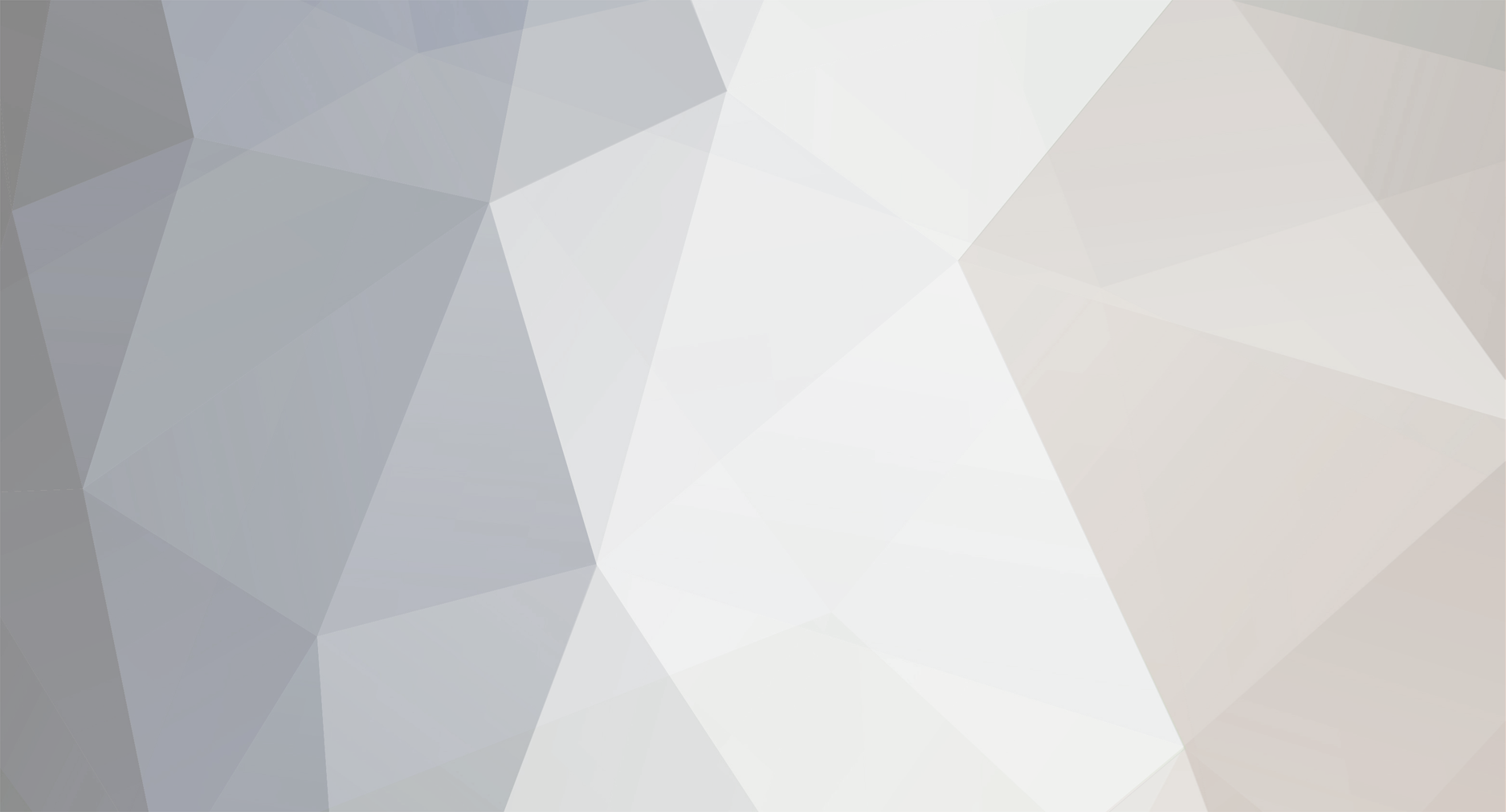
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Can you try this (if the ip column is in the users table): $sql="UPDATE users SET activationkey = '', status='activated', ip = '{$_SERVER['REMOTE_ADDR']}' WHERE (id = $row[id])"; It should replace the existing update query. You may also want to replace the select with this: $query = "SELECT * FROM users WHERE activationkey = '" . mysql_real_escape_string($queryString) . "'"; But that isn't necessary to get the script to work, it's just a sensible optimization.
