Basically, ths %s and %d will be filled in by the other arguments to sprintf, in the order they appear. The first mysql_real_escape_string() will go in the first %s, and the second one in the second %s Then finally, $_POST['user_id'] will be converted from an integer to a string and placed in %d. The general rule is you use %d for integers, %f for floating point numbers and %s for strings. This can be confusing in PHP, since strings and integers and floats are often converted automatically. sprintf() is based on a C function, where such conversions had to be done explicitly.
-
Without knowing your table structure and the purpose of the query, the only odd thing I notice is that you select user, but you group by client. Normally you will select the column you group by.
-
Can you show us the error from mysql rather than making us guess?
-
passing variables, arays, objects by reference
btherl replied to Liquid Fire's topic in PHP Coding Help
Yes, PHP uses "copy on write" for function arguments. If you pass a huge array into a function, it will not be copied unless you modify it, in which case a copy is triggered at the point of modification. Similarly with foreach loops over an array - if you modify the original array within a foreach loop, then a copy is triggered, and all modifications are made on the copy. At the end of the foreach loop, the original array is replaced with the modified version. This allows a foreach loop to run consistently even if the array is modified during the loop. The only thing I use references for in PHP is when I want to pass a large data structure to a function which will modify that data structure, such as a function that will fill in extra data in a large array. I can't help with the class stuff, sorry.. but I'm pretty sure that PHP5 does NOT need references when creating objects. PHP4, maybe. If you don't get a reply, try asking in the OO sub-forum. -
I personally find nested functions difficult to read. I would write it. $topicname_stripped = strip_tags($_POST['topicname']); $topicname_esc = mysql_real_escape_string($topicname_stripped); It's an extra line, but the combined benefit of seperate variable names and a single function per line outweighs the increase in number of lines, in my opinion. Plus, with this method you know that you must only use a "$varname_esc" in your mysql, and never the original "$varname". But it's all a matter of preference. A good exercise is to look over old code that you wrote and see which parts you can understand easily, and which parts take some effort. Whatever is easy to understand, use that style more often. Whatever is confusing, stop using that style
-
Help with querying MySQL database with a form
btherl replied to Swerve1000's topic in PHP Coding Help
id is typically used by javascript or CSS to uniquely identify an HTML tag. It's nothing to do with SQL, although SQL very often has its own ids to uniquely identify a row of data. So although it's "id", it's not related at all to the ids you see in SQL. Its purpose is similar though - unique identification of a thing. Hope that clarifies things! -
You can use the "complex" syntax (which is actually simple): echo "Your IP is {$_SERVER["REMOTE_ADDR"]}";
-
FYI, here is the output on a 64 bit php: 2^0 = 1 2^1 = 2 2^2 = 4 2^3 = 8 2^4 = 16 2^5 = 32 2^6 = 64 2^7 = 128 2^8 = 256 2^9 = 512 2^10 = 1024 2^11 = 2048 2^12 = 4096 2^13 = 8192 2^14 = 16384 2^15 = 32768 2^16 = 65536 2^17 = 131072 2^18 = 262144 2^19 = 524288 2^20 = 1048576 2^21 = 2097152 2^22 = 4194304 2^23 = 8388608 2^24 = 16777216 2^25 = 33554432 2^26 = 67108864 2^27 = 134217728 2^28 = 268435456 2^29 = 536870912 2^30 = 1073741824 2^31 = 2147483648 2^32 = 4294967296 2^33 = 8589934592 2^34 = 17179869184 2^35 = 34359738368 2^36 = 68719476736 2^37 = 137438953472 2^38 = 274877906944 2^39 = 549755813888 2^40 = 1099511627776 That'll give you at least 63 bits to play with (and the 64th too if you are careful with it). But again there is a hard limit based on the size of an integer.
-
Try the following script: <? foreach (range(0,40) as $pow) { print "2^$pow = " . intval(pow(2,$pow)) . "\n"; } ?> If your php uses 32 bit integers, you should see it fail at 2^31.
-
Are you using unix or windows?
-
Here is a curl function to fetch a url: function fetch_url($url) { $ch = curl_init($url); curl_setopt($ch, CURLOPT_HEADER, 0); curl_setopt($ch, CURLOPT_FAILONERROR, 1); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1); $content = curl_exec($ch); return $content; } You should probably use it like this: echo fetch_url("http://vsevjednom.cz/guestbook.php?gb=1248&odpoved=".$_GET['odpoved']."&gb_pg=" .$_GET['gb_pg']."&hledat=".$_GET['hledat']."&ie=".$ie);
-
[SOLVED] Error in Code? Can anyone be my Hero and Spot it?
btherl replied to jeaker's topic in PHP Coding Help
Oh, I didn't look properly. You should use mysql_query($sql), not mysql_query($query) -
[SOLVED] Error in Code? Can anyone be my Hero and Spot it?
btherl replied to jeaker's topic in PHP Coding Help
Can you show us the output of "echo $sql;" just after you set $sql for your delete statement? -
I would start by working out how to calculate the number of days between the post date and now. These functions will help: http://sg.php.net/manual/en/ref.datetime.php That's the messy part.. after that, you just need to decide on what color you want for how many days. You might want to setup some colour settings in css for each age, so you can modify the colours for each age group by modifying your main css file.
-
That description is very general.. do you have something more specific in mind? Are you looking at changes between two rows that differ over some axis (like time or location)? Are graphs and tables the most common visual and numeric reports you want to generate? And is it only ever 2 rows, or sometimes 3 or more?