-
Simple PHP based Web Application!!help needed!!
btherl replied to Adnan628's topic in PHP Coding Help
Ok, those links fugix gave are the manuals for the SQL commands you need. In each case you will want "WHERE id = $id" to ensure that you update or delete only the requested data, and not any other. Apart from that, updating and deleting is not much different from inserting new data. The id can be passed with a hidden input in the form. -
Do you have a script already? If you do, please post it. If you don't have anything yet, I suggest looking for a tutorial or some sample code in google first. Eg you can search for "php activation link code" or "php activation link tutorial"
-
Simple PHP based Web Application!!help needed!!
btherl replied to Adnan628's topic in PHP Coding Help
Does your phone book table have a primary key? -
One approach is to make an associative array with the distinguishing feature as the key (aka a hash table). $lookup_table = array(); foreach ($data as $d) { $key = $d['day'] . "-" . $d['item']; if ($lookup_table[$key]) { # Merge $d into existing data in $lookup_table[$key] } else { $lookup_table[$key] = $d; } } And then you can loop through $lookup_table to get the merged data.
-
Congratulations Recursion is tricky but once you get used to it it's very useful.
-
MD5 hashes have 128 bits = 16 bytes. The number of possible combinations is therefore 2^128, which is a lot. Assuming we need 0 space for indexing (which is not true), this still requires 2^128 * 16 bytes to complete the rainbow table. To give an idea of the space required: 2^10 * 16 bytes = 16kb 2^20 * 16 bytes = 16mb 2^30 * 16 bytes = 16gb 2^40 * 16 bytes = 16 tb 2^50 * 16 bytes = 16 pb 2^60 * 16 bytes = 16 eb 2^70 * 16 bytes = 16 zb ... 2^128 * 16 bytes = ? Well, you get the idea. We don't even have a name for the amount of storage required to make a rainbow table for the sha1() of all 32 character md5 hashes. Such a rainbow table will not exist for the likely lifetime of the application. A rainbow table could be generated for just the md5 hashes in the exisiting md5 rainbow table, but I am not aware of any such table existing. Using a unique per-user salt is still a good idea, even though a rainbow table for all 32 character inputs is not possible. requinix, I don't agree with you there. Although entropy is not increased by applying sha1(), the difficulty of an attack is increased. The whole point of a rainbow table is that it is pre-generated and can be shared between attacks. md5() has rainbow tables because it is commonly used, but I'm not aware of any rainbow tables for sha1(md5()).
-
Recursion always has a base case and a recursive case. You need to be clear on what to do for each one. 1. Base case - the tree node has no children. What action to take? Is the correct action to return an empty array? 2. Recursive case - the tree node has a left child. What action to take? Process left child, keep results in an array, and .. 3. Recursive case - the tree node has a right child. What action to take? Process right child, keep results in an array, and combine with any results from recursive case 2. Then return combined results. Now the questions I have for you: 1. What does your code do if the node has no children? What value does it return? It should be an empty array, written "array()", which is different from an uninitialized variable. PHP won't like it if you try to merge an uninitialized variable with an array. 2. What does your code do if the left child exists but the right child doesn't? It should process just the left child if only the left child exists. Same with the right child. The problem with the arrays being inside arrays is because you used $eh[] instead of $eh, and $eh1[] instead of $eh1. wildteen is correct there, and adding the brackets will only give the wrong results, it doesn't actually fix the problem. The actual problem was that your function does not always return an array, it sometimes returns an unintialized $child.
-
That should be: $testemail3 = trim(preg_replace('/\)$/','', $testemail2)); Because the end of the string, denoted by "$", occurs after the final bracket. If you are ok with removing all brackets instead of just the first and the last you can use str_replace(array('(', ')'), '', $string);
-
if statement inside if statement not working
btherl replied to HDFilmMaker2112's topic in PHP Coding Help
This code $_SESSION['username']=$_POST['username']; $_SESSION['password']=$_POST['password']; will overwrite the session data as soon as you access another page. You should put another "if" around it to check if the login form is being submitted. -
I don't see why sha1(md5()) won't work. And you can apply it to your existing database of users. Make double sure it's correct though, and keep a backup of the original md5 passwords (eg on a usb stick) in case it goes pear shaped. There's a few things it'll achieve 1. sha1(md5()) takes longer to compute than md5(), making brute force cracking take a bit longer. 2. Rainbow tables typically use md5() only, so rainbow tables won't work. 3. Out of the box cracking tools typically won't use sha1(md5()), so someone without programming knowledge will have more difficulty programming a cracking attack correctly. You will still benefit from unique salts for each user though, regardless of whether you use sha1(md5()) or md5().
-
How are you storing your permissions? The "$permission == 'admin'" test in my code was just an example. The exact test depends on how your permissions are stored. I would expect to see a column in the members table called "permissions", with some value stored in there. For a more complicated permission system you might have a whole new table with many types of permissions, but you probably just need something basic like "admin" and "user". Do you know how to add a column to a table? And how to read the contents of the column to use it in php? And how to change the contents? The reason I ask is so I know whether to focus on general concepts like "store the permissions in a table" or if we need to look at the details of how that storing happens.
-
For the first question, md5 with salt is perfectly adequate for an online game. Your time is better spent on other things than trying to use SHA2. As for question 2, I'm not sure if I misunderstand you but it sounds like you can do something like this: if ($permission == 'admin') { print "<a href="/link.html">I'm a secret link</a>"; } PS Which game are you re-making?
-
Please help this code is Zero division(FUZZY C MEANS)
btherl replied to edrianhadinata's topic in PHP Coding Help
What does that code do? -
The Mayhem, try printing out the values you are about to compare using var_dump(). That might show what the problem is. Alternatively you can print urlencode($string1) and urlencode($string2), which can also help to find differences in the strings. Zurev's suggestion is not quite correct - it will return false if the string is found and it evaluates to 0. What you have there with strlen() will work, but the most straightforward way is this if (strstr($string1, $string2) !== false) { print "YES<br />"; } strstr() will only return false if the string is not found, so this comparison will work 100% of the time.
-
If it doesn't get past the "if", print out the values that are being compared.
-
Get only first three occurances with preg-match_all()
btherl replied to Psycho's topic in Regex Help
preg_match_all() applies the full expression multiple times, so that means "Find this pattern 1-3 times as many times as possible", which isn't quite what you want. You might want to use preg_split() and invert your pattern. It can take a limit on the number of splits. I think you would need a limit of 4, so you would get 3 words and the rest of the string. -
Can I also clarify that you want to get 2 items from the id list and insert both ids into one row of the new table?
-
Try this code: while($rows = mysql_fetch_array($Files)) { $current_file = $rows['File']; print "<table><tr><td>"; mysql_data_seek($Amendments, 0); while ($changes = mysql_fetch_array($Amendments)) { if ($changes['File'] == $current_file) { echo $changes['Reference'] . "</td><td>" . $changes['Text']; } } print "</td></tr></table>"; } Make sure you are selecting Reference from the table as well if you are going to try to display it.
-
I'm asking for this to be moved to the HTML or CSS forum. BTW you are including a full HTML page within another HTML page there - probably your included file should be a div or a table.
-
You will need to mysql_data_seek($Amendments, 0) before you run the inner loop each time. http://php.net/manual/en/function.mysql-data-seek.php Then you can reinstate the "if" that you had in your earlier code, to ensure that only changes related to the current file are displayed inside each table.
-
I've already posted the solution.
-
$query="SELECT EventStartTime EventEndTime DanceType FROM classSchedule WHERE EventStartTime >= "3:00" "; Here you are using double quotes inside a double quoted string. You can do it like this instead: $query="SELECT EventStartTime EventEndTime DanceType FROM classSchedule WHERE EventStartTime >= \"3:00\" ";
-
This is simple I know but I can't find it
btherl replied to calvinschools's topic in PHP Coding Help
This looks sus to me: $photo = $_FILES['photo']['partner_name']; -
You typically do not need to urldecode a value from $_GET, because a single urldecoding pass is applied by PHP automatically. You would only need to urldecode it if it got double url encoded in the first place. As for why it's not working, I will need to see the full code, or a small sample code which demonstrates the problem.